5.8. Manage User Settings Note: Learn how to programmatically modify user's account information using the Membership class. So far, you have seen how to modify users' information using the built-in controls that ship with ASP.NET 2.0. A much more versatile approach would be to modify user data programmatically. The Membership class makes this possible and is, in fact, the source of many of the APIs driving all the new Login controls in ASP.NET 2.0. 5.8.1. How do I do that? In this lab, you will learn how to programmatically modify and access a user's settings. You will create a Web Form and populate it with text boxes and Button controls to let users perform tasks such as changing their passwords and identity validation questions, as well as updating their email addresses. All these tasks will be performed using the Membership and MembershipUser classes. Using the same project created in the last lab (C:\ASPNET20\chap-5-SecurityControls), add a new Web Form to the project (right-click the Members folder in Solution Explorer and then select Add New Item...; select Web Form). Name the Web Form UserInfo.aspx. Add a 3 6 table to the Web Form (Layout Insert Table) and populate it with the controls shown in Figure 5-36. Figure 5-36. The various controls on the UserInfo.aspx page 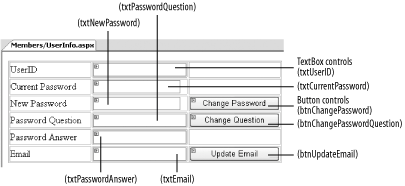
When the form is first loaded, retrieve the user's information using the GetUser( ) method from the Membership class. It returns a MembershipUser object. To code the Form_Load event, double-click on the Web Form to switch to the code-behind: Protected Sub Page_Load(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles Me.Load Dim user As MembershipUser '---get info about current user user = Membership.GetUser Response.Write("Your last login was on: " & _ user.LastLoginDate & "<br/>") Response.Write("Your account was created on: " & _ user.CreationDate & "<br/>") Response.Write("Your password was last changed on: " & _ user.LastPasswordChangedDate & "<br/>") If Not IsPostBack Then txtUserID.Text = user.UserName.ToString txtPasswordQuestion.Text = user.PasswordQuestion txtEmail.Text = user.Email End If End Sub Note that the MembershipUser class contains several pieces of information about the user, such as last login date, user creation date, and password last changed date. You can also retrieve the password question (but not the answer to the question). Tip: If the user is not authenticated, the Membership.GetUser method returns a null reference.
Add the following displayMessage( ) subroutine to format and display a message on the page: Private Sub displayMessage(ByVal str As String) Response.Write("<br/><b>" & str & "</b>") End Sub When the Change Password button is clicked, you can change the user's password via the ChangePassword( ) method in the MembershipUser class. This method returns a Boolean value indicating whether the change is successful: Protected Sub btnChangePassword_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnChangePassword.Click Dim user As MembershipUser = Membership.GetUser '==change password=== If txtCurrentPassword.Text <> "" And _ (txtNewPassword.Text <> txtCurrentPassword.Text) And _ txtNewPassword.Text <> "" Then '---means the user wants to change password If user.ChangePassword(txtCurrentPassword.Text, _ txtNewPassword.Text) Then displayMessage("Password Changed.") Else displayMessage("Password Changed Failed.") End If Else displayMessage("Required fields missing") End If End Sub You can change the password question and answer using the ChangePasswordQuestionAndAnswer( ) method. It, too, returns a value indicating whether the change is successful: Protected Sub btnChangePasswordQuestion_Click( _ ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnChangePasswordQuestion.Click Dim user As MembershipUser = Membership.GetUser If txtCurrentPassword.Text <> "" And _ txtPasswordQuestion.Text <> "" And _ txtPasswordAnswer.Text <> "" Then If user.ChangePasswordQuestionAndAnswer( _ txtCurrentPassword.Text, _ txtPasswordQuestion.Text, _ txtPasswordAnswer.Text) Then displayMessage("Password Question Changed.") Else displayMessage("Password Question Failed.") End If Else displayMessage("Required fields missing") End If End Sub When the user clicks on the Update Email button, the email is updated via the UpdateUser( ) method in the Membership class: Protected Sub btnUpdateEmail_Click(ByVal sender As Object, _ ByVal e As System.EventArgs) _ Handles btnUpdateEmail.Click Dim user As MembershipUser = Membership.GetUser user.Email = txtEmail.Text Membership.UpdateUser(user) displayMessage("Email Updated.") End Sub Press F5 to test the page. When the page is loaded, you will be prompted to log in (use the Administrator account created in the previous lab). Figure 5-37 shows the page in action. To change the password, type in the current and new passwords and then click on Change Password. To change the password question and answer, fill in the new question and answer and then click Change Question. To change the email address, fill in the new email address and click Update Email. Figure 5-37. Modifying a user's information 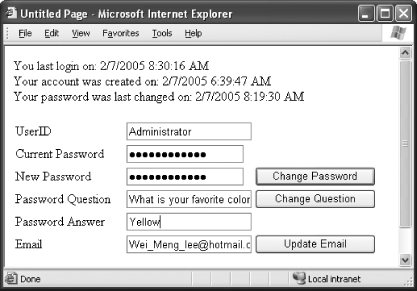
Take note that this UserInfo.aspx page should be appropriately protected (using SSL, for example), since passwords are transmitted in the clear. 5.8.2. What about ... ...retrieving all users' information? You can retrieve all the users' information in your application using the GetAllUsers( ) method in the MembershipUser class: Dim users As MembershipUserCollection users = Membership.GetAllUsers The users' information is returned as a MembershipUserCollection. You can then bind it to, say, a GridView control: GridView1.DataSource = users GridView1.DataBind( ) If you need to reset a user's password, you can use the ResetPassword( ) method in the MembershipUser class. You just need to supply a password answer (if your user account requires one): Dim newPassword As String = user.ResetPassword(txtPasswordAnswer.Text) The ResetPassword( ) method returns the new randomly generated password. 5.8.3. Where can I learn more? Check out the MSDN Help topics "Membership Members" and "MembershipUser Members" for all the properties and methods in the two classes. For more comprehensive coverage of the GridView control, refer to Chapter 4. To learn how to configure SSL on IIS, check out: - http://www.microsoft.com/resources/documentation/iis/6/all/proddocs/en-us/nntp_sec_securing_connections.mspx
|