We're not finished quite yet. To help solidify your understanding of inheritance, we'll make a few enhancements to the Animal class so you can see how easy it is to update an object-oriented/inheritance-based project.
1. | The Library panel should still be open from the preceding exercise. Double-click the Balloon movie clip to edit its Timeline.
This movie clip's Timeline is simple. It contains a transparent white box and a text field instance called name. The name of this field is important to remember.
|
2. | Return to the main Timeline. In the library, right-click (Ctrl-click on a Macintosh) the Balloon movie and select Linkage from the menu that appears. In the Linkage Properties dialog box, give this movie clip an identifier of balloon; then click OK.
This identifier will be used in a moment to dynamically attach this movie clip to our animal movie clip instances when the mouse rolls over them.
|
3. | Animal.as should still be open. Click its tab to make Animal.as the active window.
Over the next several steps we'll add functionality to this class; by extension, that new functionality will filter down into the Dog and Cat classes because they inherit from this class.
|
4. | Insert the following script just below private var speed:Number;:
private var balloon:MovieClip; private var name:String; This step creates two new private properties called balloon and name in the Animal class. The name property eventually will be used to hold the name we give to instances of the Animal class, or, by extension, the Dog and Cat classes. The balloon property will hold a reference to the movie clip that will be used to show the name of the animal on stage.
|
5. | Insert the following method just below the end of the stop() method definition:
public function setName(name:String):Void { this.name = name; } This step creates a setter method called setName() that accepts a single parameter, name. The parameter value that's passed in is used to set the name property of an instance. For example, the following will set dog's name property to have a value of "Fido":
dog.setName("Fido"); In a moment, we'll add script that will invoke the setName() method to set the name properties of both the dog and cat instances. First we'll add several lines of script to the Animal constructor function.
|
6. | Insert the following line of script into the Animal constructor method, just below this.speed = 5:
name = "Animal"; Remember that the script inside the constructor function is executed as soon as an instance of the class is created. In our project, because the Dog and Cat classes inherit from Animal, and the Dog and Cat movie clips in the library are associated with the Dog and Cat classes, when an instance of either clip appears in the movie this constructor function is executed in relation to the instance.
With the line of script in this step, we give a default name value to instances of the Animal class as well as the Dog and Cat classes. So when dog and cat first appear in the movie, each will have a default name value of Animal. This value can be changed as a result of the setName() method we added in Step 5, which we'll use shortly.
|
7. | Add the following method to the animal class:
private function onRollOver():Void { useHandCursor = false; balloon = attachMovie("balloon", "balloon", 0); balloon._x = _xmouse; balloon._y = _ymouse - 50; balloon.name.text = name; } This method overrides the onRollOver method of the MovieClip class. This script executes when the dog or cat instance appears on the stage and the user rolls the mouse over the instance. The purpose of this script is to display the value of the instance's name property in a little window based on the Balloon clip in the library (we discussed the Balloon clip in Step 1).
The first line of the function sets the instance's useHandCursor property to false; therefore, the hand cursor won't appear when the instance is rolled over. The next line attaches the movie clip with the balloon identifier in the library. The attached instance is given a name of balloon and a depth of 0. The next two lines position the newly attached instance so that it appears at the same x position as the mouse, but 50 pixels less than the y position of the mouse; therefore, the balloon will appear slightly offset from the mouse cursor.
Remember that the Balloon movie clip has a text field instance called name on its Timeline. When you attach that clip and give it an instance name of balloon, you can set the text displayed in that field by referencing it as follows:
balloon.name.text The last line of the script uses this reference to display the name property of the instance in the text field.
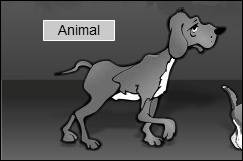 Let's create the functionality that will remove the balloon when the user rolls away from the instance.
|
8. | Add the following method to the animal class:
private function onRollOut():Void { balloon.removeMovieClip(); } This script removes the balloon movie clip instance when the user rolls away.
|
9. | Choose File > Save to save the Animal class file with its new functionality.
The Animal class is now complete. All that's left to do is to return to the FLA file for this project and add a couple of lines of script.
|
10. | PetParade1.fla should already be open in the authoring environment. Click its tab to make PetParade1.fla the active window. With the Actions panel open, select Frame 1 of the Actions layer and add the following script at the end of the current script:
dog.setName("Fido"); cat.setName("Fluffy"); These two lines of script utilize the new setName() method created in the Animal class file in Step 5. The name property of the dog instance is set to "Fido" and the name property of the cat instance is set to "Fluffy". These new values override the default name value for Animal instances, discussed in Step 6.
This property value will appear in a balloon when the instance is rolled over. Let's do one final test.
|
11. | Choose Control > Test Movie.
When the movie appears, you can move the mouse over either the dog or cat, and the animal's name will appear in a balloon. The important thing to realize about this new functionality is that it was set up in a single file: the Animal class. All instances that inherited from this class automatically inherited this new behavior when the class file was updated. We hope that you can now appreciate the power of inheritance and how it allows you to create more manageable projects.
|
12. | Close the test movie and save your work as PetParade2.fla.
Due to page constraints, most of the projects in the remainder of the book will not use a class-based object-oriented structure. However, the concepts you learned here can be applied in class-based projects you create on your own.
|