This extension adds a custom data source to the Bindings panel for Macromedia ColdFusion documents. Users can specify the variable they want from the new data source. This example creates a data source called MyDatasource, which includes a MyDatasource.js JavaScript file, a MyDatasource_DataRef.edml file, and MyDatasource Variable command files to implement a dialog box for users to enter the name of a specific variable. The MyDatasource example is based on the implementation of the Cookie Variable data source and the URL Variable data source. The files for these data sources reside in the Configuration/DataSources/ColdFusion folder. You create this data source by performing the following steps: - Creating the data source definition file
- Creating the EDML file
- Creating the JavaScript file that implements the Data Sources API functions
- Creating the supporting command files for user input
- Testing the new data source
Creating the data source definition file The data source definition file tells Dreamweaver the name of the data source as it will appear in the Bindings Plus (+) menu and also tells Dreamweaver where to find the supporting JavaScript files for the data source implementation. When a user clicks on the Bindings Plus (+) menu, Dreamweaver searches the DataSources folder for the current server model to gather all available data sources defined in the folder's HTML (HTM) files. So, to make a new data source available to the user, you need to create a data source definition file that simply provides the name of the data source using the TITLE tag and the location of all supporting JavaScript files using the SCRIPT tag. In addition, several supporting files are necessary for implementing this data source. In general, you might not need to use the functions in these supporting files, but they are often useful (and necessary in this example). For example, the dwscriptsServer.js file contains the dwscripts.stripCFOutputTags() function used in the implementation of this data source. And, using the DataSourceClass.js file, you create an instance of the DataSource class to use as the return value of the findDynamicSources() function. To create the data source definition file: 1. | Create a new blank file.
| 2. | Enter the following:
<HTML> <HEAD> <TITLE>MyDatasource</TITLE> <SCRIPT src="/books/4/533/1/html/2/../../Shared/Common/Scripts/dwscripts.js"></SCRIPT> <SCRIPT src="/books/4/533/1/html/2/../../Shared/Common/Scripts/dwscriptsServer.js"></SCRIPT> <SCRIPT src="/books/4/533/1/html/2/../../Shared/Common/Scripts/DataSourceClass.js"></SCRIPT> <SCRIPT src="/books/4/533/1/html/2/MyDatasource.js"></SCRIPT> </HEAD> <body></body> </HTML>
| 3. | Save the file as MyDatasource.htm in the Configuration/DataSources/ColdFusion folder.
| Creating the EDML file The EDML file defines the code that Dreamweaver inserts into the document to represent the data source value. (For more information about EDML files, see Chapter 15, "Server Behaviors," on page 321). When a user adds a particular value from a data source to a document, Dreamweaver inserts the code that will translate into the actual value at runtime. The participant EDML file defines the code for the document (for more information, see "Participant EDML files" on page 347). For the MyDatasource Variable, you want Dreamweaver to insert the ColdFusion code <cfoutput>#MyXML.variable#</cfoutput> where variable is the value the user wants from the data source. To create the EDML file: 1. | Create a new blank file.
| 2. | Enter the following:
<participant> <quickSearch><![CDATA[#]]></quickSearch> <insertText location="replaceSelection"><![CDATA[<cfoutput>#MyDatasource .@@bindingName@@#</cfoutput>]]></insertText> <searchPatterns whereToSearch="tag+cfoutput"> <searchPattern paramNames="sourceName,bindingName"><![CDATA[/#(?:\s*\w+\s*\()? (MyDatasource)\.(\w+)\b[^#]*#/i]]></searchPattern> </searchPatterns> </participant>
| 3. | Save the file as MyDatasource_DataRef.edml in the Configuration/DataSources/ColdFusion folder.
| Creating the JavaScript file that implements the Data Sources API functions After you have defined the name of the data source, the name of the supporting script files, and the code for the working Dreamweaver document, you need to specify the JavaScript functions for Dreamweaver to provide the user with the ability to add, insert, and delete the necessary code into a document. Based on the construction of the Cookie Variable data source, you can implement the MyXML data source, as shown in the following example. (The MyDatasource_Variable command used in the addDynamicSource() function is defined in "Creating the supporting command files for user input" on page 387.) To create the JavaScript file: 1. | Create a new blank file.
| 2. | Enter the following:
//************** GLOBALS VARS ***************** var MyDatasource_FILENAME = "REQ_D.gif"; var DATASOURCELEAF_FILENAME = "DSL_D.gif"; //****************** API ********************** function addDynamicSource() { MM.retVal = ""; MM.MyDatasourceContents = ""; dw.popupCommand("MyDatasource_Variable"); if (MM.retVal == "OK") { var theResponse = MM.MyDatasourceContents; if (theResponse.length) { var siteURL = dw.getSiteRoot(); if (siteURL.length) { dwscripts.addListValueToNote(siteURL, "MyDatasource", theResponse); } else { alert(MM.MSG_DefineSite); } } else { alert(MM.MSG_DefineMyDatasource); } } } function findDynamicSources() { var retList = new Array(); var siteURL = dw.getSiteRoot() if (siteURL.length) { var bindingsArray = dwscripts.getListValuesFromNote(siteURL, "MyDatasource"); if (bindingsArray.length > 0) { // Here you create an instance of the DataSource class as defined in the // DataSourceClass.js file to store the return values. retList.push(new DataSource("MyDatasource", MyDatasource_FILENAME, false, "MyDatasource.htm")) } } return retList; } function generateDynamicSourceBindings(sourceName) { var retVal = new Array(); var siteURL = dw.getSiteRoot(); // For localized object name... if (sourceName != "MyDatasource") { sourceName = "MyDatasource"; } if (siteURL.length) { var bindingsArray = dwscripts.getListValuesFromNote(siteURL, sourceName); retVal = getDataSourceBindingList(bindingsArray, DATASOURCELEAF_FILENAME, true, "MyDatasource.htm"); } return retVal; } function generateDynamicDataRef(sourceName, bindingName, dropObject) { var paramObj = new Object(); paramObj.bindingName = bindingName; var retStr = extPart.getInsertString("", "MyDatasource_DataRef", paramObj); // We need to strip the cfoutput tags if we are inserting into a CFOUTPUT tag // or binding to the attributes of a ColdFusion tag. So, we use the // dwscripts .canStripCfOutputTags() function from dwscriptsServer.js if (dwscripts.canStripCfOutputTags(dropObject, true)) { retStr = dwscripts.stripCFOutputTags(retStr, true); } return retStr; } function inspectDynamicDataRef(expression) { var retArray = new Array(); if(expression.length) { var params = extPart.findInString("MyDatasource_DataRef", expression); if (params) { retArray[0] = params.sourceName; retArray[1] = params.bindingName; } } return retArray; } function deleteDynamicSource(sourceName, bindingName) { var siteURL = dw.getSiteRoot(); if (siteURL.length) { //For localized object name if (sourceName != "MyDatasource") { sourceName = "MyDatasource"; } dwscripts.deleteListValueFromNote(siteURL, sourceName, bindingName); } }
| 3. | Save this file MyDatasource.js in the Configuration/DataSources/ColdFusion.
