[Page 402 (continued)] Table 7.21 contains some lengths in terms of feet. Write a program that displays the nine different units of measure; requests the unit to convert from, the unit to convert to, and the quantity to be converted, and then displays the converted quantity. A typical outcome is shown in Figure 7.10. Table 7.21. Equivalent lengths.1 inch = .0833 foot | 1 rod = 16.5 feet | 1 yard = 3 feet | 1 furlong = 660 feet | 1 meter = 3.28155 feet | 1 kilometer = 3281.5 feet | 1 fathom = 6 feet | 1 mile = 5280 feet |
Figure 7.10. Possible outcome of Programming Project 1. 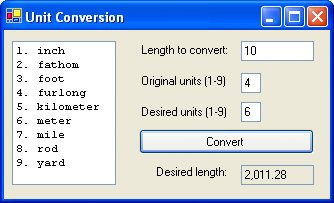 Statisticians use the concepts of mean and standard deviation to describe a collection of data. The mean is the average value of the items, and the standard deviation measures the spread or dispersal of the numbers about the mean. Formally, if x1,x2,x3,...,xn,is a collection of data, then 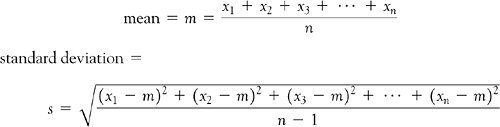
[Page 403]Write a computer program to place the exam scores 59, 60, 65, 75, 56, 90, 66, 62, 98, 72, 95, 71, 63, 77, 65, 77, 65, 50, 85, and 62 into an array; calculate the mean and standard deviation of the exam scores; assign letter grades to each exam score, ES, as follows: ES m + 1.5s | A | m + .5s ES < m + 1.5s | B | m .5s ES <m + .5s | C | m 1.5s ES m .5s | D | ES < m 1.5s | F |
For instance, if m were 70 and s were 12, then grades of 88 or above would receive A's, grades between 76 and 87 would receive B's, and so on. A process of this type is referred to as curving grades. Display a list of the exam scores along with their corresponding grades as shown in Figure 7.11. Figure 7.11. Output of Programming Project 2. 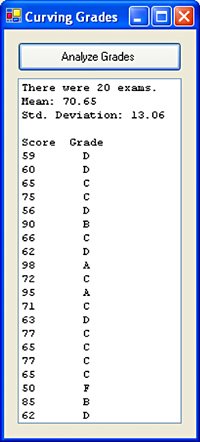
[Page 404]Rudimentary Translator. Table 7.22 gives English words and their French and German equivalents. Store these words in a text file and read them into a structure having a member for each language. Write a program that sorts arrays according to the English words. The program should then request an English sentence as input from the keyboard and translate it into French and German. For example, if the English sentence given is MY PENCIL IS ON THE TABLE, then the French translation will be MON CRAYON EST SUR LA TABLE, and the German translation will be MElN BLEISTIFT IST AUF DEM TISCH. Note: Assume that the only punctuation in the sentence is a period at the end of the sentence. Use a binary search to locate each English word. Table 7.22. English words and their French and German equivalents.English | French | German | English | French | German |
---|
YES | OUI | JA | LARGE | GROS | GROSS | TABLE | TABLE | TISCH | NO | NON | NEIN | THE | LA | DEM | HAT | CHAPEAU | HUT | IS | EST | IST | PENCIL | CRAYON | BLEISTIFT | YELLOW | JAUNE | GELB | RED | ROUGE | ROT | FRIEND | AMI | FREUND | ON | SUR | AUF | SICK | MALADE | KRANK | AUTO | AUTO | AUTO | MY | MON | MEIN | OFTEN | SOUVENT | OFT |
Write a program that allows a list of no more than 50 soft drinks and their percent changes in sales volume for a particular year to be input and displays the information in two lists titled gainers and losers. Each list should be sorted by the amount of the percent change. Try your program on the data for the seven soft drinks in Table 7.23. Note: You will need to store the data initially in an array to determine the number of gainers and losers. Table 7.23. Changes in sales volume from 2002 to 2003 of leading soft-drink brands.Brand | % Change in Volume | Brand | % Change in Volume |
---|
Coke Classic | -3.0 | Sprite | -5.0 | Pepsi-Cola | -4.5 | Dr. Pepper | -3.9 | Diet Coke | 5.0 | Diet Pepsi | 6.1 | Mt. Dew | -1.5 | Source: Beverage Digest, 2004 |
Each team in a six-team soccer league played each other team once. Table 7.24 shows the winners. Write a program to Place the team names in an array of structures that also holds the number of wins. Place the data from Table 7.24 in a two-dimensional array. [Page 405]Table 7.24. Soccer league winners. | Jazz | Jets | Owls | Rams | Cubs | Zips |
---|
Jazz | | Jazz | Jazz | Rams | Cubs | Jazz | Jets | Jazz | | Jets | Jets | Cubs | Zips | Owls | Jazz | Jets | | Rams | Owls | Owls | Rams | Rams | Jets | Rams | | Rams | Rams | Cubs | Cubs | Cubs | Owls | Rams | | Cubs | Zips | Jazz | Zips | Owls | Rams | Cubs | |
Place the number of games won by each team in the array of structures. Display a listing of the teams giving each team's name and number of games won. The list should be in decreasing order by the number of wins.
