3.6. Enable Web Parts to Talk to Each Other Note: Learn how the various Web Parts on a page can communicate with each other. The various Web Parts on a page are not standalone islands of information. You can enable them to talk to each other by connecting them. This is useful in cases where you need to pass information obtained from one Web Part to another. For example, you may enter a Zip Code to obtain address information in a Web Part. The same Zip Code may be sent to another Web Part so that it can retrieve weather information. 3.6.1. How do I do that? To illustrate how two Web Parts can be connected, you will first create a new Web User control that contains a Calendar control. When the user clicks a date in the Calendar control, the date selected will be sent to and displayed by the text box in the Google Web User control that you created in the lab. Using the project created in the previous lab (C:\ASPNET20\chap03-Webparts), add a new class to the project (right-click the project name in Solution Explorer and select Add New Item..., then select Class) and name it ISelectedDate.vb. Code the ISelectedDate.vb class as follows. The ISelectedDate interface would be implemented by both the connection provider (the CalendarUC.ascx) and provider consumer (Google.ascx). This interface serves as a contract for the communication between the provider and consumer: Imports Microsoft.VisualBasic Public Interface ISelectedDate ReadOnly Property SelectedDate( ) As Date End Interface Add a new Web User control to the project by right-clicking the project name (C:\ASPNET20\chap03-Webparts) in Solution Explorer, selecting Add New Item..., and then selecting Web User Control. Note: Communications between Web Parts is based on the provider and consumer model. To ensure that a Web Part can receive data from another Web Part, the Web Part that is providing data must publish a contract specifying the type of data it is publishing. The Web Part consuming the data can then use this contract to obtain the data.
Name the Web User control project CalendarUC.ascx. Populate CalendarUC.ascx with a Calendar control and apply the Simple scheme (via the Auto Format... link in the Calendar Tasks menu). The CalendarUC.ascx should now look like Figure 3-37. Figure 3-37. CalendarUC.ascx 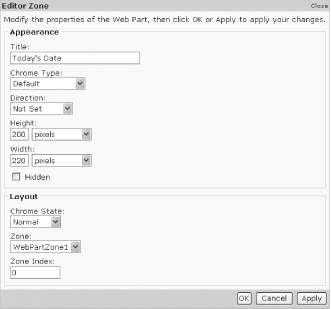
Double-click on CalendarUC.ascx to switch to its code-behind, and add the following code to configure the Web User control as a provider: Partial Class CalendarUC_ascx Inherits System.Web.UI.UserControl Implements ISelectedDate Public ReadOnly Property SelectedDate( ) As Date _ Implements ISelectedDate.SelectedDate Get Return Calendar1.SelectedDate.Date End Get End Property <ConnectionProvider("SelectedDate", "SelectedDate")> _ Public Function GetSelectedDate( ) As ISelectedDate Return Me End Function End Class The first parameter to the ConnectionProvider attribute assigns a friendly name to the provider connection point. The second parameter assigns a unique ID to the provider connection point. Essentially, you are implementing the ISelectedDate interface and creating a provider connection point by using the ConnectionProvider attribute. You also implemented the SelectedDate property to return the selected date in the Calendar control. In the Google.ascx user control, add the following lines of code that are shown in bold: Partial Class Google_ascx Inherits System.Web.UI.UserControl Protected Sub btnSearch_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnSearch.Click Response.Write(Page.IsValid) Dim queryStr As String = HttpUtility.UrlEncode(txtSearch.Text) Response.Redirect("http://www.google.com/search?q=" & queryStr) End Sub Private _selectedDate As ISelectedDate <ConnectionConsumer("SelectedDate", "SelectedDate")> _ Sub setSearchText(ByVal SearchText As ISelectedDate) Me._selectedDate = SearchText End Sub Protected Sub Page_PreRender(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles Me.PreRender If _selectedDate IsNot Nothing Then txtSearch.Text += _selectedDate.SelectedDate.ToShortDateString End If End Sub End Class The first parameter to the ConnectionConsumer attribute assigns a friendly name to the consumer connection point. The second parameter assigns a unique ID to the consumer connection point. Essentially, you are using the ConnectionConsumer attribute to define a consumer connection point, and allowing it to act as a receiver of the ISelectedDate interface. The date that is received is then appended to the text box in Google.ascx in the PreRender event of the Page class. Add the CalendarUC.ascx Web User control to WebPartZone2 in Default.aspx. Ensure that they are named as shown in Figure 3-38 (the Google Web Part was added in the previous lab). Also, add the title attribute for the Calender Web User control: <ZoneTemplate> <uc1:Google title="Google Search" runat="server" /> <uc3:CalendarUC title="Calendar Web Part" runat="server" /> </ZoneTemplate> Figure 3-38. Adding the provider and consumer Web Parts to the page 
