 | Before you learn about Web services, it's a good idea to examine what a regular service is. When someone does a task for you, he's providing you a service. For example, you can go to a gas station and fill up your gas tank, or receive a tune-up. These are services provided to you by the gas station so that you don't have to do it yourself. Imagine if everyone had to have their own gas pump. Not an ideal situation. You also go to restaurants to receive food service. This is the type of task you can do yourself, but it requires work that you may not be willing to do. So a service is a value-added task provided by a person (or company) that frees you from having to do it yourself. | A Web service is the same thing. Web sites can provide a service that visitors, or even other Web sites, can take advantage of. Imagine a Web portal that presents information such as local and national news, weather information, sports scores, and other personalized content. It provides a service to visitors by compiling information from many different sources into one place. However, unless the portal has a very large budget and a huge staff, it's nearly impossible to keep writing up-to-date content for all these different sections. Instead, the portal can rely on content from other sites and simply provide the display mechanism. The portal still provides a service to users, but it relies on services provided by other sites. This is already widespread in news reporting. The Associated Press provides a news service that newspapers can tap into. Next time you're reading a newspaper, look for articles written by the Associated Press. They've been pulled from a news service. This type of system hasn't been widely used on the Internet because of complications involving how services should communicate. Many companies have attempted to build proprietary communication systems that allow services to be exchanged, but these are often too complex and expensive to be adopted by the general community. Also, problems have arisen due to the structure of security systems on the Internet. Many of these proprietary systems have difficulty transferring data across firewalls, which are designed to stop unauthorized traffic. Web services, provided by the .NET Framework, are a solution to these and other problems. A Web service is a programmable object (just like a business object) that provides functionality that's accessible to any number of systems over the Internet. The reason Web services work is that they rely on standardized technologies for objects to communicate. Customized systems and proprietary mechanisms aren't necessary for Web services to work. All you need is an Internet connection. XML Web services rely on the fact that any type of system or application can use HTTP, the standard protocol for Internet communication, and can use and convert XML, a standard for delivering data over the Web. XML Web services use XML to send commands and move data to and from objects residing on one server. The applications that use the data and send the commands can be written in any language, for any computer architecture, and they can be simple or complex. All the applications need to know is the Web service's location (basically, its Internet address). Web services provide a new level of computing. In much the same way that you can assemble various objects and components to build an application, developers can assemble a group of Web services from completely different locations and use them in their own applications. Completely different platforms can now communicate easily, enabling disparate systems around the world to be tied together. Web Service Scenarios Imagine that you've built a component that performs simple calculation functions, like the calculator you built on Day 2, "Building ASP.NET Pages." Recall that the calculator performed simple arithmetic operations. Another Web site somewhere has built a component that can place orders to a home improvement store. Assuming these two components are Web services, a savvy developer could link them to create an application that allows a user to design her home. When the user needs to determine measurements and calculations, she uses your Web service's calculation functions. Then she places an order to the home improvement store through the other Web service. All this is accomplished from within one application, and the user doesn't need to know where the pieces of functionality came from. Figure 16.3 illustrates this example. Figure 16.3. A single application can tie together many Web services.