Web server controls are similar to HTML server controls. They're created on the server and allow you to build complex user interfaces easily. They require the runat="server" attribute to work correctly. They also provide rich programmatic capabilities. Unlike HTML server controls, however, Web controls don't necessarily map one-to-one to HTML elements and can represent more complex UI elements. For example, the Calendar Web control represents a complex HTML table with multiple rows and columns that displays a calendar. You've already seen a few Web controls in action. The Label and Button controls used throughout today's lesson are two of them. To create a Web control, you use the following syntax: <asp:Control docEmphasis">name" runat="server"> For example, a Label control looks like this: <asp:Label runat="server" /> This control displays a <div> section when rendered in the browser. Once you know the names of the controls, creating them is easy. Table 5.2 summarizes the list of ASP.NET controls. Table 5.2. Web Server Controls Web Control | Description | AdRotator | Displays a predefined or random sequence of images. | Button | Used to perform a task. | Calendar | Displays a graphic calendar that allows users to select a date. | CheckBox | Displays a box that users can click to turn something on or off. | CheckBoxList | Creates a grouping of check boxes. The list control makes it easy to create multiple check boxes that belong together. | DataGrid | Displays information, usually data-bound, in tabular form with columns. Provides mechanisms to allow editing and sorting. | DataList | Like the Repeater control, but with more formatting and layout options, including the ability to display information in a table. The DataList control also allows you to specify editing behavior. | DropDownList | Allows users to either select from a list or enter text. | HyperLink | Creates Web navigation links. | Image | Displays an image. | ImageButton | Like a Button control, but incorporates an image instead of text. | Label | Displays text that users can't edit directly. | LinkButton | Like a Button control, but has the appearance of a hyperlink. | ListBox | Displays a list of choices. Optionally, the list can allow multiple selections. | Panel | Creates a borderless division on the form that serves as a container for other controls. | RadioButton | Displays a single button that can be turned on or off. | RadioButtonList | Creates a grouping of radio buttons. Within the group, only one button can be selected. | Repeater | Displays information from a data set using HTML elements and controls you specify, repeating the elements once for each record in the data set. | Table | Creates a table. | TableCell | Creates an individual cell within a table row. | TableRow | Creates an individual row within a table. | TextBox | Displays text entered at design time that can be edited by users at runtime or changed programmatically. | | Note: Although other controls allow users to edit text (for example, DropDownList), their primary purpose isn't usually text editing. | Appendix C, "ASP.NET Controls: Properties and Methods," lists the properties, methods, and events for all of these controls. Also, the DataList, DataGrid, and Repeater controls will be saved for the discussion of data binding on Day 9, "Using Databases with ASP.NET." Using Web Controls Using Web controls is just like using HTML server controls. You create them on the server and specify properties and methods to handle their events. Many of them post data to the server. You've already seen how to work with the Label and Button controls, two of the most common Web controls. Let's look at an example of some Web controls in action. Listing 5.7 shows a typical demographic form that asks for name, age, and income. Once it gets the user's input, it outputs some information dynamically. Listing 5.7 User Demographics with Web Controls 1: <%@ Page Language="VB" %> 2: 3: <script runat="server"> 4: sub Submit(Sender as Object, e as EventArgs) 5: dim strIncome as string = lbIncome.SelectedItem.Text 6: dim strAge as string = rlAge.SelectedItem.Text 7: 8: lblMessage.Text = "Hello " & tbName.Text & "!<p>" & _ 9: "Your income is: " & strIncome & "<br>" & _ 10: "Your age is: " & strAge & "<br>" 11: 12: if rlAge.SelectedIndex < 3 then 13: lblMessage.Text += "You're a young one!<p>" 14: else 15: lblMessage.Text += "You're a wise one!<p>" 16: end if 17: 18: if cbNewsletter.Checked then 19: lblMessage.Text += "You will be receiving our" & _ 20: " newsletter shortly." 