Computers really aren't that smart. They do exactly what you tell them to, so if you make a mistake and tell them to do something completely wrong, they will try to do it anyway. An error is something that isn't supposed to happen in your program. There are many different types of errors that may occur in a computer program, such as syntax errors, logic errors, and runtime errors. Syntax errors are generally caught while you are typing the code and can be fixed easily because Visual Studio tells you it doesn't understand what you typed. Logic errors can usually be identified by incorrect operation of the program, and are fixed by examining and then correcting an erroneously designed section of code. However, errors that occur at runtime are especially disastrous, because they can happen unexpectedly and in front of the end user. Consider, for example, the following two simple lines of Visual Basic code: File.Copy ("myfile.doc","newfile.doc") MessageBox.Show ("The file has been copied.") During normal operation, the lines of code execute in order. The first line makes a copy of a file, and the second line displays a message telling the user the file has been copied. Both lines are syntactically and logically correct, and they will not cause an error when building your program. However, there is still a potential for runtime errors. For example, what happens if a user runs your program and the file myfile.doc is missing? Or the destination file already exists? These types of runtime errors must be handled by program code, otherwise the computer won't know what to do. Attempting to run the previous code on a computer where the source file does not exist will produce the message shown in Figure 5.7. Figure 5.7. Avoid cryptic messages and ungraceful exits by adding error-handling code to your programs. 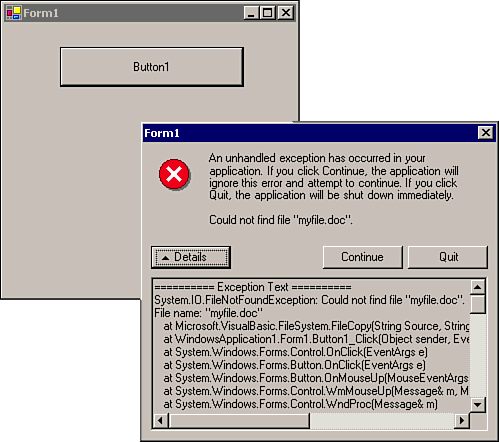 Note As you will see in Chapter 24, "Working with Files," there is a function used to determine whether or not a file exists. However, this is just one easy example; there are many other situations that can cause runtime errors. As you can see, the default runtime error message is meant for a programmer, not an end user. The user is left with a couple of choices: Click Quit and the program ends, or click Continue and the program attempts to keep running. As a programmer, you really don't want the user to do either, especially if the other parts of the program depend on the missing file! Structured Error Handling with Exceptions These types of errors are exceptions to the normal process and order of code execution. The purpose of the File.Copy function is to copy files, so when it could not find the source file, it threw an exception back to the function that called it. You can write code to catch the exception and then handle the error appropriately. It may sound a lot like baseball, but that is exactly how structured error handling works in Visual Studio .NET: by throwing and catching exceptions. When an error happens during the execution of a method, the method throws it back to the caller. If the program code does not catch the exception, it gets thrown all the way back to the users and they see the message shown in Figure 5.7. Now, let's add some error-handling code to our example: Try File.Copy("myfile.txt", "yourfile.txt") MessageBox.Show("The file has been copied") Catch FileMissingError As FileNotFoundException Dim strMessage As String strMessage = FileMissingError.Message strMessage = strMessage & "Please make sure this file exists and try again." strMessage = strMessage & "The program will now exit." MessageBox.Show(strMessage, "Error has occurred", _MessageBoxButtons.OK, MessageBoxIcon.Error) Application.Exit() End Try The additional code includes some new keywords, Try and Catch. When you are writing code to catch exceptions, you enclose both the code that may cause the exception and the code to handle it in a Try. . . End Try block. Within this block you can use one or more Catch statements to check for exceptions. When our sample code executes normally, then only the File.Copy and the first MessageBox statement will be executed. However, if an exception of type FileNotFoundException is thrown, the code beneath the Catch statement will be executed to handle the exception. Let's examine the exception-handling code a little closer. The code is very simple in that it just displays a message to the user and exits the program. However, notice that we declared an object variable, FileMissingError, which stores the returned exception. We then use the Message property of the exception object when creating our message to the user. This is where Visual Basic's object-oriented nature comes in very handy. Because an exception is an object, there may be specific properties and methods that you can use to better handle the exception in your code. In the previous sample, we know that