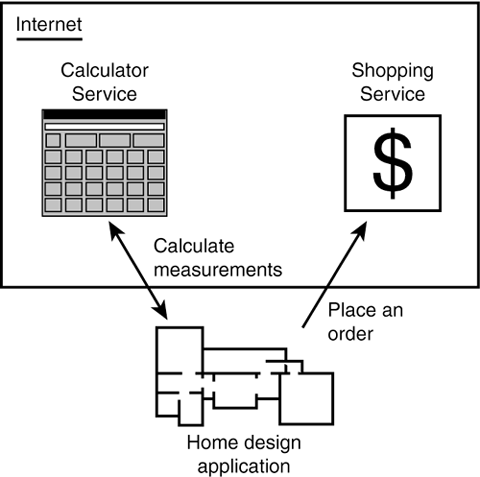 Web services can even be used by Internet applications. Imagine an e-commerce site that must calculate shipping charges for customers ordering supplies. Normally, this site would have to maintain an up-to-date table for calculating shipping costs based on the shipper, the shipping location, the priority, and so on. With Web services, the site can place a "call" to the shipping company directly, using a brief XML command, and receive the quotes instantly. Web services easily tie together applications that were once very difficult to assemble. The Web Service Programming Model As mentioned earlier, Web services use XML to communicate. But what exactly is being communicated?  | The first messages that are sent usually involve a process known as discovery, which is how a client application finds and examines a Web service. The discovery process involves messages sent to and from the service that describe what the service can do. The client needs to know this before it tries to use the service. The service also tells the client what other kinds of messages it will accept. | The discovery process is not a necessary one. For instance, if a service doesn't want anyone to know about it, it can disable the discovery process. This is a protective measure so that not just anyone can use a particular Web service you've created. Following discovery, the service must tell the client what it expects to receive (that is, which commands it will accept) and what it will send back. This is a necessary step so that both service and client know how to communicate with each other. This data is known as a Web service description. In a business object, the developer typically knows ahead of time which commands the object will support (through the documentation provided, for instance). The service description is essentially the documentation for the service in XML format. Finally, messages are sent back and forth with commands to the service and data for the client, again in XML. Figure 16.4 illustrates the entire process. Figure 16.4. Interaction with a Web service involves discovery, description, and commands.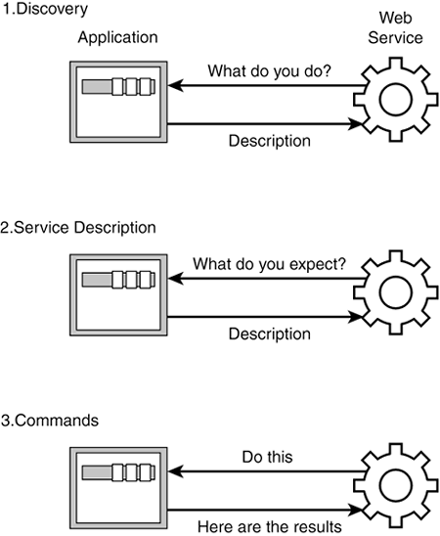 Luckily, ASP.NET provides most of the infrastructure needed to perform all these operations. After all, it can handle requests and responses, translate XML, and use business objects, making it ideal for Web service development. You just need to decide what your Web service will do and then build it. Protocols for Accessing Web Services You know that XML Web services rely on XML-formatted messages to send data and receive commands. Specifically, they use XML in the discovery process and service description. The messages containing commands aren't required to use XML, however. They can be sent in two additional methods, Http-Get and Http-Post, which are used in ASP.NET pages in querystrings and forms, respectively. Web services support three protocols for interacting with clients: Http-Post, Http-Get, and the Simple Object Access Protocol (SOAP). Discussing these protocols in any depth is beyond the scope of this book, but you should have a basic understanding of them and how they affect Web services. Http-Get Http-Get is a standard protocol that enables clients to communicate with servers via HTTP. Http-Get is the way that you typically request Web pages. Think of the Http-Get operation as a client getting a Web page from a Web server. In essence, a client sends an HTTP request to the URL of the Web site, which responds with the appropriate HTML. Any parameters that are needed to process the request are attached via the querystring. For example: http://www.myserver.com/default.aspx?id=Chris&sex=male The parameters id and sex are passed as inputs to the Web server, attached to the end of the URL. An ASP.NET page could then retrieve these values with this code: Request.Querystring("id") Request.Querystring("sex") Web services can use Http-Get and the querystring to pass commands and parameters, instead of XML messages. Note that information sent with Http-Get is part of the URL. Http-Get has limited functionality, however, because it can only send name/value pairs. Http-Post This protocol is similar to Http-Get, but instead of appending parameters onto the URL, it places them directly in the HTTP request message. When a client requests a page via Http-Post, it sends an HTTP request message with additional information that contains parameters and values. The server must then read and parse the request to figure out the parameters and their values. The most common use of Http-Post is in HTML forms. For example, imagine an HTML form with the fields id and sex, as shown in the following code snippet: <form method="post"> <input type="Text" > <input type="Text" > <input type="Submit" Value="Submit" /> </form> When