Changing the direction of the chapter just a bit, I'll introduce a couple of PHP's date- and time-related functions. The most important of these is the aptly named date() function, which returns a string of text for a certain date and time according to a format you specify. date ('format', [timestamp]); The timestamp is an optional argument representing the number of seconds since the Unix Epoch (midnight on January 1, 1970) for the date in question. It allows you to get information, like the day of the week, for a particular date. If a timestamp is not specified, PHP will just use the current time on the server. There are myriad formatting parameters available (Table 3.1), and these can be used in conjunction with literal text. For example, echo date('F j, Y'); // January 26, 2005 echo date('H:i'); // 23:14 echo date('Today is D'); // Today is Mon Table 3.1. The date() function can take any combination of these parameters to determine its returned results.Date Function Formatting |
---|
CHARACTER | Meaning | Example |
---|
Y | year as 4 digits | 2005 | y | year as 2 digits | 05 | n | month as 1 or 2 digits | 2 | m | month as 2 digits | 02 | F | month | February | M | month as 3 letters | Feb | j | day of the month as 1 or 2 digits | 8 | d | day of the month as 2 digits | 08 | l (lowercase L) | day of the week | Monday | D | day of the week as 3 letters | Mon | g | hour, 12-hour format as 1 or 2 digits | 6 | G | hour, 24-hour format as 1 or 2 digits | 18 | h | hour, 12-hour format as 2 digits | 06 | H | hour, 24-hour format as 2 digits | 18 | i | minutes | 45 | s | seconds | 18 | a | am or pm | am | A | AM or PM | PM |
You can find the timestamp for a particular date using the mktime() function. $stamp = mktime (hour, minute, second, month, day, year); Finally, the geTDate() function can be used to return an array of values (Table 3.2) for a date and time. For example, $dates = getdate(); echo $dates['month']; // January Table 3.2. The geTDate() function returns this associative array.The getdate() Array |
---|
KEY | VALUE | EXAMPLE |
---|
year | year | 2005 | mon | month | 12 | month | month name | December | mday | day of the month | 25 | weekday | day of the week | Tuesday | hours | hours | 11 | minutes | minutes | 56 | seconds | seconds | 47 |
This function also takes an optional timestamp argument. If that argument is not used, getdate() returns information for the current date and time. To practice working with these functions, the simple dateform.php script will be rewritten to preselect the current date. To use the date functions 1. | Open dateform.php (Script 3.7) in your text editor.
| 2. | Change the function definition to begin (Script 3.12).
function make_calendar_pulldowns ($m = NULL, $d = NULL, $y = NULL) { Script 3.12. This script makes use of the date() and geTDate() functions. 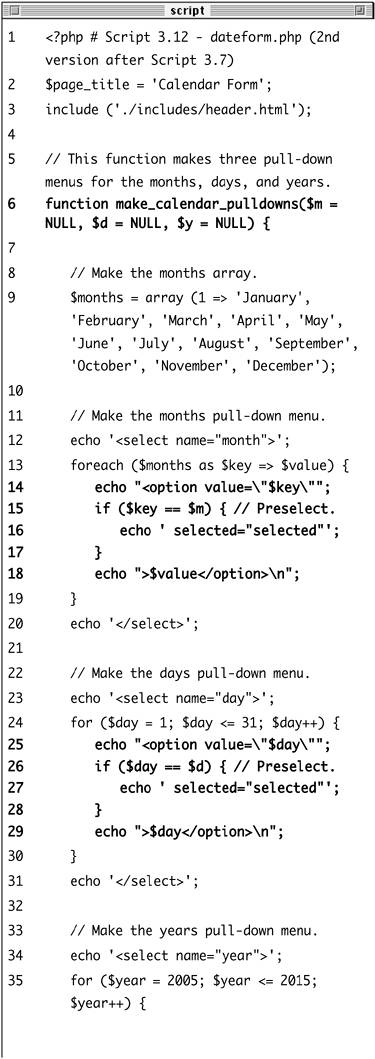 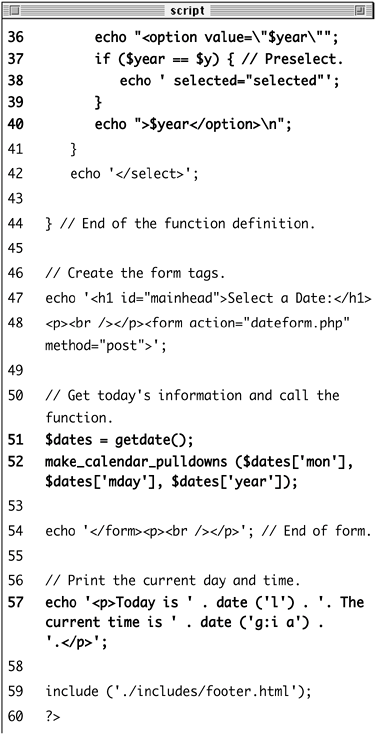
Now this function will be able to take three arguments: a day, a month, and a year. The purpose of these will be to preset the pull-down menu values, as if this were part of a sticky form.
