Once you have created a product catalog, as I have now, the actual shopping cart itself can be surprisingly simple. The method I've chosen to use in this example is to record the product IDs, prices, and quantities in a session. Knowing these three things will allow the scripts to calculate totals and do everything else required. These next two examples will provide all the necessary functionality for the shopping cart. The first script, add_cart.php, will add items to the shopping cart. The second, view_cart.php, will both display the contents of the cart and allow the customer to update it. Adding items The add_cart.php script will take one argumentthe ID of the print being purchasedand will use this to update the cart. The cart itself is a session variable, meaning it's accessed through the $_SESSION['cart'] variable. The cart will be a multidimensional array whose keys will be product IDs. The values of the array elements will themselves be arrays: one element for the quantity and another for the price (Table 14.6). Table 14.6. The $_SESSION['cart'] variable will be a multidimensional array. Each array element will use the print ID for its index. Each array value will be an array of two elements: the quantity of that print ordered and the price of that print.Sample $_SESSION['cart'] Values |
---|
(INDEX) | QUANTITY | PRICE |
---|
2 | 1 | 54.00 | 568 | 2 | 22.95 | 37 | 1 | 33.50 |
Enabling mime_content_type() This function will work on Windows, but only after you do a little configuring of PHP. The full instructions for configuring PHP are in Appendix A, "Installation," but what you'll need to do is… In your php.ini file, enable the Mime Magic extension by removing the semicolon in this line: ; extension=php_mime_magic.dll Then restart your Web server and you should be good to go! Again, if you don't understand the configuration process, see the detailed instructions in the first appendix. |
To create add_cart.php: 1. | Create a new PHP document in your text editor (Script 14.9).
<?php # Script 14.9 - add_cart.php Script 14.9. This script adds products to the shopping cart by referencing the product (or print) ID. 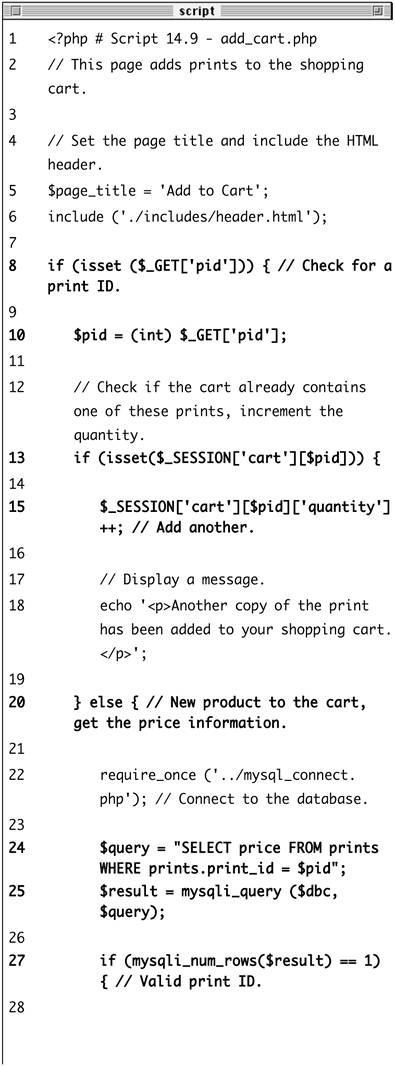 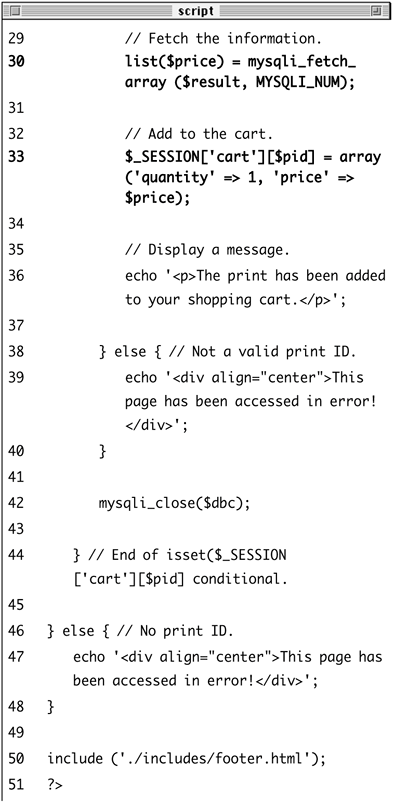
| 2. | Include the page header and check that a print was selected.
