In Chapter 9, I wrote many versions of the login.php and logout.php scripts, using variations on cookies and sessions. Here I'll develop standardized versions of both that adhere to the same practices as the whole application. The login query itself is slightly different here in that it also checks that the active column has a NULL value, which means that the user has activated their account. To write login.php 1. | Create a new document in your text editor (Script 13.8).
<?php # Script 13.8 - login.php Script 13.8. The login form will redirect the user to the home page after registering the first name and user ID values in a session 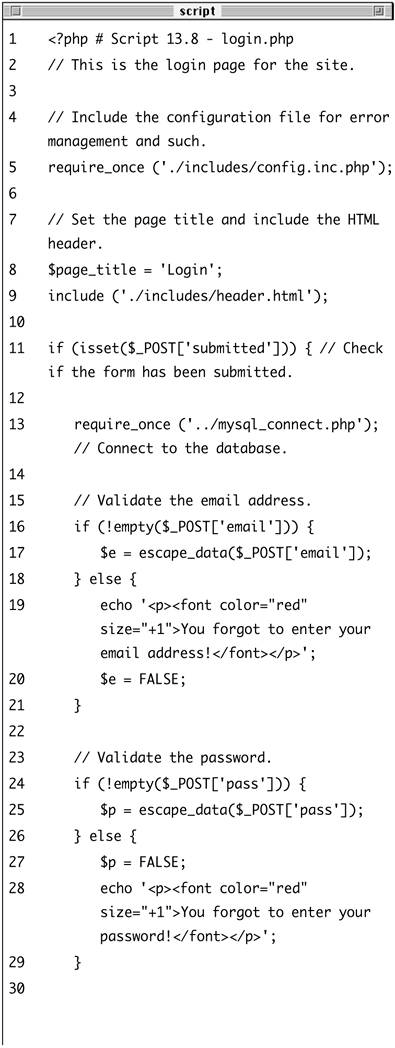 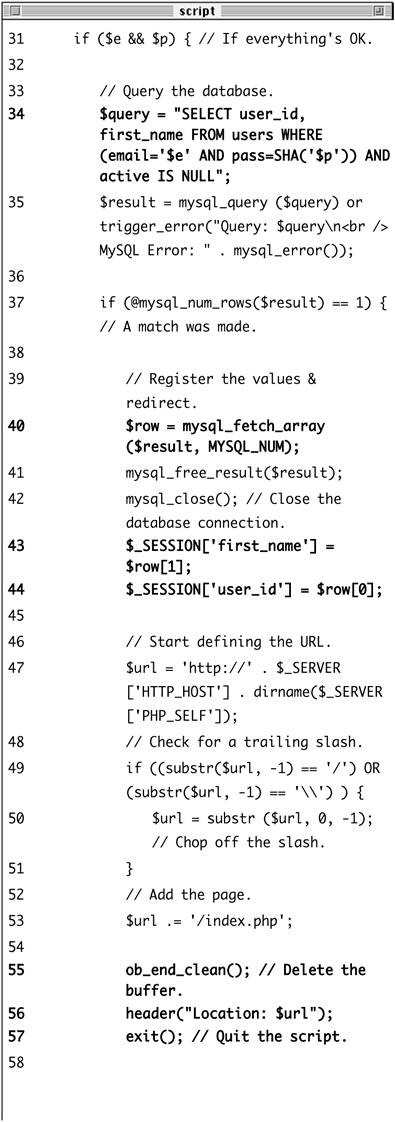 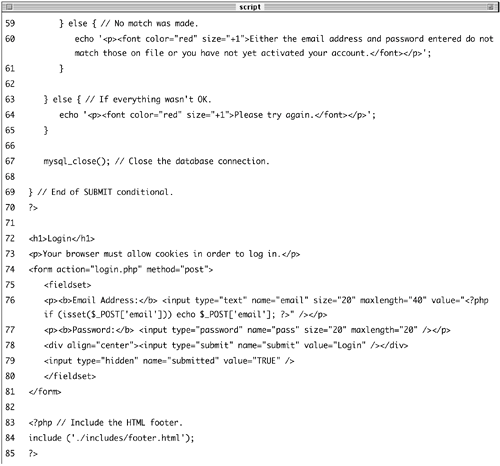
| 2. | Require the configuration file and the HTML header.
require_once ('./includes/config. inc.php); $page_title = 'Login'; include ('./includes/header.html');
| 3. | Check if the form has been submitted, require the database connection, and validate the submitted data.
if (isset($_POST['submitted'])) { require_once ('../mysql_connect. php); if (!empty($_POST['email'])) { $e = escape_data($_POST ['email]); } else { echo '<p><font color="red" size="+1>You forgot to enter your email address!</font> </p>'; $e = FALSE; } if (!empty($_POST['pass'])) { $p = escape_data($_POST ['pass]); } else { $p = FALSE; echo '<p><font color="red" size="+1>You forgot to enter your password!</font></p>'; } I could certainly validate the submitted data using regular expressions; however, that level of testing is unwarranted and will slow down the login process. If, for example, the user enters 12345 as their email address, then the login query will not return a match anyway. Therefore, it's unnecessary to apply a regular expression first.
