The last example that we examine in this chapter is a simple chat application. This program shows how more than one browser session can be made to communicate. We use a simple example of a chat application in order to demonstrate some of the techniques shown in this chapter. Chapter 5, "Collections, Loops, and Iterators," will extend this simple chat program into a more complex example. When you first access the chat page, you will be prompted to enter your login identity. This is the name by which other users will know you. This login can be nearly anything you like. Once you enter your name, you will be taken to the main chat page. (See Figures 3.2 and 3.3.) 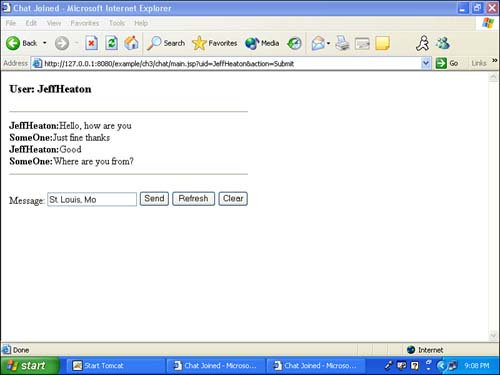 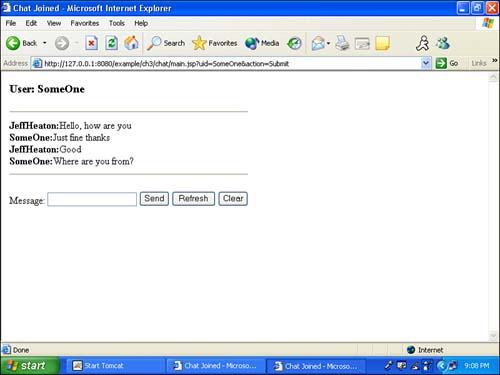 The login page for the chat application is a typical HTML form. This file appears in Listing 3.9. Listing 3.9 Our Chat Login (index.html)<html> <head> <meta http-equiv="Content-Language" content="en-us" /> <title>Simple Chat Application</title> </head> <body> <form action="main.jsp"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="33%" > <tbody> <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font size="4" color="#FFFFFF">Chat Login</font> </b> </p> </td> </tr> <tr> <td width="23%">User ID</td> <td width="77%"> <input type="text" name="uid" size="20" /> </td> </tr> <tr> <td width="100%" colspan="2"> <p align="center"> <input type="submit" value="Submit" name="action" /> <input type="reset" value="Reset" /> </p> </td> </tr> </tbody> </table> <p> </p> </form> <p> <i>Note: You may use any User ID you wish</i> </p> </body> </html> Most of the action for this program takes place on the main chat screen. Here, you have three options. First, you can send your message. This will make your message visible to the other users, including yourself. Second, you can refresh your view of the screen. As other users enter messages, they will not immediately be visible to you. If you wish to update your screen without sending a message, you must click the Refresh button. Third, a Clear button is provided that gives you the ability to clear the display. Because messages never scroll off the chat, clicking Clear lets you start from a clean slate. Listing 3.10 shows the source code for the main chat screen. Listing 3.10 Our Main Chat Page (main.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Chat Joined</title> </head> <body> <c:if test="${pageContext.request.method=='POST'}"> <c:choose> <c:when test="${param.send!=null}"> <c:set var="chat" value="${chat}<b>${param.uid}:</b>${param.text}<br />" scope="application" /> </c:when> <c:when test="${param.clear!=null}"> <c:set var="chat" value="" scope="application" /> </c:when> </c:choose> </c:if> <table border="0"> <tbody> <tr> <td> <h3>User: <c:out value="${param.uid}" /> </h3> <hr /> </td> </tr> <tr> <td> <c:out value="${chat}" escapeXml="false" /> </td> </tr> <tr> <td> <hr /> <form method="post">Message: <input type="text" name="text" size="20" /> <input type="submit" name="send" value="Send" /> <input type="submit" name="refresh" value="Refresh" /> <input type="submit" name="clear" value="Clear" /> <input type="hidden" name="uid" value="<c:out value="${param.uid}"/>" /> <br /> </form> </td> </tr> </tbody> </table> </body> </html> This program does not store the username in a session-scoped variable, as previous examples have. Instead, the username is stored as a hidden form field that is posted back to the main chat page every time you click a button. The contents of the chat are stored in an ever-increasing application-scoped variable. When you click the Send button, the message you just typed is added to this application-scoped variable via the following: <c:set var="chat" value="${chat}<b>${param.uid}:</b>${param.text}<br />" scope="application" /> This code concatenates the new message onto the messages that already exist in the application-scoped variable. Because the chat message is stored at the application level, the only way to clear the chat area is to either restart the Web server or click the Clear button. Clicking Clear executes the following line of code, which clears the chat application-scoped variable: <c:set var="chat" value="" scope="application" /> Clicking the Refresh button simply redraws the window without adding any messages. The chat area, when redrawn, is displayed with the following line of code: <c:out value="${chat}" escapeXml="false" /> You will notice that a property called escapeXml is set to false. Without this property set to false, JSTL would automatically encode the HTML tags that we are adding to the chat. This would be bad because the program would display these tags rather than processing them. For example, the HTML tag <br/> would be encoded to be <br/> this would prevent our formatting effects from displaying. In summary, the chat application is a simple JSTL application that makes use of some of the most basic tags in the JSTL tag library. The primary purpose of these tags is to control the flow of information through the chat application. This flow of information begins with the form, where users enter their message. The form is then posted back to itself, and <c:choose> and <c:when> tags are used to see if a conversation already exists. Then a <c:set> tag is used to store the conversation to an application-scoped variable so that all sessions can see the conversation. Finally, this conversation is presented to the user along with a form the user can use to add to the conversation. This completes the cycle. |