$page_title = 'Add to Cart'; include ('./includes/header.html'); if (isset ($_GET['pid'])) { As with the view_print.php script, I do not want to proceed with this script if no print ID has been received.
| 3. | Determine if a copy of this print has already been selected.
$pid = (int) $_GET['pid']; if (isset($_SESSION['cart'][$pid])) { $_SESSION['cart'][$pid]['quantity' ]++; echo '<p>Another copy of the print has been added to your shopping cart.</p>'; Before adding the current print to the shopping cart (by setting its quantity to 1), I need to check if a copy is already in the cart. For example, if the customer selected print #519 and then decided to order another, the cart should now contain two copies of the print. So I first check if the cart has a value for the current print ID. If so, the quantity is determined by adding 1 to that value and a message is displayed (Figure 14.31).
Figure 14.31. The result after clicking an Add to Cart link for an item that was already present in the shopping cart. 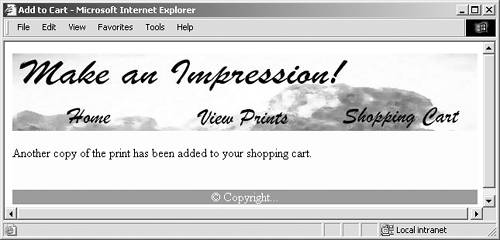
| 4. | Add the new product to the cart.
} else { require_once ('../mysql_connect. php); $query = "SELECT price FROM prints WHERE prints.print_id = $pid; $result = mysqli_query ($dbc, $query); if (mysqli_num_rows($result) == 1) { list($price) = mysqli_fetch_ array ($result, MYSQLI_NUM); $_SESSION['cart'][$pid] = array ('quantity => 1, 'price => $price); echo '<p>The print has been added to your shopping cart. </p>'; If the product is not currently in the cart, this else clause comes into play. Here, the print's price is retrieved from the database using the print ID. If the price is successfully retrieved, a new element is added to the $_SESSION['cart'] multidimensional array.
Since each element in the $_SESSION ['cart'] cart array is itself an array, I use the array() function to set the quantity and price. A simple message is then displayed (Figure 14.32).
Figure 14.32. The result after adding a new item to the shopping cart. 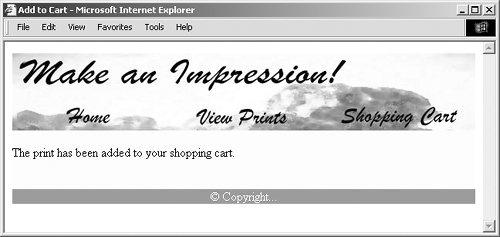
| 5. | Complete the conditionals.
} else { echo '<div align="center"> This page has been accessed in error!</div>'; } mysqli_close($dbc); } } else { echo '<div align="center"> This page has been accessed in error!</div>'; } The first else applies if no price could be retrieved from the database, meaning that the submitted print ID is invalid. The second else applies if no print ID is received by this page at all.
| 6. | Include the HTML footer and complete the PHP page.
include ('./includes/footer.html'); ?>
| 7. | Save the file as add_cart.php, upload to your Web server, and test in your Web browser (by clicking an Add to Cart link).
| Tips If you would rather display the contents of the cart after something's been added, you could combine the functionality of this script with that of view_cart.php, written next. Similarly, you could easily copy the technique used in view_print.php to this script so that it would display the details of the product just added.
Viewing the shopping cart The view_cart.php script will be more complicated than add_cart.php because it serves two purposes. First, it will display the contents of the cart in detail. Second, it will give the customer the option of updating the cart by changing the quantities of the items therein (or deleting an item by making its quantity 0). To fulfill both roles, I'll display the cart's contents as a form and have the page submit the form back to itself. Finally, this page will link to a checkout.php script, intended as the first step in the checkout process. To create view_cart.php 1. | Create a new PHP document in your text editor (Script 14.10).
