The JFrame class itself has only a few methods for changing how frames look. Of course, through the magic of inheritance, most of the methods for working with the size and position of a frame come from the various superclasses of JFrame. Among the most important methods are the following ones: The dispose method that closes the window and reclaims any system resources used in creating it; The setIconImage method, which takes an Image object to use as the icon when the window is minimized (often called iconized in Java terminology); The setTitle method for changing the text in the title bar; The setResizable method, which takes a boolean to determine if a frame will be resizeable by the user. Figure 7-5 illustrates the inheritance hierarchy for the JFrame class. Figure 7-5. Inheritance hierarchy for the JFrame and JPanel classes 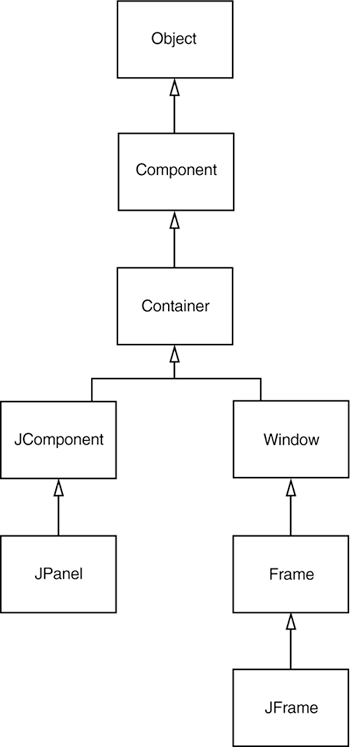
As the API notes indicate, the Component class (which is the ancestor of all GUI objects) and the Window class (which is the superclass of the Frame class) are where you need to look to find the methods to resize and reshape frames. For example, the setLocation method in the Component class is one way to reposition a component. If you make the call setLocation(x, y) the top-left corner is located x pixels across and y pixels down, where (0, 0) is the top-left corner of the screen. Similarly, the setBounds method in Component lets you resize and relocate a component (in particular, a JFrame) in one step, as setBounds(x, y, width, height) NOTE  | For a frame, the coordinates of the setLocation and setBounds are taken relative to the whole screen. As you will see in Chapter 9, for other components inside a container, the measurements are taken relative to the container. |
Remember: if you don't explicitly size a frame, all frames will default to being 0 by 0 pixels. To keep our example programs simple, we resize the frames to a size that we hope works acceptably on most displays. However, in a professional application, you should check the resolution of the user's screen and write code that resizes the frames accordingly: a window that looks nice on a laptop screen will look like a postage stamp on a high-resolution screen. As you will soon see, you can obtain the screen dimensions in pixels on the user's system. You can then use this information to compute the optimal window size for your program. TIP  | The API notes for this section give what we think are the most important methods for giving frames the proper look and feel. Some of these methods are defined in the JFrame class. Others come from the various superclasses of JFrame. At some point, you may need to search the API docs to see if there are methods for some special purpose. Unfortunately, that is a bit tedious to do with the JDK documentation. For subclasses, the API documentation only explains overridden methods. For example, the toFront method is applicable to objects of type JFrame, but because it is simply inherited from the Window class, the JFrame documentation doesn't explain it. If you feel that there should be a method to do something and it isn't explained in the documentation for the class you are working with, try looking at the API documentation for the methods of the superclasses of that class. The top of each API page has hyperlinks to the superclasses, and inherited methods are listed below the method summary for the new and overridden methods. |
To give you an idea of what you can do with a window, we end this section by showing you a sample program that positions one of our closable frames so that For example, if the screen was 800 x 600 pixels, we need a frame that is 400 x 300 pixels and we need to move it so the top left-hand corner is at (200,150). To find out the screen size, use the following steps. Call the static getdefaultToolkit method of the Toolkit class to get the Toolkit object. (The Toolkit class is a dumping ground for a variety of methods that interface with the native windowing system.) Then call the getScreenSize method, which returns the screen size as a Dimension object. A Dimension object simultaneously stores a width and a height, in public (!) instance variables width and height. Here is the code: Toolkit kit = Toolkit.getDefaultToolkit(); Dimension screenSize = kit.getScreenSize(); int screenWidth = screenSize.width; int screenHeight = screenSize.height; We also supply an icon. Because the representation of images is also system dependent, we again use the toolkit to load an image. Then, we set the image as the icon for the frame. Image img = kit.getImage("icon.gif"); setIconImage(img); Depending on your operating system, you can see the icon in various places. For example, in Windows, the icon is displayed in the top-left corner of the window, and you can see it in the list of active tasks when you press ALT+TAB. Example 7-2 is the complete program. When you run the program, pay attention to the "Core Java" icon. TIP  | It is quite common to set the main frame of a program to the maximum size. As of JDK 1.4, you can simply maximize a frame by calling frame.setExtendedState(Frame.MAXIMIZED_BOTH);
