An inner class is a class that is defined inside another class. Why would you want to do that? There are four reasons: An object of an inner class can access the implementation of the object that created it including data that would otherwise be private. Inner classes can be hidden from other classes in the same package. Anonymous inner classes are handy when you want to define callbacks on the fly. Inner classes are very convenient when you are writing event-driven programs. You will soon see examples that demonstrate the first three benefits. (For more information on the event model, please turn to Chapter 8.) C++ has nested classes. A nested class is contained inside the scope of the enclosing class. Here is a typical example: a linked list class defines a class to hold the links, and a class to define an iterator position. class LinkedList { public: class Iterator // a nested class { public: void insert(int x); int erase(); . . . }; . . . private: class Link // a nested class { public: Link* next; int data; }; . . . }; The nesting is a relationship between classes, not objects. A LinkedList object does not have subobjects of type Iterator or Link. There are two benefits: name control and access control. Because the name Iterator is nested inside the LinkedList class, it is externally known as LinkedList::Iterator and cannot conflict with another class called Iterator. In Java, this benefit is not as important since Java packages give the same kind of name control. Note that the Link class is in the private part of the LinkedList class. It is completely hidden from all other code. For that reason, it is safe to make its data fields public. They can be accessed by the methods of the LinkedList class (which has a legitimate need to access them), and they are not visible elsewhere. In Java, this kind of control was not possible until inner classes were introduced. However, the Java inner classes have an additional feature that makes them richer and more useful than nested classes in C++. An object that comes from an inner class has an implicit reference to the outer class object that instantiated it. Through this pointer, it gains access to the total state of the outer object. You will see the details of the Java mechanism later in this chapter. Only static inner classes do not have this added pointer. They are the Java analog to nested classes in C++. | Using an Inner Class to Access Object State The syntax for inner classes is somewhat complex. For that reason, we will use a simple but somewhat artificial example to demonstrate the use of inner classes. We will write a program in which a timer controls a bank account. The timer's action listener object adds interest to the account once per second. However, we don't want to use public methods (such as deposit or withdraw) to manipulate the bank balance because anyone could call those public methods to modify the balance for other purposes. Instead, we will use an inner class whose methods can manipulate the bank balance directly. Here is the outline of the BankAccount class: class BankAccount { public BankAccount(double initialBalance) { . . . } public void start(double rate) { . . . } private double balance; private class InterestAdder implements ActionListener // an inner class { . . . } } Note the InterestAdder class that is located inside the BankAccount class. This does not mean that every BankAccount has an InterestAdder instance field. Of course, we will construct objects of the inner class, but those objects aren't instance fields of the outer class. Instead, they will be local to the methods of the outer class. The InterestAdder class is a private inner class inside BankAccount. This is a safety mechanism since only BankAccount methods can generate InterestAdder objects, we don't have to worry about breaking encapsulation. Only inner classes can be private. Regular classes always have either package or public visibility. The InterestAdder class has a constructor which sets the interest rate that should be applied at each step. Since this inner class implements the ActionListener interface, it also has an actionPerformed method. That method actually increments the account balance. Here is the inner class in more detail: class BankAccount { public BankAccount(double initialBalance) { balance = initialBalance; } . . . private double balance; private class InterestAdder implements ActionListener { public InterestAdder(double aRate) { rate = aRate; } public void actionPerformed(ActionEvent event) { . . . } private double rate; } } The start method of the BankAccount class constructs an InterestAdder object for the given interest rate, makes it the action listener for a timer, and starts the timer. public void start(double rate) { ActionListener adder = new InterestAdder(rate); Timer t = new Timer(1000, adder); t.start(); } As a result, the actionPerformed method of the InterestAdder class will be called once per second. Now let's look inside this method more closely: public void actionPerformed(ActionEvent event) { double interest = balance * rate / 100; balance += interest; NumberFormat formatter = NumberFormat.getCurrencyInstance(); System.out.println("balance=" + formatter.format(balance)); } The name rate refers to the instance field of the InterestAdder class, which is not surprising. However, there is no balance field in the InterestAdder class. Instead, balance refers to the field of the BankAccount object that created this InstanceAdder. This is quite innovative. Traditionally, a method could refer to the data fields of the object invoking the method. An inner class method gets to access both its own data fields and those of the outer object creating it. For this to work, of course, an object of an inner class always gets an implicit reference to the object that created it. (See Figure 6-3.) Figure 6-3. An inner class object has a reference to an outer class object 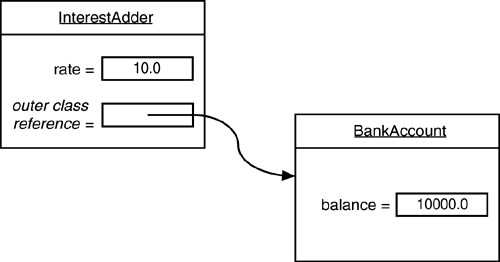 This reference is invisible in the definition of the inner class. However, to illuminate the concept, let us call the reference to the outer object outer. Then, the actionPerformed method is equivalent to the following: public void actionPerformed(ActionEvent event) { double interest = outer.balance * this.rate / 100; // "outer" isn't the actual name outer.balance += interest; NumberFormat formatter = NumberFormat.getCurrencyInstance(); System.out.println("balance=" + formatter.format(outer.balance)); } The outer class reference is set in the constructor. That is, the compiler adds a parameter to the constructor, generating code like this: public InterestAdder(BankAccount account, double aRate) { outer = account; // automatically generated code rate = aRate; } Again, please note, outer is not a Java keyword. We just use it to illustrate the mechanism involved in an inner class. When an InterestAdder object is constructed in the start method, the compiler passes the this reference to the current bank account into the constructor: ActionListener adder = new InterestAdder(this, rate); // automatically generated code Example 6-4 shows the complete program that tests the inner class. Have another look at the access control. The Timer object requires an object of some class that implements the ActionListener interface. Had that class been a regular class, then it would have needed to access the bank balance through a public method. As a consequence, the BankAccount class would have to provide those methods to all classes, which it might have been reluctant to do. Using an inner class is an improvement. The InterestAdder inner class is able to access the bank balance, but no other class has the same privilege. Example 6-4 InnerClassTest.java 1. import java.awt.event.*; 2. import java.text.*; 3. import javax.swing.*; 4. 5. public class InnerClassTest 6. { 7. public static void main(String[] args) 8. { 9. // construct a bank account with initial balance of $10,000 10. BankAccount account = new BankAccount(10000); 11. // start accumulating interest at 10% 12. account.start(10); 13. 14. // keep program running until user selects "Ok" 15. JOptionPane.showMessageDialog(null, "Quit program?"); 16. System.exit(0); 17. } 18. } 19. 20. class BankAccount 21. { 22. /** 23. Constructs a bank account with an initial balance 24. @param initialBalance the initial balance 25. */ 26. public BankAccount(double initialBalance) 27. { 28. balance = initialBalance; 29. } 30. 31. /** 32. Starts a simulation in which interest is added once per 33. second 34. @param rate the interest rate in percent 35. */ 36. public void start(double rate) 37. { 38. ActionListener adder = new InterestAdder(rate); 39. Timer t = new Timer(1000, adder); 40. t.start(); 41. } 42. 43. private double balance; 44. 45. /** 46. This class adds the interest to the bank account. 47. The actionPerformed method is called by the timer. 48. */ 49. private class InterestAdder implements ActionListener 50. { 51. public InterestAdder(double aRate) 52. { 53. rate = aRate; 54. } 55. 56. public void actionPerformed(ActionEvent event) 57. { 58. // update interest 59. double interest = balance * rate / 100; 60. balance += interest; 61. 62. // print out current balance 63. NumberFormat formatter 64. = NumberFormat.getCurrencyInstance(); 65. System.out.println("balance=" 66. + formatter.format(balance)); 67. } 68. 69. private double rate; 70. } 71. } Special Syntax Rules for Inner Classes In the preceding section, we explained the outer class reference of an inner class by calling it outer. Actually, the proper syntax for the outer reference is a bit more complex. The expression OuterClass.this denotes the outer class reference. For example, you can write the actionPerformed method of the InterestAdder inner class as public void actionPerformed(ActionEvent event) { double interest = BankAccount.this.balance * this.rate / 100; BankAccount.this.balance += interest; . . . } Conversely, you can write the inner object constructor more explicitly, using the syntax: outerObject.new InnerClass(construction parameters) For example, ActionListener adder = this.new InterestAdder(rate); Here, the outer class reference of the newly constructed InterestAdder object is