In the previous examples I've accomplished the same tasks (logging in and logging out) using cookies and sessions. Obviously, both are easy to use in PHP, but the true question is when to use one or the other. Sessions have the following advantages over cookies: They are generally more secure (because the data is being retained on the server). They allow for more data to be stored. They can be used without cookies. Whereas cookies have the following advantages over sessions: In general, to store and retrieve just a couple of small pieces of information, use cookies. For most of your Web applications, though, you'll use sessions. But since sessions do rely upon cookies by default, I'll discuss how to better manage this relationship. Changing the session cookie settings As it stands, the cookie sent by the session_start() function uses certain default parameters: an expiration of 0 (meaning the cookie will last as long as the browser remains open), a path of '/' (the cookie is available in the current folder and all of its subfolders), and no domain name. To change any of these settings, you can use the session_set_cookie_params() function: session_set_cookie_params(expiration, 'domain', secure); The expiration setting is the only required value and is set in seconds with 0 as the default. This is not the number of seconds from the epoch (as is the case with the setcookie() function), and therefore you would use just 300 (for five minutes) rather than time() + 300 (for five minutes from now). To change the session cookie settings 1. | Open login.php (refer to Script 9.10) in your text editor.
| 2. | Prior to the session_start() call (line 37), add the following (Script 9.13):
session_set_cookie_params (900, '/ch09/', 'www.domain.com); Script 9.13. This version of the login.php script sets explicit cookie parameters. 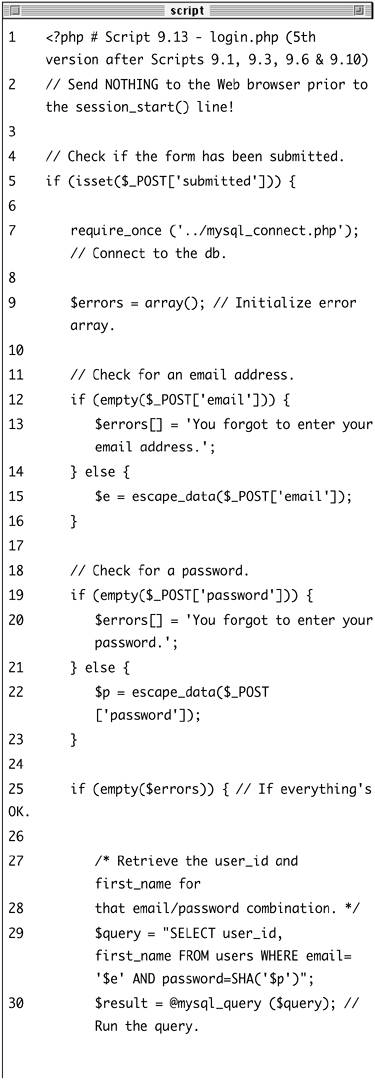 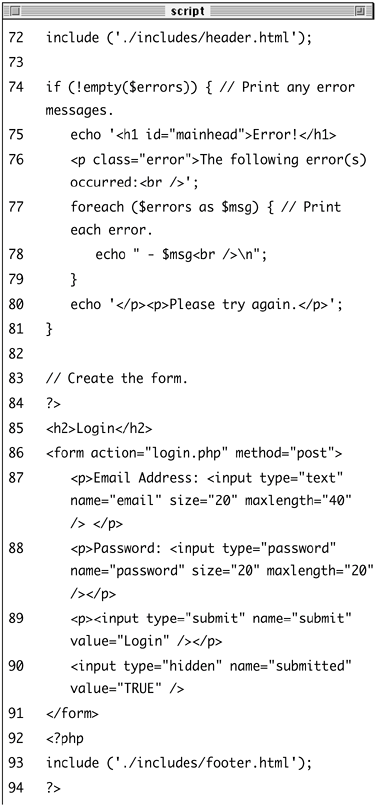
The session_set_cookie_params() function must be used before session_start() to be effective. Change the path and domain setting to those values that make sense for your application, or omit the values to use the defaults. In this example, I'll give the cookie an expiration time that's 15 minutes from now.
| 3. | Save the file as login.php, upload to your Web server, and test in your browser.
After 15 minutes, the cookie will expire and the PHP scripts should no longer be able to access the session values (first_name and user_id).
| 4. | View the cookie being sent (Figure 9.19).
