Many more event-listening interfaces are supported in Java. In this section we will examine a number of the most commonly used ones. Events can be broken down into two types, semantic or high-level events, and low-level events. The high-level events deal with meaning more than with the hardware action that caused them. The semantic events that Java supports are ActionEvent A button is clicked or a menu item is selected. AdjustmentEvent A scrollbar is adjusted. ItemEvent A user selected from a list of objects. TextEvent A text field has been changed. In addition, there are eight low-level event classes: ComponentEvent A component was resized, moved, shown, or hidden. KeyEvent A key was pressed or released. MouseEvent A mouse button was pressed or released. The mouse was moved or dragged. FocusEvent A component got or lost focus. WindowEvent A window was iconified, activated, deactivated, and so on. ContainerEvent A component was put on or removed from the container. PaintEvent This event is not intended to be used with a listener, but contains methods that can be overridden. InputEvent This event is mainly used to intercept events before they get to the component so that a Component event won't be thrown. Associated with each event class is at least one event-listener interface. The following is a list of event classes, and the listener interface(s) that support it. Table 14.1. The Events and Their Associated Listeners Event | Listener |
---|
ActionEvent | ActionListener | AdjustmentEvent | AdjustmentListener | ItemEvent | ItemListener | TextEvent | TextListener | ComponentEvent | ComponentListener | KeyEvent | KeyListener | MouseEvent | MouseListener, MouseMotionListener | FocusEvent | FocusListener | WindowEvent | WindowListener | ContainerEvent | ContainerListener | Notice that the MouseEvent has two listeners associated with it. The reason for this is that the mouse moves in the x,y plane, but it also has buttons that act somewhat like keys on the keyboard. Each of these interfaces has one or more methods associated with it. The WindowListener has the most methods, with seven. The ActionListener, AdjustmentListener, ItemListener, and TextListener have only one each. Some of the listeners that have several methods also have a special class called an adapter class provided for it. These classes implement the interface for you and provide dummy calls to each of the methods. You extend these classes and override the methods that you want to implement. If you don't implement them all, no error is thrown because the adapter class has already implemented it for you. The adapter classes are ComponentAdapter ContainerAdapter FocusAdapter KeyAdapter MouseAdapter MouseMotionAdapter WindowAdapter The following examples use some of the interfaces and adapter classes to demonstrate how each works. The ActionListener Interface The ActionListener interface is the easiest interface to implement. It has only one method that must be created, actionPerformed(). The actionPerformed() method expects to receive a java.awt.ActionEvent object as a parameter. The ActionEvent class has three methods that you can use to determine how to proceed after a semantic action event occurs: getActionCommand() This returns the name associated with the command that just occurred. By retrieving this name, your program can differentiate among the different components that generate action event. getModifiers() This tells you if a shift or control key was pressed when the action event was created. paramString() This method returns the param string associated with this action event, if any. See Listings 14.1, 14.2, and 14.3 for examples that implement the ActionListener interface. Because there is only one method in this interface, there is no need for an adapter class for it. The AdjustmentListener Interface The AdjustmentListener interface is another single-method interface. This interface is associated with the Scrollbar class. Whenever a Scrollbar's elevator (little box) is moved up or down, an AdjustmentEvent is generated. Your program can then obtain the current value of the Scrollbar and react to it. The original purpose of the Scrollbar class was to enable scrolling of text in both directions. The ScrollPane does that as well, so many programmers skip the Scrollbar and always use ScrollPanes. Listing 14.4 shows how this works. Listing 14.4 The TestScrollbar.java File /* * TestScrollbar.java * * Created on August 9, 2002, 2:32 PM */ package ch14; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts */ public class TestScrollbar extends Frame implements AdjustmentListener { Scrollbar sb; TextField tField; /** Creates a new instance of TestScrollbar */ public TestScrollbar() { sb=new Scrollbar(Scrollbar.VERTICAL, 0, 1, 0, 255); sb.addAdjustmentListener(this); add(sb); tField = new TextField(30); add(tField); this.