Another method of making data available to multiple pages of a Web site is to use sessions. The premise of a session is that data is stored on the server, not in the Web browser, and a session identifier is used to locate a particular user's record (session data). This session identifier is normally stored in the user's Web browser via a cookie, but the sensitive data itselflike the user's ID, name, and so onalways remains on the server. The question may arise: why use sessions at all when cookies work just fine? First of all, sessions are more secure in that all of the recorded information is stored on the server and not continually sent back and forth between the server and the client. Second, some users reject cookies or turn them off completely. Sessions, while designed to work with a cookie, can function without them, as you'll see in the next section of this chapter. To demonstrate sessionsand to compare them with cookiesI will rewrite the previous scripts. Setting session variables The most important rule with respect to sessions is that each page that will use them must begin by calling the session_start() function. This function tells PHP to either begin a new session or access an existing one. The first time this function is used, session_start() will attempt to send a cookie with a name of PHPSESSID (the session name) and a value of something like a61f8670baa8e90a30c878df89a2074b (32 hexadecimal letters, the session ID). Because of this attempt to send a cookie, session_start() must be called before any data is sent to the Web browser, as is the case when using the setcookie() and header() functions. Once the session has been started, values can be registered to the session using $_SESSION['key'] = 'value'; $_SESSION['name'] = 'Jessica'; $_SESSION['id'] = 48; I'll rewrite the login.php script with this in mind. Allowing for Sessions on Windows Sessions in PHP requires a temporary directory on the server where PHP can store the session data. For Unix and Mac OS X users, this isn't a problem, as the /tmp directory is available explicitly for purposes such as this. For Windows users, you also do not need to do anything special as of version 4.3.6 of PHP. But if you are running Windows and an earlier version of PHP, you must configure the server. Here's how: 1. | Create a new folder on your server, such as C:\temp.
| 2. | Make sure that Everyone (or just the Web server user, if you know that value) can read and write to this folder.
| 3. | Edit your php.ini file (see Appendix A, "Installation"), setting the value of session.save_path to this folder (C:\temp).
| 4. | Restart the Web server.
| If you see errors about the session.save_path when you first use sessions, pay attention to what the error messages say. Also double-check your path name and that you edited the correct php.ini file. |
To begin a session 1. | Open login.php (refer to Script 9.3) in your text editor.
| 2. | Replace the setcookie() lines (3637) with these lines (Script 9.6):
session_start(); $_SESSION['user_id'] = $row[0]; $_SESSION['first_name'] = $row[1]; Script 9.6. The login.php script now uses sessions instead of cookies. 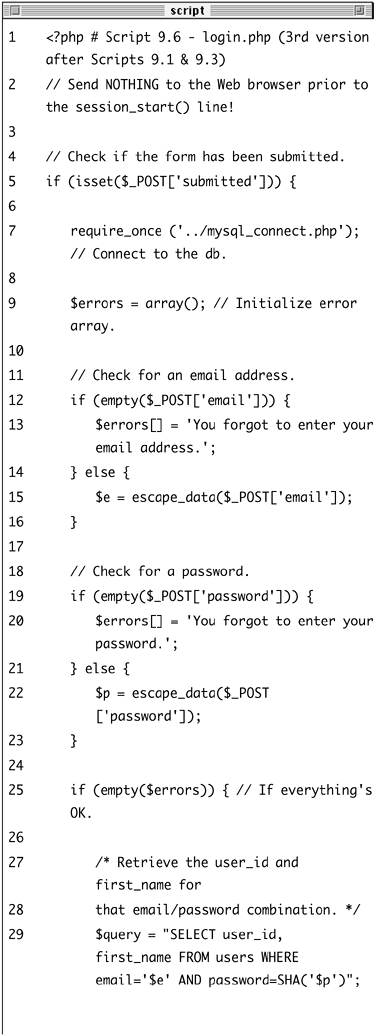 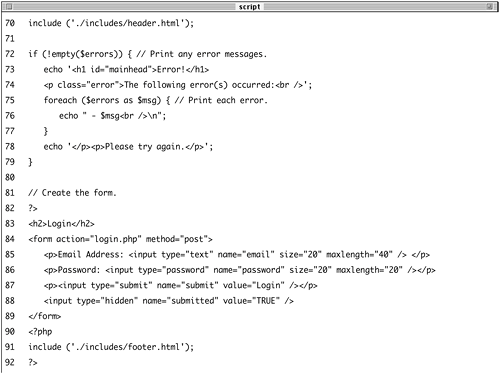 The first step is to begin the session. Since there are no echo() statements, include calls, or HTML prior to this point in the script, it will be safe to use session_start() now, although I could have placed it at the top of the script as well. Then, I add two key-value pairs to the $_SESSION superglobal array to register the user's first name and user ID to the session.
