Objects are simply classes developed in VB.NET or C# (or whatever language you're most comfortable with), organized into logical groups. Let's get right into development. Listing 15.1 shows the framework for a simple object in VB.NET, and Listing 15.2 shows the same object in C#. Listing 15.1 A Sample Framework for a Business Object in VB.NET 1: Imports System 2: Imports System.Data 3: Imports System.Data.OleDb 4: 5: Namespace TYASPNET 6: 7: Public Class Database 8: End Class 9: 10: End Namespace Listing 15.2 A Sample Framework for a Business Object in C# 1: using System; 2: using System.Data; 3: using System.Data.OleDb; 4: 5: namespace TYASPNET { 6: 7: public class Database { 8: } 9: 10: }  | Save this file as Database.vb or (Database.cs for Listing 15.2). This object will be used to provide abstract database functionality for your pages, such as connecting to, querying, and returning data. In other words, it represents a real-world database and should have all the properties and methods that define a database. | First, you import the namespaces you'll be using in your object. In this case, you'll need System, System.Data, and System.Data.OleDb (lines 1, 2, and 3, respectively). On line 5, you declare the namespace that this current object belongs to. Where did the namespace TYASPNET come from? Nowhere you created it just now. It's that easy to extend the .NET Framework simply declaring that you're using a new namespace creates it automatically. When you develop more objects for your applications, you can insert them into this namespace as well. Thus, it serves as a logical group for all your business logic. Note For example, the System.Data.OleDb namespace has many classes, including OleDbCommand, OleDbConnection, and OleDbDataAdapter. Namespaces are simply used to group classes logically. If you wanted to, you could have specified an existing namespace, such as System.Web. Your object would be added to that namespace. Remember, though, that namespaces are for logical grouping of objects. The object you're developing doesn't belong in any existing namespace, so you create your own. You can have a different namespace for every ASP.NET application you develop! Do | Don't | Do create unique namespaces for objects in your applications. | Don't use existing namespaces for your objects. It will end up confusing you and could create problems. | | Finally, you declare your class, which currently is empty, on line 7. You'll be adding to it shortly. Don't forget to write the closing class and namespace tags. Let's compile this object so you can use it in your ASP.NET pages. To do this, you'll be using the VB.NET compiler that comes with the .NET Framework SDK. Open a command prompt window by clicking Start, Run and then typing cmd.exe. First, navigate to the root directory of your application (c:\inetpub\wwwroot\) and create a new bin directory with the following command: mkdir bin You'll place your business objects here in a moment. Next, navigate to the directory you saved this file in for instance, c:\inetpub\wwwroot\tyaspnet21days\day15. Type the following command and press Enter: vbc /t:library /out:..\..\bin\TYASPNET21Days.dll /r:System.dll /r:System.Data.dll Database.vb Or for the C# version: csc /t:library /out:..\..\bin\TYASPNET21Days.dll /r:System.dll /r:System.Data.dll Database.cs The VB.NET and C# compilers have a lot of options and are very complex, so this section will only examine the options used with this particular command. (Most likely, these will be all you ever need for ASP.NET development.) vbc.exe is the name of the VB.NET compiler. csc.exe is the name of the C# compiler. You use these program to compile your file into a DLL (also known as an assembly) for use with your application. /t specifies the type of file you want to compile to. library means an object that will be used by other applications it can't be used by itself. The /t option can also be exe or winexe, which creates an executable file. /out specifies the directory and filename where you want to send the compiled output. You need to place this in your /bin directory, so you specify the path relative to the current directory. The /r option means reference. In Listing 15.1, you referenced the System, System.Data, and System.Data.OleDb namespaces. These namespaces are stored in the System.dll and System.Data.dll files, so you add references to both of them when compiling. Caution Remember to use references in your compilation command, and not just in the Import command in your code. Without these references, the Import commands would do nothing and you would receive errors when you tried to compile your object. Finally, you specify the file that you want to compile, Database.vb. This class file will be compiled and loaded into your assembly cache, for use with your pages. You should see something similar to Figure 15.2. Figure 15.2. Using the VB.NET compiler to build your objects.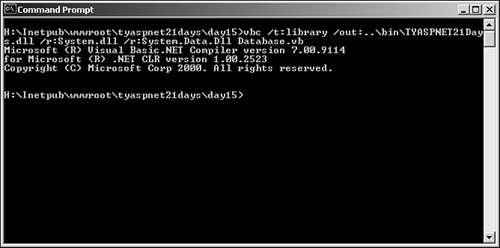 Note Don't worry if you're not very comfortable using the VB.NET compiler. You can usually use the same command over and over again, and where the syntax is different, it will be pointed out. For more information, see the .NET Framework SDK documentation. Listing 15.3 shows a simple ASP.NET page that implements this object. Listing 15.3 Using the Business Object 1: <%@ Page Language="VB" %> 2: 3: <script runat="server"> 4: sub Page_Load(Sender as Object, e as EventArgs) 5: dim objDatabase as new TYASPNET.Database 6: lblMessage.Text = "Object created" 7: end sub 8: </script> 9: 10: <html><body> 11: <asp:Label runat="server" /> 12: </body></html>  | On line 5, you declare a new object based on the business object you just created. You can't do anything with this object yet because you didn't implement any methods for it. You'll add some methods later today in "Developing Business Objects." | Note that you use the full name TYASPNET.Database for your object. You could simply use the object name (without the namespace name) by importing your custom namespace, just as you've done for other namespaces. For example, let's say you add the following code to line 2: <%@ Import Namespace="TYASPNET" %> This would allow you to use the following on line 5: dim objDatabase as new Database |