An integrated development environment (IDE) is a GUI application that allows you to develop a Java application using a convenient editor. Though using an IDE makes it convenient to develop your application, one of the chief benefits to using an IDE is debugging. You have likely already used an IDE to debug your Java applications. It is also possible to use an IDE to debug a custom tag library, such as the tag library used in Chapter 11. In this section, we show you how to use an IDE to debug a custom tag library. Using the IDE, you can easily debug your application as you step through your routines and observe the values of variables as your program runs. This can be invaluable for debugging your program and learning why it does what it does. Commercial IDEs include Visual Cafe, JBuilder, and Visual Age. Many IDEs are available for free. For example, Sun's Forte includes a community edition that you can download for free Forte at http://java.sun.com/. In addition, the Eclipse IDE is a completely open source project being developed by IBM. Next, we show you how to install this IDE to use with Tomcat. Introducing Eclipse Eclipse is an advanced and very popular IDE for the Java platform. There is some speculation as to where the name for Eclipse came from. Some say that it IBM meant to imply that they will "eclipse" Sun Microsystems in the Java tools market. Whatever the meaning behind the name, it is a valuable tool. You can download Eclipse from the IBM Web site at http://www.eclipse.org/. The Eclipse IDE can be used with many platforms. If you're using Windows or Linux, you can download a binary version that is ready for install. When you download Eclipse, you'll notice that the program is in one large Zip archive. Inside this archive is a single folder, named eclipse. You should copy the eclipse folder to a convenient location on your computer. Inside the eclipse archive is a single executable file named eclipse.exe. Double-click this file to start the Eclipse system. It may be convenient to create a shortcut, or link, to this executable and place this shortcut at a convenient location, such as your desktop. The Eclipse IDE does not come with a JDK. You must have already downloaded and installed a JDK prior to installing Eclipse. We suggest you use JDK version 1.4 or higher. Once you have installed the JDK and Eclipse on your system, you are ready to begin using Eclipse. When Eclipse first starts, you'll see the blue Eclipse splash screen as the program loads. Once Eclipse loads, you'll see the Eclipse main screen, shown in Figure 12.4. 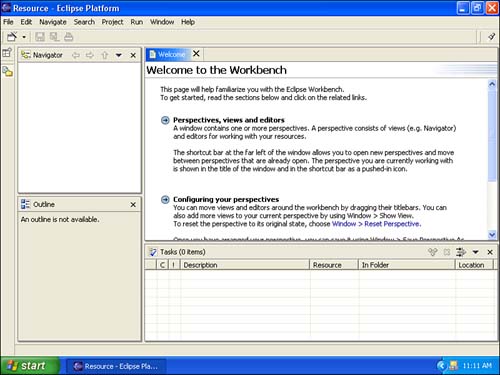 For the IDE debugging example shown in this chapter, we debug the tag library we presented in Chapter 11. To debug the forum application, you must set up a project file that builds the forum tag library and includes the Tomcat Web server. In the next section, you'll learn how to set up a project that uses Tomcat. Using Eclipse with Tomcat Debugging a Web application is not as simple as debugging a normal Java application using an IDE. To debug a JSP-based application, you must bring the entire Web server, in this case Tomcat, into the debugging context. There are several ways that you can do this. One approach is to use a third-party plug-in that has been developed for the Eclipse IDE. Sysdeo developed the Tomcat plug-in that allows you to use Tomcat with Eclipse. This free tool makes it easier to work with Tomcat and Eclipse. You can download this tool from http://www.sysdeo.com/eclipse/tomcatPlugin.html. For our examples, we don't use a third-party Tomcat plug-in. It is easy enough to configure Eclipse to recognize the JAR files included with the Tomcat distribution. However, if the process we describe here causes difficulty, you might consider using a third-party plug-in for Tomcat. To debug your Web application with Eclipse, you must set up an Eclipse project. Eclipse stores projects in individual folders. These folders contain the settings, Java files, and other information needed to execute to that project. You can download the project file being used here from the Sams Publishing Web site. This folder is named eclipse-forum; copy the folder to a convenient location on your computer. The source files available from the Web site contain a sample project in a directory named eclipse-forum. In this chapter, we'll assume you've copied the folder to your local directory (c:\eclipse-forum). At this point, you must inform Eclipse of the folder's existence by creating a new project. When you choose to create a new project in Eclipse, you'll see a screen similar to the one shown in Figure 12.5. 