The Basics of Web Application Programming Now that you have a good understanding of JSTL variables and application data, you can start to create some simple Web applications. This section provides examples that illustrate common Web programming techniques. Processing the Query String The query string has always been a popular conduit for information between Web pages. The query string allows variable information to be embedded directly into a URL. For example, the URL http://www.jeffheaton.com/search.jsp?n=5 would pass the value 5 through the n variable to the script search.jsp. Anything containing a ? that follows the URL is considered part of the query string. By separating the values with ampersands ( & ), you can send more than one value through the query string. To pass the value 10 for x and 20 for y, you would use the URL http://www.jeffheaton.com/search.jsp0&y=20. These values can easily be accessed by the JSTL code. You will recall from the section "Accessing Application Data" earlier in this chapter that JSTL provides a variable called paramValues that allows you to access parameter arrays. There is also a variable called param that allows access to individual form and query string items. Note that neither param nor parmValues makes any distinction between form items and query string items. You would use the following tag to display a form or query string item named x : <c:out value="${param.x}"/> Listing 2.7 shows a program that uses this technique. This simple example asks users what their favorite color is. Listing 2.7 Favorite Color (color.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Query String Example</title> </head> <body>Your favorite color is: <b> <c:out value="${param.color}" /> </b> <br /> <br /> Choose your favorite color: <br /> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=red"> red</a> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=blue"> blue</a> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=green"> green</a> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=yellow"> yellow</a> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=other"> other</a> <br /> </body> </html> The program in Listing 2.7 displays the current favorite color, which is initially blank. Then, the user is allowed to select from several hyperlinks to specify a new favorite color. You can see the output from this program in Figure 2.7. 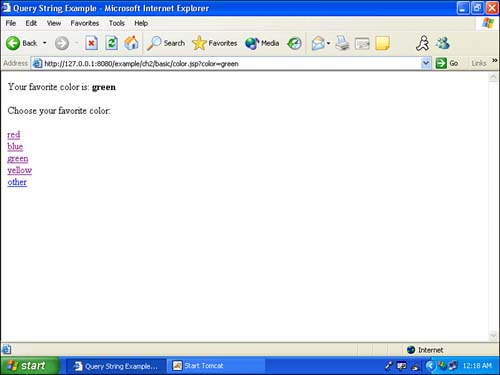 This program works by passing the current favorite color on the query string. Notice the hyperlinks that allow users to select their favorite color: <a href="<c:out value="${pageContext.request.requestURI}"/>?color=yellow"> yellow</a> These hyperlinks embed a color, such as yellow, as part of the URL line. The requestURI is also used to ensure that the program links back to the same page. We could have simply used the name color.jsp rather than the requestURI; however, using the requestURI ensures that the code will continue to function, even if the script filename changes. When the user actually clicks the hyperlink, the resulting URL will be something in the form of http://127.0.0.1:8080/example/ch2/basic/color.jsp?color=yellow. When the user clicks on one of the color selection hyperlinks, the page will be reloaded. However, this time it will have a value for the query string. The program will then display the favorite color using this code: <body>Your favorite color is: <b> <c:out value="${param.color}" /> </b> Working with Forms Forms are a common part of any Web application. The param variable allows you to access forms and retrieve individual entries made by the user. In this section, we look at a simple example that asks the user for a user ID and password. The form posts the results to a second JSP page that greets the user. This is a simple example that shows how to retrieve a single value from a form. You will perform this operation many times while creating Web applications. Listing 2.8 shows the form that inputs the user ID and password. Listing 2.8 Our HTML Login Form (form1.html)<html> <head> </head> <body> <form method="POST" action="form2.jsp"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="42%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font size="4" color="#FFFFFF">Please Login</font> </b> </p> </td> </tr> <tr> <td width="19%">User Name</td> <td width="81%"> <input type="text" name="uid" size="20" /> </td> </tr> <tr> <td width="19%">Password</td> <td width="81%"> <input type="password" name="pwd" size="20" /> </td> </tr> <tr> <td width="100%" colspan="2"> <p align="center"> <input type="submit" value="Submit" name="action" /> <input type="reset" value="Reset" name="B2" /> </p> </td> </tr> </table> </form> <p align="left"> <i>Note: you may use any ID/Password, security is not checked.</i> </p> </body> </html> This is a typical form that posts to another page. The user ID and password information is collected from the user and then posted to form2.jsp, shown in Listing 2.9. Listing 2.9 Processing the Login Form (form2.