Now that you've created a data-storage architecture, you need to develop a way to retrieve the information from storage and that involves some new syntax that provides a means of writing a dot syntax path dynamically. Square brackets are used to evaluate an expression. Here's how it works. Assume we have three variables that contain animal sounds: var dog:String = "bark"; var cat:String = "meow"; var duck:String = "quack"; We'll create a variable and assign it a text value of "dog", "cat", or "duck" (note that these text values have the same names as the variables we just mentioned). We'll start with "dog": var currentAnimal:String = "dog"; Using this syntax, we can access the value of the variable named dog: var animalSound = this[currentAnimal]; Here, animalSound is assigned a value of "bark". The expression to the right of the equals sign looks at currentAnimal and sees that it currently contains a string value of "dog". The brackets surrounding currentAnimal tell ActionScript to treat this value as a variable name. Flash sees this line of script like this: var animalSound:String = this.dog; Because dog has a value of "bark", that's the value assigned to animalSound. If we were to change the value of currentAnimal to "cat," animalSound would be assigned a value of "meow". Note that you must use this in the expression to include the target path of the variable used to set the dynamic variable name (in this case currentAnimal), because it relates to the variable to the left of the equals sign (in our case animalSound). Using this denotes that these two variables are on the same timeline. If animalSound existed in a movie clip instance's timeline while currentAnimal was on the root (main) timeline, the syntax would look like this: var animalSound:String = _root[currentAnimal]; Here are a couple of other examples: var animalSound:String = _parent[currentAnimal]; var animalSound:String = myMovieClip[currentAnimal]; This syntax is critical to the way you retrieve information from the objects in this exercise. Remember that all the objects are built with the same array names and structure; they have different parent object names (monday and tuesday). We can use the aforementioned syntax to dynamically access the data in these objects based on the current value of a variable. With newsFlash4.fla open, select Frame 1 of the Actions layer. Open the Actions panel and enter these variables at the end of the current script: var day:String = "monday"; var section:String = "entertainment"; 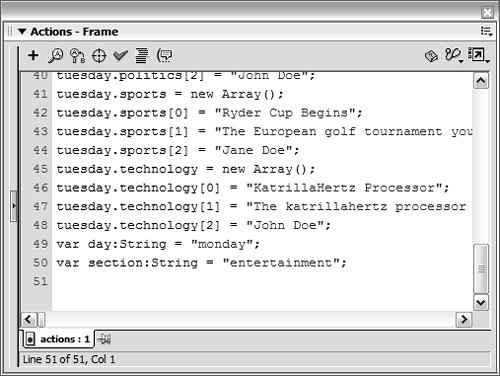 When the application plays for the first time, we want Monday to appear initially, and entertainment news to be displayed. The two variables set in Step 1 allow us to accomplish this you'll see how in the next few steps.
NOTE These variables are known as initializing variables. Because the information our application displays depends on the buttons the user clicks, these variables provide some starting settings prior to user input. Select the frame labeled Sit in the Actions layer. Enter this script in that frame: function refresh(category) { section = category; } This function is called when any of the section buttons is clicked. The button events that call this function will be added later in this exercise. The purpose of this function (by the end of this exercise) is to refresh what is shown on the screen based on the current values of the day and section variables (which were initially set to "monday" and "entertainment", respectively, in Step 1 of this exercise). Currently there is only one line of ActionScript inside this function definition. It updates the value of the section variable to that of the parameter value passed to the function. If the Entertainment button is clicked, it will call this function and pass it a value of "entertainment". The function will then assign the section variable a value of "entertainment". We'll explain the purpose over the next several steps as we add more actions to this refresh() function.
Add this script at the end of the refresh() function: date_txt.text = this[day].date; This script uses the syntax we introduced at the beginning of this exercise. The text displayed in the date_txt field is set by dynamically referencing another variable. Because day initially has a value of "monday" (as set in Step 1), ActionScript sees the code as this: date_txt.text = monday.date; You'll remember that monday.date contains the text value of "Monday, August 25 2003" and date_txt is the name of the text field in the bottom-left portion of the screen. As a result of this action, "Monday, August 25 2003" will be displayed in the date_txt text field. You can begin to see how dynamically named variables can be useful. If the variable day had a value of "tuesday", this line of code would reference the date variable in the object called tuesday.