Sessions in Older Versions of PHP Prior to version 4.1 of PHP (when the $_SESSION superglobal became available), session variables were set using the special session_register() function. The syntax was session_start(); $name = 'Jessica'; session_register('name'); It's very important to notice that the session_register() function takes the name of a variable to register without the initial dollar sign (so name rather than $name). Once a session variable is registered, you can refer to is using $HTTP_SESSION_VARS['var']. To delete a session variable, you use the session_unregister() function. To repeat, you only need to use these functions if you are using an old version of PHP (between 4.0 and 4.1). As always, see the PHP manual for more information on these functions. |
| 3. | Save the page as login.php, upload to your server, and test in your Web browser (Figure 9.13).
Figure 9.13. The login form remains unchanged to the end user, but the underlying functionality now uses sessions. 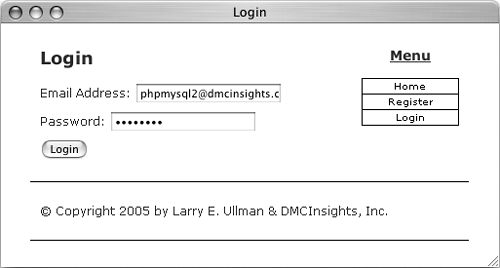 Although loggedin.php and the header and script will need to be rewritten, you can still test the login script and see the resulting cookie (Figure 9.14). The loggedin.php page should redirect you back to the home page, though, as it's still checking for the presence of a $_COOKIE variable.
Figure 9.14. This cookie, created by PHP's session_start() function, stores the session ID. 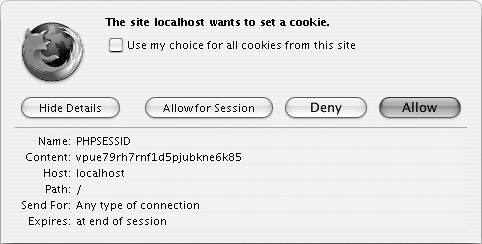
| Tips Because sessions will normally send and read cookies, you should always try to begin them as early in the script as possible. Doing so will help you avoid the problem of attempting to send a cookie after the headers (HTML or white space) have already been sent (see Figure 9.1). If you want, you can set session.auto_start in the php.ini file to 1, making it unnecessary to use session_start() on each page. This does put a greater toll on the server and, for that reason, shouldn't be used without some consideration of the circumstances. You can store arrays in sessions (making $_SESSION a multidimensional array), just as you can strings or numbers.
Accessing session variables Once a session has been started and variables have been registered to it, you can create other scripts that will access those variables. To do so, each script must first enable sessions, again using session_start(). This function will give the current script access to the previously started session (if it can read the PHPSESSID value stored in the cookie) or create a new session if it cannot (in which case, it won't be able to access stored values because a new session will have been created). To then refer to a session variable, use $_SESSION['var'], as you would refer to any other array. To access session variables 1. | Open loggedin.php (refer to Script 9.2) in your text editor.
| 2. | Add a call to the session_start() function (Script 9.7).
session_start(); Script 9.7. I've updated loggedin.php so that it refers to $_SESSION and not $_COOKIE. 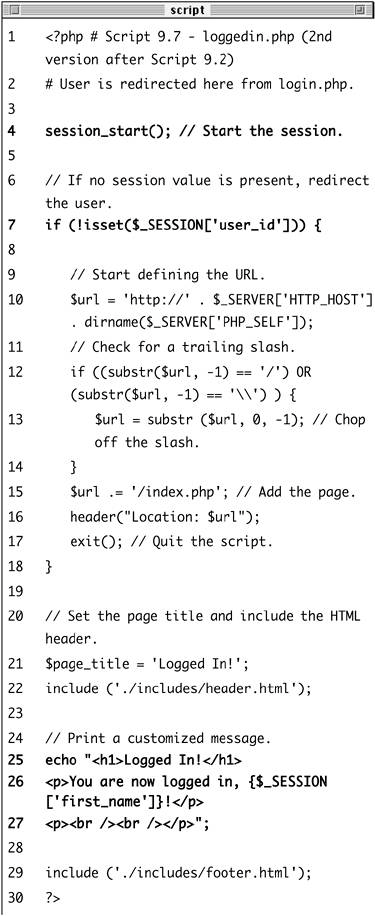
Every PHP script that either sets or accesses session variables must use the session_start() function. This line must be called before the header.html file is included and before anything is sent to the Web browser.
