Web applications must interact with the user, and to interact with the user, you must be able to access JSTL application data. Application data falls into two categories in JSTL. JSP makes two implicit variables available for your use. The first category is page context data. Page context data lets you know general information about the request, such as the request method or how long the session has been running. The second category, a collection named params, is made available to read the content posted from forms. Responding to parameter data is often a primary means of interaction between the Web application and the user. Accessing Page Context Data JSP includes a variable named pageContext that allows you to access certain contextual information about the page. To see what sort of information is available through the pageContext variable, let's look at the example shown in Listing 2.4. This simple JSP page will list the important items stored in the page context. Listing 2.4 Accessing Application Data<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Page Data Example</title> </head> <body> <h3> </h3> <table border="1" width="539"> <tr> <td colspan="2" width="529" bgcolor="#0000FF"> <b> <font color="#FFFFFF" size="4">HTTP Request(pageContext.request.)</font> </b> </td> </tr> <tr> <td width="210">Access Method</td> <td width="313">  <c:out value="${pageContext.request.method}" /> </td> </tr> <tr> <td width="210">Authentication Type</td> <td width="313">  <c:out value="${pageContext.request.authType}" /> </td> </tr> <tr> <td width="210">Context Path</td> <td width="313">  <c:out value="${pageContext.request.contextPath}" /> </td> </tr> <tr> <td width="210">Path Information</td> <td width="313">  <c:out value="${pageContext.request.pathInfo}" /> </td> </tr> <tr> <td width="210">Path Translated</td> <td width="313">  <c:out value="${pageContext.request.pathTranslated}" /> </td> </tr> <tr> <td width="210">Query String</td> <td width="313">  <c:out value="${pageContext.request.queryString}" /> </td> </tr> <tr> <td width="210">Request URI</td> <td width="313">  <c:out value="${pageContext.request.requestURI}" /> </td> </tr> </table> <table border="1" width="539"> <tr> <td colspan="2" width="529" bgcolor="#0000FF"> <b> <font color="#FFFFFF" size="4">HTTP Session(pageContext.session.)</font> </b> </td> </tr> <tr> <td width="210">Creation Time</td> <td width="313">  <c:out value="${pageContext.session.creationTime}" /> </td> </tr> <tr> <td width="210">Session ID</td> <td width="313">  <c:out value="${pageContext.session.id}" /> </td> </tr> <tr> <td width="210">Last Accessed Time</td> <td width="313">  <c:out value="${pageContext.session.lastAccessedTime}" /> </td> </tr> <tr> <td width="210">Max Inactive Interval</td> <td width="313">  <c:out value="${pageContext.session.maxInactiveInterval}" /> seconds</td> </tr> <tr> <td width="210">You have been on-line for</td> <td width="313">  <c:out value="${(pageContext.session.lastAccessedTime- pageContext.session.creationTime)/1000}" /> seconds</td> </tr> </table> </body> </html> As you can see in Listing 2.4, this program displays in the browser many of the values contained in the page context. You can easily access the page context by using the <c:out> tag. Use the following line to display the query string for a page: <c:out value="${pageContext.request.queryString}" /> We examine query strings in the section "Processing the Query String," later in this chapter. For now, just be aware that you can access the query string only from within the page context. You can see other page context values in Figure 2.4, which shows the output from Listing 2.4. 