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a Scrollbar Object"); setBounds( 100, 100, 400, 400); setVisible(true); } public void adjustmentValueChanged(AdjustmentEvent ae) { String newString = String.valueOf(sb.getValue()); tField.setText(newString); } public static void main(String[] args) { TestScrollbar tsb= new TestScrollbar(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } The AdjustmentListener interface is implemented by the class itself. public class TestScrollbar extends Frame implements AdjustmentListener The Scrollbar object is created to be vertical, with an initial value of 0, an elevator of size 1, with a minimum set to 0 and a maximum set to 255. sb=new Scrollbar(Scrollbar.VERTICAL, 0, 1, 0, 255); We add a listener to the scrollbar, which is this class itself. sb.addAdjustmentListener(this); We add the scrollbar to the frame. add(sb); This is the only method that is required by this interface. public void adjustmentValueChanged(AdjustmentEvent ae) The getValue() method returns an int, so we convertit to a String before we display it. String newString = String.valueOf(sb.getValue()); tField.setText(newString); } The result of running this example is shown here in Figure 14.3. Figure 14.3. The AdjustmentListener interface is used to process events generated by scrollbars. 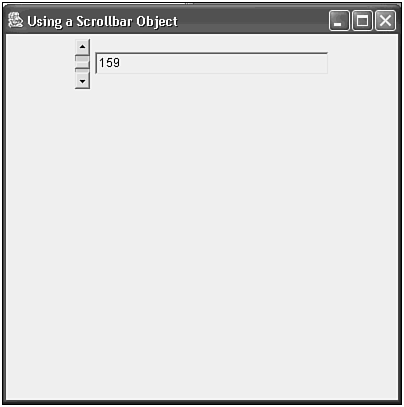 Notice how smooth the operation of the scrollbar and text field is. It is hard to believe that an event is being generated each time the Scrollbar's value changes. The ItemListener Interface The ItemListener interface requires only one method, itemStateChanged(). This method is called whenever the user changes an object such as a List, Checkbox, or Choice. The most useful method that the ItemEvent provides is getItemSelectable(), which tells what item created the event. After you know which item created the event, you can cast the item to its subtype and use the subtype's methods to discover what the user intends to be changed. Listing 14.5 shows an example of how this listener works with checkboxes. Listing 14.5 The TestCheckBoxes2.java File /* * TestCheckboxes.java * * Created on July 30, 2002, 2:36 PM */ package ch14; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestCheckboxes2 extends Frame implements ItemListener { Checkbox cbWhiteBread; Checkbox cbWheatBread; Checkbox cbRyeBread; Checkbox cbToasted; TextField tField; /** Creates new TestCheckboxes2*/ public TestCheckboxes2() { cbWhiteBread = new Checkbox("White Bread"); cbWhiteBread.setState(false); cbWhiteBread.addItemListener(this); cbWheatBread = new Checkbox("Wheat Bread"); cbWheatBread.setState(false); cbWheatBread.addItemListener(this); cbRyeBread = new Checkbox("Rye Bread"); cbRyeBread.setState(false); cbRyeBread.addItemListener(this); cbToasted= new Checkbox("Toasted"); cbToasted.setState(false); cbToasted.addItemListener(this); tField = new TextField(30); setLayout(new FlowLayout()); add(cbWhiteBread); add(cbWheatBread); add(cbRyeBread); add(cbToasted); add(tField); addWindowListener(new WinCloser()); setTitle("Using Checkboxes"); setBounds( 100, 100, 300, 300); setVisible(true); } public void itemStateChanged(ItemEvent ie) { Checkbox cb = (Checkbox)ie.getItemSelectable(); if( cb.getState()) tField.setText(cb.getLabel() + " was set to true"); else tField.setText(cb.getLabel() + " was set to false"); } public static void main(String[] args) { TestCheckboxes2 tcb = new TestCheckboxes2(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } This class implements the ItemListener interface itself. public class TestCheckboxes2 extends Frame implements ItemListener We create four Checkboxes and one text field to