| 3. | Replace the references to $_COOKIE with $_SESSION (lines 5 and 24 of the original file).
if (!isset($_SESSION['user_id'])) { and
echo "<h1>Logged In!</h1> <p>You are now logged in, {$_SESSION ['first_name]}!</p> <p><br /><br /></p>"; Switching a script from cookies to sessions requires only that you change uses of $_COOKIE to $_SESSION.
| 4. | Save the file as loggedin.php, upload to your Web server, and test in your browser (Figure 9.15).
Figure 9.15. After logging in, the user is redirected to loggedin.php, which will welcome the user by name using the stored session value. 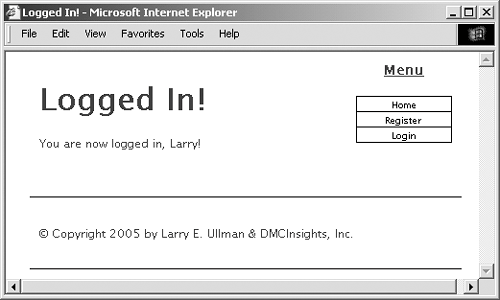
| 5. | Replace the reference to $_COOKIE with $_SESSION in header.html (from Script 9.5 to Script 9.8).
if ( (isset($_SESSION['user_id'])) && (!strpos($_SERVER['PHP_SELF], 'logout.php)) ) { Script 9.8. The header.html file now also references $_SESSION. 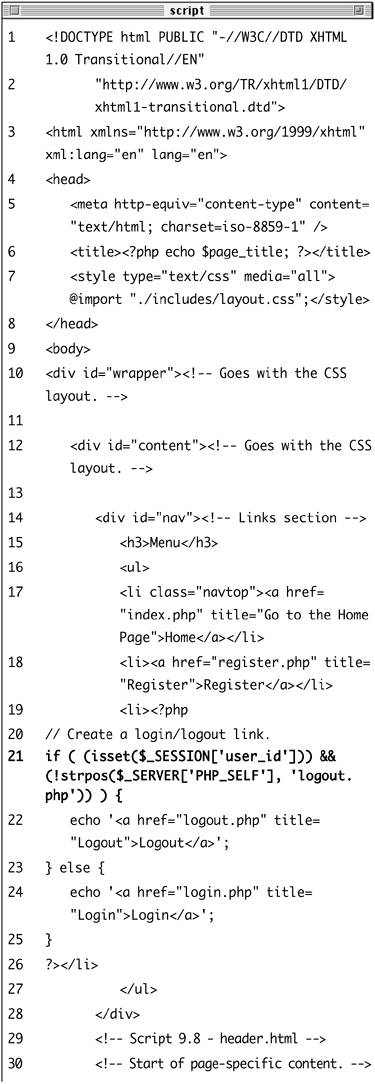
For the Login/Logout links to function properly (notice the incorrect link in Figure 9.15), the reference to the cookie variable within the header file must be switched over to sessions. The header file does not need to call the session_start() function, as it'll be included by pages that do.
| 6. | Save the header file, upload to the Web server, and test in your browser (Figure 9.16).
Figure 9.16. With the header file altered for sessions, the proper Login/Logout links will be displayed (compare with Figure 9.15). 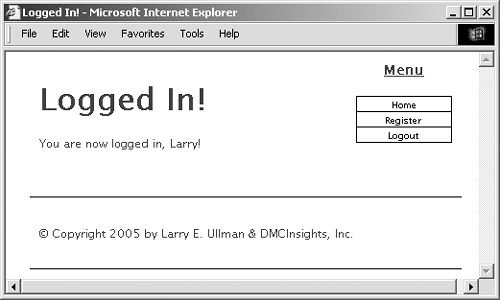
| Tips For the Login/Logout links to work on the other pages (register.php, index.php, etc.), you'll need to add the session_start() command to each of those. If you have an application where the session data does not seem to be accessible from one page to the next, it could be because a new session is being created on each page. To check for this, compare the session ID (the last few characters of the value will suffice) to see if it is the same. You can see the session's ID by viewing the session cookie as it is sent or by using the session_id() function: echo session_id();
Session variables are available as soon as you've established them. So, unlike when using cookies, you can assign a value to $_SESSION['var'] and then refer to $_SESSION['var'] later in that same script.