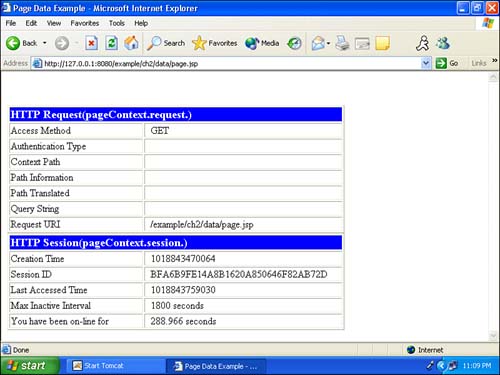 Figure 2.4 shows that the page context data is broken up into the groups request and session . The request data is accessed using the object pageContext.request ; the session data is accessed using the pageContext.session object. The following two tables list the most important request and session properties. Table 2.2 lists the request page context properties, and Table 2.3 lists the session page context properties. Table 2.2. Request Page Context Objects ${pageContext.request.authType} | The authentication type (for example, SSL). | ${pageContext.request.contextPath} | The portion of the request URI that indicates the context of the request. Usually an empty string. | ${pageContext.request.method} | The request method (GET/HEAD/POST). | ${pageContext.request.pathInfo} | Returns extra path information passed to the script (test.jsp/path/). | ${pageContext.request.pathTranslated} | Returns extra path information passed to the script (test.jsp/path/) translated to a real path. | ${pageContext.request.queryString} | The query string from the URL. | ${pageContext.request.requestURI} | The URI portion of the current URL. This is the URL minus the hostname and query string. | Table 2.3. Session Page Context Objects ${pageContext.session.creationTime} | The time (in milliseconds since 1/1/1970) that this session was created. | ${pageContext.session.id} | The ID of this session. | ${pageContext.session.lastAccessedTime} | The time (in milliseconds since 1/1/1970) that this session was last accessed. | ${pageContext.session.maxInactiveInterval} | The number of milliseconds before a session is timed out. | Session and request properties give you access to information about the context in which the page was last ran. However, programmers are generally more interested in data that is retrieved from user forms that are posted to your application. In the next section, we show you how to process form data that has been posted to your application. The paramValues Collection Using the paramValues collection, you can access the form data that a user submitted to your page. To understand how to process data from an HTML form, we must first examine the structure of a form. The form that we use for this example appears in Figure 2.5. 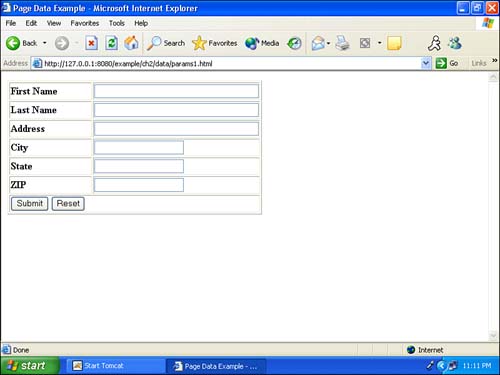 The form in Figure 2.5 requests that users enter their name and address. This form is stored in the file params1.html. An HTML file cannot process data by itself; the form on the HTML page simply collects data and posts it to a file that can process data, such as a JSP page. This HTML file is configured to post the data entered into its form to a JSP page named params2.jsp. You can see the structure of the HTML form in Listing 2.5. Listing 2.5 An HTML File That Creates a Form (params1.html)<html> <head> <title>Page Data Example</title> </head> <body> <table border="1"> <form method="POST" action="params2.jsp"> <tr> <td width="33%"> <b>First Name</b> </td> <td width="73%"> <input type="text" name="first" size="40" /> </td> </tr> <tr> <td width="33%"> <b>Last Name</b> </td> <td width="73%"> <input type="text" name="last" size="40" /> </td> </tr> <tr> <td width="33%"> <b>Address</b> </td> <td width="73%"> <input type="text" name="address" size="40" /> </td> </tr> <tr> <td width="33%"> <b>City</b> </td> <td width="73%"> <input type="text" name="city" size="20" /> </td> </tr> <tr> <td width="33%"> <b>State</b> </td> <td width="73%"> <input type="text" name="state" size="20" /> </td> </tr> <tr> <td width="33%"> <b>ZIP</b> </td> <td width="73%"> <input type="text" name="zip" size="20" /> </td> </tr> <tr> <td colspan="2"> <input type="submit" value="Submit" name="action" /> <input type="reset" value="Reset" name="action" /> </td> </tr> </form> </table> </body> </html> As you can see in Listing 2.6, there are several HTML tags that you can use to create a form. You begin a form with this tag: <form method="POST" action="params2.jsp"> This form tag contains several important pieces of information. The method attribute specifies how this data is to be sent to the Web server. This is usually either POST or GET. POST is almost always used because compared to GET, it can transfer larger amounts of data. The second