6.2. Dynamically Generate Web Service Proxy Classes Note: Now you can simply drop a WSDL file into the App_Code folder and the web proxy class is dynamically generated. Cool! In Visual Studio .NET 2003, you must manually add a web reference to a web service using Add Web Reference or the WSDL.exe tool so that the appropriate web proxy class is generated. In Visual Studio 2005, this step is no longer required. Instead, you simply add a WSDL document that describes the service into the App_Code folder and the web proxy class needed to access the service is generated automatically. This allows you to handle situations where you are only provided a WSDL file by a web service provider and not a browsable URL endpoint containing the WSDL document. What Is a Web Service? I have always liked to describe a web service as a web application without a user interface. Technically, a web service is a business object residing on a server that you can programmatically access through the network. For example, a stock exchange could deploy a web service that allows its customers to check for the latest stock prices. Rather than using a web browser to check for current stock prices, customers can write their own custom applications and programmatically access price data through the web service and then integrate the results back into their application. To ensure interoperability between web services and its users (known as web service consumers), most web services use standards such as XML, HTTP, and WSDL: XML is used as the language for exchanging messages between a web service and its consumer. HTTP is used as the transport protocol to carry web services messages. WSDL is used to write the contract that defines what the web service has to offer. In Visual Studio (2003 and 2005), you need to obtain the WSDL file of a web service so that you know how to communicate with it. The advantage of using Visual Studio for web services is that it will automatically generate a proxy class for you (based on the WSDL document) so that you can simply invoke the web service just like a normal object. |
6.2.1. How do I do that? In this lab, you will see how easy it is to consume a web service, given its WSDL document. You will enable an application to consume a language translation web service by simply saving the WSDL document in the App_Code folder. IntelliSense will then show the web proxy class generated, based on the WSDL document. Launch Visual Studio 2005 and create a new web site project. Name the project C:\ASPNET20\chap06-DynamicWebProxy. Right-click the project name in Solution Explorer and select Add Folder App_Code Folder. To see the WSDL document in Solution Explorer, right-click the App_Code folder and select Refresh Folder (see Figure 6-6). Figure 6-6. Saving a WSDL file within the App_Code folder 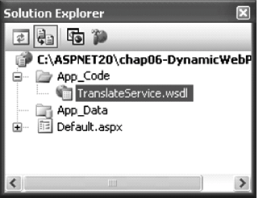
A web proxy class would then be dynamically generated. To test the web proxy class, populate the default Web Form for the project with the controls shown in Figure 6-7. Figure 6-7. Populating the default Web Form 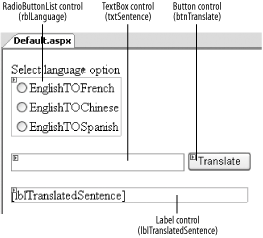
Double-click the Translate button to reveal the code-behind of the Web Form. Code the Click event of the Translate button as follows: Protected Sub btnTranslate_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnTranslate.Click Dim ws As New TranslateService Dim targetLanguage As String Select Case rblLanguage.SelectedIndex Case 0 : targetLanguage = _ ws.Translate(Language.EnglishTOFrench, _ txtSentence.Text) Case 1 : targetLanguage = _ ws.Translate(Language.EnglishTOChinese, _ txtSentence.Text) Case 2 : targetLanguage = _ ws.Translate(Language.EnglishTOSpanish, _ txtSentence.Text) End Select lblTranslatedSentence.Text = targetLanguage End Sub Note: IntelliSense will automatically display the web proxy class that was dynamically generated (TranslateService) as one of its choices.
Press F5 to test the application. Enter a sentence, select a language option, and then click the Translate button (see Figure 6-8). Tip: Your browser must be installed with the language pack for you to display some languages correctly. To do so, in IE select View Encoding More and select the desired language (Chinese, for example). You will then be prompted to install the language pack (you need the Windows installation disk). Figure 6-8. Testing the application 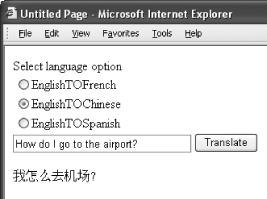 6.2.2. What about... ...the old way of generating a web service proxy class using Add Web Reference? In ASP.NET 2.0, you can still use Add Web Reference to add a reference to a web service. Simply right-click the project name in Solution Explorer and select Add Web Reference... (see Figure 6-9). Figure 6-9. Adding a web reference 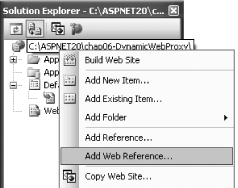 You will be prompted to enter the URL of the web service. If you enter http://www.webservicex.com/TranslateService.asmx?WSDL, the web reference will then be added to the project under the App_WebReferences folder (see Figure 6-10). Figure 6-10. The web reference added to the project 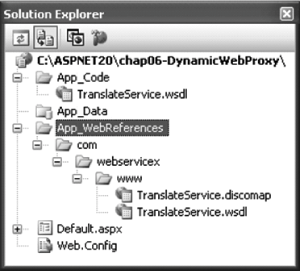 To invoke the web service, add the following statement to your code: Dim ws As New com.webservicex.www.TranslateService 6.2.3. Where can I learn more? If you want to learn how to develop web services using Visual Studio, check out the following tutorials: http://www.programmingtutorials.com/webservices.aspx. For a whitepaper on web services architecture and its specifications, read the following article from MSDN: http://msdn.microsoft.com/webservices/default.aspx?pull=/library/en-us/dnwebsrv/html/introwsa.asp. Note that this paper contains a lot of information, and you may find it overwhelming, especially if you are a newcomer to web services. However, I do recommend that you read it once you have mastered the fundamentals of the topic. |