To wire up the provider and consumer Web Parts, add the following static connections in Default.aspx under the WebPartManager control: <asp:WebPartManager runat="server"> <StaticConnections> <asp:WebPartConnection Provider ProviderConnectionPoint Consumer ConsumerConnectionPoint /> </StaticConnections> </asp:WebPartManager> Press F5 to test the application. When you select a date in the Calendar control, notice that the text box in the Google Web Part will display the selected date (see Figure 3-39). Figure 3-39. Connecting two Web Parts 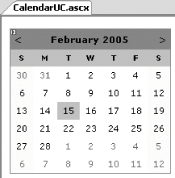 3.6.2. What about... ...creating connections on the fly while the application is running? In this lab, you learned how to statically connect two Web Parts together at design time. What happens if you need to connect a new Web Part (newly added to the page, for example) to another provider? In this case, you need to dynamically allow the user (not the developer) to create the connection. The following steps will show how you can use the ConnectionsZone control to link two Web Parts together during runtime. The nice feature of this control is that there isn't much you need to do; it simply does the work for you. Add the Google Web User control (Google.ascx) into WebPartZone2. There should now be two Google Search Web Parts and one Calendar Web Part (see Figure 3-40). Figure 3-40. The Web Parts in WebPartZone2 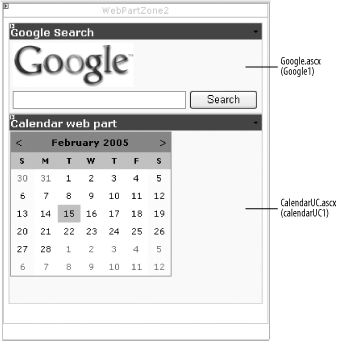
Drag and drop the ConnectionsZone control (found under the WebParts tab in the Toolbox) onto Default.aspx. Apply the Classic scheme (see Figure 3-41) to the ConnectionsZone control (via the Auto Format... link in the ConnectionsZone Tasks menu). Figure 3-41. Applying the Classic scheme to the ConnectionsZone control 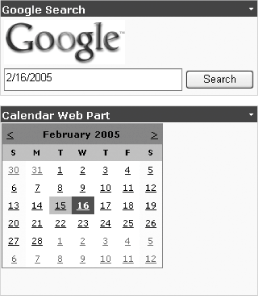
In the code-behind, add the following line (shown in bold) to the SelectedIndexChanged event of the radio button list control (see Figure 3-42). This will cause the ConnectionsZone control to be displayed when the Connect Display Mode item in the radio button list control is clicked: Protected Sub rblMode_SelectedIndexChanged( _ ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles rblMode.SelectedIndexChanged Select Case rblMode.SelectedIndex Case 0 : WebPartManager1.DisplayMode = _ WebPartManager.BrowseDisplayMode Case 1 : WebPartManager1.DisplayMode = _ WebPartManager.DesignDisplayMode Case 2 : WebPartManager1.DisplayMode = _ WebPartManager.CatalogDisplayMode Case 3 : WebPartManager1.DisplayMode = _ WebPartManager.EditDisplayMode Case 4 : WebPartManager1.DisplayMode = _ WebPartManager.ConnectDisplayMode End Select End Sub Figure 3-42. Adding the Connect Display Mode item to the radio button list control 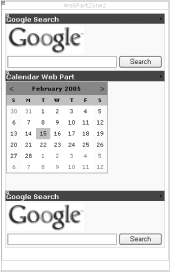
To test the page, press F5. Click on the Connect Display Mode option in the radio button list control. Note that there is no visual change to the page. Click on the top-right corner of the Google Search [2] Web Part. You will see that there is now a Connect link (see Figure 3-43). Click on the Connect link. Figure 3-43. Configuring connection for a Web Part 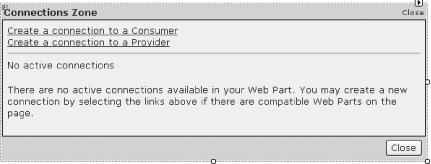
You will now see the Connections Zone (see Figure 3-44). Click on the "Create a connection to a Provider" link to connect the Google Search Web Part to another Web Part. Figure 3-44. Creating a connection for a Web Part 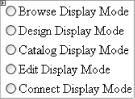
You can now select a provider connection for this Web Part. In the From: drop-down listbox, select "Calendar Web Part." Click Connect (see Figure 3-45). Figure 3-45. Selecting a provider connection 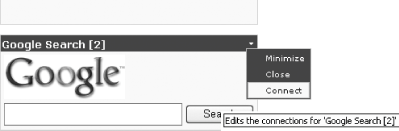
You will now notice that the two Google Search Web Parts are connected to the Calendar Web Part (see Figure 3-46). Figure 3-46. Connecting the two Google Search Web Parts to the Calendar Web Part 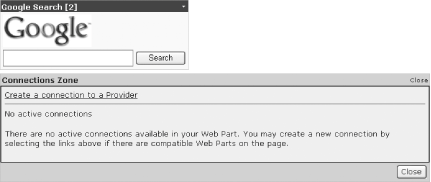
To dislodge the connection, click on the Disconnect button in the Connections Zone (see Figure 3-47). Figure 3-47. Disconnecting a connection 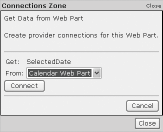 3.6.3. Where can I learn more? To learn more about how Web Parts and cross-page connections work, check out the following useful discussion: http://www.nikhilk.net/CrossPageConnections.aspx. |