| 3. | Change the months foreach loop so that it pre-selects a particular month.
foreach ($months as $key => $value) { echo "<option value=\"$key\""; if ($key == $m) { echo ' selected="selected"'; } echo ">$value</option>\n"; } The code is as it was before except for the inclusion of the conditional. If the function is called with an $m value (for a particular month), the corresponding month should be selected in the form. To test for this, see if $m is equal to $key, where $key is the array's key, a value from 1 to 12. In such a case, the selected="selected" code will be added to the appropriate option tag.
| 4. | Modify the day for loop so that it is also sticky.
for ($day = 1; $day <= 31; $day++) { echo "<option value=\"$day\""; if ($day == $d) { echo ' selected="selected"'; } echo ">$day</option>\n"; } This is exactly like the months foreach loop except that it's a simple for loop. If the $day value is equal to the $d value, assigned when calling the function, the selected="selected" is added to the option tag.
| 5. | Modify the year for loop so that it is also sticky.
for ($year = 2005; $year <= 2015; $year++) { echo "<option value=\"$year\""; if ($year == $y) { echo ' selected="selected"'; } echo ">$year</option>\n"; }
| 6. | Alter the function call line so that it is called with today's date.
$dates = getdate(); make_calendar_pulldowns ($dates['mon], $dates['mday'], $dates['year]); To send the month, day, and year parameters to the function, I'll first use the getdate() function to retrieve an array of values for today. Then I call the function, supplying the appropriate array elements.
| 7. | Print the current day and time.
echo '<p>Today is ' . date ('l') . '. The current time is ' . date ('g:i a) . '.</p>'; This final string will print something along the lines of <p>Today is Tuesday. The current time is 11:14 pm.</p> using the appropriate formatting parameters for the date() function.
| 8. | Save the file as dateform.php, upload to your Web server, and test in your Web browser (Figure 3.19).
Figure 3.19. The date() and getdate() functions help add some dynamic behavior to this page. 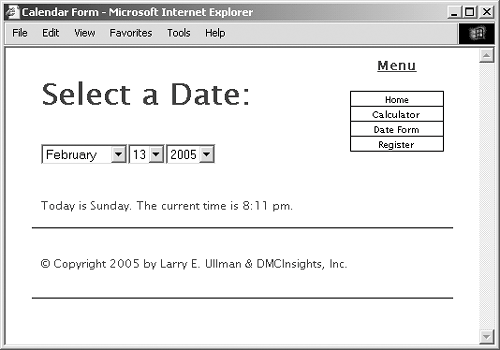
| 9. | If you want, view the source code of the page to see how the select menus were made sticky (Figure 3.20).
Figure 3.20. The source code reveals how the selected="selected" attribute makes the pull-down menus sticky. 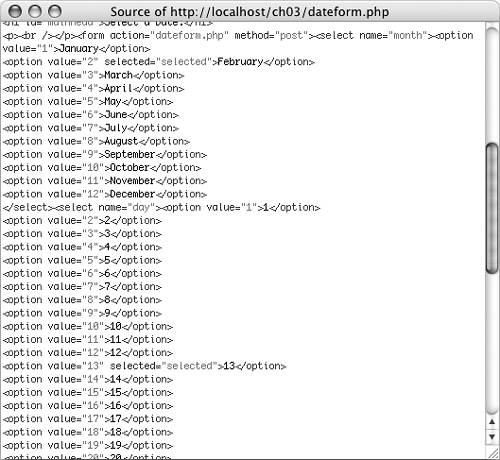
| Tips As you'll see in Chapter 4, "Introduction to SQL and MySQL," MySQL has its own date functions. PHP's date functions reflect the time on the server (because PHP runs on the server); you'll need to use JavaScript if you want to determine the date and time on the client computer. The checkdate() function takes three parametersmonth, day, and yearand checks if it is a valid date (one that actually exists or existed).
|