<?php # Script 14.10 - view_cart.php $page_title = 'View Your Shopping Cart; include ('./includes/header.html'); Script 14.10. The view_cart.php script both displays the contents of the shopping cart and allows the user to update the cart's contents. 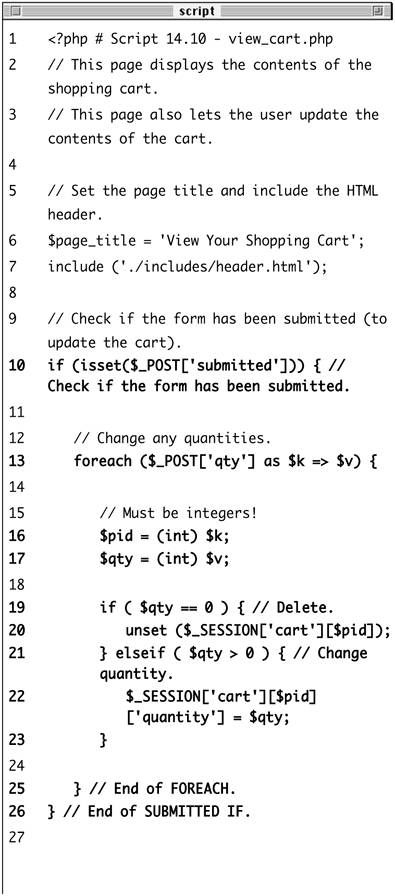 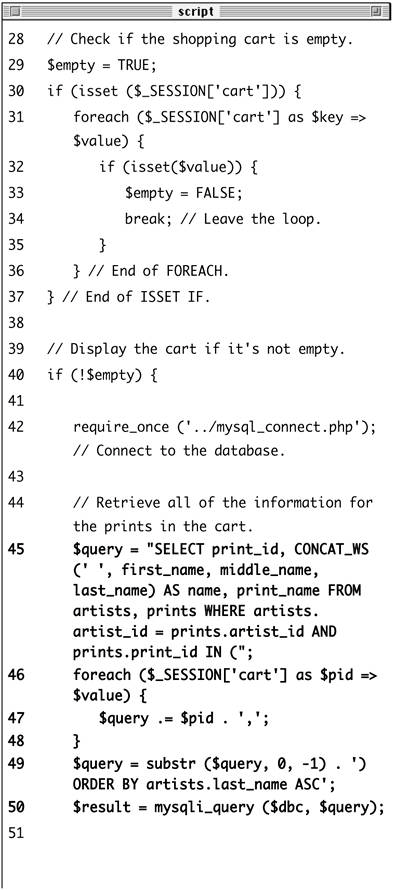 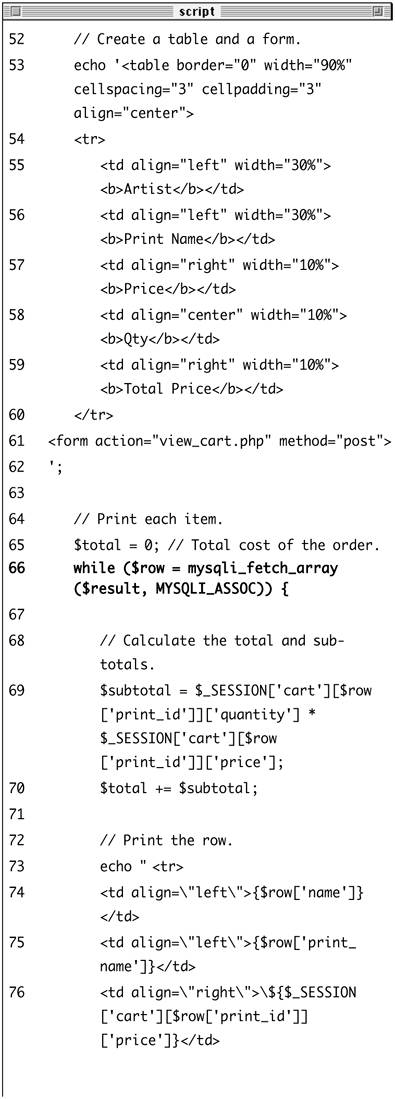 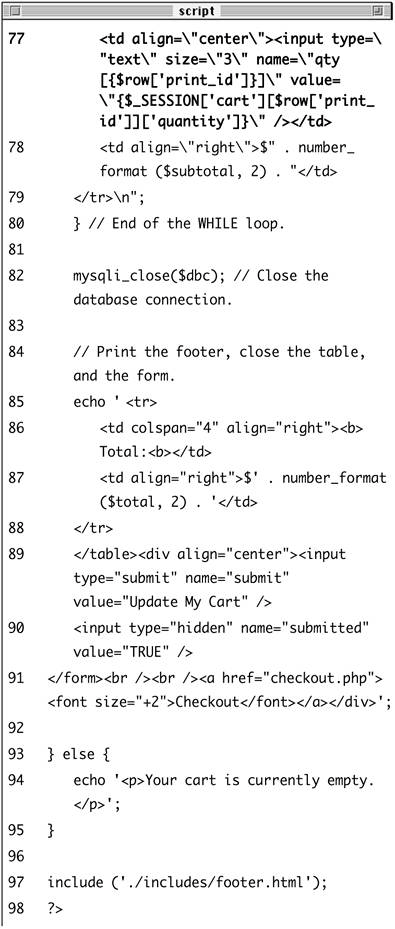
| 2. | Update the cart if the form has been submitted.