set to the this reference of the method that creates the inner class object. This is the most common case. As always, the this. qualifier is redundant. However, it is also possible to set the outer class reference to another object by explicitly naming it. For example, if InterestAdder was a public inner class, you could construct an InterestAdder for any bank account: BankAccount mySavings = new BankAccount(10000); BankAccount.InterestAdder adder = mySavings.new InterestAdder(10); Note that you refer to an inner class as OuterClass.InnerClass when it occurs outside the scope of the outer class. For example, if InterestAdder had been a public class, you could have referred to it as BankAccount.InterestAdder elsewhere in your program. Are Inner Classes Useful? Are They Actually Necessary? Are They Secure? Inner classes are a major addition to the language. Java started out with the goal of being simpler than C++. But inner classes are anything but simple. The syntax is complex. (It will get more complex as we study anonymous inner classes later in this chapter.) It is not obvious how inner classes interact with other features of the language, such as access control and security. Has Java started down the road to ruin that has afflicted so many other languages, by adding a feature that was elegant and interesting rather than needed? While we won't try to answer this question completely, it is worth noting that inner classes are a phenomenon of the compiler, not the virtual machine. Inner classes are translated into regular class files with $ (dollar signs) delimiting outer and inner class names, and the virtual machine does not have any special knowledge about them. For example, the InterestAdder class inside the BankAccount class is translated to a class file BankAccount$InterestAdder.class. To see this at work, try out the following experiment: run the ReflectionTest program of Chapter 5, and give it the class BankAccount$InterestAdder to reflect upon. You will get the following printout: class BankAccount$InterestAdder { public BankAccount$InterestAdder(BankAccount, double); public void actionPerformed(java.awt.event.ActionEvent); private double rate; private final BankAccount this$0; } If you use Unix, remember to escape the $ character if you supply the class name on the command line. That is, run the ReflectionTest program as java ReflectionTest 'BankAccount$InterestAdder' | You can plainly see that the compiler has generated an additional instance field, this$0, for the reference to the outer class. (The name this$0 is synthesized by the compiler you cannot refer to it in your code.) You can also see the added parameter for the constructor. If the compiler can do this transformation, couldn't you simply program the same mechanism by hand? Let's try it. We would make InterestAdder a regular class, outside the BankAccount class. When constructing an InterestAdder object, we pass it the this reference of the object that is creating it. class BankAccount { . . . public void start(double rate) { ActionListener adder = new InterestAdder(this, rate); Timer t = new Timer(1000, adder); t.start(); } } class InterestAdder implements ActionListener { public InterestAdder(BankAccount account, double aRate) { outer = account; rate = aRate; } . . . private BankAccount outer; private double rate; } Now let us look at the actionPerformed method. It needs to access outer.balance. public void actionPerformed(ActionEvent event) { double interest = outer.balance * rate / 100; // ERROR outer.balance += interest; // ERROR . . . } Here we run into a problem. The inner class can access the private data of the outer class, but our external InterestAdder class cannot. Thus, inner classes are genuinely more powerful than regular classes, since they have more access privileges. You may well wonder how inner classes manage to acquire those added access privileges, since inner classes are translated to regular classes with funny names the virtual machine knows nothing at all about them. To solve this mystery, let's again use the ReflectionTest program to spy on the BankAccount class: class BankAccount { public BankAccount(double); static double access$000(BankAccount); public void start(double); static double access$018(BankAccount, double); private double balance; } Notice the static access$000 and access$018 methods that the compiler added to the outer class. The inner class methods call those methods. For example, the statement balance += interest in the actionPerformed method of the InterestAdder class effectively makes the following call: access$018(outer, access$000(outer) + interest); Is this a security risk? You bet it is. It is an easy matter for someone else to invoke the access$000 method to read the private balance field or, even worse, to call the access$018 method to set it. The Java language standard reserves $ characters in variable and method names for system usage. However, for those hackers who are familiar with the structure of class files, it is an easy (if tedious) matter to produce a class file with virtual machine instructions