In this task, you will build a new class for items that are added to the shopping cart. The new class will need to keep track of which product was added and the quantity of product; you will build a method that calculates the subtotal for that item. You will also build the skeleton for a ShoppingCart class that will handle all the logic for the shopping cart, including adding items to the cart. 1. | Create a new ActionScript class file by choosing File > New > ActionScript class. Set the Package to valueObjects, which will automatically add this class to the folder you created earlier. Enter ShoppingCartItem as the name and leave all other fields with the following default values:
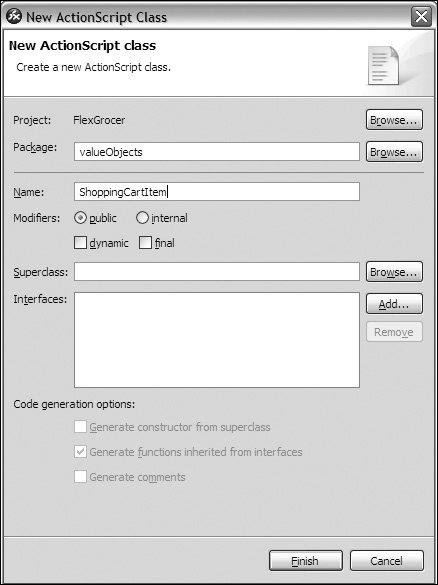 In this class, you will calculate the quantity of each unique item as well as the subtotal.
| | | 2. | Within the class definition, define a public property with the name of product and a data type of Product, as shown:
package valueObjects { public class ShoppingCartItem { public var product:Product; } } An important piece of the shopping cart is which item has been added. You have already created the Product class to track all this data, so it makes perfect sense to attach an instance of this class to the ShoppingCartItem class.
| 3. | Define a public property with the name of quantity and a data type of uint, as shown:
package valueObjects { public class ShoppingCartItem { public var product:Product; public var quantity:uint; } } The uint data type means unsigned integer, which is a non-fractional, non-negative number (0, 1, 2, 3, . . .). The quantity of an item added to the shopping cart will either be zero or a positive number so it makes sense to type it as a uint.
| 4. | Define a public property with the name of subtotal and a data type of Number, as shown:
package valueObjects { public class ShoppingCartItem { public var product:Product; public var quantity:uint; public var subtotal:Number; } } Each time a user adds an item to the shopping cart, you will want the subtotal for that item to be updated. Eventually, you will display this data in a visual control.
| | | 5. | Immediately after the subtotal property, define the constructor function of the class and specify the parameters that will be passed to this function.
The parameters should match the data type of the properties you already defined, and the names should match but begin with the this keyword to avoid name collision. Set the quantity parameter in the function to 1, which is creating a default. Set the subtotal property to equal the product.listPrice * quantity. Be sure that the name of the function matches the class name and that the constructor is public. Your constructor function should look as follows:
public function ShoppingCartItem(product:Product, quantity:uint=1){ this.product = product; this.quantity = quantity; this.subtotal = product.listPrice * quantity; } The constructor function is automatically called every time an object is created from a class. The constructor will set the properties that are passed in; in this case, an instance of the Product class, and the quantity which is automatically set to 1 as a default. This method will only be used when an item is added to the shopping cart so it makes sense to define the initial quantity as 1.
In this code, we have a variable in the ShoppingCartItem class called product, and we have an argument of the constructor called product, so we need to tell the compiler specifically which one to use. We use the this keyword, to accomplish this goal and avoid something called name collision, when the same name could refer to two separate variables. The line 'this.product = product;' tells the compiler to set the product variable that resides in the ShoppingCartItem equal to the product variable that was passed in as an argument of the constructor. You used a different method, underscores, in the product class to illustrate the same concept. It is not important which strategy you choose to use, just that you are specific so that the compiler understands which variable you intend to modify.
| 6. | Create a public method with the name of recalc() that will calculate the subtotal of each item by multiplying the listPrice of the product by the quantity, as follows:
public function recalc():void{ this.subtotal = product.listPrice * quantity; } When the user adds items to the shopping cart, you need to perform calculations so that the total can be updated. You also need to check to see whether the item has been added to the cart already; if so, update the quantity. You will learn how to loop through a data structure in the next lesson.