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <h3>Welcome back <c:out value="${param.uid}" /> !</h3> </html> This is a simple JSP page that displays the user's ID, stored in the uid input tag, to the browser. You have seen how forms are processed using JSTL. Forms are an important way of retrieving information from the user, and you will use them often in Web programming. Understanding State State is an important concept for nearly any transactional Web site. If the user is to log in, some form of state must be used so that the system remembers which browser represents the user who just logged in. Without state, the Web server will forget a user as soon as he or she logs in. State is what the Web server remembers from one request to the next. In this example, the state would be which user is actually logged on. To help you better understand state, we have combined the previously mentioned examples. This program asks the user to log into the Web site. After logging in, the user is prompted to enter his or her favorite color. Unlike in the previous examples, this program remembers the name of the user who is logged in, and greets him or her for each new color picked. The login screen is also somewhat different, as you can see in Listing 2.10. Listing 2.10 Our Stateful Login Form (session1.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <c:if test="${pageContext.request.method=='POST'}"> <c:set var="uid" value="${param.uid}" scope="session" /> <jsp:forward page="session2.jsp" /> </c:if> <html> <head> </head> <body> <form method="POST"> <table border="1" cellpadding="0" cellspacing="0" style="border-collapse: collapse" bordercolor="#111111" width="42%" > <tr> <td width="100%" colspan="2" bgcolor="#0000FF"> <p align="center"> <b> <font size="4" color="#FFFFFF">Please Login</font> </b> </p> </td> </tr> <tr> <td width="19%">User Name</td> <td width="81%"> <input type="text" name="uid" size="20" /> </td> </tr> <tr> <td width="19%">Password</td> <td width="81%"> <input type="password" name="pwd" size="20" /> </td> </tr> <tr> <td width="100%" colspan="2"> <p align="center"> <input type="submit" value="Submit" name="action" /> <input type="reset" value="Reset" name="B2" /> </p> </td> </tr> </table> </form> <p align="left"> <i>Note: you may use any ID/Password, security is not checked.</i> </p> </body> </html> The first obvious difference is the form tag. As you can see, the <form method="POST"> tag does not specify an action. If you do not specify an action for a form tag, the form will post to the current page. This may sound like an endless loop, and without JSTL it would be. However, at the top of session1.jsp are a few lines of code that intercept the post, as shown here: <c:if test="${pageContext.request.method=='POST'}"> <c:set var="uid" value="${param.uid}" scope="session" /> <jsp:forward page="session2.jsp" /> </c:if> Here, an if statement is used to check the request method. (We cover if statements in greater detail in Chapter 3, "Understanding Basic Tag Logic.") If the request method is POST, this page is currently being posted to. If this is the case, the user ID is read and stored as a session variable named uid. The page then is forwarded to session2.jsp, causing the session2.jsp page to be displayed. The user will never even know that we briefly revisited session1.jsp. The session2.jsp file is shown in Listing 2.11. Listing 2.11 Our Favorite Color Example Using State (session2.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Session Example</title> </head> <body> <h3>Welcome <c:out value="${uid}" /> </h3> Your favorite color is: <b> <c:if test="${param.color!=null}"> <c:out value="${param.color}" /> </c:if> </b> <br /> <br /> Choose your favorite color: <br /> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=red"> red</a> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=blue"> blue</a> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=green"> green</a> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=yellow"> yellow</a> <br /> <a href="<c:out value="${pageContext.request.requestURI}"/>?color=other"> other</a> <br /> </body> </html> The file session2.jsp is identical to color.jsp, except that it greets the current user. It knows who the current user is because of the session-scoped variable. The following lines of code display this variable: <h3>Welcome <c:out value="${uid}" /> </h3> For this code, it is not necessary to include the scope="session" portion of the command. If you do not specify a session, JSTL will examine the request, page, session, and finally application scopes until a match is found. State is an important part of Web development. In this section, you learned how to manage the state, which is what the Web server remembers from request to request. You will use state often as you design and implement interactive Web applications. |