If the user does not enter any values into the form, error messages will be printed (Figure 13.19).
Figure 13.19. The login form checks only if values were entered, without using regular expressions. 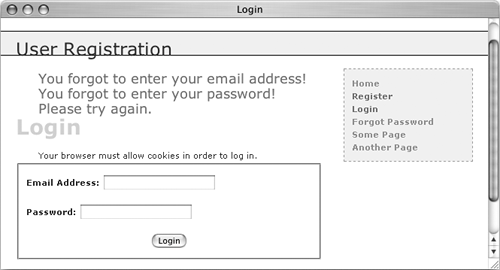
| 4. | If both validation routines were passed, retrieve the user information.
if ($e && $p) { $query = "SELECT user_id, first_ name FROM users WHERE (email= '$e AND pass=SHA('$p')) AND active IS NULL; $result = mysql_query ($query) or trigger_error("Query: $query\n <br />MySQL Error: " . mysql_ error()); The query will attempt to retrieve the user ID and first name for the record whose email address and password match those submitted. The MySQL query uses the SHA() function on the pass column, as the password is encrypted using that function in the first place. The query also checks that the active column has a NULL value, meaning that the user has successfully accessed the activate.php page.
| 5. | If a match was made in the database, log the user in and redirect them.
if (@mysql_num_rows($result) == 1) { $row = mysql_fetch_array ($result, MYSQL_NUM); mysql_free_result($result); mysql_close(); $_SESSION['first_name'] = $row[1]; $_SESSION['user_id'] = $row[0]; $url = 'http://' . $_SERVER ['HTTP_HOST] . dirname($_SERVER ['PHP_SELF]); if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); } $url .= '/index.php'; ob_end_clean(); header("Location: $url"); exit(); The login process consists of storing the retrieved values in the session (which was already started in header.html) and then redirecting the user to the home page. The ob_end_clean() function will delete the existing buffer (the output buffering is also begun in header.html), since it will not be used. The redirection code is in accordance with the preferred method, as described in Chapter 8.
| 6. | Complete the conditionals and close the database connection.
} else { echo '<p><font color="red" size="+1>Either the email address and password entered do not match those on file or you have not yet activated your account. </font></p>'; } } else { echo '<p><font color="red" size="+1>Please try again. </font></p>'; } mysql_close(); } ?> The error message (Figure 13.20) indicates that the login process could fail for two possible reasons. One is that the submitted email address and password do not match those on file. The other reason is that the user has not yet activated their account.
Figure 13.20. An error message is displayed if the submitted username and password do not match any registered values or if the account has not yet been activated. 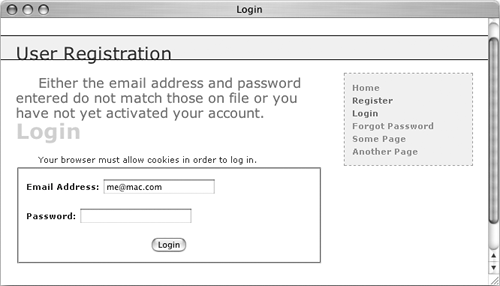
| 7. | Display the HTML login form (Figure 13.21).
<h1>Login</h1> <p>Your browser must allow cookies in order to log in.</p> <form action="login.php" method="post> <fieldset> <p><b>Email Address:</b> <input type="text name="email" size="20 maxlength="40" value="<?php if (isset($_POST ['email])) echo $_POST ['email]; ?>" /></p> <p><b>Password:</b> <input type="password name="pass" size="20 maxlength="20" /></p> <div align="center"><input type="submit name="submit" value="Login /></div> <input type="hidden" name= "submitted value="TRUE" /> </fieldset> </form> Figure 13.21. The login form. 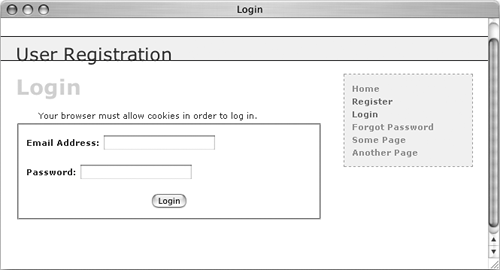 The login form, like the registration form, will submit the data back to itself. It also remembers the submitted email address, should there be a problem and the form is displayed again.