to call that method. Of course, such a class file would need to be generated manually (for example, with a hex editor). Because the secret access methods have package visibility, the attack code would need to be placed inside the same package as the class under attack. To summarize, if an inner class accesses a private data field, then it is possible to access that data field through other classes that are added to the package of the outer class, but to do so requires skill and determination. A programmer cannot accidentally obtain access but must intentionally build or modify a class file for that purpose. Local Inner Classes If you look carefully at the code of the BankAccount example, you will find that you need the name of the type InterestAdder only once: when you create an object of that type in the start method. When you have a situation like this, you can define the class locally in a single method. public void start(double rate) { class InterestAdder implements ActionListener { public InterestAdder(double aRate) { rate = aRate; } public void actionPerformed(ActionEvent event) { double interest = balance * rate / 100; balance += interest; NumberFormat formatter = NumberFormat.getCurrencyInstance(); System.out.println("balance=" + formatter.format(balance)); } private double rate; } ActionListener adder = new InterestAdder(rate); Timer t = new Timer(1000, adder); t.start(); } Local classes are never declared with an access specifier (that is, public or private). Their scope is always restricted to the block in which they are declared. Local classes have a great advantage they are completely hidden from the outside world not even other code in the BankAccount class can access them. No method except start has any knowledge of the InterestAdder class. Local classes have another advantage over other inner classes. Not only can they access the fields of their outer classes, they can even access local variables! However, those local variables must be declared final. Here is a typical example. public void start(final double rate) { class InterestAdder implements ActionListener { public void actionPerformed(ActionEvent event) { double interest = balance * rate / 100; balance += interest; NumberFormat formatter = NumberFormat.getCurrencyInstance(); System.out.println("balance=" + formatter.format(balance)); } } ActionListener adder = new InterestAdder(); Timer t = new Timer(1000, adder); t.start(); } Note that the InterestAdder class no longer needs to store a rate instance variable. It simply refers to the parameter variable of the method that contains the class definition. Maybe this should not be so surprising. The line double interest = balance * rate / 100; is, after all, ultimately inside the start method, so why shouldn't it have access to the value of the rate variable? To see why there is a subtle issue here, let's consider the flow of control more closely. The start method is called. The object variable adder is initialized via a call to the constructor of the inner class InterestAdder. The adder reference is passed to the Timer constructor, the timer is started, and the start method exits. At this point, the rate parameter variable of the start method no longer exists. A second later, the actionPerformed method calls double interest = balance * rate / 100;. For the code in the actionPerformed method to work, the InterestAdder class must have made a copy of the rate field before it went away as a local variable of the start method. That is indeed exactly what happens. In our example, the compiler synthesizes the name BankAccount$1$InterestAdder for the local inner class. If you use the ReflectionTest program again to spy on the BankAccount$1$InterestAdder class, you get the following output: class BankAccount$1$InterestAdder { BankAccount$1$InterestAdder(BankAccount, double); public void actionPerformed(java.awt.event.ActionEvent); private final double val$rate; private final BankAccount this$0; } Note the extra double parameter to the constructor and the val$rate instance variable. When an object is created, the value rate is passed into the constructor and stored in the val$rate field. This sounds like an extraordinary amount of trouble for the implementors of the compiler. The compiler must detect access of local variables, make matching data fields for each one of them, and copy the local variables into the constructor so that the data fields can be initialized as copies of them. From the programmer's point of view, however, local variable access is quite pleasant. It makes your inner classes simpler by reducing the instance fields that you need to program explicitly. As we already mentioned, the methods of a local class can refer only to local variables that are declared final. For that reason, the rate parameter was declared final in our example. A local variable that is declared final cannot be modified. Thus, it is guaranteed that the local variable and the copy that is made inside the local class always have the same value. You have seen final variables used for constants, such as public static final double