From a user interface standpoint, extra thought is required when you're writing a program that is designed to run on a PC other than your own. The Windows operating system leaves a lot of room for user customization. One of the most noticeable differences is screen resolution. If you go into the Display applet in Control Panel, you will notice that several options for screen size are available. As a developer and owner of a 21-inch monitor, I like to leave my resolution set to 1,280x1,024 pixels, which allows me to place a lot of things on the screen at once. However, most of your end users are likely to be operating at a lower resolution, say 800x600 or even 640x480. The easiest solution to this problem is to design your forms for a minimum 800x600 resolution. Users at that resolution will see your application fill up the whole screen, while users at higher resolutions will have extra desktop space to open other windows. Using the Anchor Property to Handle Form Size Changes Even if you do plan for a minimum screen resolution, what happens if your user resizes your application's forms? Remember, just because a user's display is set to a particular screen resolution, he can make the window much smaller than that resolution if he desires. Or, if he uses a high screen resolution and makes the window very large, there may be a lot of wasted space. If you do not plan for this situation, users who resize their windows could get unpleasant results, as shown in Figure 14.7. Figure 14.7. If you do not respond to users resizing your form, they may not see things as planned. 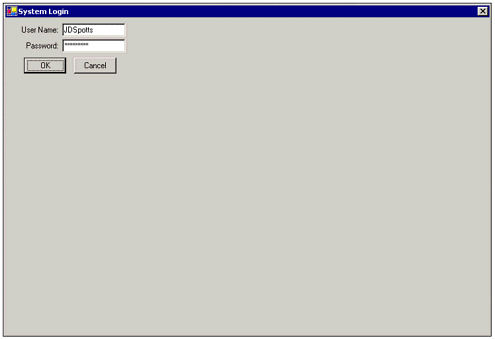 Previously, in order to deal with this situation, you had to write code such as the following in the form's Resize event handler to resize and reposition your controls whenever a form was resized: Private Sub Form_Resize() If Me.Height <= 1365 Then Exit Sub lstMain.Height = Me.Height - 1365 lstMain.Width = Me.Width - 420 cmdOK.Top = lstMain.Height + 360 cmdOK.Left = lstMain.Width - cmdOK.Width cmdCancel.Top = cmdOK.Top cmdCancel.Left = cmdOK.Left - cmdCancel.Width - 120 End Sub Visual Basic .NET has introduced a nice new feature to make this task much easier. Most controls now support an Anchor property, which allows you to specify some combination of the four edges of a control's parent form that it should be bound to. By default, a control is anchored (bound) to the top and left edges of the form that contains it. If the user resizes the form, the control's position relative to the top and left edges of the form stays constant. As the form is made wider and/or taller, the right and bottom edges of the form get further away from the right and bottom edges of the control. You can seta control's Anchor property to any combination of the four edges of the form Top, Bottom, Left, and Right. When a form is resized, the distance from each edge of the control specified by the Anchor property to the respective edge of the form remains constant, even if and this is the key to how the property works the control must be resized and/or repositioned in order to maintain these distances. The resizing and repositioning of the control are handled automatically. Tip The Anchor property works best when you use it for a small number of controls on a form. Figures 14.8, 14.9, and 14.10 illustrate a Button control whose Anchor property has been set to Bottom, Right and a Label control whose Anchor property has been set to Top, Left, Right. As the form is resized, the Exit button's position relative to the bottom and right edges of the form remains constant. This has the effect of making the Exit button stay in the lower-right corner of the form, as the developer intended. In addition, the Label control is resized as the form's size changes, making the text contained in the label react to the form's new size. Figure 14.8 shows the form at its original size, while Figures 14.9 and 14.10 show the form smaller and larger, respectively. Figure 14.8. This form's Exit button is anchored to the lower-right corner, and its Label control is anchored to three edges of the form. 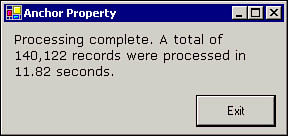 Figure 14.9. As the form gets smaller, the label and the button are repositioned to maintain the distances to their respective anchor edges. 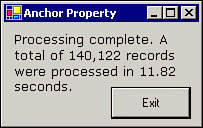 Figure 14.10. Even as the form grows larger, the Exit button's position relative to the lower-right corner is unchanged. 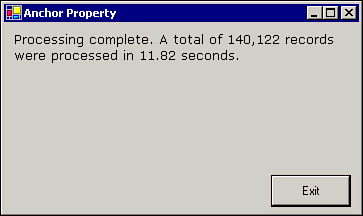 Regional Options Another customization that can adversely affect your program is the Regional Options applet in the Control Panel, pictured in Figure 14.11. This dialog box allows users to set their own currency, date, and time formats, among other things. If you use these predefined formats in your program, make sure that your variables, database fields, and calculations can handle them. Figure 14.11. Make sure your program is prepared to handle changes users make in the Regional Options Control Panel. 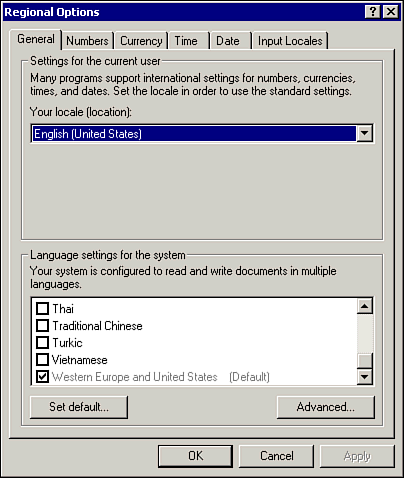 |