| Creating the supporting command files for user input The addDynamicSource() function contains the command dw.popupCommand("MyDatasrouce_Variable"), which opens a dialog box for the user to enter a specific variable name. However, you still need to create the dialog box for MyDatasource Variable. To provide a dialog box for the user, you must create a new set of command files: a command definition file in HTML and a command implementation file in JavaScript (for more information about command files, see "How commands work" on page 167). The command definition file tells Dreamweaver the location of the supporting implementation JavaScript files as well as the form for the dialog box that the user sees. The supporting JavaScript file determines the buttons for the dialog box and how to assign the user input from the dialog box. To create the command definition file: 1. | Create a new blank file.
| 2. | Enter the following:
<!DOCTYPE HTML SYSTEM "-//Macromedia//DWExtension layout-engine 5.0//dialog"> <html> <head> <title>MyDatasource Variable</title> <script src="/books/4/533/1/html/2/MyDatasource_Variable.js"></script> <SCRIPT src="/books/4/533/1/html/2/../Shared/MM/Scripts/CMN/displayHelp.js"></SCRIPT> <SCRIPT src="/books/4/533/1/html/2/../Shared/MM/Scripts/CMN/string.js"></SCRIPT> <link href="../fields.css" rel="stylesheet" type="text/css"> </head> <body> <form> <div ALIGN="center"> <table border="0" cellpadding="2" cellspacing="4"> <tr> <td align="right" valign="baseline" nowrap>Name:</td> <td valign="baseline" nowrap> <input name="theName" type="text" > </td> </tr> </table> </div> </form> </body> </html>
| 3. | Save the file as MyDatasource_Variable.htm in the Configuration/Commands folder.
| NOTE The file MyDatasource_Variable.js is the implementation file that you create in the next procedure. To create the supporting JavaScript file: 1. | Create a new blank file.
| 2. | Enter the following:
//******************* API ********************** function commandButtons(){ return new Array(MM.BTN_OK,"okClicked()",MM.BTN_Cancel,"window.close()"); } //***************** LOCAL FUNCTIONS ****************** function okClicked(){ var nameObj = document.forms[0].theName; if (nameObj.value) { if (IsValidVarName(nameObj.value)) { MM.MyDatasourceContents = nameObj.value; MM.retVal = "OK"; window.close(); } else { alert(nameObj.value + " " + MM.MSG_InvalidParamName); } } else { alert(MM.MSG_NoName); } }
| 3. | Save the file as MyDatasource_Variable.js in the Configuration/Commands folder.
| Testing the new data source You can now open Dreamweaver (or restart it if you already have it open), and open a ColdFusion file or create a new one. To test your new data source: 1. | With the pointer in the document, click on the Bindings Plus (+) menu to see all the available data sources. MyDatasource should appear at the bottom of the list:
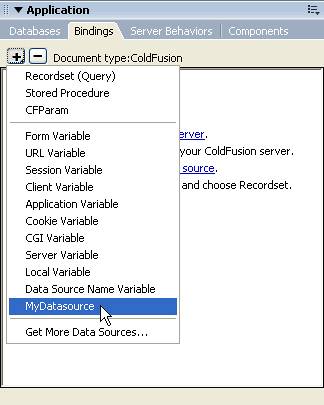
| 2. | Click the MyDatasource data source option, and the MyDatasource Variable dialog box you created appears:
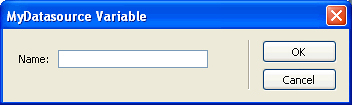
| 3. | Enter a value in the dialog box and click OK.
The Bindings panel displays the data source in a tree with the variable from the dialog box under the data source name:
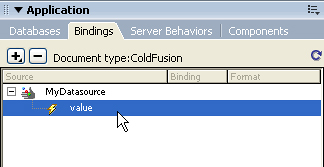
| 4. | Drag the variable to your document, and Dreamweaver will insert the appropriate code from the EDML file:
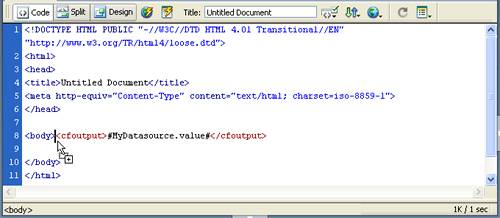 | |