Deleting session variables When using sessionsparticularly with a login/logout system as I've established hereyou need to create a method to delete the session variables. In the current example, this would be necessary when the user logs out. Whereas a cookie system only requires that another cookie be sent to destroy the existing cookie, sessions are more demanding, since there are both the cookie on the client and the data on the server to consider. To delete an individual session variable, you can use the unset() function (which works with any variable in PHP): unset($_SESSION['var']); To delete every session variable, reset the entire $_SESSION array: $_SESSION = array(); Finally, to remove all of the session data from the server, use session_destroy(): session_destroy(); Note that prior to using any of these methods, the page must begin with session_start() so that the existing session is accessed. To delete a session 1. | Create a new PHP script in your text editor (Script 9.9).
<?php # Script 9.9 - logout.php Script 9.9. Destroying a session requires special syntax. 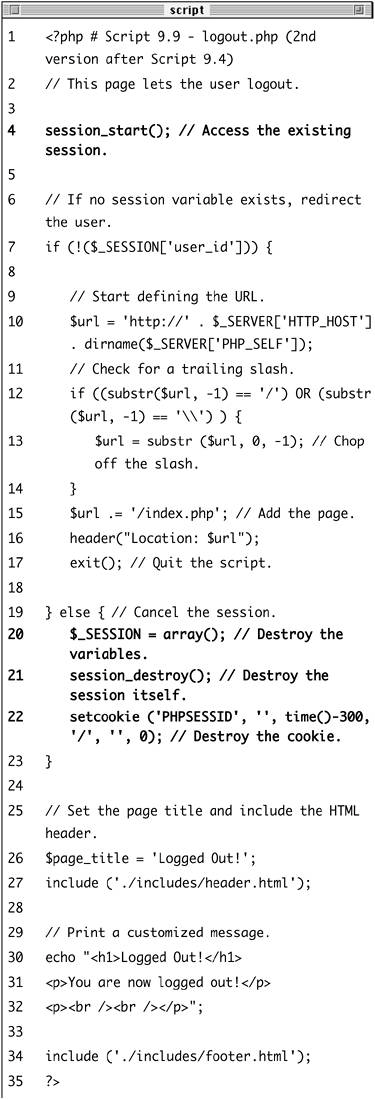
The logout script will log out the user and delete all the session information.
| 2. | Invoke the session.
session_start(); Anytime you are using sessions, you must use the session_start() function, preferably at the very beginning of a page. This is true even if you are deleting a session.
| 3. | Check for the presence of the $_SESSION['user_id'] variable.
if (!($_SESSION['user_id'])) { $url = 'http://' . $_SERVER ['HTTP_HOST] . dirname($_SERVER ['PHP_SELF]); if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); } $url .= '/index.php'; header("Location: $url"); exit(); As with the logout.php script in the cookie examples, if the user is not currently logged in, he or she will be redirected.
| 4. | Destroy all of the session material.
} else { $_SESSION = array(); session_destroy(); setcookie ('PHPSESSID', '', time()-300, '/', '', 0); } The second line here will reset the entire $_SESSION variable as a new array, erasing its existing values. The third line removes the data from the server, and the fourth sends a cookie to replace the existing session cookie in the browser.
| 5. | Create the HTML and print a message.
$page_title = 'Logged Out!'; include ('./includes/header.html'); echo "<h1>Logged Out!</h1> <p>You are now logged out!</p> <p><br /><br /></p>"; include ('./includes/footer.html'); ?> Unlike when using the cookie logout.php script, you cannot refer to the user by their first name anymore, as all of that data has been deleted.
| 6. | Save the file as logout.php, upload to your Web server, and test in your browser (Figure 9.17).
Figure 9.17. The logout page. 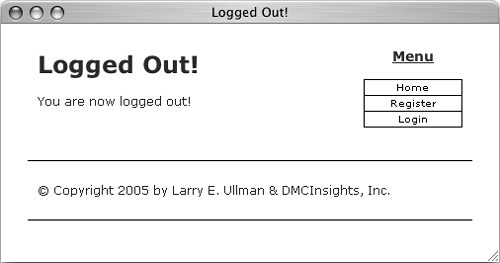
| Tips If you are using an older version of PHP (prior to version 4.1) and the $_SESSION array is not available, use session_unset() in lieu of $_SESSION = array(). Never set $_SESSION equal to NULL, because that could cause problems on some servers. To delete just one session variable, use unset($_SESSION['var']).
