[Page 228]5.6. From the Java Library: java.text.NumberFormat Although the Math.round() method is useful for rounding numbers, it is not suitable for business applications. Even for rounded values, Java will drop trailing zeroes. So a value such as $10,000.00 would be output as $10000.0. This wouldn't be acceptable for a business report.  | java.sun.com/docs |
Fortunately, Java supplies the java.text.NumberFormat class precisely for the task of representing numbers as dollar amounts, percentages, and other formats (Fig. 5.10). Figure 5.10. The java.text.NumberFormat class. 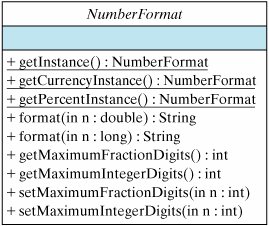 The NumberFormat class is an abstract class, which means that it cannot be directly instantiated. Instead, you would use its static getInstance() methods to create an instance that can then be used for the desired formatting tasks. Once a NumberFormat instance has been created, its format() method can be used to put a number into a particular format. The setMaximumFractionDigits() and setMaximumIntegerDigits() methods can be used to control the number of digits before and after the decimal point. For example, the following statements can be used to format a decimal number as a currency string in dollars and cents: NumberFormat dollars = NumberFormat.getCurrencyInstance(); System.out.println(dollars.format(10962.555)); These statements would cause the value 10962.555 to be shown as $10,962.56. Similarly, the statements, NumberFormat percent = NumberFormat.getPercentInstance(); percent.setMaximumFractionDigits(2); System.out.println(percent.format(0.0655)); would display the value 0.0655 as 6.55%. The utility of the Math and NumberFormat classes illustrates the following principle: Effective Design: Code Reuse  | Often the best way to solve a programming task is to find the appropriate methods in the Java class library. |
[Page 229]Self-Study Exercise Exercise 5.20 | A Certificate of Deposit (CD) is an investment instrument that accumulates interest at a given rate for an initial principal over a fixed number of years. The formula for compounding interest is shown in Table 5.12. It assumes that interest is compounded annually. For daily compounding, the annual rate must be divided by 365, and the compounding period must be multiplied by 365, giving: a = p(1 + r/365)365n . Implement a BankCD class that calculates the maturity value of a CD. Figure 5.11 gives the design of the class. It should have three instance variables for the CD's principal, rate, and years. Its constructor method sets the initial values of these variables when a BankCD is instantiated. Its two public methods calculate the maturity value using yearly and daily compounding interest, respectively. Use the Math.pow() method to calculate maturity values. For example, the following expression calculates maturity value with annual compounding: principal * Math.pow(1 + rate, years) Figure 5.11. The BankCD class. 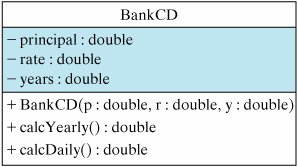 Table 5.12. Formula for calculating compound interesta = p(1 + r)n where | a is the CD's value at the end of the nth year p is the principal, or original investment amount r is the annual interest rate n is the number of years, or the compounding period |
| Exercise 5.21 | Design a command-line user interface to the BankCD class that lets the user input principal, interest rate, and years, and reports the CD's maturity value with both yearly and daily compounding. Use NumberFormat objects to display percentages and dollar figures in an appropriate format. The program's output should look something like the following (user's inputs are in green): ************************ OUTPUT ******************** Compare daily and annual compounding for a Bank CD. Input CD initial principal, e.g. 1000.55 > 2500 Input CD interest rate, e.g. 6.5 > 7.8 Input the number of years to maturity, e.g., 10.5 > 5 For Principal = $2,500.00 Rate= 7.8% Years= 5.0 The maturity value compounded yearly is $3,639.43 The maturity value compounded daily is: $3,692.30 ************************ OUTPUT ******************** | |