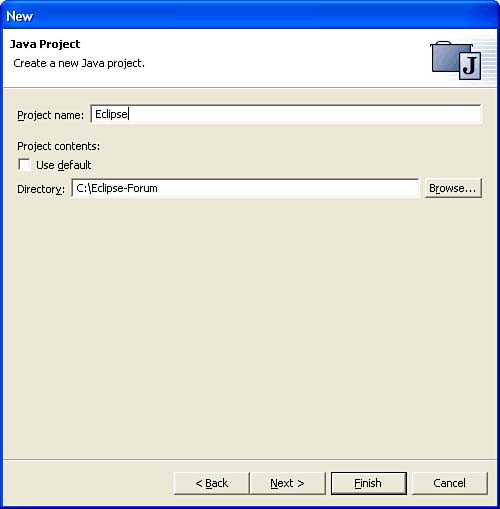 We are not really creating a new project, but it is necessary to use this method to make Eclipse aware of the forum folder. Simply type in the name of the copied folder, and Eclipse will begin using that folder. Once you've successfully created a new project, you'll see a screen like the one shown in Figure 12.6. 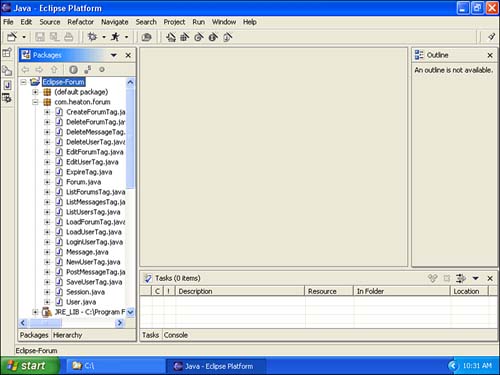 Next, make sure that everything is accessible by attempting to rebuild your project. From the Project menu, select Rebuild All. If all goes well, you'll see a progress bar indicating that your project is being recompiled. After the build completes, you should not see any errors. If errors are reported, you must investigate the causes of these errors and correct them. If you are receiving errors, the most likely cause is that the JAR files, which are necessary for Tomcat to run, are not being found by the Eclipse IDE. These JAR files were set up in the Project Properties window and have absolute paths pointing to them. If you installed Tomcat to a folder other than the standard c:\Program Files\Apache Tomcat 4.0, then you must modify the location of each JAR file. If you installed Tomcat into a nonstandard location, you'll have to adjust the library setting in Project Properties. Begin by right-clicking on the project node on the tree at the left side of the Eclipse IDE. This opens a window that allows you to choose from several tabs. Select the Java Build Path option, and then select the Libraries tab to display a window similar to the one shown in Figure 12.7. 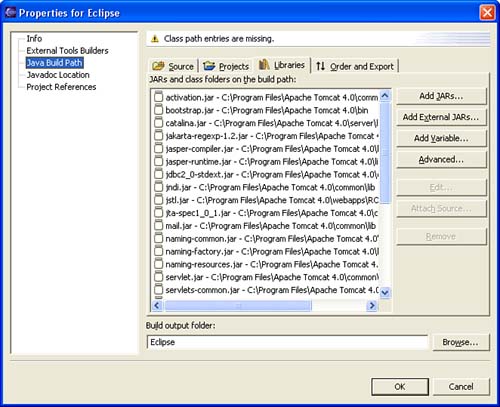 You must now go through each of the entries in the libraries and make sure they point to the correct location of the JAR files. The JAR files are all included within the Tomcat directory. When you've corrected the locations of the JAR files, you should be able to recompile the forum application. Once you've recompiled the forum application, you should run it to ensure that it actually works. To run the application from Eclipse, click the toolbar item that looks like a running man. This launches the application and allows you to test it. To test the application, launch a Web browser and attempt to access the forum examples just as you did previously when you were running Tomcat without a debugger. With Tomcat running, you should be able to display the URL http://127.0.0.1:8080/example. If this URL displays, this means you have Tomcat working properly with Eclipse. If you examine the project file, you may notice that one additional source file was added other than what was already available with the forum application. This source file, named DebugTomcat.Java, starts the Tomcat Web server and allows you to debug it. This short program is shown in Listing 12.4. Listing 12.4 The DebugTomcat File (DebugTomcat.java)import java.io.*; import org.apache.catalina.startup.*; public class DebugTomcat { public static void main(String args[]) { String a[] = {"start"}; System.setProperty("catalina.home","c:\\Program Files\\Apache Tomcat 4.0"); try { Bootstrap.main(a); } catch (Exception e) { System.out.println(e); } } } As you can see, the main point of this file is to simulate launching Tomcat from the command line. You should also notice the path that is hard-coded into the file. Keep in mind that you will have to change this file path if you stored Tomcat in a nonstandard location. Now that you have successfully launched Tomcat from the Eclipse IDE, you're ready to begin debugging the forum application. Creating Your Own Project If you are going to create your own project, you must add all of the necessary files yourself. You should start with an empty Java project in Eclipse. Next, import all of the files that you need to compile your