While the objects we've created thus far contain property values that can be evaluated and used in various ways, they cannot perform tasks. To understand what this means, let's take a look at some of the things that Flash's built-in objects can do: Movie clip instances can play() , stop() , loadMovie() , getURL() , and so forth. Sound object instances can loadSound() , setVolume() , setPan() , and so forth. In contrast, our custom Person and Doctor classes don't do anything besides just sit there. We'll change that in this section by creating methods for our custom classes enabling instances of those classes to perform useful tasks. The first thing you need to be aware of is that a method is nothing more than a function that has been assigned to a class by placing it on that class' prototype object. To see how this works, let's begin an exercise. -
Open class3.fla in the Lesson06/Assets folder. With the Actions panel open, select Frame1. Remove the five trace actions at the end of the script, then add the following: Person.prototype.talk = function(){ trace("Hello, my name is " + this.name + ". I am " + this.age + " years old."); } NOTE Alternate syntax for creating this method would be: //Define a function first function soonToBeMethod(){ trace("Hello, my name is " + this.name + ". I am " + this.age + " years old."); } //Set the function as the talk method of the Person class Person.prototype.talk = soonToBeMethod; This creates a new method for the Person class of objects. We've made the function a method of the Person class by placing it on the Person class' prototype object. 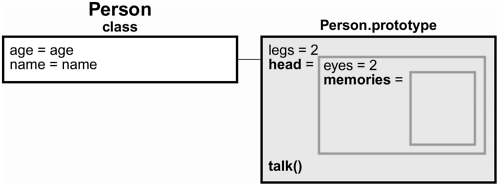 This custom method, named talk() , can be used by any instance of that class. In our case, this would be person1 or person2 . Thus, either one of these instances can use this method in the following manner: person2.talk(); This method does just one thing: It outputs a message to the Output window when the movie is tested. You can see that the trace action in the method definition uses the terms this.name and this.age . When a function is a method, this is a reference to the instance that calls the method. Thus, if person2 calls this method, these could be replaced to read person2.name and person2.age . NOTE When the term this is used in a regular function, it refers to the timeline that contains the function definition. -
Place the following line of script at the end of the current script: person2.talk(); This will cause person2 to invoke the talk() method. -
Choose Control > Test Movie to test the project up to this point. The Output window will open and display the following: Hello, my name is Derek. I am 29 years old. While the talk() method has enabled instances of our Person class to actually do something, we can customize it a bit further. -
Close the test movie to return to the authoring environment. With the Actions panel open, select Frame 1 and update the talk() method as follows: Person.prototype.talk = function(tone){ var message = "Hello, my name is " + this.name + ". I am " + this.age + " years old."; if (tone == "scream"){ trace(message.toUpperCase()); }else{ trace(message); } } You'll notice that the method now accepts a parameter named tone . The method will operate in one of two ways based on the value of this passed parameter. Let's look at the method's updated definition. First, a local variable named message is created and given an expression as its value. This expression is the same one that was used inside the trace actions before. NOTE As mentioned in the previous lesson on functions, a local variable is a variable that only has meaning within the function definition where it exists. If we hadn't made message a local variable, its value would be accessible by scripts anywhere. As a local variable, it will get deleted as soon as the method finishes executing. After you've set the message variable's value, you'll notice an if statement. This statement says that if the value of tone equals "scream", convert the value of message to all uppercase characters and then display it in the Output window. If tone has any other value, message will be output normally. The use of the String object method toUppercase() demonstrates how a method from a different class can be used to define the functionality of other methods. Custom methods can contain actions, methods, and so on practically anything ActionScript offers to facilitate interactivity. -
Add the following line of script to the end of the current script: person2.talk("scream"); This will cause person2 to invoke the talk() method, while passing it a value of "scream". Since the last two lines of script both invoke the talk() method (in different ways; one passes it the value "scream"), the Output window will display the results of both. -
Choose Control > Test Movie to test the project up to this point. The Output window will open and display the following: Hello, my name is Derek. I am 29 years old. HELLO, MY NAME IS DEREK. I AM 29 YEARS OLD. Let's look at one more interesting aspect of our talk() method. -
Close the test movie to return to the authoring environment. With the Actions panel open, select Frame 1 and update the talk() method calls (last two lines currently on Frame 1) as follows: frankenstein.talk(); frankenstein.talk("scream"); 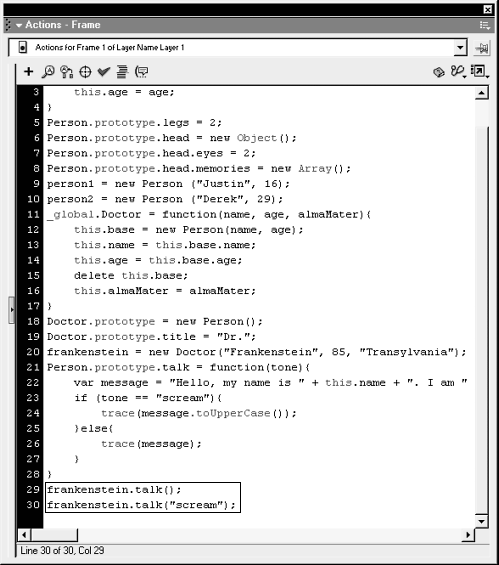 The talk() method is actually a method of the Person class, but since the Doctor class is a subclass of the Person class, Doctor class instances can access this method as well which is why the frankenstein instance can use it as shown. The Doctor class can also have methods unique to it: You would simply place functions on the Doctor.prototype object, as demonstrated with the Person.prototype object in Step 1. NOTE If we created a talk() method on the Doctor.prototype object that had different functionality from the one on the Person.prototype object, then the frankenstein instance would use that method first, before accessing the talk() method on the Person.prototype object. This is because frankenstein , as an instance of the Doctor class first and foremost, will always look first at the Doctor.prototype object for its functionality. -
Choose Control > Test Movie to test the project up to this point. The Output window will open and display the following: Hello, my name is Frankenstein. I am 85 years old. HELLO, MY NAME IS FRANKENSTEIN. I AM 85 YEARS OLD. While our talk() method performs a simple task, custom methods can get quite complex. -
Close the test movie to return to the authoring environment. Save your work as class4.fla. The knowledge you've gained about object mechanics will prove useful in the sections to follow, as you learn how to extend Flash's prebuilt objects. Although you've seen how objects can be used to organize and work with data, Flash is primarily a visual medium which means you're probably wondering how objects can help in that realm. You're now ready to make that journey. |