Switching gears, I want to conclude this chapter by discussing how you can use HTTP headers in your application. HTTP (Hypertext Transfer Protocol) is the technology at the heart of the World Wide Web because it defines the way clients and servers communicate (in layman's terms). When a browser requests a Web page, it receives a series of HTTP headers in return. PHP's built-in header() function can be used to take advantage of this protocol. The most common example of this will be demonstrated here, when the header() function will be used to redirect the Web browser from the current page to another. However, in Chapter 12, "ExampleContent Management," you'll use it to send files to the Web user. To use header() to redirect the Web browser, type header ('Location: http://www.url.com/ page.php); Because this should be the last thing to occur on the current page (since the browser will soon be leaving it), this line would normally be followed by a call to the exit() function, which stops execution of the current script. The absolutely critical thing to remember about the header() function is that it must be called before anything is sent to the Web browser. This includes HTML or even blank spaces. If your code has any echo() or print() statements, has blank lines outside of PHP tags, or includes files that do any of these things before calling header(), you'll see an error message like that in Figure 8.17. Figure 8.17. The headers already sent error is a common occurrence when learning how to use the header() function. 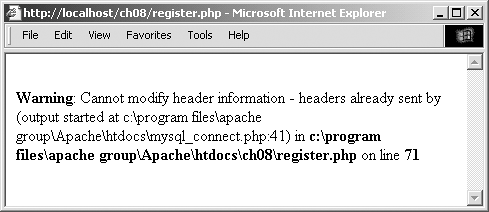
You can avoid this problem using the headers_sent() function, which checks whether or not data has been sent to the Web browser. if (!headers_sent()) { header ('Location: http://www.url. com/page.php); exit(); } else { echo 'Wanted to redirect you but I could not do it!'; } I'll rewrite the registration script (from previous chapters) so that the user is redirected to a thank-you page upon successful registration. To do so will require that I restructure the page to ensure that no text is sent to the browser before I call header(). Also, since header() calls should use an absolute URL (http://www.somesite.com/page.php) instead of a relative one (page.php), the $_SERVER variable will be used to dynamically determine the full redirection URL (see the sidebar on page 321 "Using Absolute URLs with header()"). To use the header() function 1. | Create a new PHP page in your text editor or IDE (Script 8.7).
<?php # Script 8.7 - register.php Script 8.7. The registration script will now redirect the user to a Thank You page once they've successfully registered. Doing so requires that nothing be sent to the Web browser prior to invoking the header() function. 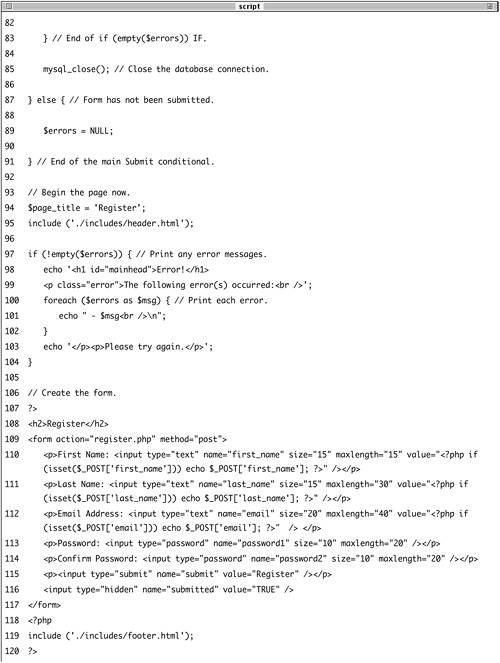
| 2. | Check if the form has been submitted.
if (isset($_POST['submitted'])) { Notice that I am not including the HTML header before this line as I have in previous examples. I need the script to be able to redirect the user once they've registered, so I cannot include the header file (sending its HTML to the Web browser) beforehand.
| 3. | Include the MySQL connection script and initialize the $errors array.
require_once ('../mysql_connect. php); $errors = array(); I no longer need to define the escape_data() function in this script, as it is now defined within mysql_connect.php.
| 4. | Validate the form inputs.
if (empty($_POST['first_name'])) { $errors[] = 'You forgot to enter your first name.'; } else { $fn = escape_data($_POST['first_ name]); } if (empty($_POST['last_name'])) { $errors[] = 'You forgot to enter your last name.'; } else { $ln = escape_data($_POST['last_ name]); } if (empty($_POST['email'])) { $errors[] = 'You forgot to enter your email address.'; } else { $e = escape_data($_POST['email']); } if (!empty($_POST['password1'])) { if ($_POST['password1'] != $_POST ['password2]) { $errors[] = 'Your password did not match the confirmed password.'; } else { $p = escape_data($_POST ['password1]); } } else { $errors[] = 'You forgot to enter your password.'; } This entire process is exactly the same as it has been in other registration examples.