display feedback. Checkbox cbWhiteBread; Checkbox cbWheatBread; Checkbox cbRyeBread; Checkbox cbToasted; TextField tField; We populate each of the checkboxes, give it a state, and add the listener. cbWhiteBread = new Checkbox("White Bread"); cbWhiteBread.setState(false); cbWhiteBread.addItemListener(this); The itemStateChanged() method is handed an ItemEvent object. public void itemStateChanged(ItemEvent ie) { The getItemSelectable() method hands the item back that caused the event. We have to cast it to the Checkbox data type before we can call methods on it. Checkbox cb = (Checkbox)ie.getItemSelectable(); The getState() method returns either true or false, based on the current value of the Checkbox. We examine this value, and then get the text by calling the getLabel() method. if( cb.getState()) tField.setText(cb.getLabel() + " was set to true"); else tField.setText(cb.getLabel() + " was set to false"); The result of running this example is shown here in Figure 14.4. Figure 14.4. The ItemListener interface is used to process events generated by Lists, Checkboxes, and Choices. 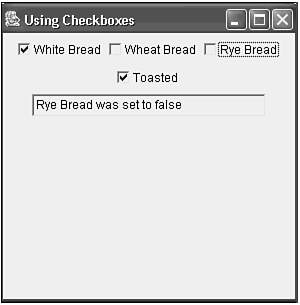 Notice that the latest change that was made is displayed. The Checkbox that you are most interested in is the one that changed most recently. The TextListener Interface The TextListener is another one-method interface that has no Adapter class associated with it. TextEvents are created by text components whenever their contents change. These events enable your program to take action whenever a keystroke takes place inside a text component that is being listened to. Listing 14.6 shows an example where we used the TextListener interface to verify that a keystroke is a number before we store it in another text field. Listing 14.6 The TestTextListener.java File /* * TestTextListener.java * * Created on August 9, 2002, 2:32 PM */ package ch14; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts */ public class TestTextListener extends Frame implements TextListener { TextField tField; TextField tField2; /** Creates a new instance of TestTextListener */ public TestTextListener() { tField = new TextField(20); tField2 = new TextField(20); tField.addTextListener(this); add(tField); add(tField2); this.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a TextListener Object"); setBounds( 100, 100, 400, 400); setVisible(true); } public static void main(String[] args) { TestTextListener ttl= new TestTextListener(); } public void textValueChanged(java.awt.event.TextEvent te) { TextField tf = (TextField)te.getSource(); String s1 = tf.getText(); int strlen = s1.length(); String lastCharString = s1.substring(strlen-1); try { int i = Integer.parseInt(lastCharString); tField2.setText(tField2.getText() + lastCharString); }catch (NumberFormatException nfe) { System.out.println("not a number"); } } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } We implement the TextListener in this class instead of creating a whole different class just to hold one method(). public class TestTextListener extends Frame implements TextListener We declare two text fields: one to receive text and the other to display the filtered version. TextField tField; TextField tField2; We instantiate both of the text fields, but we only listen to the input field. tField = new TextField(20); tField2 = new TextField(20); tField.addTextListener(this); The textValueChanged() method is required by the TextListener interface. It receives a TextEvent, which contains information about the TextField that caused the event. public void textValueChanged(java.awt.event.TextEvent te) We obtain a handle to the text field that caused the event to be created. We will use this handle to call methods to discover the source, contents, and so on. TextField tf = (TextField)te.getSource(); We get the current state of the contents of the text field. String s1 = tf.getText(); We are only interested in the last character entered, so we use substring() to obtain a string with only this character. int strlen = s1.length(); String lastCharString = s1.substring(strlen-1); We use the Java error trapping mechanism to help our program decide whether the character typed in is an integer. try { If you try to convert a string containing a number to an integer, it works fine. If the string contains a non-number, an exception is thrown. int i = Integer.parseInt(lastCharString); If the exception is thrown, this line never executes. tField2.setText(tField2.getText() + lastCharString); Because we catch and handle the exception here, the program continues without interruption. The user is never aware that the exception occurred. }catch (NumberFormatException nfe) { System.out.println("not a number"); } The result of running this program is shown here in Figure 14.5. Figure 14.5. The TextListener interface is great for filtering input. 