18.10. Reading and Deserializing Data from a Sequential-Access Text File The preceding section showed how to create a sequential-access file using object serialization. In this section, we discuss how to read serialized objects sequentially from a file. Figure 18.15 reads and displays the contents of the file created by the program in Fig. 18.14. Line 10 creates the BinaryFormatter that will be used to read objects. The program opens the file for input by creating a FileStream object (line 35). The name of the file to open is specified as the first argument to the FileStream constructor. Figure 18.15. Sequential file read using deserialzation. 1 ' Fig. 18.15: FrmReadSequentialAccessFile.vb 2 ' Reading a sequential-access file using deserialization. 3 Imports System.IO 4 Imports System.Runtime.Serialization.Formatters.Binary 5 Imports System.Runtime.Serialization 6 Imports BankLibrary 7 8 Public Class FrmReadSequentialAccessFile 9 ' object for deserializing Record in binary format 10 Private reader As New BinaryFormatter() 11 Private input As FileStream ' stream for reading from a file 12 13 ' invoked when user clicks Open button 14 Private Sub btnOpen_Click(ByVal sender As System.Object, _ 15 ByVal e As System.EventArgs) Handles btnOpen.Click 16 ' create dialog box enabling user to open file 17 Dim fileChooser As New OpenFileDialog() 18 Dim result As DialogResult = fileChooser.ShowDialog() 19 Dim fileName As String ' name of file containing data 20 21 ' exit event handler if user clicked Cancel 22 If result = Windows.Forms.DialogResult.Cancel Then 23 Return 24 End If 25 26 fileName = fileChooser.FileName ' get specified file name 27 ClearTextBoxes() 28 29 ' show error if user specified invalid file 30 If fileName = "" Or fileName Is Nothing Then 31 MessageBox.Show("Invalid File Name", "Error", _ 32 MessageBoxButtons.OK, MessageBoxIcon.Error) 33 Else 34 ' create FileStream to obtain read access to file 35 input = New FileStream(fileName, FileMode.Open, FileAccess.Read) 36 37 btnOpen.Enabled = False ' disable Open File button 38 btnNext.Enabled = True ' enable Next Record button 39 End If 40 End Sub ' btnOpen_Click 41 42 ' invoked when user clicks Next button 43 Private Sub btnNext_Click(ByVal sender As System.Object, _ 44 ByVal e As System.EventArgs) Handles btnNext.Click 45 ' deserialize Record and store data in TextBoxes 46 Try 47 ' get next RecordSerializable available in file 48 Dim record As RecordSerializable = _ 49 CType(reader.Deserialize(input), RecordSerializable) 50 51 ' store Record values in temporary string array 52 Dim values() As String = { _ 53 record.Account.ToString(), record.FirstName.ToString(), _ 54 record.LastName.ToString(), record.Balance.ToString()} 55 56 ' copy string array values to TextBox values 57 SetTextBoxValues(values) 58 ' handle exception when there are no Records in file 59 Catch ex As SerializationException 60 input.Close() ' close FileStream if no Records in file 61 btnOpen.Enabled = True ' enable Open File button 62 btnNext.Enabled = False ' disable Next Record button 63 64 ClearTextBoxes() 65 66 ' notify user if no Records in file 67 MessageBox.Show("No more records in file", "", _ 68 MessageBoxButtons.OK, MessageBoxIcon.Information) 69 End Try 70 End Sub ' btnNext_Click 71 End Class ' FrmReadSequentialAccessFile 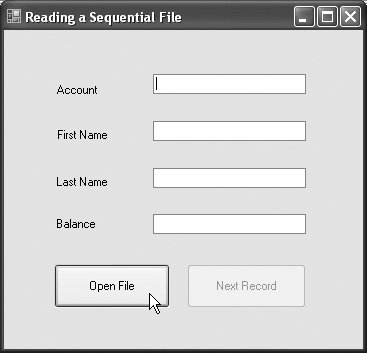 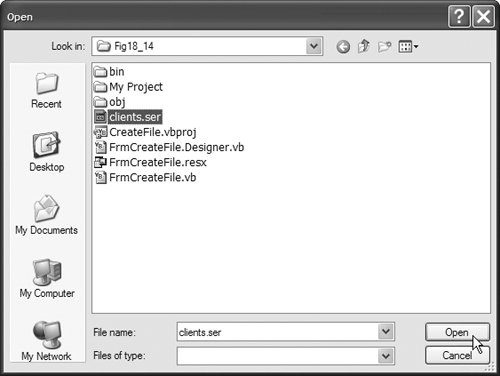 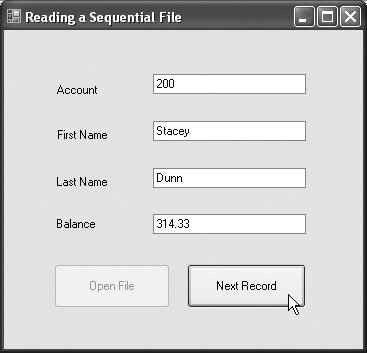 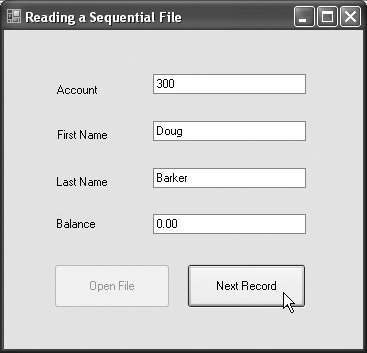 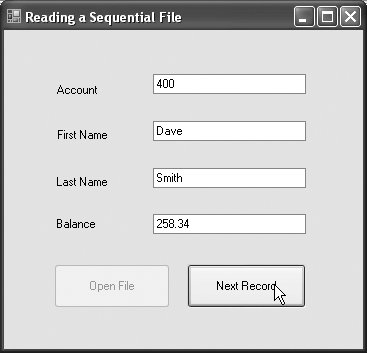 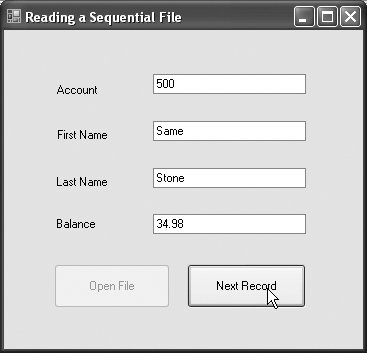 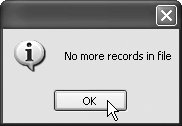 | The program reads objects from a file in event handler btnNext_Click (lines 4370). We use method Deserialize (of the BinaryFormatter created in line 10) to read the data (lines 4849). Note that we cast the result of Deserialize to type RecordSerializable (line 49)this cast is necessary because Deserialize returns a reference of type object and we need to access properties that belong to class RecordSerializable. If an error occurs during deserialization, a SerializationException is thrown, and the FileStream object is closed (line 60). |