SPEED_LIMIT = 55; The final keyword can be applied to local variables, instance variables, and static variables. In all cases it means the same thing: You can assign to this variable once after it has been created. Afterwards, you cannot change the value it is final. However, you don't have to initialize a final variable when you define it. For example, the final parameter variable rate is initialized once after its creation, when the start method is called. (If the method is called multiple times, each call has its own newly created rate parameter.) The val$rate instance variable that you can see in the BankAccount$1$InterestAdder inner class is set once, in the inner class constructor. A final variable that isn't initialized when it is defined is often called a blank final variable. | Anonymous inner classes When using local inner classes, you can often go a step further. If you want to make only a single object of this class, you don't even need to give the class a name. Such a class is called anonymous inner class. public void start(final double rate) { ActionListener adder = new ActionListener() { public void actionPerformed(ActionEvent event) { double interest = balance * rate / 100; balance += interest; NumberFormat formatter = NumberFormat.getCurrencyInstance(); System.out.println("balance=" + formatter.format(balance)); } }; Timer t = new Timer(1000, adder); t.start(); } This is a very cryptic syntax indeed. What it means is: Create a new object of a class that implements the ActionListener interface, where the required method actionPerformed is the one defined inside the braces { }. Any parameters used to construct the object are given inside the parentheses ( ) following the supertype name. In general, the syntax is new SuperType(construction parameters) { inner class methods and data } Here, SuperType can be an interface, such as ActionListener; then, the inner class implements that interface. Or, SuperType can be a class; then, the inner class extends that class. An anonymous inner class cannot have constructors because the name of a constructor must be the same as the name of a class, and the class has no name. Instead, the construction parameters are given to the superclass constructor. In particular, whenever an inner class implements an interface, it cannot have any construction parameters. Nevertheless, you must supply a set of parentheses as in: new InterfaceType() { methods and data } You have to look very carefully to see the difference between the construction of a new object of a class and the construction of an object of an anonymous inner class extending that class. If the closing parenthesis of the construction parameter list is followed by an opening brace, then an anonymous inner class is being defined. Person queen = new Person("Mary"); // a Person object Person count = new Person("Dracula") { ... }; // an object of an inner class extending Person Are anonymous inner classes a great idea or are they a great way of writing obfuscated code? Probably a bit of both. When the code for an inner class is short, just a few lines of simple code, then they can save typing time, but it is exactly timesaving features like this that lead you down the slippery slope to "Obfuscated Java Code Contests." It is a shame that the designers of Java did not try to improve the syntax of anonymous inner classes, since, generally, Java syntax is a great improvement over C++. The designers of the inner class feature could have helped the human reader with a syntax such as: Person count = new class extends Person("Dracula") { ... }; // not the actual Java syntax But they didn't. Because many programmers find code with too many anonymous inner classes hard to read, we recommend restraint when using them. Example 6-5 contains the complete source code for the bank account program with an anonymous inner class. If you compare this program with Example 6-4, you will find that in this case the solution with the anonymous inner class is quite a bit shorter, and, hopefully, with a bit of practice, as easy to comprehend. Example 6-5 AnonymousInnerClassTest.java 1. import java.awt.event.*; 2. import java.text.*; 3. import javax.swing.*; 4. 5. public class AnonymousInnerClassTest 6. { 7. public static void main(String[] args) 8. { 9. // construct a bank account with initial balance of $10,000 10. BankAccount account = new BankAccount(10000); 11. // start accumulating interest at 10% 12. account.start(10); 13. 14. // keep program running until user selects "Ok" 15. JOptionPane.showMessageDialog(null, "Quit program?"); 16. System.exit(0); 17. } 18. } 19. 20. class BankAccount 21. { 22. /** 23. Constructs a bank account with an initial balance 24. @param initialBalance the initial balance 25. */ 26. public BankAccount(double initialBalance) 27. { 28. balance = initialBalance; 29. } 30 31. /** 32. Starts a simulation in which interest is added once per 33. second 34. @param rate the interest rate in percent 35. */ 36. public void start(final double rate) 37. { 38. ActionListener adder = new 39. ActionListener() 40. { 41. public void actionPerformed(ActionEvent event) 42. { 43. // update interest 44. double interest = balance * rate / 100; 45. balance += interest; 46. 