tag library. You can import files by right-clicking the project directory and choosing Import. If your .java files are inside packages, create corresponding packages in Eclipse. You create packages by right-clicking the project, selecting New, and choosing Package. You must also include the starter .java file that we discussed in the previous section. This file is named DebugTomcat.java. Modify the path information, as we described earlier. This allows Tomcat to find your JSP and configuration files. Now you must include all of the JAR files from Tomcat. We usually like to add all the JAR files from Tomcat into the project. They may not all be necessary, depending on which features of Tomcat you are using, but adding them all saves you from running into problems later, when Tomcat can't find them. The Tomcat JAR files are in the /common directory. You should include the JSTL JAR files as well, which are usually in the WEB-INF/lib directory. Now that you've set up your project, you are ready to launch Tomcat. If prompted for a class to execute, you should choose the DebugTomcat file we added earlier. Debugging the Forum Application You're now ready to begin debugging the forum application. The first step is to place a breakpoint. A breakpoint is a specific line in your program that should pause execution when that line is reached. The program will run normally until the breakpoint is hit. At that point, you can pause and examine variables. You can also step through the program one line at a time once you have reached the breakpoint. When you've finished debugging that portion of the program, you can allow the program to continue executing. The program will then continue until it reaches another breakpoint. For this example, let's place a breakpoint inside one of the source files used for the forum tag library. We'll place the breakpoint in one of the most important source files contained in the forum application: the Session.java file. This file handles database connections used by the forum application. Nearly every tag in the forum tag library uses this source file. To begin, open the Session.java file. You want to place a breakpoint on the first line of the getSession() method. Right-click the gray area to the left of every line in the source file. This will open up a small pop-up menu that allows you to choose to add a breakpoint. Once you have added this breakpoint, your screen should look like the one in Figure 12.8. 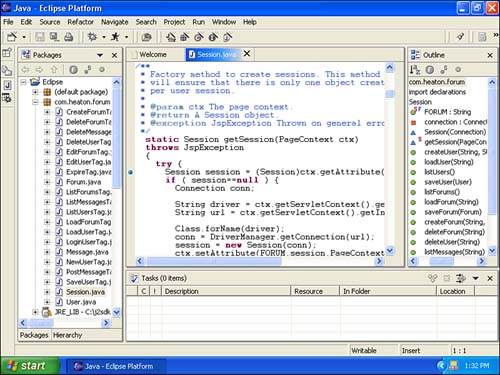 Once you've set the breakpoint, you are ready to execute your program. Keep in mind that you should not click the running-man icon that we used in the previous section; if you use this icon to start your program, breakpoints will not be honored because the program is only running in execute mode. (Execute mode is much faster than debug mode, and you should use it when you do not need breakpoints.) To start the program in debug mode, click the toolbar icon that looks like a bug. Tomcat launches, and you should see the output from Tomcat near the bottom of the Eclipse screen. This output should look exactly like it does when you start Tomcat in a standalone mode. You can use a browser and open your Web page. You should now open the forum application and proceed to log on normally. Once this process is complete, wait until your program hits the breakpoint. At this point, you are free to perform any of the debugging operations. This includes examining variables, using watches, or stepping through your program line by line. WARNING The Web server may reach your breakpoint and not stop. There could be several reasons for this. The most common is that a compiled JAR file that contains the tag libraries is already in the Tomcat path. If Tomcat has access to the forum.jar file that we previously used, it will use the tags in this JAR file, not the tags in the debugging session. The result is that Eclipse never gets a chance to see the breakpoints, and thus never stops. You have now seen how to debug your application. Once an application is debugged, you will likely want to prepare it for distribution. The next section explains how you can package your Web application for easy distribution. |