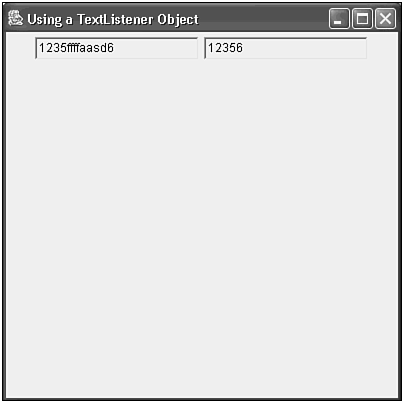 Using the Java exception handling mechanism to create program logic is very convenient, but somewhat more resource consumptive than other techniques. It should only be used in situations where CPU resources are plentiful. Normally, a GUI program is light enough that the CPU cycles needed are readily available. The KeyListener and KeyAdapter Interfaces The KeyListener interface requires the implementation of three methods: keyPressed(), keyReleased(), and keyTyped(). Whenever a key is pressed, the keyPressed() method is called. When that key is released, keyReleased() is called. When a couplet of press and release is complete, the keyTyped() method is called. The KeyAdapter class provides dummy implementations of each of these methods. This enables you to extend this class and implement only those methods that you choose to. One complication that arises from the fact that KeyAdapter is a class and not an interface is that classes that already extend one class, such as Frame, cannot extend a second class under the rules of Java. The reason for this is that certain logical conundrums can occur when a class inherits from more than one class; if those classes share a common ancestor. To solve this problem, the Java fathers decided to outlaw multiple inheritance altogether. Some would argue that they went too far, but our job is not to redesign the language, but rather to gripe about it. Listing 14.7 contains a class that extends the KeyAdapter class. This is done to add variety to the examples. Listing 14.7 The TestKeyAdapter /* * TestKeyAdapter.java * * Created on August 9, 2002, 2:32 PM */ package ch14; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts */ public class TestKeyAdapter extends Frame { TextField tField; TextField tField2; TextField tField3; TextField tField4; /** Creates a new instance of TestKeyAdapter */ public TestKeyAdapter() { tField = new TextField(15); tField2 = new TextField(15); tField3 = new TextField(15); tField4 = new TextField(20); Panel testPanel = new Panel(); testPanel.setBackground(Color.gray); KeyStrokeHandler ksh = new KeyStrokeHandler(); tField.addKeyListener(ksh); testPanel.add(tField); testPanel.add(tField2); testPanel.add(tField3); testPanel.add(tField4); add(testPanel); this.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a KeyAdapter Object"); setBounds( 100, 100, 600, 400); setVisible(true); } public static void main(String[] args) { TestKeyAdapter tka= new TestKeyAdapter(); } class KeyStrokeHandler extends KeyAdapter { public void keyTyped(KeyEvent ke) { String newString = String.valueOf(ke.getKeyChar()); tField2.setText(newString); tField3.setText("keyTyped() was called"); } public void keyPressed(KeyEvent ke) { String newString = String.valueOf(ke.getKeyChar()); tField2.setText(newString); tField4.setText("keyPressed() was called"); } public void keyReleased(KeyEvent ke) { String newString = String.valueOf(ke.getKeyChar()); tField2.setText(newString); tField4.setText("keyReleased() was called"); } } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } Notice that this class does not extend the KeyAdapter class itself because it already extends the Frame class and a second extends statement or class name would generate a compiler error. public class TestKeyAdapter extends Frame { We create the usual set of text fields on a panel so that we will have a place to put text on. We then declare a new handle to a class called KeyStrokeHandler, which we create ourselves in this same class. KeyStrokeHandler ksh = new KeyStrokeHandler(); We make this class the key listener for one of the text fields. tField.addKeyListener(ksh); Next we create an inner class