Changing the session behavior As part of PHP's support for sessions, there are about 20 different configuration options you can set for how PHP handles sessions. Table 9.1 lists the most important of these. Table 9.1. PHP's session configuration options, with the default setting listed as most of the examples.Session Configuration Settings |
---|
SETTING | EXAMPLE | MEANING |
---|
session.auto_start | 0 | If sessions should be automatically used (0 means no). | session.cookie_domain | www.dmcinsights.com | The URL wherein the session cookie should be accessible. | session.cookie_lifetime | 0 | How long, in seconds, the session cookie should exist (0 means for the life of the browser). | session.cookie_path | / | The domain path wherein the cookie should be accessible. | session.cookie_secure | 0 | Whether or not the cookie must be sent over a secure connection (0 means no). | session.gc_probability | 1 | The odds of performing garbage collection from 1 to 100. | session.gc_maxlifetime | 1440 | The time period in seconds a session should last. | session.name | PHPSESSID | The name given to all sessions. | session.save_handler | files | How the session data will be stored. | session.save_path | /tmp | Where session data will be stored. | session.serialize_handler | php | What method should be used to serialize the session variables. | session.use_cookies | 1 | Whether or not the session ID should be stored in a cookie (0 means no). | session.use_only_cookies | 0 | Whether or not the session ID must be stored in a cookie (0 means no). | session.use_trans_sid | 0 | Whether or not PHP should add the session ID to every link in an application (0 means no). |
Each of these settings, except for session.use_trans_sid, can be set within your PHP script using the ini_set() function (covered in the preceding chapter): ini_set (parameter, new_setting); For example, to change where PHP stores the session data, use ini_set ('session.save_path', '/to/folder'); To set the name of the session (perhaps to make a more user-friendly one), you can use either ini_set() or the simpler session_name() function. session_name('YourSession'); The benefits of creating your own session name are twofold: it's marginally more secure and it may be better received by the end user (since the session name is the cookie name the end user will see). That being said, for session_name() to work, it must be called before every use of session_start() in your entire Web application. I'll rewrite the example with this in mind. To use your own session names 1. | Open login.php (refer to Script 9.6) in your text editor.
| 2. | Before the session_start() call (line 36), add the following (Script 9.10):
session_name ('YourVisitID'); Script 9.10. The login.php script now uses an original session name. 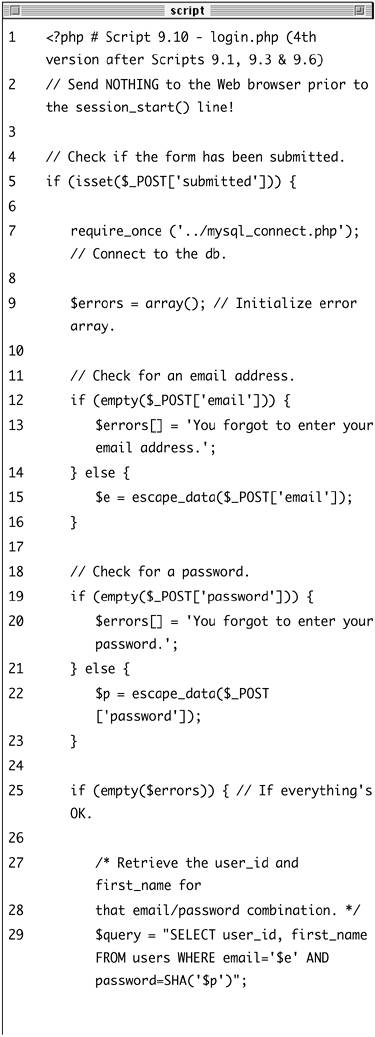 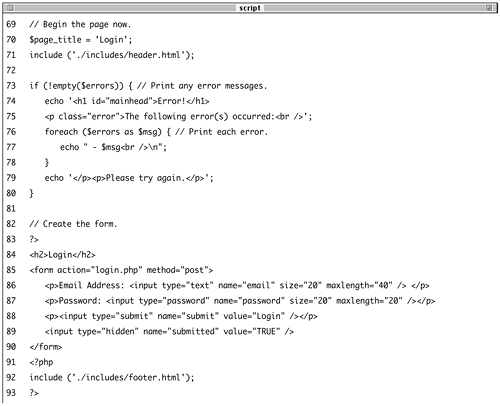 Instead of having the session be named PHPSESSID, which may be imposing as a cookie name, I'll use the friendlier YourVisitID.
| 3. | Repeat the process for loggedin.php (compare Script 9.7 with Script 9.11).