the Message property contained the file path of the missing file, so we displayed it for the user. Note The help files contain specific exception types associated with the included classes. The File.Copy method can throw other types of exceptions besides a FileNotFound exception. You can code for as many specific exceptions as you want using multiple Catch statements, or just use the generic System.Exception class to handle any other exceptions generically: Try File.Copy("myfile.txt", "yourfile.txt") MessageBox.Show("The file has been copied") Catch FileMissingError As FileNotFoundException Dim strMessage As String strMessage = FileMissingError.Message strMessage = strMessage & "Please make sure this file exists and try again." strMessage = strMessage & "The program will now exit." MessageBox.Show(strMessage, "Error has occurred",_ MessageBoxButtons.OK, MessageBoxIcon.Error) Application.Exit() Catch BadErr As System.Exception MessageBox.Show(BadErr.ToString, "Error has occurred",_ MessageBoxButtons.OK, MessageBoxIcon.Error) Application.Exit() End Try In the examples we have looked at so far, our only action in the case of an exception has been to terminate the program. For an unknown exception this may be okay, but in the case of a missing file it may be a little drastic. By careful placement of the Try statement, we can provide the users with an error message that gives them the ability to fix the error: Dim Answer As DialogResult Dim bKeepTrying As Boolean = True While bKeepTrying Try File.Copy("c:\myfile.txt", "c:\yourfile.txt") MessageBox.Show("The file has been copied") bKeepTrying = False Catch FileMissingError As FileNotFoundException Dim strMessage As String strMessage = FileMissingError.Message strMessage = strMessage & "Make sure this file exists and try again." strMessage = strMessage & "Fix the problem and press RETRY or CANCEL." Answer = Message Box.Show(strMessage, "Error has occurred",_ MessageBoxButtons.RetryCancel, MessageBoxIcon.Stop) If Answer = DialogResult.Cancel Then bKeepTrying = False End If End Try End While If Answer = DialogResult.Cancel Then Application.Exit() End If The previous example uses a While loop to allow the user to retry the file copy operation as many times as he wants. In this section, we have described the basics of catching exceptions. However, there is a lot more to know in the area of error handling. An additional statement can be added to the Try block, the Finally statement, to add code that must always run whether there is an exception or not. The code typically placed here is used to close resources and clean up after the function executes. We will cover other exception-related topics, such as logging errors to the event log and throwing your own exceptions, in Chapter 9. To learn more about user-defined errors, p.223 Unstructured Error Handling with On Error Goto Structured error handling with the Try, Catch, Finally statements is new to Visual Basic .NET. If you have been programming in VB for a while you also may be familiar with the On Error Goto statement, also known as unstructured error handling. Our file copy example rewritten using the older syntax might look like the following: Dim Answer As DialogResult = DialogResult.Retry On Error Goto FileCopyError RetryHere: File.Copy("myfile.txt", "yourfile.txt") MessageBox.Show("The file has been copied") Answer = DialogResult.OK Exit Sub FileCopyError: Dim strMessage As String If err.Number = 53 Then strMessage = "Missing file: " & err.Description Else strMessage = "Error " & err.number & ": " & err.Description End If Answer = Messagebox.Show(strMessage, "Error has occurred",_ messagebox.IconStop + messagebox.retrycancel) If answer = winforms.dialogresult.Retry Then Resume RetryHere Else Application.Exit() End If End Sub To initiate unstructured error handling, you add an On Error Goto statement and provide a label for the program to branch to in case of an error. You can label lines of code by placing text followed by a colon (:) on a line. In the previous example, RetryHere and FileCopyError are labels. If an error occurs, the program jumps to the code labeled FileCopyError. When an error has occurred, you use one of several statements to control the flow of the program: Exit the subroutine after informing the user of the error, with the Exit Sub statement. Use a Resume label statement to jump to another portion of code Use a Resume Next statement to continue executing on the line following the error. Although this style of error handling is still supported in Visual Basic .NET, it is based on the infamous Goto statement, which may make the order of code execution harder to follow. Another disadvantage is the Error object passed back to the program is not as detailed as the specific exceptions-type objects you can use with Catch. In our example, we use the Number and Description properties of the Error object. |