| 5. | If there were no registration errors, check if the email address has already been registered, and then run the INSERT query.
if (empty($errors)) { $query = "SELECT user_id FROM users WHERE email='$e"; $result = mysql_query($query); if (mysql_num_rows($result) == 0) { $query = "INSERT INTO users (first_name, last_name, email, password, registration_ date) VALUES ('$fn, '$ln', '$e, SHA('$p'), NOW() )"; $result = @mysql_query ($query); if ($result) { Again, there's nothing new here. Refer to previous register.php scripts in Chapter 7 if you're confused by any of this code.
| 6. | Dynamically determine the redirection URL and then call the header() function.
$url = 'http://' . $_SERVER['HTTP_ HOST] . dirname($_SERVER['PHP_ SELF]); if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); } $url .= '/thanks.php'; header("Location: $url"); exit(); Before calling the header() function, I need to determine the absolute URL (you can just make $url equal to thanks.php, but it's better to be absolute). To start, I assign $url the value of http:// plus the host name (which could be either localhost or www.dmcinsights.com). To this I add the name of the current directory using the dirname() function, in case the redirection is taking place within a subfolder.
Because the existence of a subfolder might add an extra slash (/) or backslash (\, for Windows), I have to check for and remove this. To do so, I use the substr() function to see if the last character in $url is either / or \. But since the backslash is the escape character in PHP, I need to use \\to refer to a single backslash. If $url concludes with either of these characters, the substr() function is then called again to assign $url the value of $url minus this last character. Finally, the specific page name is appended to the $url. This may all seem to be quite complicated, but it's a very effective way to ensure that the redirection works no matter on what server, or from what directory, the script is being run.
The header() function will then send the user to a thank-you page if everything went OK. Then the script is exited, which means that everything else in the script will not be executed.
| 7. | Complete the INSERT, email, and $errors conditionals.
} else { $errors[] = 'You could not be registered due to a system error. We apologize for any inconvenience.'; $errors[] = mysql_error() . '<br /><br />Query: ' . $query; } } else { $errors[] = 'The email address has already been registered.'; } } In previous versions of this script, these errors would immediately be printed. But since the HTML header has not yet been included, that's not an option. So instead, the existing $errors array is used to handle all errors, even system ones.
| 8. | Close the database connection and complete the submission conditional.
mysql_close(); } else { $errors = NULL; } The else clause takes effect if the page has not yet been submitted. In that case, I need to indicate that there are no errors, which I do by initializing that variable with a NULL value.
| 9. | Include the HTML header.
$page_title = 'Register'; include ('./includes/header.html'); After the main conditional and once the header() function would have been called, it's now safe to use the included file.
| 10. | Print out any error messages.
if (!empty($errors)) { echo '<h1 >Error! </h1> <p >The following rror(s) occurred:<br />'; foreach ($errors as $msg) { echo " - $msg<br />\n"; } echo '</p><p>Please try again. </p>'; } Web users will be seeing this page under two circumstances: once when they first arrive and again if they fail to fill out the form completely. Under this second situation, the $errors variable will have a value (of what went wrong) and its values will be printed here, above the form.
| 11. | Create the HTML form and complete the PHP page.
?> <h2>Register</h2> <form action="register.php" method="post> <p>First Name: <input type="text" name="first_name size="15" maxlength="15 value="<?php if (isset($_POST['first_name])) echo $_POST['first_name]; ?>" /></p> <p>Last Name: <input type="text" name="last_name size="15" maxlength="30 value="<?php if (isset($_POST['last_name])) echo $_POST['last_name]; ?>" /></p> <p>Email Address: <input type="text name="email" size="20 maxlength="40" value="<?php if (isset($_POST ['email])) echo $_POST ['email]; ?>" /> </p> <p>Password: <input type="password name="password1 size="10" maxlength="20 /></p> <p>Confirm Password: <input type="password name="password2 size="10" maxlength="20 /></p> <p><input type="submit" name="submit value="Register" /></p> <input type="hidden" name="submitted value="TRUE" /> </form> <?php include ('./includes/footer.html'); ?>
| 12. | Save the file as register.php and upload it to your Web server.
| Now I'll put together a quick thank-you page. To create a thank-you page 1. | Create a new PHP document in your text editor (Script 8.8).