you submit this form, your browser takes the values entered in the text boxes and adds them to HTTP request message to the server. The server can retrieve these values with this syntax: Request.Form("id") Request.Form("sex") This protocol, like the Http-Get protocol, is limited to sending name/value pairs. Note Note that you can also send information via Http-Get with forms. In the form method, simply specify "Get" and the form will add the information to the querystring instead of the HTTP request header. For instance: <form method="Get"> SOAP The Simple Object Access Protocol is a relatively new standard that allows clients to send data to servers and vice versa. Unlike Http-Get and Http-Post, SOAP relies on XML to relay its information instead of an HTTP request message. This means that SOAP can send not only name/value pairs, but more complex objects as well, such as various rich data types, classes, objects, and others. Sending SOAP messages is a bit different than what we're used to. With Http-Get, we send information via the querystring, and with Http-Post, we send it via form submissions. Both methods rely on the request/response model of operation. SOAP information is also transported via HTTP, but isn't limited to the request/response model. It can be used to send any types of messages, whether a client has requested them or not. Thus, SOAP is a very flexible medium to send data. Note Many people get confused when comparing SOAP and XML. If SOAP is such a great way to send messages, why not use it instead of XML? The difference is that XML defines a data format, while SOAP defines a protocol for exchanging that data. SOAP relies on XML to send its messages. Plain XML is ideal for transporting most types of data. It's only when you want to transfer things such as commands and instructions that SOAP applies. Because SOAP is based on XML, you can use it to transport data around the Web. XML is simply pure text, so it can be sent anywhere HTML pages can, including through firewalls. This is the default protocol that Web services use to communicate with clients. Why Web Services? Now that you know what Web services are, why should you use them? Try to remember the world 10 15 years ago, before the rise of the Internet. Computer systems were usually standalone entities with no access to other systems. Applications were designed for use on one machine, with no sharing of data in any way. There was very little communication via computer within or between companies. Even interpersonal messages often had to be delivered by hand. The Internet has changed the way the world communicates. It's also transformed the way applications are built. There are very few applications that don't take advantage of the Internet somehow. Many applications, such as instant messaging, rely on the Internet to provide users with data. The next step in connecting users over the Internet is to deliver applications this way. Already, corporations are trying to tie traditional applications together into a single composite entity. When you consider the number of legacy applications still in use, this is a daunting task. Web services provide a very simple mechanism for applications to communicate with each other. They allow components to be shared and functionality to be delivered to anyone, anywhere. Imagine never having to install another program on your computer again simply connect to the Web and access the service. All this may be great, but how do Web services make Web development better? One of the ways is through code reuse. Recall that one of the reasons for using business objects is that you can reuse the same code over and over again without having to create it each time. Even branching logic such as functions and subroutines promotes code reuse (see Day 3, "Using Visual Basic.NET and C#."). With Web services, you can now reuse code that other people have developed without having to download, copy, or install anything. This saves you time and energy, enabling you to easily add functionality to your ASP.NET applications. Another benefit of Web services is ease of deployment and maintenance. No longer will you have to install custom objects or applications on many different systems. Instead, users will have a standard framework for accessing your application directly over the Internet. Also, when changes need to be made, you won't have to issue a new release or an update. Simply make the changes in your Web service in one place, and all clients will benefit from it automatically. (Unless, of course, the change removes functionality that clients depend on, in which case they have to adjust. Either way, the process is easier for the developer.) |