| | | 7. | Create a new ActionScript class file by choosing File > New > ActionScript class. Set the Package to valueObjects, which will automatically add this class to the valueObjects folder you created earlier. Name the class ShoppingCart and leave all other fields with the defaults, as shown in the following example:
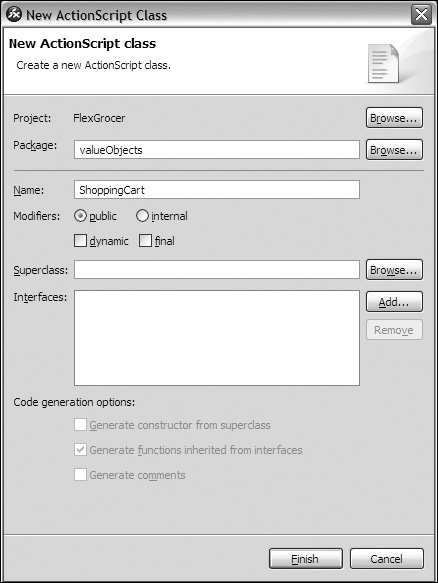 You are creating a new class that will handle the manipulation of the data in the shopping cart. You have already created the visual look and feel of the shopping cart and you will place all your business logic in the ShoppingCart class. This business logic includes adding an item to the cart, deleting an item from the cart, updating an item in the cart, and so on.
| 8. | Add an import statement that will allow you to use Flash utilities such as the trace statement within the class, as shown:
package valueObjects{ import flash.utils.* public class ShoppingCart{ } } Just as you have to import your own custom classes for use, you need to import the appropriate classes from the framework to use them. You will be using a trace() function and other utilities, which require the import of these classes.
| | | 9. | Create the skeleton of an addItem() method that will accept an instance of the ShoppingCartItem class. Add a trace statement that will trace the product added to the cart:
package valueObjects{ import flash.utils.* public class ShoppingCart{ public function addItem(item:ShoppingCartItem):void{ trace(item.product); } } } This is the method in which you will add a new item to the shopping cart. You will add much more business logic to this method in later lessons. For now, you will just trace the name of the item added to the cart. Remember that the toString() function you wrote earlier is automatically called whenever an instance of the Product class is traced.
| 10. | Open the EComm.mxml in Flex Builder and locate the script block. Just below the Product import statement, import the ShoppingCartItem and ShoppingCart classes from the valueObjects folder, as shown:
import valueObjects.ShoppingCartItem; import valueObjects.ShoppingCart; To use a class, Flex Builder needs an import statement that references the location or package in which the class is located.
| 11. | Just below the import statements, instantiate a public instance of the ShoppingCart class, name the instance cart, and add a [Bindable] metadata tag, as follows:
[Bindable] public var cart:ShoppingCart = new ShoppingCart(); When the user clicks the Add To Cart button, you want to call the addItem() method of the ShoppingCart class you created earlier. You will pass the addItem() method an instance of the ShoppingCartItem class. By instantiating the class here, you will ensure that you have access to it throughout the class.
| | | 12. | Locate the prodHandler() method in the <mx:Script> block. Immediately after this method, add a skeleton of a new private method with the name of addToCart(). Be sure that the method accepts a parameter of product data typed to Product, as shown:
private function addToCart(product:Product):void { } This method will be called when the user clicks Add, and you will pass the Product value object that the user selected. This is a method of the MXML file and will not be called outside of this file. Therefore, you can use the identifier private, which means that the method cannot be accessed from outside the class and helps to provide better data protection.
| 13. | Inside the addToCart() method, create a new instance of the ShoppingCartItem class with the name of sci and pass the constructor the Product class, as shown:
var sci:ShoppingCartItem = new ShoppingCartItem(product); | 14. | Inside the addToCart() method, call the addItem() method of the ShoppingCart class. Be sure to pass the method the sci (Instance of the ShoppingCartItem class), as follows:
cart.addItem(sci); This code will call the addItem() method of the ShoppingCart class you built earlier. In this next lesson, you will learn how to loop through the data structure to see whether the item is added. For now, this method simply traces the name of the product added to the cart.
| 15. | Add a click event to the Add To Cart button. Call the addToCart() method, passing the method an instance of theProduct:
<mx:Button label="Add To Cart" click="addToCart(theProduct)"/> The addToCart() method will create an instance of the ShoppingCartItem and pass it to the addItem() method of the cart class.
| 16. | Save and debug the application.
You should see that the words [Product]Milk appear in the output panel every time you click Add To Cart.
| |