if (isset($_POST['submitted'])) { foreach ($_POST['qty'] as $k => $v) { $pid = (int) $k; $qty = (int) $v; if ( $qty == 0 ) { unset ($_SESSION['cart'] [$pid]); } elseif ( $qty > 0 ) { $_SESSION['cart'][$pid] ['quantity] = $qty; } } } If the form has been submitted, then the script needs to update the shopping cart to reflect the entered quantities. These quantities will come in as an array called $_POST['qty'] whose index is the print ID and whose value is the new quantity (see Figure 14.33 for the HTML source code of the form). If the new quantity is 0, then that item should be removed from the cart by unsetting it. If the new quantity is not 0 but is a positive number, then the cart is updated to reflect this.
Figure 14.33. The HTML source code of the view shopping cart form shows how the quantity fields reflect both the product ID and the quantity of that print in the cart. 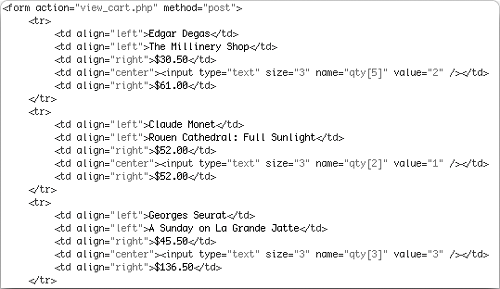 If the quantity is not a number greater than or equal to 0, then no change will be made to the cart. This will prevent a user from entering a negative number, creating a negative balance due, and getting a refund.
| 3. | Determine if the shopping cart is empty.
$empty = TRUE; if (isset ($_SESSION['cart'])) { foreach ($_SESSION['cart'] as $key => $value) { if (isset($value)) { $empty = FALSE; break; } } } Because the contents of the shopping cart may have just changed (if the form was submitted), I need to check that it's not empty before attempting to display it. To do so, I first check that the cart session variable is set (which means that at least one product has been added to it, even if that product has since been removed). Then I loop through the cart, testing each item for a quantity. If at least one item has a quantity, then the cart is not empty. To save the hassle of having to access every potential quantity, the first time that a quantity is found, the loop will be exited using break.
| 4. | If the cart is not empty, create the query to display its contents.
if (!$empty) { require_once ('../mysql_connect. php); $query = "SELECT print_id, CONCAT_WS(' ', first_name, middle_name, last_name) AS name, print_name FROM artists, prints WHERE artists.artist_id = prints.artist_id AND prints. print_id IN ("; foreach ($_SESSION['cart'] as $pid => $value) { $query .= $pid . ','; } $query = substr ($query, 0, -1) . ') ORDER BY artists.last_name ASC; $result = mysqli_query ($dbc, $query); The query is a join similar to one I've used many times in this chapter. One addition is the use of the IN SQL clause. Instead of just retrieving the information for one print (as in the view_print.php example), I'll want to retrieve all the information for every print in the shopping cart. To do so, I use a list of print IDs in a query like SELECT... print_id IN (519, 42, 427).... I could have also used SELECT... WHERE print_id=519 OR print_id=42 or print_id=427..., but that's unnecessarily long-winded.
| 5. | Create the table and begin the HTML form.
echo '<table border="0" width="90%" cellspacing="3 cellpadding="3" align="center> <tr> <td align="left" width="30%"> <b>Artist</b></td> <td align="left" width="30%"> <b>Print Name</b></td> <td align="right" width="10%"> <b>Price</b></td> <td align="center" width="10%"> <b>Qty</b></td> <td align="right" width="10%"> <b>Total Price</b></td> </tr> <form action="view_cart.php" method="post> ';
| 6. | Print out the returned records (Figure 14.34).