attribute, named action , is used to specify what action the browser should take after the user clicks the Submit button. Here, we can see that the results of this post will be sent to the page params2.jsp. Internally, the form consists of input elements. Each input element will have its entered value posted to the page that was specified in the action attribute of the form element. Consider the following input item: <input type="text" name="state" size="20" /> This input item presents itself as a text input item. A text input item provides a blank area into which the user can enter text, as seen in Figure 2.5. When users finish entering data, they click the Submit button, which causes the form to post all its data to the page specified in the action attribute of the form tag. The action attribute of our form specified that the file params2.jsp will handle the form data. When the Submit is complete, params2.jsp loads your browser, and you should see something like the display shown in Figure 2.6. 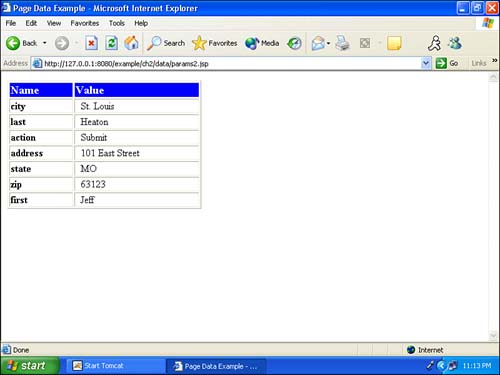 As you can see in Figure 2.6, your data is formatted as a table. The file params2.jsp was built to display all form input items that it is sent. If you were to add an input item to params1.html, a row would automatically be generated in params2.jsp. Listing 2.6 shows the params2.jsp file. Listing 2.6 Accessing Form Data (params2.jsp)<%@ taglib uri="http://java.sun.com/jstl/core" prefix="c" %> <html> <head> <title>Page Data Example</title> </head> <body> <table border="1" width="310"> <tr> <td bgcolor="#0000FF" width="98"> <b> <font color="#FFFFFF" size="4">Name</font> </b> </td> <td bgcolor="#0000FF" width="196"> <b> <font color="#FFFFFF" size="4">Value</font> </b> </td> </tr> <c:forEach var="aItem" items="${paramValues}"> <tr> <td width="98"> <b> <c:out value="${aItem.key}" /> </b> </td> <td width="196">  <c:forEach var="aValue" items="${aItem.value}"> <c:out value="${aValue}" /> </c:forEach> </td> </tr> </c:forEach> </table> </body> </html> The params2.jsp file works by listing every item that is in the paramValues collection. This is done with a <c:forEach> tag, which is used to access every element in a collection. We discuss the <c:forEach> tag in much greater detail in Chapter 5, "Collections, Loops, and Iterators." The following code sets up the file to read every item in the paramValues collection: <c:forEach var="aItem" items="${paramValues}"> This code causes the variable aItem to contain each of the form properties one by one. If a form contains more than one element with the same name, elements of the same name will form an array. The params collection is used to access these element arrays. Consider if the following two form elements were submitted: <input type="text" name="state" size="20" /> <input type="text" name="state" size="20" /> Both form elements have the name state . Consequently, the form element for state would be a collection that contains two items. The code used to loop through an input item's collection (designated by aItem ) is shown here: <c:forEach var="aValue" items="${aItem.value}"> <c:out value="${aValue}" /> </c:forEach> Usually, the form elements that you access are individual elements rather than arrays. The usual case is when you seek input items with specific names. See the section "Working with Forms" later in this chapter for more information. Now, let's see how the paramValues collection can be used for types to retrieve information from the query string as well as forms. The paramValues collection does not just process forms; it also receives information from the query string. For example, the URI script.jsp?a=valuea&b=valueb contains parameters named a and b, contained on the query string. There are other implicit variables that you may find helpful as well. You can access variables from the page, request, session, and application scopes with the variables pageScope, requestScope, sessionScope, and applicationScope. |