|
NOTE  | If you write an application that takes advantage of multiple display screens, you should use the GraphicsEnvironment and GraphicsDevice classes to find the dimensions of the display screens. As of JDK 1.4, the GraphicsDevice class also lets you execute your application in full-screen mode. |
Example 7-2. CenteredFrameTest.java 1. /**import java.awt.*; 2. import java.awt.event.*; 3. import javax.swing.*; 4. 5. public class CenteredFrameTest 6. { 7. public static void main(String[] args) 8. { 9. CenteredFrame frame = new CenteredFrame(); 10. frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); 11. frame.setVisible(true); 12. } 13. } 14. 15. class CenteredFrame extends JFrame 16. { 17. public CenteredFrame() 18. { 19. // get screen dimensions 20. 21. Toolkit kit = Toolkit.getDefaultToolkit(); 22. Dimension screenSize = kit.getScreenSize(); 23. int screenHeight = screenSize.height; 24. int screenWidth = screenSize.width; 25 26. // center frame in screen 27. 28. setSize(screenWidth / 2, screenHeight / 2); 29. setLocation(screenWidth / 4, screenHeight / 4); 30. 31. // set frame icon and title 32. 33. Image img = kit.getImage("icon.gif"); 34. setIconImage(img); 35. setTitle("CenteredFrame"); 36. } 37. } 
java.awt.Component 1.0 boolean isVisible() checks whether this component is set to be visible. Components are initially visible, with the exception of top-level components such as JFrame. void setVisible(boolean b) shows or hides the component depending on whether b is TRue or false. boolean isShowing() checks whether this component is showing on the screen. For this, it must be visible and be inside a container that is showing. boolean isEnabled() checks whether this component is enabled. An enabled component can receive keyboard input. Components are initially enabled. void setEnabled(boolean b) enables or disables a component. Point getLocation() 1.1 returns the location of the top-left corner of this component, relative to the top-left corner of the surrounding container. (A Point object p encapsulates an x- and a y-coordinate which are accessible by p.x and p.y.) Point getLocationOnScreen() 1.1 returns the location of the top-left corner of this component, using the screen's coordinates. void setBounds(int x, int y, int width, int height) 1.1 moves and resizes this component. The location of the top-left corner is given by x and y, and the new size is given by the width and height parameters. void setLocation(int x, int y) 1.1 void setLocation(Point p) 1.1 move the component to a new location. The x- and y-coordinates (or p.x and p.y) use the coordinates of the container if the component is not a top-level component, or the coordinates of the screen if the component is top level (for example, a JFrame). Dimension getSize() 1.1 gets the current size of this component. void setSize(int width, int height) 1.1 void setSize(Dimension d) 1.1 resize the component to the specified width and height. 
java.awt.Window 1.0 
java.awt.Frame 1.0 void setResizable(boolean b) determines whether the user can resize the frame. void setTitle(String s) sets the text in the title bar for the frame to the string s. void setIconImage(Image image) Parameters: | image | The image you want to appear as the icon for the frame |
void setUndecorated(boolean b) 1.4 removes the frame decorations if b is TRue. boolean isUndecorated() 1.4 returns true if this frame is undecorated. int getExtendedState() 1.4 void setExtendedState(int state) 1.4 get or set the window state. The state is one of Frame.NORMAL Frame.ICONIFIED Frame.MAXIMIZED_HORIZ Frame.MAXIMIZED_VERT Frame.MAXIMIZED_BOTH

java.awt.Toolkit 1.0 static Toolkit getDefaultToolkit() returns the default toolkit. Dimension getScreenSize() gets the size of the user's screen. Image getImage(String filename) loads an image from the file with name filename. |