to serve as the extension of the KeyAdapter class. An inner class is declared inside the definition of another class. Because it is located inside the TestKeyAdapter class, it has visibility to the class-level variables, which are the text boxes in this example. class KeyStrokeHandler extends KeyAdapter { We override the three methods in the KeyAdapter class. These methods get called whenever the key action that they represent occurs. public void keyTyped(KeyEvent ke) { String newString = String.valueOf(ke.getKeyChar()); tField2.setText(newString); tField3.setText("keyTyped() was called"); } public void keyPressed(KeyEvent ke) { String newString = String.valueOf(ke.getKeyChar()); tField2.setText(newString); tField4.setText("keyPressed() was called"); } public void keyReleased(KeyEvent ke) { String newString = String.valueOf(ke.getKeyChar()); tField2.setText(newString); tField4.setText("keyReleased() was called"); } } Running this example generates the result shown in Figure 14.6. Figure 14.6. The KeyAdapter class enables you to monitor each keystroke and differentiate between the press and the release. 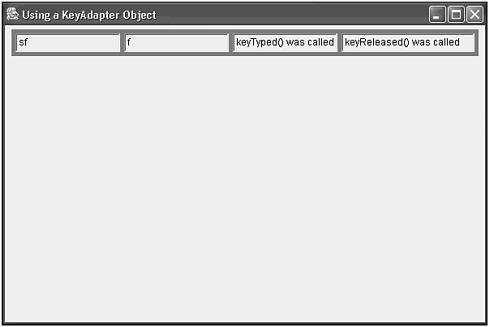 The WindowListener and WindowAdapter Interfaces The WindowListener interface is used to receive notification when an event occurs that pertains to the window as a whole. We have used the WindowAdapter class to declare a class that handles the windowClosing() method in most of our AWT examples. This is required because AWT will not stop the process just because the window is closed. Listing 14.7 has the following class declared at the bottom. class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } All this class does is terminate the whole process by executing the System.exit(0) command. There are seven methods in this interface, however. Listing 14.8 shows these in action. Listing 14.8 The TestWindowListener.java File /* * TestWindowListener.java * * Created on August 9, 2002, 2:32 PM */ package ch14; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts */ public class TestWindowListener extends Frame { Scrollbar sb; TextField tField; /** Creates a new instance of TestWindowListener */ public TestWindowListener() { sb=new Scrollbar(Scrollbar.VERTICAL, 0, 1, 0, 255); add(sb); tField = new TextField(30); add(tField); this.setLayout(new FlowLayout()); addWindowListener(new WinHandler()); setTitle("Using a Scrollbar Object"); setBounds( 100, 100, 400, 400); setVisible(true); } public static void main(String[] args) { TestWindowListener twl= new TestWindowListener(); } } class WinHandler implements WindowListener { public void windowClosing(WindowEvent e) { System.out.println("windowClosing() called"); System.exit(0); } public void windowActivated(java.awt.event.WindowEvent windowEvent) { System.out.println("windowActivated() called"); } public void windowClosed(java.awt.event.WindowEvent windowEvent) { System.out.println("windowClosed() called"); } public void windowDeactivated(java.awt.event.WindowEvent windowEvent) { System.out.println("windowDeactivated() called"); } public void windowDeiconified(java.awt.event.WindowEvent windowEvent) { System.out.println("windowDeiconified() called"); } public void windowIconified(java.awt.event.WindowEvent windowEvent) { System.out.println("windowIconified called"); } public void windowOpened(java.awt.event.WindowEvent windowEvent) { System.out.println("windowOpened() called"); } } This class shows us another look at class structures. Instead of declaring this class to be its own listener, we declare a separate class to do it. In addition, we never created a handle to this class, we just placed the new statement inside the add listener method call as a parameter. addWindowListener(new WinHandler()); The Windhandler class implements the WindowListener interface and provides implementations for all the methods that are required. Notice that this class is not an inner class because it is declared outside the definition of the TestWindowListener class. class WinHandler implements WindowListener { public void windowClosing(WindowEvent e) { System.out.println("windowClosing() called"); System.exit(0); } public void windowActivated(java.awt.event.WindowEvent windowEvent) { System.out.println("windowActivated() called"); } public