A poker hand can be stored in a two-dimensional array. The statement Dim hand(3, 12) As Integer declares an array with 52 elements, where the first subscript ranges over the four suits and the second subscript ranges over the thirteen denominations. A poker hand is specified by placing ones in the elements corresponding to the cards in the hand. See Figure 7.12. Figure 7.12. Array for the poker hand A A 5 9 Q . 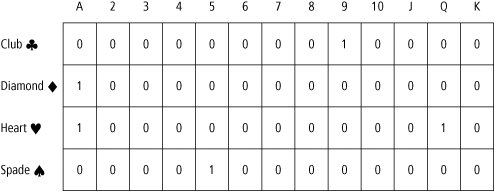 Write a program that requests the five cards as input from the user, creates the related array, and passes the array to procedures to determine the type of the hand: flush (all cards have the same suit), straight (cards have consecutive denominationsace can come either before 2 or after King), straight flush, four-of-a-kind, full house (3 cards of one denomination, 2 cards of another denomination), three-of-a-kind, two pairs, one pair, or none of the above. [Page 406]Airline Reservations. Write a reservation system for an airline flight. Assume the airplane has 10 rows with 4 seats in each row. Use a two-dimensional array of strings to maintain a seating chart. In addition, create an array to be used as a waiting list in case the plane is full. The waiting list should be "first come, first served," that is, people who are added early to the list get priority over those added later. Allow the user the following three options: Add a passenger to the flight or waiting list. Request the passenger's name. Display a chart of the seats in the airplane in tabular form. If seats are available, let the passenger choose a seat. Add the passenger to the seating chart. If no seats are available, place the passenger on the waiting list.
Remove a passenger from the flight. Request the passenger's name. Search the seating chart for the passenger's name and delete it. If the waiting list is empty, update the array so the seat is available. If the waiting list is not empty, remove the first person from the list, and give him or her the newly vacated seat.
Quit.