21: end if 22: end sub 23: </script> 24: 25: <html><body> 26: <form runat="server"> 27: <asp:Label runat="server" 28: Height="25px" Width="100%" BackColor="#ddaa66" 29: ForeColor="white" Font-Bold="true" 30: Text="A Web Controls Example" /> 31: <br> 32: <asp:Label runat="server" /><p> 33: 34: Enter your name: 35: <asp:TextBox runat="server" /><p> 36: 37: Choose your age:<br> 38: <asp:RadioButtonList runat="server" 39: RepeatDirection="horizontal"> 40: <asp:ListItem><18</asp:ListItem> 41: <asp:ListItem>19-24</asp:ListItem> 42: <asp:ListItem>25-34</asp:ListItem> 43: <asp:ListItem>35-49</asp:ListItem> 44: <asp:ListItem>50-65</asp:ListItem> 45: </asp:RadioButtonList><p> 46: 47: Choose your income:<br> 48: <asp:ListBox runat="server" 49: size=1> 50: <asp:ListItem>< $999/year</asp:ListItem> 51: <asp:ListItem>$1000-$9999</asp:ListItem> 52: <asp:ListItem>$10000-$49999</asp:ListItem> 53: <asp:ListItem>> $50000</asp:ListItem> 54: </asp:ListBox><p> 55: 56: Do you want to receive our newsletter?<br> 57: <asp:CheckBox runat="server" 58: Text="Yes!" /><p> 59: 60: <asp:Button runat="server" 61: Text="Submit" OnClick="Submit" /> 62: </form> 63: </body></html>  | First, let's examine the HTML portion of the page. This example uses several different Web controls. Lines 27 30 display a familiar Label control, but with some additional style attributes specified. These style attributes can be used with nearly any Web control, so feel free to customize your displays. Line 32 holds another Label that you'll use to display messages. Line 35 contains a TextBox control that allows the user to enter her name. Lines 38 45 display a RadioButtonList a collective group of radio buttons with five options, denoted by the ListItem control. Lines 48 54 display a ListBox, similar to a select HTML element. This control displays four different options. On line 57 is a simple CheckBox control, and line 59 has a familiar Button control. Figure 5.9 shows what this listing looks like in the browser. | Figure 5.9. The demographic form with Web controls.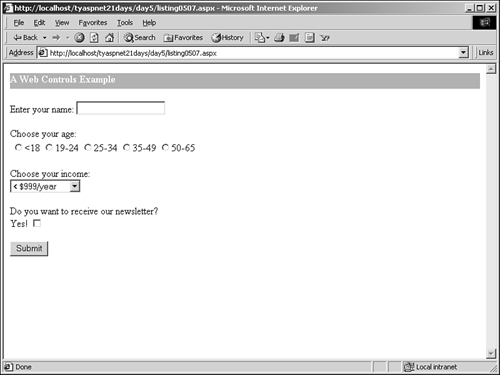 Each Web control renders to an existing HTML element, and no controls are actually sent to the browser. If you view the source from this page, you'll see that there's nothing new all of the HTML is perfectly satisfactory to the browser. In the code declaration block, you perform some actions based on the user's input. Lines 5 and 6 retrieve the selected values from the ListBox and RadioButtonList controls, respectively. You can see that these two controls share the SelectedItem property, which returns the item selected. The Text property of the selected item returns any text that was contained in the item ("< $999/year," for example). Lines 8 10 simply display the entered information back to the user. The Text property of the tbName TextBox control retrieves the user's name. The if statement on lines 12 16 displays a message depending on the user's age. The SelectedIndex property returns the index of the selected item in the rlAge RadioButtonList control. For example, the first item has an index of 0, and the last item has an index of 4. If this index is less than 3, meaning the user's age is under 35, one message is displayed, while another message is displayed if he's older. Finally, the if statement on line 18 checks the CheckBox control's Checked property to determine if the check box is checked. (Say that three times fast!) If it is, you display a message alerting the user that he'll be added to your newsletter list. Figure 5.10 shows the output after the user has entered some information and submitted the form. Figure 5.10. The submitted demographic form.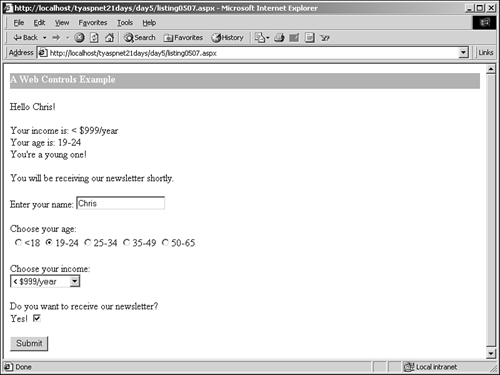 Tip Most Web controls share a common set of properties. For example, the TextBox, Label, LinkButton, Hyperlink, Button, and CheckBox controls all share the Text property, which returns or sets the text in the control. The ListBox, ComboBox, DataList, and DataGrid controls all share the SelectedItem property. This standard interface makes it very easy for you to develop pages with Web controls. You'll be using Web controls extensively throughout this book, so it pays to get comfortable with them as soon as possible. Once you master a few controls, the rest will come easily. Posting Data Immediately As mentioned earlier today, you don't have to cache events and send them to the server all at once. In fact, sometimes you may not want to wait. For example, imagine you've built a form that displays cities in a state. The two lists, cities and states, are provided in ListBox controls. Once a state is selected, you post the form, do some processing, and filter the list of cities to display only those in that particular state. In this case, you wouldn't want to wait until the user submits the form himself. You can post data immediately with the AutoPostBack property of Web controls. Setting this property to true tells the Web control to post the data as soon as any event occurs. Let's look at Listing 5.8, which relies on two events to post data. Listing 5.8 Using