void windowClosed(java.awt.event.WindowEvent windowEvent) { System.out.println("windowClosed() called"); } public void windowDeactivated(java.awt.event.WindowEvent windowEvent) { System.out.println("windowDeactivated() called"); } public void windowDeiconified(java.awt.event.WindowEvent windowEvent) { System.out.println("windowDeiconified() called"); } public void windowIconified(java.awt.event.WindowEvent windowEvent) { System.out.println("windowIconified called"); } public void windowOpened(java.awt.event.WindowEvent windowEvent) { System.out.println("windowOpened() called"); } } When you run this program, you need the command window open so that you can see the output from System.out.println() calls. Figure 14.7 shows the result of running this example. Figure 14.7. The WindowListener interface keeps your program aware of what is happening with the window. 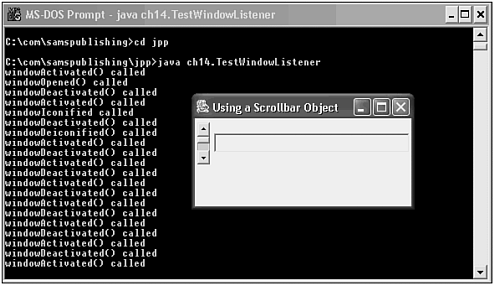 The ComponentListener and ComponentAdapter Interfaces The ComponentListener interface contains four methods that tell your program whenever a component is hidden, made visible, moved, or resized. The ComponentAdapter provides dummy versions of all four methods. Listing 14.9 shows an example of this interface. Listing 14.9 The TestComponentListener.java File /* * TestComponentListener.java * * Created on July 30, 2002, 2:36 PM */ package ch14; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestComponentListener extends Frame implements ComponentListener { Button btnExit; /** Creates new TestComponentListener*/ public TestComponentListener() { btnExit = new Button("Exit"); btnExit.setFont(new Font("Courier", Font.BOLD, 24)); btnExit.setBackground(Color.cyan); addComponentListener(this); add(btnExit); this.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a ComponentListener"); setBounds( 100, 100, 300, 300); setVisible(true); } public void componentResized(ComponentEvent ce) { System.out.println("Component resized"); } public void componentMoved(ComponentEvent ce) { System.out.println("Component moved"); } public void componentHidden(ComponentEvent ce) { System.out.println("Component hidden"); } public void componentShown(ComponentEvent ce) { System.out.println("Component shown"); } public static void main(String[] args) { TestComponentListener tcl = new TestComponentListener(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } The class itself is the component and the implementer of the interface to handle it. addComponentListener(this); We implement all four of the required methods in this class. public void componentResized(ComponentEvent ce) { System.out.println("Component resized"); } public void componentMoved(ComponentEvent ce) { System.out.println("Component moved"); } public void componentHidden(ComponentEvent ce) { System.out.println("Component hidden"); } public void componentShown(ComponentEvent ce) { System.out.println("Component shown"); } The result is shown in Figure 14.8. Figure 14.8. The ComponentListener interface keeps your program aware of what is happening with a registered component. 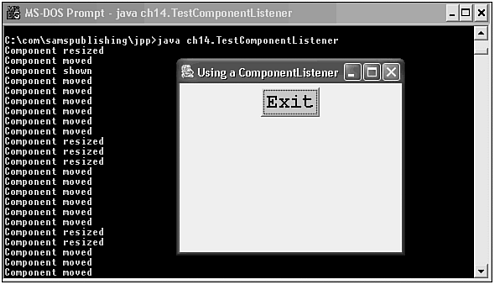 The MouseListener and MouseAdapter Interfaces The MouseListener provides a high level look at the mouse. It tells you when a mouse is over a component that you are listening for, as well as when a mouse button has been clicked. The MouseAdapter provides the usual dummy implementations of each of the methods in this interface. Listing 14.10 shows an example of the MouseListener interface. Listing 14.10 The TestMouseListener.java File /* * TestMouseListener.java * * Created on July 30, 2002, 2:36 PM */ package ch14; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestMouseListener extends Frame implements MouseListener { Button btnExit; /** Creates new TestComponentListener*/ public TestMouseListener() { btnExit = new Button("Exit"); btnExit.setFont(new Font("Courier", Font.BOLD, 24)); btnExit.setBackground(Color.cyan); addMouseListener(this); add(btnExit); this.