The firstarguably most importantvariable type I'll go into is strings. A string is merely a quoted chunk of letters, numbers, spaces, punctuation, and so forth. These are all strings: 'Tobias' "In watermelon sugar" '1,000' 'February 3, 2005' To make a string variable, assign a string value to a valid variable name: $first_name = 'Tobias'; $today = 'February 3, 2005'; To print out the value of a string, use either echo() or print(): echo $first_name; To print the value of string within a context, use double quotation marks: echo "Hello, $first_name"; In a way, you've already worked with strings oncewhen using the predefined variables in the preceding section. To use strings 1. | Create a new HTML document in your text editor, along with the PHP tags (Script 1.7).
<!DOCTYPE html PUBLIC "-//W3C// DTD XHTML 1.0 Transitional//EN "http://www.w3.org/TR/xhtml1/DTD/ xhtml1-transitional.dtd> <html xmlns="http://www.w3.org/1999/ xhtml xml:lang="en" lang="en"> <head> <meta http-equiv="content-type" content="text/html; charset=iso-8859-1 /> <title>Strings</title> </head> <body> <?php # Script 1.7 - strings.php ?> </body> </html> Script 1.7. String variables are created and their values sent to the Web browser in this introductory script. 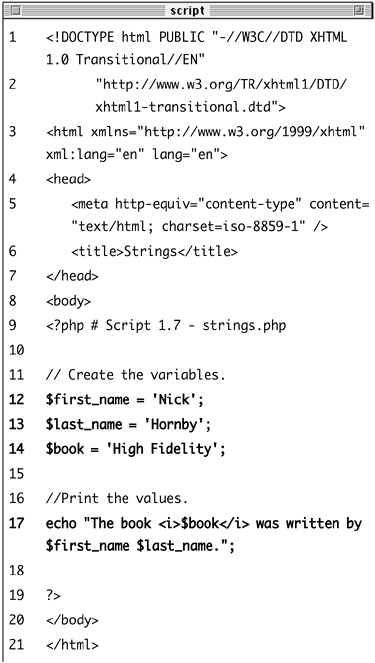
| 2. | Within the PHP tags, create three variables.
$first_name = 'Nick'; $last_name = 'Hornby'; $book = 'High Fidelity'; In this rudimentary example, I'm going to create $first_name, $last_name, and $book variables that will then be printed out in a message.
| 3. | Create the echo() statement.
echo "The book <i>$book</i> was written by $first_name $last_name."; All this script does is to print a statement of authorship based upon three established variables. A little HTML formatting (the italics) is thrown in to make it more attractive. Remember to use double quotation marks here for the variable values to be printed out appropriately (more on the importance of double quotation marks at the chapter's end).
| 4. | Save your file as strings.php, upload to your Web server, and test in your Web browser (Figure 1.15).
Figure 1.15. The resulting Web page is based upon printing out the values of three variables. 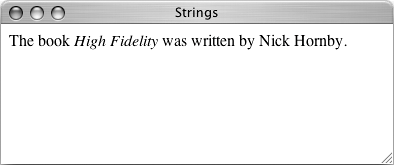
| 5. | If desired, change the values of your three variables and run the script again (Figure 1.16).
Figure 1.16. The output of the script is changed by altering the variables in it. 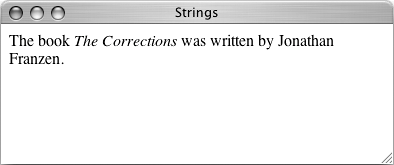
| Tips If you assign another value to an existing variable (say $book), the new value will overwrite the old one. For example: $book = 'High Fidelity'; $book = 'The Corrections'; /* $book now has a value of 'The Corrections'. */
PHP has no set limits on how big a string can be. It's theoretically possible that you'll be limited by the resources of the server, but it's doubtful that you'll ever encounter such a problem.
Concatenating strings Concatenationan important tool when creating dynamic Web sitesis like addition for strings and is performed using the concatenation operator: the period (.). $city= 'Seattle'; $state = 'Washington'; $address = $city_name . $state; The $address variable now has the value SeattleWashington, which almost achieves the desired result (Seattle, Washington). To improve upon this, I could write $address = $city . ', ' . $state; so that a space is added to the mix. Concatenation also works with strings or numbers. Either of these statements will produce the same result (Seattle, Washington 98101): $address = $city . ', ' . $state . ' 98101; $address = $city . ', ' . $state . ' ' . 98101; Concatenation is commonly used with string variables and will be used extensively when building database queries in later chapters. In the meantime, I'll modify the strings.php script to use this new tool. To use concatenation 1. | Open strings.php (refer to Script 1.7) in your text editor.
| 2. | After you've established the $first_name and $last_name variables (lines 12 and 13), add (Script 1.8).
$author = $first_name . ' ' . $last_name; Script 1.8. Concatenation gives you the ability to easily manipulate strings, like creating an author's name from the combination of their first and last names. 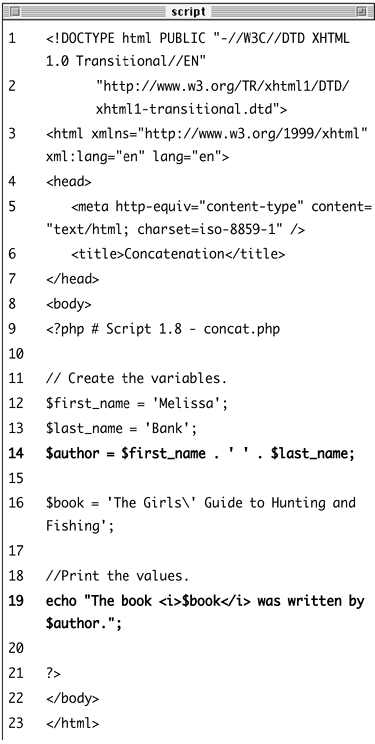
As a demonstration of concatenation, a new variable$authorwill be created as the concatenation of two existing strings and a space in between.
| 3. | Change the echo() statement to use this new variable.
echo "The book <i>$book</i> was written by $author."; Since the two variables have been turned into one, the echo() statement should be altered accordingly.
| 4. | If desired, change the HTML page title and the values of the first name, last name, and book variables.
| 5. | Save your file as concat.php, upload to your Web server, and test in your Web browser (Figure 1.17).
Figure 1.17. The end result of concatenation may never be apparent to the user (compare with Figures 1.15 and 1.16). 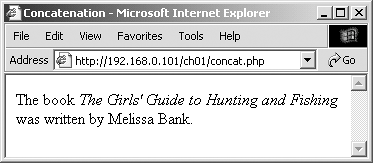
| Tips PHP has a slew of useful string-specific functions, which you'll see over the course of this book. For example, to calculate how long a string is (how many characters it contains), use strlen(): $num = strlen($string);
You can have PHP convert the case of your strings with: strtolower(), which makes it entirely lowercase; strtoupper(), which makes it entirely uppercase; ucfirst(), which capitalizes the first character; and ucwords(), which capitalizes the first character of every word. If you are merely concatenating one value to another, you can use the concatenation assignment operator (.=). The following are equivalent: $title = $title . $subtitle; $title .= $subtitle;
The initial example in this section could be rewritten using either $address = "$city, $state";
or $address = $city; $address .= ', '; $address .= $state;
The concatenation operator can be used when calling functions, like so: $num = strlen ($first_name . $last_name);
|