AutoPostBack 1: <%@ Page Language="VB" %> 2: <%@ Import Namespace="System.Drawing" %> 3: 4: <script runat="server"> 5: sub NameHandler(Sender as Object, e as EventArgs) 6: lblMessage.Text = tbName.Text & ", select a color: " 7: end sub 8: 9: sub ListHandler(Sender as Object, e as EventArgs) 10: lbColor.BackColor = Color.FromName _ 11: (Sender.SelectedItem.Text) 12: end sub 13: </script> 14: 15: <html><body> 16: <form runat="server"> 17: <asp:TextBox runat="server" 18: OnTextChanged="NameHandler" 19: AutoPostBack="true" /><p> 20: 21: <asp:Label runat="server" 22: Text="Select a color: " /><p> 23: <asp:ListBox runat="server" 24: OnSelectedIndexChanged="ListHandler" 25: AutoPostBack="true" > 26: <asp:Listitem>Red</asp:Listitem> 27: <asp:Listitem>blue</asp:Listitem> 28: <asp:Listitem>green</asp:Listitem> 29: <asp:Listitem>white</asp:Listitem> 30: </asp:ListBox> 31: </form> 32: </body></html>  | On line 17, you declare your TextBox Web control. When the TextChanged occurs, you execute the NameHandler method on line 5. Notice that the AutoPostBack property of this control is set to true, which means that a post will occur as soon as the TextChanged event occurs. | Note The form will be auto-posted only on the events that you specify handlers for. In the preceding TextBox, the form will be submitted on the TextChanged event and no other. On line 21, a Label control displays an instruction to the user. On line 23 is a ListBox control with four items, again with the AutoPostBack property set to true. Whenever the user selects a different item in the list, a post will occur and the ListHandler method will be executed. Notice that there's no Submit button, so the user cannot submit the form manually. The NameHandler method simply takes the value of the text box and adds it to the Label for a customized message. The ListHandler method sets the background color of ListBox depending on the item selected. The color can't be set by using just the color name (such as "red") by itself or by supplying a hexadecimal value (such as "#FF0000") alone. You have to use the .NET Framework's Color object to set the color. The Color.FromName method creates a Color object based on the supplied color name. Color.FromName("red") creates a red object, and so on. You can use this object to set the color of your ListBox. (The Color object belongs to the System.Drawing namespace, hence the Import directive on line 2.) Figure 5.11 shows this page after the user selects a color and inputs a value in the text box. Figure 5.11. Using AutoPostBack to submit a form.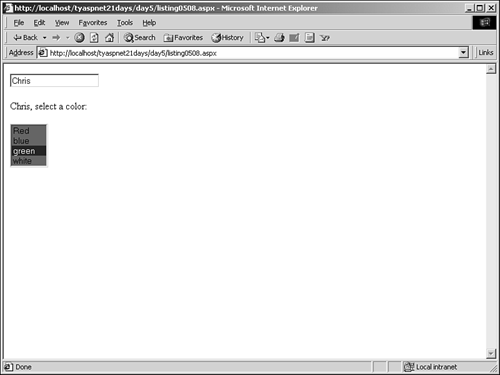 Note For the color example to work, you need to be using a browser that supports cascading style sheets (IE or Netscape 4+). Older browsers don't support setting background colors for list boxes. Web Server Controls Versus HTML Server Controls A lot of the server controls seem to provide overlapping functionality. For example, the HtmlAnchor HTML server control and the HyperLink Web control both do the same thing. When should you use one instead of the other? In general, HTML server controls make it easy to convert traditional HTML forms to Web forms. It's very simple to just add runat="server" to each HTML element and gain access to it programmatically. This makes converting old pages a snap and adds power to your applications. One drawback of HTML server controls is that they don't provide a standard interface. HTML elements provide many different attributes and keywords to set properties, which may be confusing at times. Also, HTML server controls don't provide down-level support inherently, so ASP.NET won't generate different HTML for each browser version automatically. This gives you less automatic control, but it allows you to customize the pages manually. Web controls should be used when you're building pages from scratch. They usually provide a more powerful mechanism than HTML server controls, and they support a standard interface. They also provide down-level support. Do | Don't | Do use regular HTML elements when you don't need to manipulate them programmatically. For example, normally you wouldn't need to manipulate a link, so just use a standard <a href> tag instead of an HtmlAnchor or HyperLink control. | Don't create unnecessary server controls (that is, controls that you'll never access programmatically). The more objects the server needs to create, the more resources are required. The performance loss is very small, but it still isn't worth it. | Do use HTML server controls when you don't want to spend a lot of time converting existing pages to Web forms. | | Do use Web server controls when you're creating new Web forms that require programmatic access. | | Do convert existing elements to Web server controls instead of HTML server controls, if you have the time. | | | |