<?php # Script 8.8 - thanks.php $page_title = 'Thank You!'; include ('./includes/header.html'); ?> <h1 >Thank you!</h1> <p>You are now registered. In chapter 9--just around the corner--you will actually be able to log in!</p><p><br /></p> <?php include ('./includes/footer.html'); ?> Script 8.8. The user will be redirected to this simple page upon successfully registering. 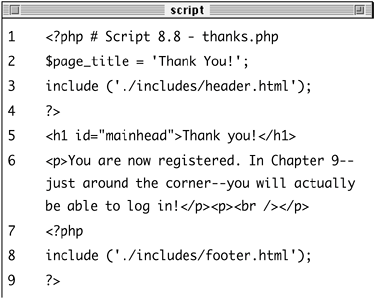
| 2. | Save the script as thanks.php, upload to your Web server along with the modified register.php, and test in your Web browser (Figures 8.18 and 8.19).
Figure 8.18. Once the user has been registered in the database, they are redirected to thanks.php. 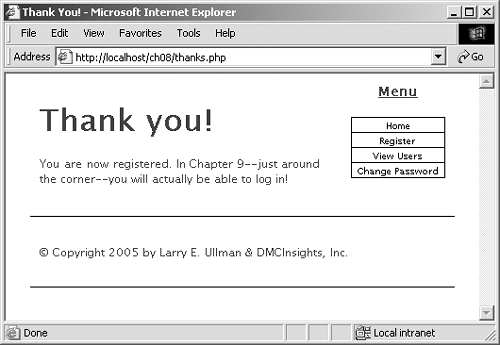 Figure 8.19. If any registration errors occurred, those errors are displayed above the registration form, as they had been in the past. 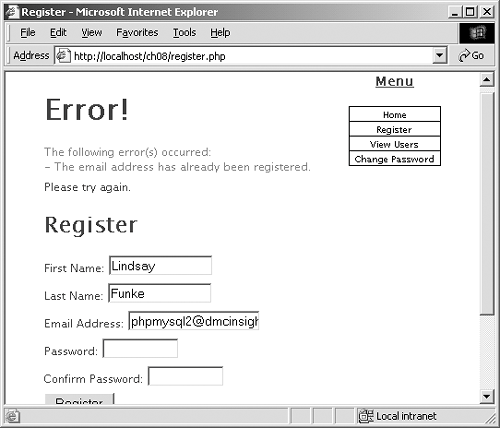
| Tips I cannot stress strongly enough that nothing can be sent to the Web browser before using the header() function. Even if your mysql_connect.php file has a blank line after the closing PHP tag, this will make the header() function unusable. On the bright side, PHP's error messages when it comes to headers having already been sent are quite informative. The error in Figure 8.17 indicates that line 41 of mysql_connect.php sent some output to the browser that meant that line 71 of register.php couldn't do its thing. So open up mysql_connect.php to find and fix the problem. Using Absolute URLs with header() You should ideally use an absolute URL with the header() function when using the Location directive (e.g., http://www.dmcinsights.com/admin/page.php rather than just page.php). To do so in your scripts, you have two choices. First, you could hard-code the absolute URL using header ('Location: http://www. dmcinsights.com/admin/page.php); This method will work unless the server configuration changes, the URL changes, or you move the script. A more flexible method is to make use of the $_SERVER superglobal and the dirname() function (which returns the directory path of the current script). The PHP manual recommends the following code to redirect to a page located in the same directory as the current script: header ('Location: http://' . $_SERVER['HTTP_HOST] . dirname($_ SERVER['PHP_SELF]) . '/newpage. php); This will effectively redirect the user to the current domain (www.dmcinsights.com), the current directory (admin), and the new page. This is the format I use in this chapter with just a minor modification. |
The substr() function is a very useful one that has not been given much attention in the book so far. For more information about its syntax and how you can use it, check out the PHP manual. The header() function is also frequently used when you have a PHP script that generates PDFs, images, and other non-HTML media. For everything you might want to know about HTTP and more, see www.w3.org/Protocols/. HTTP is a stateless protocol, which means it has no memory from one Web page to another. Chapter 9, "Cookies and Sessions," will show PHP's work-around for this. You can add name=value pairs to the URL in a header() call to pass values to the target page. In this example, if you added this line to the script, prior to redirection: $url .= '?name=' . urlencode ("$fn $ln);
then the thanks.php page could greet the user by $_GET['name'].
|