Notice that I add a message informing the user that cookies must be enabled to use the site (if a user does not allow cookies, the user will never get access to the logged-in user pages).
| 8. | Include the HTML footer.
<?php include ('./includes/footer.html'); ?>
| 9. | Save the file as login.php, upload to your Web server, and test in your Web browser (Figure 13.22).
Figure 13.22. Upon successfully logging in, the user will be redirected to the home page, where they will be greeted by name. 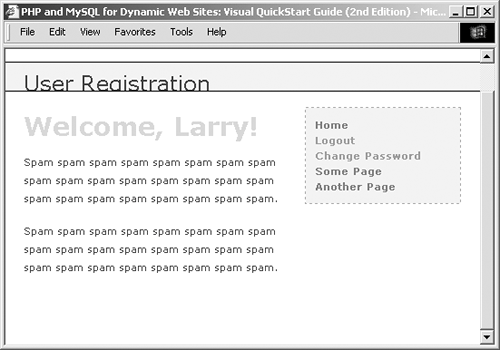
| To write logout.php 1. | Create a new document in your text editor and include the necessary files (Script 13.9).
<?php # Script 13.9 - logout.php require_once ('./includes/config. inc.php); $page_title = 'Logout'; include ('./includes/header.html'); Script 13.9. The logout form destroys all of the session information, including the cookie. 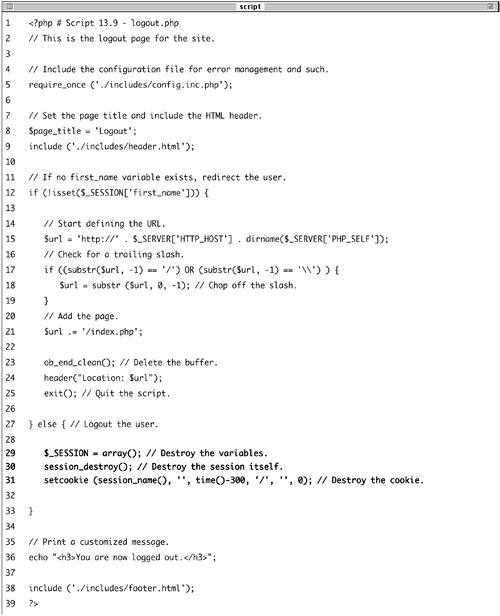 | 2. | Redirect the user if they are not logged in.
if (!isset($_SESSION['first_name'])) { $url = 'http://' . $_SERVER ['HTTP_HOST] . dirname($_SERVER ['PHP_SELF]); if ((substr($url, -1) == '/') OR (substr($url, -1) == '\\') ) { $url = substr ($url, 0, -1); } $url .= '/index.php'; ob_end_clean(); header("Location: $url"); exit(); If the user is not currently logged in (determined by checking for a $_SESSION['first_name'] variable), the user will be redirected to the home page.
| 3. | Log out the user if they are currently logged in.
} else { $_SESSION = array(); session_destroy(); setcookie (session_name(), '', time()-300, '/', '', 0); } To log the user out, the session values will be reset, the session data will be destroyed on the server, and the session cookie will be deleted. These lines of code were first used and described in Chapter 9.
| 4. | Print a logged-out message and complete the PHP page.
echo "<h3>You are now logged out. </h3>"; include ('./includes/footer.html'); ?>
| 5. | Save the file as logout.php, upload to your Web server, and test in your Web browser (Figure 13.23).
Figure 13.23. The results of successfully logging out. 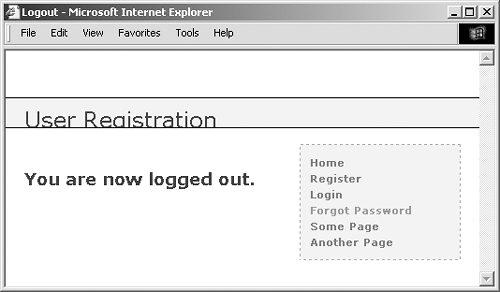
| Tips If you added a loggedin field to the users table, you could set this to 1 when a user logs in, and then be able to count how many people are currently online at any given moment. Set the value to 0 when the user logs out. By adding a last_login DATETIME field to the users table, you could update it when a user logs in. Then you would know the last time a person accessed the site and have an alternative method for counting how many users are currently logged in (say, everyone that logged in within the past so many minutes).
|