47. // print out current balance 48. NumberFormat formatter 49. = NumberFormat.getCurrencyInstance(); 50. System.out.println("balance=" 51. + formatter.format(balance)); 52. } 53. }; 54. 55. Timer t = new Timer(1000, adder); 56. t.start(); 57. } 58. 59. private double balance; 60. } Static Inner Classes Occasionally, you want to use an inner class simply to hide one class inside another, but you don't need the inner class to have a reference to the outer class object. You can suppress the generation of that reference by declaring the inner class static. Here is a typical example of where you would want to do this. Consider the task of computing the minimum and maximum value in an array. Of course, you write one function to compute the minimum and another function to compute the maximum. When you call both functions, then the array is traversed twice. It would be more efficient to traverse the array only once, computing both the minimum and the maximum simultaneously. double min = d[0]; double max = d[0]; for (int i = 1; i < d.length; i++) { if (min > d[i]) min = d[i]; if (max < d[i]) max = d[i]; } However, the function must return two numbers. We can achieve that by defining a class Pair that holds two values: class Pair { public Pair(double f, double s) { first = f; second = s; } public double getFirst() { return first; } public double getSecond() { return second; } private double first; private double second; } The minmax function can then return an object of type Pair. class ArrayAlg { public static Pair minmax(double[] d) { . . . return new Pair(min, max); } } The caller of the function then uses the getFirst and getSecond methods to retrieve the answers: Pair p = ArrayAlg.minmax(d); System.out.println("min = " + p.getFirst()); System.out.println("max = " + p.getSecond()); Of course, the name Pair is an exceedingly common name, and in a large project, it is quite possible that some other programmer had the same bright idea, except that the other programmer made a Pair class that contains a pair of strings. We can solve this potential name clash by making Pair a public inner class inside ArrayAlg. Then the class will be known to the public as ArrayAlg.Pair: ArrayAlg.Pair p = ArrayAlg.minmax(d); However, unlike the inner classes that we used in previous examples, we do not want to have a reference to any other object inside a Pair object. That reference can be suppressed by declaring the inner class static: class ArrayAlg { public static class Pair { . . . } . . . } Of course, only inner classes can be declared static. A static inner class is exactly like any other inner class, except that an object of a static inner class does not have a reference to the outer class object that generated it. In our example, we must use a static inner class because the inner class object is constructed inside a static method: public static Pair minmax(double[] d) { . . . return new Pair(min, max); } Had the Pair class not been declared as static, the compiler would have complained that there was no implicit object of type ArrayAlg available to initialize the inner class object. You use a static inner class whenever the inner class does not need to access an outer class object. Some programmers use the term nested class to describe static inner classes. | Example 6-6 contains the complete source code of the ArrayAlg class and the nested Pair class. Example 6-6 StaticInnerClassTest.java 1. public class StaticInnerClassTest 2. { 3. public static void main(String[] args) 4. { 5. double[] d = new double[20]; 6. for (int i = 0; i < d.length; i++) 7. d[i] = 100 * Math.random(); 8. ArrayAlg.Pair p = ArrayAlg.minmax(d); 9. System.out.println("min = " + p.getFirst()); 10. System.out.println("max = " + p.getSecond()); 11. } 12. } 13. 14. class ArrayAlg 15. { 16. /** 17. A pair of floating point numbers 18. */ 19. public static class Pair 20. { 21. /** 22. Constructs a pair from two floating point numbers 23. @param f the first number 24. @param s the second number 25. */ 26. public Pair(double f, double s) 27. { 28. first = f; 29. second = s; 30. } 31. 32. /** 33. Returns the first number of the pair 34. @return the first number 35. */ 36. public double getFirst() 37. { 38. return first; 39. } 40. 41. /** 42. Returns the second number of the pair 43. @return the second number 44. */ 45. public double getSecond() 46. { 47. return second; 48. } 49. 50. private double first; 51. private double second; 52. } 53. 54. /** 55. Computes both the minimum and the maximum of an array 56. @param a an array of floating point numbers 57. @return a pair whose first element is the minimum and whose 58. second element is the maximum 59. */ 60. public static Pair minmax(double[] d) 61. { 62. if (d.length == 0) return new Pair(0, 0); 63. double min = d[0]; 64. double max = d[0]; 65. for (int i = 1; i < d.length; i++) 66. { 67. if (min > d[i]) min = d[i]; 68. if (max < d[i]) max = d[i]; 69. } 70. return new Pair(min, max); 71. } 72. } |