With the current frame still selected, add this script to the end of the refresh() function: days_mc.gotoAndStop(day); icon_mc.gotoAndStop(this[day].weather[0]); weatherBlurb_txt.text = this[day].weather[1]; 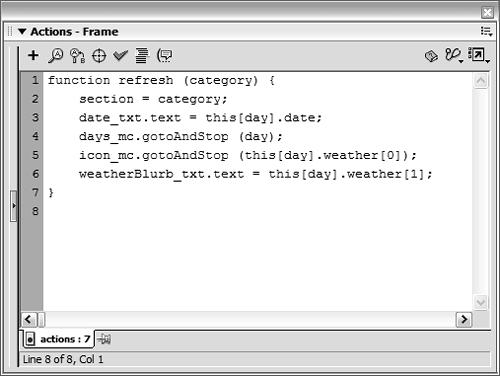 These three actions depend on the current value of day (which we set to an initial value of "monday" in Step 1) when they are executed. Here's how. The movie clip instance named days_mc in the bottom-right portion of the stage contains five buttons (M, T, W, T, and F, with instance names of monday_btn through friday_btn) that will enable the user to select the day's news that he or she wishes to see. This movie clip also contains five frame labels (Monday, Tuesday, and so on), one for each day of the business week. Each of the frame labels displays a different day in yellow. The first line of the script tells the days_mc movie clip instance to go to the appropriate frame, based on the current value of day. This cues the user to which day's news he or she is viewing. Because day has an initial value of "monday", that day will initially appear in yellow. The weather icon (instance name icon_mc) contains three frame labels, one for each weather type (Sunny, Stormy, and Rainy). Remember that the zero element of the weather array in the monday and tuesday objects contains one of these three values. The second line of the script dynamically pulls that value from the weather array using the correct object and sends the icon_mc movie clip instance to the correct frame. Flash sees : icon_mc.gotoAndStop(this[day].weather[0]); as icon_mc.gotoAndStop(this.monday.weather[0]); Let's take it a step further. Consider that monday.weather[0] has a value of "Rainy": icon_mc.gotoAndStop("rainy"); In the same way, the last action will populate the weatherBlurb_txt text field with the value of monday.weather[1], which is "Very wet". Keep in mind that if day had a value of "tuesday", these actions would be executed based on the respective values in the tuesday object.
Add this script to end of the refresh() function: high_txt.text = this[day].weather[2]; low_txt.text = this[day].weather[3]; Using the same syntax as in the preceding step, the high_txt and low_txt text fields are populated by referencing the second and third elements of the weather array dynamically.
Add these actions to the refresh() function: headline_txt.text = this[day][section][0]; article_txt.text = this[day][section][1]; author_txt.text = this[day][section][2]; The dynamic referencing performed in this step is one level deeper into the storage objects than the dynamic referencing was in Step 5. We're trying to dynamically pull information about a news article from an object and an array because the day can be either Monday or Tuesday and the section can be Entertainment, Politics, Sports, or Technology. The initialization variables you set in Step 1 of this exercise set section = "entertainment". Using the values of our initialization variables, Flash will read the three lines of ActionScript like this when the refresh() function is executed: headline_txt.text = this.monday.entertainment[0]; article_txt.text = this.monday.entertainment[1]; author_txt.text = this.monday.entertainment[2]; The only text field instances affected by the current section variable are headline_txt, article_txt, and author_txt.
Add this function call to the end of the frame: refresh(section); 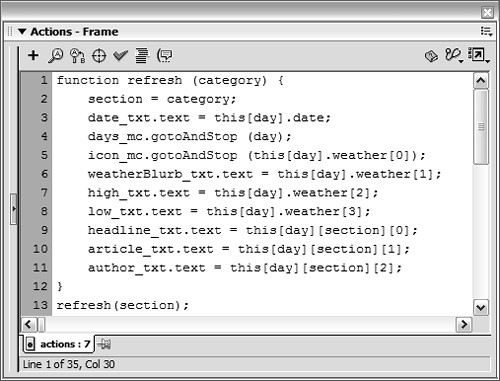 This script calls the function we created and passes it the current value of the section variable, to which we gave an initial value of "entertainment". As a result, the function will cause our project to initially display Monday's entertainment news. Although the news displayed in our project will be highly dependent on choices the user makes by pressing buttons (which we will script momentarily), this function call has an initializing effect: it determines what is displayed before the user makes the first choice.