Figure 9.19. The session cookie now has an expiration time set. 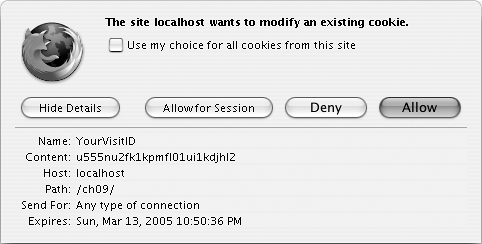
| 5. | Alter loggedin.php and logout.php so that the setcookie() function uses the same parameters as login.php (except for the expiration time on the logout page, of course).
| Tips The session_get_cookie_params() function returns an array of the current session cookie settings. The session cookie parameters can also be altered using the ini_set() function. The expiration time of the cookie refers only to the longevity of the cookie in the Web browser, not to how long the session data will be stored on the server.
Using sessions without cookies One of the problems with sessions is that, by default, they rely on the use of a cookie to work properly. When a session is started, it sends a cookie that resides in the user's Web browser. Every subsequent page that calls session_start() makes use of the cookie, which contains the session name and ID, to know to use an existing session and to not create a new one. The problem is that users may have cookies turned off in their Web browser or may not accept the cookie because they do not understand its purpose. If this is the case, PHP will create a new session for each page and none of the registered variables will be accessible. Garbage Collection Garbage collection with respect to sessions is the process of deleting the session files (where the actual data is stored). Creating a logout system that destroys a session is ideal, but there's no guarantee all users will formally log out as they should. For this reason, PHP includes a cleanup process. Whenever the session_start() function is called, PHP's garbage collection kicks in, checking the last modification date of each session (a session is modified whenever variables are set or retrieved). The server overhead of all this can become costly for busy sites, so you can tweak PHP's behavior in this regard. Two settings dictate garbage collection: session_gc_maxlifetime and session.gc_probability. The first states after how many seconds of inactivity a session is considered idle and will therefore be deleted. The second setting determines the probability that garbage collection is performed, on a scale of 1 to 100. So, with the default settings, each call to session_start() has a 1 percent chance of invoking garbage collection. If PHP does start the cleanup, any sessions that have not been used in more than 1,440 seconds will be deleted. With this in mind, you can alter PHP's garbage collection habits to better suit your application. Twenty-four minutes is a reasonable amount of idle time, but you'll want to increase the probability to somewhere closer to 30 percent so that there is a good balance between performance and clutter. |
You can use sessions without cookies by passing along the session name and ID from page to page. This is simple enough to do, but if you forget to pass the session in only one instance, the entire process is shot. To pass the session name from page to page, you can use the SID constant, which stands for session ID and has a value like session_name=session_ID. If this value is appended to every URL within the site, the sessions will still work even if the user did not accept the cookie. Note, though, that PHP only assigns a value to SID if no session cookie is present. To use sessions without cookies 1. | Open login.php (refer to Script 9.13) in your text editor.
| 2. | Replace the session_set_cookie_params() line with this one (Script 9.14):
ini_set('session.use_cookies', 0); Script 9.14. This version of the login.php script does not use cookies at all, instead maintaining the state by passing the session ID in the URL. 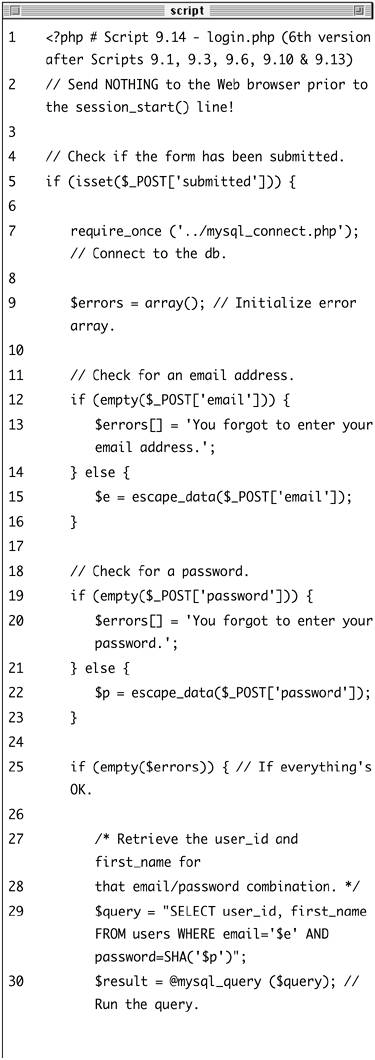 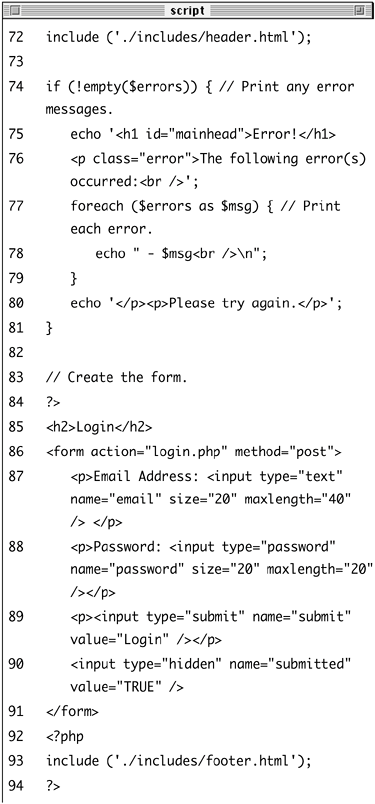
This code will tell PHP to specifically not use any cookies.