Script 9.11. The same session name (YourVisitID) must be used across every script. 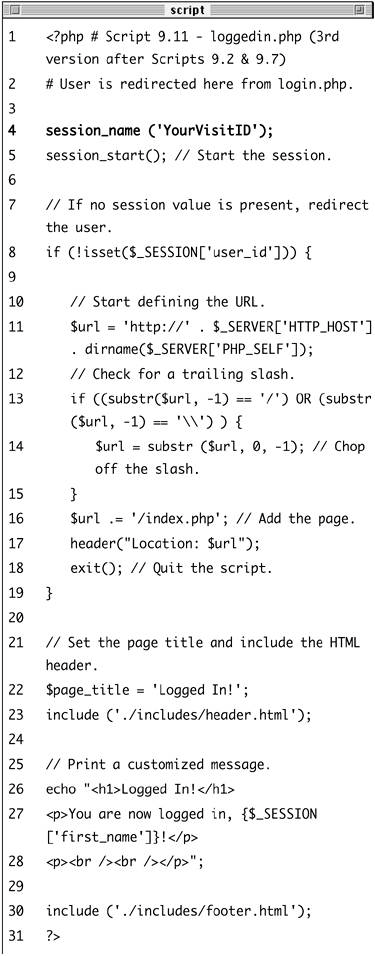
Because every page must use the same session name, this line of code has to be added to the loggedin.php and logout.php scripts for them to work properly.
| 4. | Add the following line to logout.php (compare Script 9.9 with Script 9.12):
session_name ('YourVisitID'); Script 9.12. The logout.php page uses the session_name() function to also determine the name of the cookie to be sent. 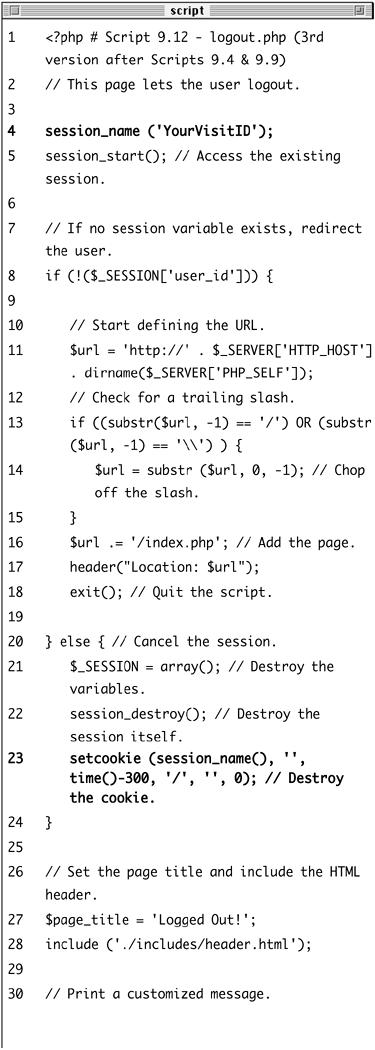 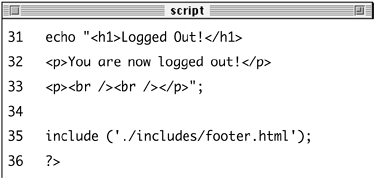
| 5. | Change the setcookie() line of logout.php so that it uses the session_name() function:
setcookie (session_name(), '', time()-300, '/', '', 0); The session_name() function will set the session name or return the current session name (if no argument is given). Since I want to send a cookie using the same cookie name as was used to create the cookie, the session_name() function will set that value appropriately.
| 6. | Save all the files, upload to your Web server, and test in your Web browser.
| 7. | If desired, view the cookie that was set during the login process (Figure 9.18).
Figure 9.18. The cookie's name will correspond to the session name. 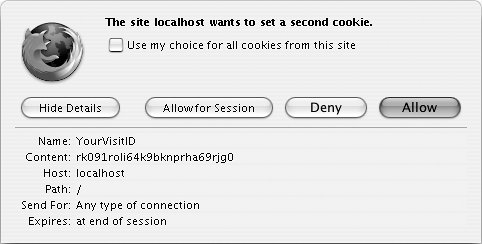
| |