Enter a stop() action after the refresh() function. When you test the movie, you don't want the entire movie to loop repeatedly. Instead, the movie will initialize the storage variables on Frame 1 and move to the Sit label and populate the text fields on the screen. The stop() action keeps the movie from playing past the Sit frame.
Choose Control > Test Movie. Your on-screen text fields should be populated with information pertaining to Monday entertainment. The weather icon movie clip should display the correct icon, and the days_mc movie clip instance (on the bottom-right) should have the M (for Monday) highlighted.
Close the test movie to return to the authoring environment. Add this script to the bottom of the frame you have been working with: entertainment_btn.onRelease = function() { refresh("entertainment"); }; This scripts the Entertainment button so that when it is released, the refresh() function is called and the string "entertainment" is passed as the section name. This changes the display to an entertainment-related article if one isn't already showing.
Add these button event handlers for the remaining three news section buttons: sports_btn.onRelease = function() { refresh("sports"); }; politics_btn.onRelease = function() { refresh("politics"); }; technology_btn.onRelease = function() { refresh("technology"); }; 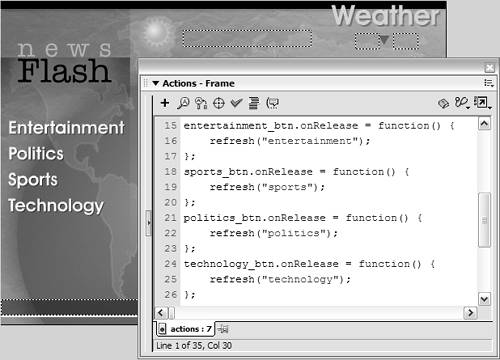 This script does the same thing the ActionScript attached to the Entertainment button does the only difference is in the string value passed into the refresh() function. The Politics button passes in "politics", the Sports button passes in "sports", and the Technology button passes in "technology".
Choose Control > Test Movie. Click the four category buttons to see the headlines, articles, and authors change. The information should change very easily now. It would be easy to add more sections of news stories using this object-oriented technique.
Close the test movie to return to the authoring environment. Select the frame we've been adding script to and add these button event handlers for the buttons found in the days_mc movie clip instance: days_mc.monday_btn.onRelease = function() { day = "monday"; refresh("entertainment"); }; days_mc.tuesday_btn.onRelease = function() { day = "tuesday"; refresh("entertainment"); }; 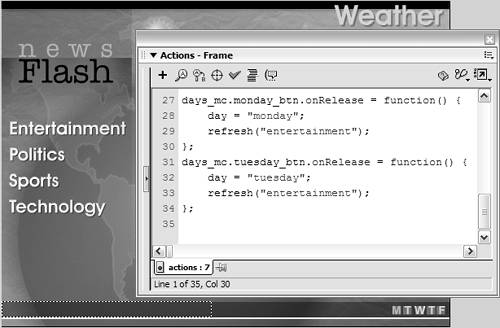 Earlier in this exercise, we mentioned that the days_mc movie clip contains five buttons for the five business days of the week. They are named monday_btn through friday_btn. In this step we add event handlers for the onRelease event of the monday_btn and tuesday_btn buttons. When the monday_btn button is released, it changes the day variable to "monday" and calls the refresh() function, passing "entertainment". This action changes the current day to "monday" and the current news section to "entertainment". Likewise, when the tuesday_btn is released, the day variable to changed to "tuesday" and the news section is changed to "entertainment".
Choose Control > Test Movie. Click the M and T on the bottom-right of the screen to change the display. Notice that the weather and date change. The way we've coded this makes it easy to add day objects without changing the information retrieval code.
Close the test movie and save your work as newsFlash5.fla. You have completed a project in which displayed information is retrieved dynamically from a logical storage structure. The next logical step in a project like this would be to put all of our project's data into a database or text file. The information would then be grabbed and routed into the storage structure you created. We'll cover loading information in and out of Flash in Lesson 11, "Getting Data In and Out of Flash," and Lesson 12, "Using XML with Flash."
|