In this task, you improve how the products are placed in the shopping cart. You create a shopping cart component and use that when products are added to the cart.
1. | Create another component in the views/ecomm folder named Cart.mxml and use a VBox tag as the base tag. Remove any width and height values.
This will eventually be your shopping cart display component.
|
2. | In an <mx:Script> block, import the following three classes from the valueObjects folder: ShoppingCart, ShoppingCartItem, and Product.
|
3. | Create a bindable public variable named cart, data typed as ShoppingCart.
This is the property to which the shopping cart data will be passed.
|
4. | Create a private function named renderLabel(), data typed as String. The function should accept a parameter named item, data typed as ShoppingCartItem.
This function will create and return a String value that will be added to the List component that is currently the shopping cart display.
|
| |
5. | In the function, return the item quantity, product name and subtotal, concatenated as a single String. There should be a space between the quantity and name, and a colon between the name and subtotal:
return String(item.quantity)+" "+item.product.prodName+":"+String(item.subtotal); This will populate the shopping cart as follows:
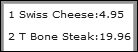 Because the quantity and subtotal are numeric, you should cast them as Strings to be concatenated, although it is not required.
|
6. | Below the <mx:Script> block, insert an <mx:List> tag. Give the List an id of cartView, bind the dataProvider to the aItems array of the cart property, and set the labelFunction property equal to the renderLabel function you just created.
<mx:List dataProvider="{cart.aItems}" labelFunction="renderLabel"/> Each time an item is added to the shopping cart, the cart property of this component will be updated, causing the dataProvider to change; and the List updated.
|
7. | Return to EComm.mxml and locate the <mx:DataGrid> tag in the cartView state.
|
8. | Replace the <mx:DataGrid> tag with an instantiation of your Cart.mxml component. Set the id equal to shoppingCart, set the width to 100%, and bind the cart property to the cart variable:
<v:Cart width="100%" cart="{cart}"/> Notice the binding. This means that any time the cart variable changes on this page (items added or removed from the cart, for instance) the binding will be redone, and changes rippled into your Cart component.
|
| |
9. | In GroceryDetail.mxml, locate the itemAdded() function in which you currently trace the product. Replace the trace statement with an invocation of the addToCart() method of the EComm.mxml application file. Pass the prod variable as a parameter. To reference the EComm.mxml file, use the mx.core.Application.application property:
mx.core.Application.application.addToCart(prod); What you are doing here is not a best practice. You will find in the next lesson a better way to pass the product to the addToCart() function.
Note  | To access properties and methods of the top-level application, you can use the application property of the mx.core.Application class. This property provides a reference to the Application object from anywhere in your Flex application. Although this is possible, you should carefully consider its use because it will lead to tightly coupled components and might not be a best practice. | |
10. | In EComm.mxml, change the access modifier of the addToCart() function from private to public.
This has to be done because you are accessing the method from outside the file itself.
|
11. | Test the new functionality by running EComm.mxml.
After you add some items to the cart, click the View Cart button to see your shopping cart. You will see two shopping carts, one of which you will remove from a State in the next step.
|
12. | In EComm.mxml, look for an <mx:RemoveChild> tag in the State in which the linkbutton1 object is the target. Add another <mx:RemoveChild> tag under the existing one in which the target removed is cartView.
<mx:RemoveChild target="{cartView}"/> This prevents the two shopping carts, the one you created in the Cart component and the one in the bodyBox HBox near the end of the file, from being displayed at the same time.
|
13. | Create a new folder under the FlexGrocer project named as.
|
14. | Right-click on the new as directory and choose New > ActionScript File. In the New ActionScript File dialog box, set the filename to be ecomm.as; then click Finish. Remove any comments in the new file.
|
15. | In EComm.mxml, cut all the ActionScript code that is between the character data tags in the <mx:Script> block and paste the code into the ecomm.as file.
You have moved this code into a separate file to keep the size of the MXML file more manageable.
Tip  | To remove the excess tabs, highlight all the code and press the Shift+Tab key combination three times to move the appropriate code to the left margin. | |
| |
16. | In EComm.mxml, remove the <mx:Script> block and replace it with an <mx:Script> tag that uses the source property to point to the new ActionScript file just created:
<mx:Script source="as/ecomm.as"/> Specifying a source property to include the ActionScript file is just as if the ActionScript were still in the MXML file. Again, this is not required and is just to make file sizes more manageable.
|
17. | Test EComm.mxml; you should see no differences in functionality after moving the <mx:Script> block into a separate ActionScript file.
|