The Internet is a wonderful thing. It allows people from all over the world to communicate with each other via their computers. This technology has brought about many new possibilities, including e-mail, instant messaging, and the World Wide Web. Originally, Web sites were very simple. There were HTML pages on any topic you could imagine. People could share whatever they liked, and there was always an audience for it. Those early pages were static visitors couldn't interact with them in any way. The Web quickly evolved and new levels of functionality were added, including images, tables, and forms. This finally allowed visitors to interact with Web sites, giving rise to guestbooks and user polls. Web site developers began to build other neat little tricks into their sites, such as image rollovers and dropdown menus. This allowed interactivity, but true dynamic content was still lacking. Then server processing was introduced. You could now interact with databases, process content, and determine new types of visitor demographics over the Web. ASP.NET is a server technology that brings together the different pieces of the Web to give Web site developers more power than ever. But before we get too far into ASP.NET, let's take a look at how dynamic processing works. Dynamic Processing  | The Internet works on the client/server model. Two computers work together, sending information back and forth, to perform a task. The most common scenario is communication between a server (a computer that holds information) and a client (a computer that wants the information). |  | The client computer sends a request for information to the server computer. The server then responds to the client with the information that was requested of it. This paradigm is the request/response model, and it's an integral part of the client/server model. | A Web server is a computer that holds information about a Web site its HTML pages, images, and so on. The client is the visitor to the Web site (specifically, the visitor's Web browser). Figure 1.1 illustrates this concept. Figure 1.1. The request/response model.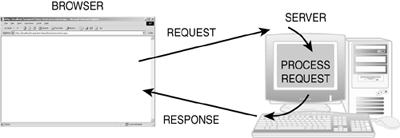 Although this is a marvelous way to communicate and distribute information, it's rather simple and static. It can't provide any dynamic information or processing. The server simply waits around for someone to request information, and then it returns the data that's already stored on its hard drive without really looking at what it's sending. Generally, a static Web request follows these four steps: The client (the Web browser) locates a Web server through its URL (such as www.microsoft.com). The client requests a page (such as index.html). The server sends the requested document. The client receives the document and displays it. Once the client has received the information, the process is finished. The server has no idea what's happening on the client. How could it, since the server and client are two separate computers? They only communicate with one another during the request response process. Once the page has been delivered, the server doesn't care what happens. Enter server processing. This comes in many forms, including the common gateway interface (CGI) and Microsoft's Active Server Pages, which is now referred to as classic ASP. In this scenario, the server takes a look at what it sends before it sends it, and it can take orders from the client. The server can return dynamic data, such as that from a database, calculations it performs, and anything else the client may ask for. The modified work flow is as follows: The client (the Web browser) locates a Web server through its URL (such as www.microsoft.com). The client requests a page (such as index.html). The server examines the requested file and processes any code it contains. The server translates the results of the processing to HTML (if necessary) and sends the requested document to the client. The client receives the document and displays it. Even in this scenario, the process is over once the client receives the page. The server has no idea what the client is doing unless it makes another request. The ASP.NET Difference  | There's another model for communicating between servers and clients, known as the event-driven model. The server waits around for something to happen on the client. Once it does, the server takes action and performs some piece of functionality. | Imagine going to the library. If you followed the request/response model, you'd ask the librarian for information, and he would either give you the answer or point you in the right direction. In the event-driven model, the librarian already knows what you're doing. If you're writing a report and need information on Benjamin Franklin, the librarian brings you the correct items automatically. If you're thirsty, the librarian brings you a glass of water. If you trip and fall, the librarian brings you a bandage. Of course, a Web server can't know what you're thinking, but it can respond to your actions. If you type some text on the Web page, the server responds to it. If you click an image, the server responds. This model is much easier for building applications than using a request/ response scenario. ASP.NET works in this way it detects actions and responds to them. But wait a second! How can ASP.NET know what's going on in your computer? How can the server react to things happening on the client? ASP.NET relies on clever client-side processing to simulate an event-driven model. Client-Side Processing Client-side processing occurs when you place some programming code in an HTML page that the client can understand. This code is simply HTML that the browser executes. For example, take a look at Listing 1.1. Example 1.1 Client-Side Code Is Executed with JavaScript 1: <html> 2: <head> 3: <script language="JavaScript"> 4: <!-- 5: alert("Hello World!"); 6: // --> 7: </script> 8: </head> 9: <body> 10: Welcome to my page! 11: </body> 12: </html> If you're familiar with client-side scripting or JavaScript, this may look familiar. When the browser receives this page, it treats the entire thing as HTML. The <script> tags denote a portion of the page that contains commands, known as script, for the client. If your browser supports client-side scripting, it will understand that line 5 is telling it to display a message box to the user saying "Hello World!", as shown in Figure 1.2. Figure 1.2. Client-side scripting allows you to interact with the client.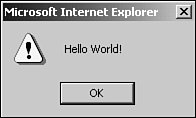 So now you have two places to execute code: on the server, where everything is returned to the client as HTML, and on the client. These two locations of code are distinct and cannot interact with each other. Table 1.1 outlines the differences between client-side and server-side code. Table 1.1. The Differences Between Client-Side and Server-Side Code Location | Description | Client side | This code isn't processed at all by the server. That's solely the client's responsibility. Code is written in scripts plain-text commands that instruct the client to do something. Generally used for performing dynamic client effects, such as image rollovers or displaying message boxes. | Server side | This code is executed only on the server. Any content or information that this code produces must be converted to plain HTML before being sent to the client. Code can be written in scripts as well, but ASP.NET uses compiled languages (more on that later). Used for processing content and returning data. | How ASP.NET Ties It Together So how does ASP.NET know what's going on with a client? Client-side script cannot interact with server-side code. However, ASP.NET cleverly skirts around this problem. The only way for a client to communicate with the server is during a request. Using client-side script, ASP.NET supplies information about what the client is doing during requests. Going back to the library scenario, this is why the librarian seems to have magical powers that can detect whatever you need. He has a network of spies that observe you, even though you don't know it. When you do something, a spy quickly runs to the librarian to tell him what has happened. The librarian can then determine the correct course of action, such as bringing you a glass of water or a bandage. ASP.NET's spies are client-side scripts. Whenever something happens on the client, a client-side script executes and sends information to the server, just as submitting a form sends information to a server. The browser is simply an unknowing accomplice. It thinks it's just doing its job displaying HTML. So, client-side scripts can't exactly interact with the server-side, but they can relay messages via posts to the server. Thus, ASP.NET ties together the server and the client, which allows developers to do things in Web pages that weren't possible before. You don't have to focus on handling requests and responses, but are free to concentrate on building the logic. You can react to user events immediately instead of waiting until forms are submitted. And you can know the structure of the user interface (UI) and how to handle it ahead of time. ASP.NET truly makes developers' lives easier. |