The .NET Framework SDK includes the Web Services Description Language tool, wsdl.exe. This tool does not require the Web service client to use Visual Studio .NET. It can take a WSDL file and generate a corresponding proxy class, which you can use to invoke the Web service: Open the Visual Studio .NET Command Prompt and navigate to the folder that contains the WSDL file you created in the previous section using disco.exe. Enter the following command to create a proxy class to call the AirportWeather Web service: wsdl /language:CS /out:AirportWeatherProxy.cs AirportWeather.wsdl. The tool reads the WSDL file and creates a new file named AirportWeatherProxy.cs. Create a new Visual C# ASP.NET Web Application project (Example5_3). Add a reference to System.Web.Services.dll. Add the AirportWeatherProxy.cs file to the project by selecting File, Add Existing Item. Place a Label control, TextBox control (txtCode), Button control (btnGetWeather), and ListBox control (lbResults) on the Web form. Change the Text property of the label to Airport Code:. Double-click the Button control and enter the following code to invoke the Web service when the user clicks the Get Weather button: private void btnGetWeather_Click(object sender, System.EventArgs e) { // Connect to the Web service AirportWeather aw = new AirportWeather(); // Invoke the service to get a summary object WeatherSummary ws = aw.getSummary(txtCode.Text); // And display the results lbResults.Items.Clear(); lbResults.Items.Add(ws.location); lbResults.Items.Add("Wind " + ws.wind); lbResults.Items.Add("Sky " + ws.sky); lbResults.Items.Add("Temperature " + ws.temp); lbResults.Items.Add("Humidity " + ws.humidity); lbResults.Items.Add("Barometer " + ws.pressure); lbResults.Items.Add("Visibility " + ws.visibility); } Build and run the project. Use the Web form to invoke the Web service. The difference between this project and Example5_1 is that this code explicitly defines the objects it uses rather than discovering them at runtime. The AirportWeather and WeatherSummary objects are proxy objects that pass calls to the Web service and return results from the Web service. Table 5.2 shows some of the command-line options you can use with wsdl.exe. You can use either the path to a local WSDL or Disco file, or the URL of a remote WSDL or Disco file with this tool. Table 5.2. Important Command-Line Options for wsdl.exeOption | Meaning |
---|
/appsettingurlkey:DomainName | Specifies the domain name to use when connecting to a server that requires authentication. | /domain:DomainName /d:DomainName | Specifies the domain name to use when connecting to a server that requires authentication. | /language:LanguageCode /l:LanguageCode | Specifies the language for the generated class. The LanguageCode parameter can be CS (for C#, which is default), VB (for Visual Basic .NET), or JS (for JScript). | /namespace:Namespace /n:Namespace | Specifies a namespace for the generated class. | /out:Filename /o:FileName | Specifies the filename for the generated output. If it's not specified, the filename is derived from the Web service name. | /password:Password /p:Password | Specifies the password to use when connecting to a server that requires authentication. | /server | Generates a class to create a server based on the input file. By default, the tool generates a client proxy object. | /username:Username /u:Username | Specifies the username to use when connecting to a server that requires authentication. | /? | Displays full help on the tool. | /appsettingurlkey:DomainName /d:DomainName | Specifies the domain name to use when connecting to a server that requires authentication. | Using Web References As an alternative to using the Web Services Discovery and WSDL tools to create explicit proxy classes, you can simply add a Web reference to your project to enable the project to use the Web service. This is the same technique you used in the Example5_1 project. In fact, the end result is no different when using the tools to create a proxy class and adding a Web reference because, behind the scenes, the Web reference creates its own proxy class. To see this, click the Show All Files toolbar button in Solution Explorer for the project Example5_1, and then expand the Web References node. You'll see a set of files similar to those in Figure 5.3. Figure 5.3. When you add a Web reference, the proxy file is automatically generated. 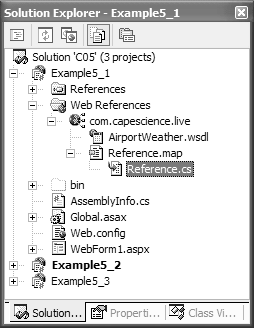 The .wsdl file is the same file that is generated by running the Web Services Discovery tool on the URL of the Web reference, and the .map file is the same as the .discomap file generated by the Web Services Discovery tool. As you can see by opening this file, the .cs file defines the proxy objects to be used with the Web service that is represented by this Web reference. The major difference between this file and the proxy you generated with the WSDL tool is that the autogenerated file uses a namespace based on the name of the Web reference. 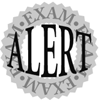 | Why use a Web reference? The major benefit of using a Web reference (as compared to constructing proxy classes with the command-line tools) is that updating the proxy classes is easier if the Web service changes. In that case, all you need to do is right-click the Web Reference node in Solution Explorer and select Update Web Reference. |
Testing a Web Service If you want to test a Web service without building an entire client application, you can use a testing tool. Several of these tools are easily available: NetTool This is a free Web services proxy tool from CapeClear. You can obtain a copy from http://capescience.capeclear.com/articles/using_nettool. .NET WebService Studio This tool comes from Microsoft. You can download a free copy from www.gotdotnet.com/team/tools/web_svc. XML Spy This tool includes a SOAP debugger that can be used to test Web services. You can download a trial copy of this XML editor and toolkit from www.xmlspy.com. All three of these tools work in the same basic way: They intercept SOAP messages between Web service clients and servers so that you can inspect and, if you want, alter the results. The following steps use the .NET WebService Studio tool to see a Web service in action: Download the .NET WebService Studio tool from www.gotdotnet.com/team/tools/web_svc and install it on your computer. Launch the WebServiceStudio.exe application. Enter http://live.capescience.com/wsdl/AirportWeather.wsdl as the WSDL endpoint and click the Get button. The tool reads the WSDL file from the Web service and constructs the necessary proxy classes to invoke it. Click the getSummary entry on the Invoke tab to use the getSummary() Web method. In the Input section, select the arg0 item. You can now enter a value for this item in the Value section. Enter an airport code, such as KDTW, for the value. Click the Invoke button. The tool sends a SOAP message to the Web service, using your chosen parameters, and then displays the results (see Figure 5.4). Figure 5.4. The .NET WebService Studio tool enables you to test a Web service without creating any client application. 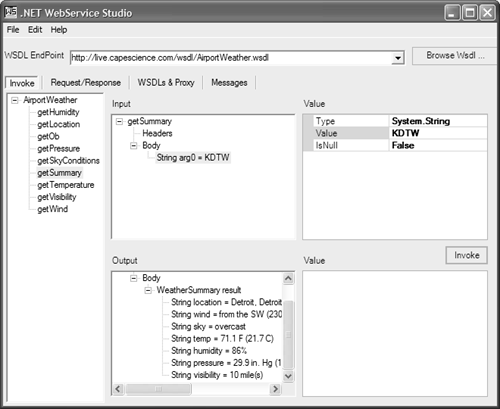
Click the Request/Response tab to view the outgoing and incoming SOAP messages. Click the WSDLs and Proxy tab to see the WSDL file and the generated proxy class for this Web service. |