When I began discussing variables, I was explicit in stating that PHP has both integer and floating-point (decimal) number types. In my experience, though, these two types can be classified under the generic title numbers without losing any valuable distinction (for the most part). Valid number-type variables in PHP can be anything like 8 3.14 10980843985 -4.2398508 4.4e2 Notice that these values are never quotedin which case they'd be strings with numeric valuesnor do they include commas to indicate thousands. Also, a number is assumed to be positive unless it is preceded by the minus sign (-). Along with the standard arithmetic operators you can use on numbers (Table 1.1), there are dozens of functions. Two I'll introduce here are round() and number_format(). The former rounds a decimal either to the nearest integer $n = 3.14; $n = round ($n); // 3 Table 1.1. The standard mathematical operators.Arithmetic Operators |
---|
OPERATOR | MEANING |
---|
+ | Addition | - | Subtraction | * | Multiplication | / | Division | % | Modulus | ++ | Increment | -- | Decrement |
or to a specified number of decimal places $n = 3.142857; $n = round ($n, 3); // 3.143 The number_format() function turns a number into the more commonly written version, grouped into thousands using commas. For example: $n = 20943; $n = number_format ($n); // 20,943 This function can also set a specified number of decimal points $n = 20943; $n = number_format ($n, 2); // 20,943.00 To practice with numbers, I'll write a mock-up script that performs the calculations one might use in an e-commerce shopping cart. To use numbers 1. | Create a new PHP document in your text editor (Script 1.9).
<!DOCTYPE html PUBLIC "-//W3C// DTD XHTML 1.0 Transitional//EN "http://www.w3.org/TR/xhtml1/DTD/ xhtml1-transitional.dtd> <html xmlns="http://www.w3.org/1999/ xhtml xml:lang="en" lang="en"> <head> <meta http-equiv="content-type" content="text/html; charset=iso-8859-1 /> <title>Numbers</title> </head> <body> <?php # Script 1.9 - numbers.php Script 1.9. The numbers.php script demonstrates the basics of an e-commerce calculator. 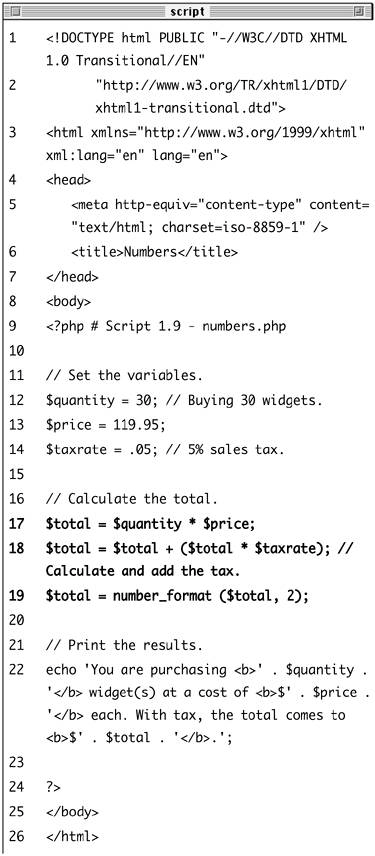
| 2. | Establish the requisite variables.
$quantity = 30; $price = 119.95; $taxrate = .05; This script will use three hard-coded variables upon which calculations will be made. Throughout the rest of the book, you'll see how these values can be dynamically determined (i.e., by user interaction with an HTML form).
| 3. | Perform the calculations.
$total = $quantity * $price; $total = $total + ($total * $taxrate); The first line establishes the order total as the number of widgets purchased multiplied by the price of each widget. The second line then adds the amount of tax to the total (calculated by multiplying the tax rate by the total).
| 4. | Format the total.
$total = number_format ($total, 2); The number_format() function will group the total into thousands and round it to two decimal places. This will make the display more appropriate to the end user.
| 5. | Print the results.
echo 'You are purchasing <b>' . $quantity . '</b> widget(s) at a cost of <b>$' . $price . '</b> each. With tax, the total comes to <b>$' . $total . '</b>.'; The last step in the script is to print out the results. To use a combination of HTML, printed dollar signs, and variables, I feed the code to the echo() statement using a combination of single-quoted text and concatenated variables.
You could also put this all within a double-quoted string (as in previous examples), but when PHP encounters, for example, at a cost of $$price in the echo() statement, the double dollar sign would cause problems. You'll see an alternative solution in the last example of this chapter.
| 6. | Complete the PHP code and the HTML page.
?> </body> </html>
| 7. | Save the file as numbers.php, upload to your Web server, and test in your Web browser (Figure 1.18).
Figure 1.18. The numbers script performs calculations based upon set values. 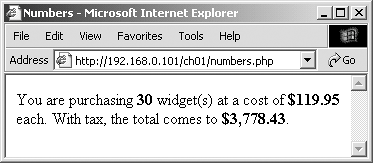
| 8. | If desired, change the initial three variables and rerun the script (Figure 1.19).
Figure 1.19. To change the generated Web page, alter any or all of the three variables (compare with Figure 1.18). 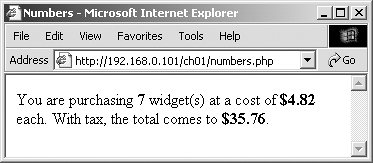
| Tips PHP supports a maximum integer of around two billion on most platforms. With numbers larger than that, PHP will automatically use a floating-point type. When dealing with arithmetic, the issue of precedence arises (the order in which complex calculations are made). While the PHP manual and other sources tend to list out the hierarchy of precedence, I find programming to be safer and more legible when I group clauses in parentheses to force the execution order (see line 18). Computers are notoriously poor at dealing with decimals. For example, the number 2.0 may actually be stored as 1.99999. Most of the time this won't be a problem, but in cases where mathematical precision is paramount, rely on integers, not decimals. The PHP manual has information on this subject, as well as alternative functions for improving computational accuracy. Many of the mathematical operators also have a corresponding assignment operator, letting you create a shorthand for assigning values. This line $total = $total + ($total * $taxrate);
could be rewritten as $total += ($total * $taxrate);
If you set a $price value without using two decimals (e.g., 119.9 or 34), you may want to apply number_format() to $price before printing it. If you use concatenation, the number_format()or any otherfunction can be used inline with an echo() statement: echo 'You are purchasing <b>' . $quantity . '</b> widget(s) at a cost of <b>$' . number_ format($price) . '</b> each. With tax, the total comes to <b>$' . number_format($total) . '</b>.';
|