The Game of Life was invented by John H. Conway to model some genetic laws for birth, death, and survival. Consider a checkerboard consisting of an n-by-n array of squares. Each square can contain one individual (denoted by 1) or be empty (denoted by ). Figure 7.13(a) shows a 6-by-6 board with four of the squares occupied. The future of each individual depends on the number of his neighbors. After each period of time, called a generation, certain individuals will survive, others will die due to either loneliness or overcrowding, and new individuals will be born. Each nonborder square has eight neighboring squares. After each generation, the status of the squares changes as follows: An individual survives if there are two or three individuals in neighboring squares. An individual dies if he has more than three individuals or less than two in neighboring squares. A new individual is born into each empty square with exactly three individuals as neighbors. Figure 7.13. Two generations. 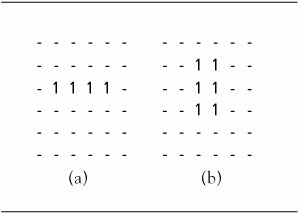
[Page 407]Figure 7.13(b) shows the status after one generation. Write a program to do the following: Declare a two-dimensional array of size n, where n is input by the user, to hold the status of each square in the current generation. To specify the initial configuration, have the user input each row as a string of length n, and break the row into 1's or dashes with the Substring method. Declare a two-dimensional array of size n to hold the status of each square in the next generation. Compute the status for each square and produce the display in Figure 7.13(b). Note: The generation changes all at once. Only current cells are used to determine which cells will contain individuals in the next generation. Assign the next-generation values to the current generation and repeat as often as desired. Display the individuals in each generation. Hint: The hardest part of the program is determining the number of neighbors a cell has. In general, you must check a 3-by-3 square around the cell in question. Exceptions must be made when the cell is on the edge of the array. Don't forget that a cell is not a neighbor of itself. (Test the program with the initial configuration shown in Figure 7.14. It is known as the figure-eight configuration and repeats after eight generations. ) Figure 7.14. The figure eight. 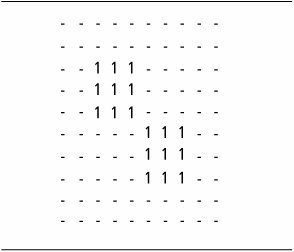
Every book is identified by a ten-character International Standard Book Number (ISBN) that is usually printed on the back cover of the book. The first nine characters are digits and the last character is either a digit or the letter X (which stands for ten). Three examples of ISBN numbers are 0-13-030657-6, 0-32-108599-X, and 0-471-58719-2. The hyphens separate the characters into four blocks. The first block usually consists of a single digit and identifies the language (0 for English, 2 for French, 3 for German, etc.). The second block identifies the publisher (for example, 13 for Prentice Hall, 32 for Addison-Wesley-Longman, and 471 for Wiley); The third block is the number the publisher has chosen for the book. The fourth block, which always consists of a single character called the check digit, is used to test for errors. Let's refer to the ten characters of the ISBN as d1,d2,d3,d4,d5,d6,d7,d8,d9,and d10.The check digit is chosen so that the sum [Page 408] 
is a multiple of 11. Note: A number is multiple of 11 if it is exactly divisible by 11.). If the last character of the ISBN is an X, then in the sum(*),d10 is replaced with 10. For example, with the ISBN 0-32-108599-X, the sum would be 
Since 165/11 is 15, the sum is a multiple of 11. This checking scheme will detect every single-digit and transposition-of-adjacent-digits error. That is, if while coping an IBSN number you miscopy a single character or transpose two adjacent characters, then the sum(*) will no longer be a multiple of 11. Write a program to accept an ISBN type number (including the hyphens) as input, calculate the sum(*), and tell if it is a valid ISBN number. (Hint: The number n is divisible by 11 if n Mod 11 is 0.) Before calculating the sum, the program should check that each of the first nine characters is a digit and that the last character is either a digit or an X. Write a program that begins with a valid ISBN number (such as 0-13-030657-6) and then confirms that the checking scheme described above detects every single-digit and transposition-of-adjacent-digits error by testing every possible error. [Hint: If d is a digit to be replaced, then the nine possibilities for the replacements are (d+1) Mod 10, (d+2) Mod 10, (d+3) Mod 10, Mod 10....(d+9) mod 10.].
User-Operated Directory Assistance. Have you ever tried to call a person at their business and been told to type in some of the letters of their name on your telephone's keypad in order to obtain their extension? Write a program to simulate this type of directory assistance. Suppose the names and telephone extensions of all the employees of a company are contained in the text file EMPLOYEES.TXT. Each set of three lines of the file has the three pieces of information: last name, first and middle name(s), and telephone extension. (We have filled the file with the names of the U.S. Presidents so that the names will be familiar.) The user should be asked to press buttons for the first three letters of the person's last name followed by the first letter of the first name. For instance, if the person's name is Gary Land, the user would type in 5264. The number 5264 is referred to as the "push-button encoding" of the name. (Note: People with different names can have the same push-button encoding for instance, Herb James and Gary Land.) The program should declare an array of structures to hold full names, extensions, and push-button encodings. The arrays should be filled while making a single pass through the file and then sorted by push-button encoding. After the user presses four keys on the keypad, the program should display the names and extensions of all the employees having the specified push-button encoding. See Figure 7.15. [Page 409] Figure 7.15. Sample run of Programming Project 10. |