$total = 0; while ($row = mysqli_fetch_array ($result, MYSQLI_ASSOC)) { $subtotal = $_SESSION['cart'] [$row['print_id]]['quantity'] * $_SESSION['cart][$row ['print_id]]['price']; $total += $subtotal; echo " <tr> <td align=\"left\">{$row ['name]}</td> <td align=\"left\">{$row ['print_name]}</td> <td align=\"right\">\${$_SESSION ['cart][$row['print_id']] ['price]}</td> <td align=\"center\"><input type=\"text\" size=\"3\" name=\"qty[{$row['print_id]}]\" value=\"{$_SESSION['cart][$row ['print_id]]['quantity']}\" /></td> <td align=\"right\">$" . number_ format ($subtotal, 2) . "</td> </tr>\n"; } Figure 14.34. The shopping cart displayed as a form where the specific quantities can be changed. 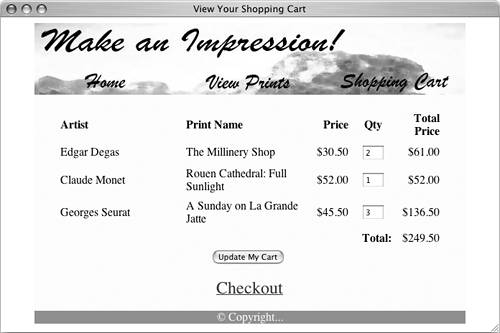 When displaying the cart, I will also want to calculate the order total, so I initialize a $total variable first. Then for each returned row (which represents one print), I multiply the price of that item times the quantity to determine the subtotal (the syntax of this is a bit complex because of the multidimensional $_SESSION['cart'] array). This subtotal is added to the $total variable.
Each record is also printed out as a row in the table, with the quantity displayed as a text input type whose value is preset (based upon the quantity value in the session).
| 7. | Close the database connection, then complete the table and the form.
mysqli_close($dbc); echo ' <tr> <td colspan="4" align="right"> <b>Total:<b></td> <td align="right">$' . number_ format ($total, 2) . '</td> </tr> </table><div align="center"><input type="submit name="submit" value="Update My Cart /> <input type="hidden" name= "submitted value="TRUE" /> </form><br /><br /><a href= "checkout.php><font size="+2"> Checkout</font></a></div>'; The running order total is displayed in the final row of the table, using the number_format() function for formatting.
| 8. | Finish the main conditional and the PHP page.
} else { echo '<p>Your cart is currently empty.</p>'; } include ('./includes/footer.html'); ?>
| 9. | Save the file as view_cart.php, upload to your Web server, and test in your Web browser (Figures 14.35 and 14.36).
Figure 14.35. If I make changes to any quantities and click Update My Cart, the shopping cart and order total are updated (compare with Figure 14.34). 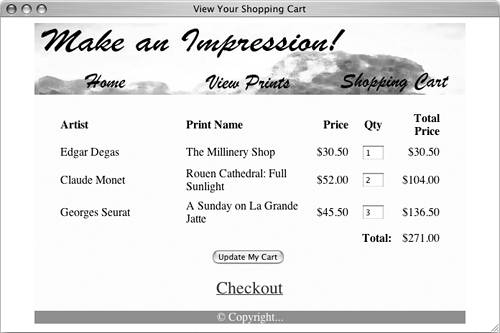 Figure 14.36. I removed everything in the shopping cart by setting the quantities to 0. 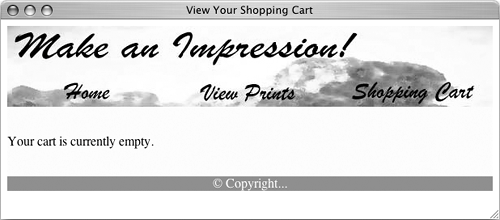
You can remove an item from the cart by setting its quantity to 0 (a note should be added to the page so that the customer knows this).
| Tips On more complex Web applications, I would be inclined to write a function strictly for the purpose of displaying a cart's contents (since several pages might do so). The key to a secure e-commerce application is to watch how data is being sent and used. For example, it would be far less secure to place a product's price in the URL where it could easily be changed.
|