| 3. | Alter the final $url creation line to be
$url .= '/loggedin.php?' . SID; The addition of ? and SID to the redirect will add ?session_name=session_ID to the URL, effectively passing the session ID to the loggedin.php script.
| 4. | Save the file, upload to your Web server, and test in your Web browser (Figure 9.20).
Figure 9.20. When the browser is redirected to the loggedin.php page, the session name and ID will be appended to the URL. 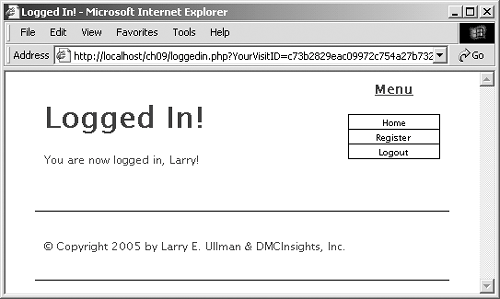
| 5. | Copy the URL from the browser and paste it into another browser (Figure 9.21).
Figure 9.21. By using an existing session ID in a new browser, I can hijack another user's session and have access to all of that user's registered session data. 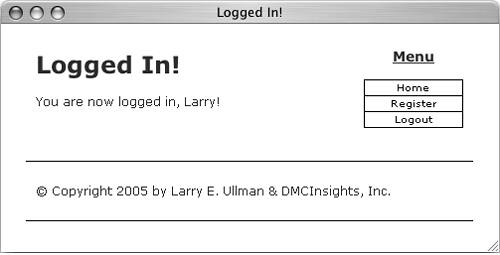
Called session hijacking, this is one of the reasons to rely upon cookies whenever possible. The next section of this chapter will introduce preventive measures.
| To spare you from having to review minor modifications to the same scripts yet again (and to save precious book space), I am not including new versions of header.html, loggedin.php, and logout.php. But since you should know how to edit these files in order to use sessions without cookies, I'll quickly outline the necessary changes. To edit the other files 1. | Edit header.html so that every link includes ?session_name=session_ID (script not shown).
The other problem with using sessions without cookies (besides the security issue) is that you must account for every link in your entire application. To modify the header file, you'll need to define the links like so:
<a href="index.php?<?php echo SID; ?>" title="Go to the Home Page>Home</a>
| 2. | Edit loggedin.php so that it also includes the ini_set() line.
This script would then begin like so:
session_name ('YourVisitID'); ini_set('session.use_cookies', 0); session_start(); You would also need to do this step for any other page that uses sessions.
| 3. | Make the same changes to logout.php.
| 4. | Remove the setcookie() line from logout.php.
Since a session cookie is no longer being used, there is no reason to set another cookie deleting it.
| Tips If you have access to your php.ini file, you can set session.use_trans_sid to 1 or On. Doing so will have PHP automatically append SID to every URL as you have done manually here. It will slow down execution of the scripts, though, because PHP will need to check every page for URLs. The session_id() function returns the current session value (or allows you to specify which session to use). You can also pass SID from one page to another by storing it as a hidden input type in a form. Depending on the Web browser being used by the client, a session may be either browser-specific or window-specific. If the latter is the case, a pop-up window in your site will not be part of the same session unless it has received the session ID. Remember that using this method of storing and passing the session ID is less secure than using cookies for that purpose. If security isn't really a concern for your Web site (for example, if you're not dealing with personal information or e-commerce), then this is less of an issue.
|