In the early days of the Web, most pages were just static HTML documents. However, eventually more complex Web applications emerged, such as online stores and library catalogs. Other than the ability to exchange data with the server, HTML by itself does not have any real programming power. To make complex Web applications work, the Web server software has to do more than just relay the contents of an HTML file back to a browser; instead it actually has to generate HTML dynamically. One early way of creating dynamic content was to write a program in C or another language that was executed by the Web server. The server acted as a "gateway" between the Web and the program, which could perform activities such as connect to a database and return the desired information, formatted as HTML. In this case, there is no physical file corresponding to the requested Web page, only the HTML output of a program. From the browser's point of view, this arrangement works out quite well, because the client browser does not really know or care what happens on the Web server. As long as the returned stream of information contains HTML codes the browser understands how to display, the browser's request can be fulfilled. Note Arguably, the most powerful enhancements to Web technology have been made on the Web server. However, for completeness we should mention that modern Web browsers also support script code and DHTML (dynamic HTML) to create a more interactive Web experience. Introducing Active Server Pages Several years ago, Microsoft came out with a technology known as Active Server Pages (ASP), which allows you to mix Visual Basic code and HTML code in a single file on your Web server. When IIS processes an ASP file, it returns the HTML directly to the browser, but executes the Visual Basic code on the server. Note When a user requests a standard .htm file, the Web server simply reads the file from the disk drive and passes it back to the browser. However, when IIS processes an .aspx file, it executes code embedded within the file. This code can take different actions on the server, including generate the HTML that is returned to the browser. ASP technology has become very popular and can be found on a significant number of Web sites. To see how it works, we will create a sample Active Server Page. To create your first ASP.NET page, perform the following steps: -
Start the Notepad editor. -
Open the HTML file we created earlier, C:\inetpub\wwwroot\asptest\welcome.htm. -
Alter the contents of the file to match Listing 17.2. -
Save your file in the same folder, with the new name and extension: welcome.aspx. Note Users familiar with previous versions of ASP should note that .aspx is the new extension used with Active Server Pages.NET. (The .asp extension is still supported for backwards compatibility, although you will not have access to ASP.NET features.) Listing 17.2 ASPTEST.ZIP Using ASP.NET to Create a Dynamic Page <HTML> <BODY> <FONT SIZE="+2" COLOR="BLUE"> This is a sample Web Page! </FONT> <BR> <% Dim intCounter AS Integer For intCounter = 1 to 10 Response.Write ("<B> I hope you enjoy it! </B><BR>") Next Response.Write ("Page Created: " & DateTime.Now) %> </BODY> </HTML> Listing 17.2 resembles Listing 17.1, except for the addition of a few lines of VB code, including a For loop. To see how the page looks, open Internet Explorer and enter the address of your new ASP.NET page, which is http://servername/ASPTest/welcome.aspx where servername is the name of your Web server. You should see the Web page as pictured in Figure 17.4. Figure 17.4. ASP.NET allows you to dynamically generate HTML from the Web server by adding Visual Basic code to an .aspx file. 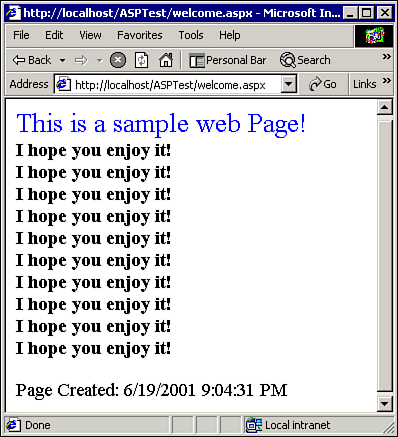 Click the Refresh button in your browser, and you should notice the time displayed on the page will change. This is because the server generated the line of HTML containing the time dynamically. Using Listing 17.2 as a guide, note the following properties of a typical ASP.NET page: The script tags <% and %> in an ASP.NET file mark Visual Basic code to be executed by the server. Any other text in the file, such as standard HTML, is sent directly back to the client browser. Several built-in objects are included in ASP.NET for manipulating Web content. In Listing 17.2, the Response.Write method is used to send a string of HTML back to the client browser. The client browser cannot view the server-side code. If you choose View Source, you will only see the HTML generated by the code, not the code itself. Although this example seems trivial, it does open up some interesting possibilities. Because ASP.NET allows you to create pages "on the fly" with Visual Basic code, you can perform more complex actions such as connecting to a database and returning HTML containing field values. Key Enhancements in ASP.NET Users of previous versions of ASP will notice the following major improvements: Compiled Code The code in ASP.NET pages is compiled for faster execution. (Previous versions of ASP were interpreted.) When you make changes to an .aspx file, IIS recompiles the file the next time it is requested. Robust Language The full power of the Visual Basic language (or any language in the Visual Studio family) is available for use with ASP.NET. (Previous versions of ASP used only the more limited VBScript and JScript languages on the server.) Forms-based Programming A new programming model, Webforms, has been created that allows greater separation between the user interface and the code in an ASP page. Of all those enhancements, the last one listed promises to make the most significant impact on the way you develop Web applications. ASP.NET is a core technology in Visual Studio .NET, used in both Web Applications and Web Service projects. Rather than thinking about sending data to and from the client browser, you can program against a control-and-event model you are already familiar with from standard VB applications. Programming with Web forms within Visual Studio, which was introduced in Chapter 3, "Creating Your First Web Application," is covered in more detail in the next two chapters. For more details on Web Applications, p.475 For more details on Web Controls, p.501 In the following sections, we will explore ASP.NET at the page level, introducing you to its built-in classes and fundamental concepts. If you are currently an ASP developer, read this chapter to get started quickly with ASP.NET. |