To create a DHTML function, you often need to have that function run repeatedly until, well, until you don't want it to run anymore. This repeated running of the function lets you animate objects or cause objects to wait for a particular event to happen in the browser window before continuing. To do this, we will be using the setTimeout() method, which lets you run a function after a specific delay. See the sidebar "Why setTimeout()" for more details. In this example (Figure 17.1), I modified the toggleVisibility() function from Chapter 16, causing it to leap continuously until the visitor clicks the image to stop it. Figure 17.1. Click the image to stop the annoying flash. Please! 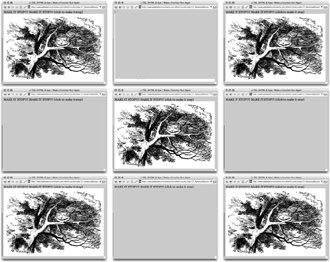 Why setTimeout()? One common question I get about running a function repeatedly with the setTimeout() function is, "Why not just call the function from within itself?" There are two reasons: Netscape Navigator 4 has a bug that causes the entire browser to crash when a function calls itself recursively. Although Netscape 4 does not need to be an ongoing concern, this can be very annoying if a user visits your site using this browser. setTimeout() makes it easy to control a pause between the function's looping back and running again. This can come in handy if you need the function to run more slowly than the computer would run it automatically. |
To call a function repeatedly: 1. | var theDelay = 500; Initialize the global variables (Code 17.1):
Code 17.1. The setUpAnnoyingFlash() function prepares the initial values of variables that are then run in the annoyingFlash() function. [View full width] <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Strict//EN" "http://www.w3.org/TR/xhtml1/DTD/ xhtml1-strict.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>CSS, DHTML & Ajax | Make a Function Run Again</title> <script type="text/javascript"> var theDelay = 500; var state = null; var object = null; var toRepeat = 0; function setUpAnnoyingFlash(objectID, onOffon) { if (onOffon == 1) { toRepeat = 1; object = document.getElementById(objectID); object.style.visibility = 'visible'; state = 'visible'; annoyingFlash(); } else toRepeat = 0; } function annoyingFlash() { if (toRepeat == 1) { if (state == 'hidden' ) object.style.visibility = 'visible'; else { if (state == 'visible') object.style.visibility = 'hidden'; else object.style.visibility = 'visible'; } state = object.style.visibility; setTimeout ('annoyingFlash()', theDelay); } else{ object.style.visibility = 'visible'; return; }} </script> <style type="text/css" media="screen"> #cheshireCat { visibility: visible; position: relative; } </style> </head> <body onload="setUpAnnoyingFlash ('cheshireCat', 1);"> <p>MAKE IT STOP!!!! MAKE IT STOP!!! (click to make it stop)</p> <div onclick= "setUpAnnoyingFlash('cheshireCat', 0);"> <img src="/books/3/292/1/html/2/alice24.gif" height="435" width="640" alt="Cheshire Cat" /></div> </body></html> | theDelay sets the amount of time in milliseconds between each running of the function. The value 1,000 milliseconds equates to a one-second delay, so 500 is half a second. object is used to record the ID of the object that is being changed and is initially set to null. toRepeat records whether the function should be repeating (1) or stopped (0). | 2. | function setUpAnnoyingFlash(objectID, onOffon) {...} Add a function that sets initial parameters for the repeating function, and then calls the function to start it.
In this example, setUpAnnoyingFlash() first checks to see whether it's being told to start or stop the function it is controlling. If starting, it finds the object to be used, sets its initial state to visible, and triggers the function annoyingFlash(). Otherwise it stops the function by setting the variable toRepeat to 0.
| | | 3. | function annoyingFlash() {...} Add the function you want to repeat. In this example, annoyingFlash() is started by the setUpAnnoyingFlash() function in Step 2.
If toStop is 1, the visibility is toggled (visible if hidden, hidden if visible). The function then uses the setTimeout() method to call itself to run again after a delay:
setTimeout ('annoyingFlash()', theDelay); The annoyingFlash() function keeps running until toStop is 0, in which case the visibility is finally set to visible, and the function stops running.
| 4. | #cheshireCat {...} Set up the CSS rules for your object(s) with the relevant stylesin this example, the visibility state.
| 5. | onload="setUpAnnoyingFlash ('cheshireCat', 1)" Add an event handler to the body element to trigger the function you created in Step 2, and pass to it the ID for the object. Indicate whether you want in this example to retain the annoying flash (1) or not (0).
| 6. | <div onclick=" setUpAnnoyingFlash('cheshireCat', 0)">...</div> Set up your object(s) as needed, based on the ID from Step 4, with an event handler to stop the repeating function.
| Tip When you run this example code, notice that you can click the cat to stop the flashing only while the image is visible. That's because the event can only be triggered when the layer is visible. |