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a ComponentListener"); setBounds( 100, 100, 300, 300); setVisible(true); } public void mouseEntered(MouseEvent me) { System.out.println("Mouse Entered"); System.out.println(me.getComponent().getName()); } public void mouseExited(MouseEvent me) { System.out.println("Mouse Exited"); System.out.println(me.getComponent().getName()); } public void mousePressed(MouseEvent me) { System.out.println("Mouse Pressed"); } public void mouseReleased(MouseEvent me) { System.out.println("Mouse Released"); } public void mouseClicked(MouseEvent me) { System.out.println("Mouse Clicked"); } public static void main(String[] args) { TestMouseListener tml = new TestMouseListener(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } This class is its own listener. public class TestMouseListener extends Frame implements MouseListener The whole frame is the component that we are tracking the mouse with. addMouseListener(this); We provide implementation for all five methods. public void mouseEntered(MouseEvent me) { System.out.println("Mouse Entered"); System.out.println(me.getComponent().getName()); } public void mouseExited(MouseEvent me) { System.out.println("Mouse Exited"); System.out.println(me.getComponent().getName()); } public void mousePressed(MouseEvent me) { System.out.println("Mouse Pressed"); } public void mouseReleased(MouseEvent me) { System.out.println("Mouse Released"); } public void mouseClicked(MouseEvent me) { System.out.println("Mouse Clicked"); } When the mouse is over the Exit button, that component is consuming the event and the frame never sees it. The result of running this example is shown in Figure 14.9. Figure 14.9. The MouseListener interface enables your program to track the movements of the mouse when it enters and exits, as well as when it is clicked. 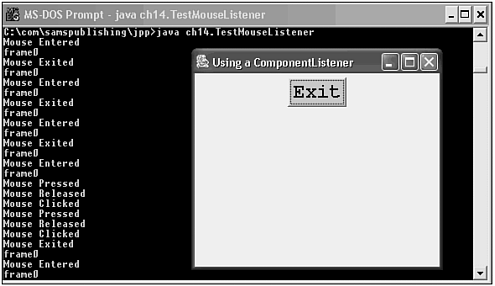 Notice that the actual coordinates of the mouse are not provided by this interface. The MouseMotionListener interface, which we will study in the following section, provides that functionality. The MouseMotionListener and MouseMotionAdapter Interfaces The final interface in this chapter is the MouseMotionListener interface. This interface receives a MouseEvent that it uses to determine the coordinates of the mouse and whether a mouse button is pressed to make it a dragging motion. The MouseMotionAdapter is also provided to make implementation simpler. Listing 14.11 shows an example. Listing 14.11 The MouseMotionListener.java File /* * TestMouseMotionListener.java * * Created on July 30, 2002, 2:36 PM */ package ch14; import java.awt.*; import java.awt.event.*; /** * * @author Stephen Potts * @version */ public class TestMouseMotionListener extends Frame implements MouseMotionListener { Button btnExit; /** Creates new TestMouseMotionListener*/ public TestMouseMotionListener() { btnExit = new Button("Exit"); btnExit.setFont(new Font("Courier", Font.BOLD, 24)); btnExit.setBackground(Color.cyan); addMouseMotionListener(this); add(btnExit); this.setLayout(new FlowLayout()); addWindowListener(new WinCloser()); setTitle("Using a ComponentListener"); setBounds( 100, 100, 300, 300); setVisible(true); } public void mouseMoved(MouseEvent me) { System.out.println("Mouse was Moved"); System.out.println(me.getX() + "," + me.getY()); } public void mouseDragged(MouseEvent me) { System.out.println("Mouse was Dragged"); System.out.println(me.getX() + "," + me.getY()); } public static void main(String[] args) { TestMouseMotionListener tmml = new TestMouseMotionListener(); } } class WinCloser extends WindowAdapter { public void windowClosing(WindowEvent e) { System.exit(0); } } This class serves as its own listener. public class TestMouseMotionListener extends Frame implements MouseMotionListener We add the listener to the whole frame. addMouseMotionListener(this); We provide implementations for both required methods. public void mouseMoved(MouseEvent me) { System.out.println("Mouse was Moved"); System.out.println(me.getX() + "," + me.getY()); } public void mouseDragged(MouseEvent me) { System.out.println("Mouse was Dragged"); System.out.println(me.getX() + "," + me.getY()); } The result of running this example is shown in Figure 14.10. Figure 14.10. . The MouseMotion Listener interface enables your program to track the movements of the mouse at the x and y coordinate level. 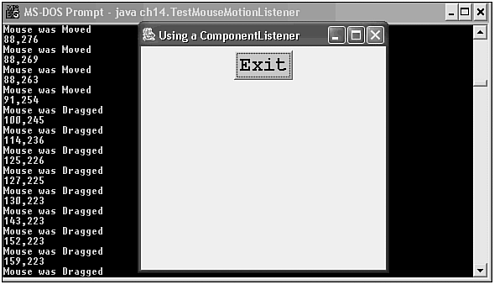 Notice that every change of even one pixel causes an event to occur. This gives you the fine control needed to create smooth screen movement. |