Users tend to have ideas about how they think Windows applications should behave. Some of these ideas make sense, and some do not. Most likely, these notions are derived from features they have seen in commercial programs. Of course, your users will then expect every simple VB program you write to behave in exactly the same way. For example, even though your program may have an Exit button, you can count on users to click the Close button in the upper-right corner. If you forget to add code to the Form_Closed event to handle this situation, your application could keep running when you don't want it to. The following sections describe several things about which you can count on users having expectations. Effective Menus One important part of form design is creating consistent, effective menus. Here are some important guidelines: Follow standard Windows layout convention: File, Edit, View, and so on. Group menu items logically and concisely. Use separator bars to group related items in a drop-down menu. Avoid redundant menu entries. Avoid top-level menu bar items without drop-down menus. Don't forget to use the ellipsis ( ... ) to denote menu entries that activate dialog boxes. Use standard shortcuts and hotkeys whenever possible. Put frequently used menu items in a toolbar. The steps involved in using the Menu Editor and in creating a shortcut (context) menu are discussed in Chapter 10, "Understanding Windows Forms." For more details on using the Menu Editor to create context menus, p. 263 Handling Multiple Instances of Your Application Most Windows programs are started when users navigate to a shortcut icon using the Start menu, or when they double-click a shortcut icon on the desktop. If users have multiple programs open, they can switch among them by using the taskbar or pressing Alt+Tab. However, many users like to use the program's shortcut icon again, even if the program is already open. Some shortcuts, such as the My Computer icon on the Windows desktop, simply bring up the existing window, whereas others launch a new instance of the program. If you provide a desktop or Start menu shortcut to your application, users may expect the shortcut to activate an existing instance of the application rather than start a new one. This is especially true if users fill out a form and then minimize it to the taskbar. They may click the shortcut expecting the form to reappear, but if you have not coded for this occurrence, another instance of your application is launched; the user will see an empty form. For certain applications, allowing multiple instances running at the same time may be desirable. However, if you do not design this capability into your application, errors will surely occur. For example, multiple copies of your application might try to write to the same database at the same time. Fortunately, preventing users from accidentally launching extra copies of your application is fairly easy. Visual Basic .NET's Diagnostics namespace provides a Process class, which gives your program access to processes (programs) running on the computer. The following lines of code, which can be placed in the Form_Load event or Sub Main(depending upon your application's Startup object), will check for the existence of a previous instance of the project, and close the new instance if a previous instance is found: If (UBound(Diagnostics.Process.GetProcessesByName _ (Diagnostics.Process.GetCurrentProcess.ProcessName)) > 0) Then End End If The preceding lines of code use the current process' ProcessName property to check the list of instances of processes with that name (as reported by the GetProcessesByName method) to end the program if another instance is already running. Although this code prevents conflicts and errors, it does not help the users because they still have to find the previously started application window manually. With a few calls to the Windows API (Applications Programming Interface), you can have your program show the previous application before exiting. Listing 14.1 shows a routine that can be placed in your main (startup) form's Code window to display the previous instance before exiting. Note The Windows API is a set of functions and procedures provided by the Windows operating system. Many of them offer functionality not normally provided via the .NET framework. By adding Declare statements to your code, as shown next, you can access these functions from within your Visual Basic .NET programs. Listing 14.1 PREVINST.ZIP Handling Multiple Application Instances Private Declare Function FindWindow Lib "user32" _ Alias "FindWindowA"(ByVal lpClassName As String, _ ByVal lpWindowName As String) As Integer Private Declare Function ShowWindow Lib "user32" _ (ByVal hWnd As Integer, ByVal nCmdShow As Integer) As Integer Private Declare Function SetForegroundWindow Lib "user32" _ (ByVal hWnd As Integer) As Integer Private Const SW_RESTORE As Short = 9 Private Sub Form1_Load(ByVal eventSender As System.Object, _ ByVal eventArgs As System.EventArgs) Handles MyBase.Load Dim sTitle As String Dim hWnd As Integer If (UBound(Diagnostics.Process.GetProcessesByName _ Dim lRetVal As Integer (Diagnostics.Process.GetCurrentProcess.ProcessName)) > 0) Then sTitle = Me.Text Me.Text = "newcopy" hWnd = FindWindow(Nothing, sTitle) If hWnd <> 0 Then lRetVal = ShowWindow(hWnd, SW_RESTORE) lRetVal = SetForegroundWindow(hWnd) End If End End If End Sub In the preceding code, you first rename the current application's form caption properties so that the FindWindow API function does not find it. Then you use three API calls to find the window belonging to the previous application, restore it, and move it to the foreground. Perceived Speed Perception is reality. I am referring here to how users' observations can influence their like or dislike of your program. Application speed is a prime example. You may have written the fastest code ever, but it matters little if users think it runs slowly. VB programmers tend to get defensive when users complain about speed because "the users don't understand how much work the program is doing." However, you can incorporate a few tricks to make your program seem faster. The key to a program's perceived speed is that something needs to happen when a user clicks an icon. Users are more willing to wait if they think the computer is working as fast as it can. Booting Windows is a good example; it usually takes quite a long time. However, all the graphics, beeps, and hard drive noise keep you distracted enough to make the wait acceptable. The techniques discussed in this section give you suggestions for creating "faster" applications. Program Startup Time When your program starts, you probably will have some initialization to perform for example, opening a network database. When set as your application's Startup object, the Sub Main procedure is an excellent place for all the initialization code required at startup time. However, this technique may cause program startup time to get a bit lengthy, so displaying a splash screen during load time is a good idea. A splash screen displays information about the program and its designer, as well as indicating to the user that some action is happening. In Figure 14.12, which illustrates a typical splash screen, notice that the mouse pointer (shown near the bottom-right of the form) has been changed to a combination arrow and hourglass by setting the form's Cursor property to AppStarting. This indicates to the user that the program is actually doing some work while in startup mode. Figure 14.12. A splash screen gives the user something to look at while program initialization takes place. 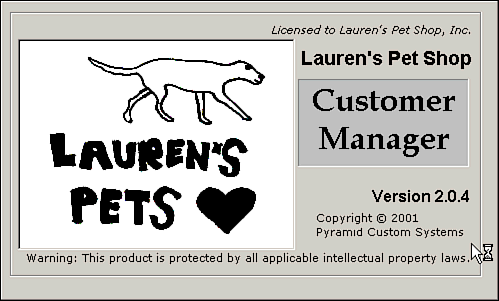 Inform the Users of Progress When your application looks like it is doing something, users tend to be more forgiving of long wait times. One way to keep them informed is to use a ProgressBar control on your form. If you are updating records in a database, you can use a progress bar to indicate the number of records processed so far. To do so, simply add an extra line of code or two to update the progress bar as you move to the next record. For more information on how to display status, p. 329 However, sometimes the progress bar is not an option. For example, Visual Basic's FileCopy function might take some time depending on the size of the file. However, FileCopy is a self-contained "black box" function, so you have no place to insert the progress bar update code. In this case, an easy alternative would be to display some type of animation, such as an animated GIF placed in a PictureBox control or an AVI animation file placed in an Animation control, before starting the file copy. Windows uses this trick when copying files and emptying the Recycle Bin. The users see the animation and think that it is linked to the file copy in progress when, in fact, it is a separate process running by itself. |