Up to now, we've been using an instance of the generic Object object to demonstrate the way our person1 object works. Now it's time to move to the next level of object mechanics: Creating a custom class which in this exercise we'll define as the Person class. This class will serve as the blueprint for creating new instances of people, representing a more efficient way of creating and defining person1 . NOTE We used the Object object in the above exercises primarily to illustrate the concept of objects. The Object object has many viable uses in regular projects; however, it's a general solution and one that may not be suitable for every application. Like the previous exercises, this one makes liberal use of the trace action to teach concepts. -
Open Flash and choose File > New to start a new authoring file. With the Actions panel open, select Frame 1 and add this script: Person = function(){ } Although this closely resembles a function definition (it's a constructor function), it is also the beginning of a class definition. NOTE The terms constructor function and class definition are used interchangeably in the following discussion. Regular function definitions and constructor functions (class definitions) employ similar syntaxes; however, they're accessed differently. We'll explain this in greater detail in a minute, but for now take a look at the following examples. In the first, the function is executed as a function; in the second, the function is executed to create an instance of the Person class: Person(); //executes as function person1 = new Person();//creates an instance of the person class NOTE Although our class definition looks like a function, we need to approach it strictly as a class definition. It's not practical to use a single block of code both ways. You should think of a class definition as a blueprint, or template, for creating instances of that class. Within the curly braces of the class definition you define the characteristics that make up that class of objects. Since our Person class does not yet have any characteristics (properties), we'll update its class definition in accordance with our previous example. -
Update the Person class definition as follows: Person = function(){ this.name = "Justin"; this.age = 16; this.legs = 2; this.head = new Object() this.head.eyes = 2; this.head.memories = new Array(); } Our class definition is now complete. In the next couple of steps, we'll discuss how to use it and explain what this means in the context of a class definition. We'll also show you how to create instances of our new class. TIP In case you hadn't noticed, we've given the Person class definition a name that starts with an uppercase letter. It's common practice to begin class names in this manner. In contrast, instances of the class are usually given names beginning in a lowercase letter. By employing this simple trick, you can make your script easier to read and understand. -
Add the following line of script at the end of the current script: person1 = new Person(); This code creates an instance of the Person object named person1 . 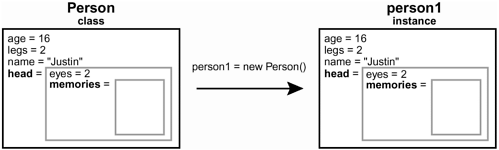 -
Choose Control > Test Movie to test the project up to this point. When the testing environment appears, choose Debug > List Variables. The Output window will open up, and the following script will appear in the information it displays: Variable _level0.person1 = [object #2] { name:"Justin", age:16, legs:2, head:[object #3, class 'Object'] { eyes:2, memories:[object #4, class 'Array'] [] } } This is information about the person1 instance that is created as a result of the action you added in the previous step. As our Person class defines, the person1 instance has a name of "Justin", an age of 16, two legs, and so on. It's important to understand how person1 = new Person(); creates an instance with the properties defined by Person class. It's actually quite simple: When the line above is executed, ActionScript knows that it's time to build a new instance of the Person class. When this occurs, a temporary object (an Activation object) is created and sent to the Person class definition that was scripted earlier (all of which happens invisibly and automatically). The Activation object, which is pretty formless initially, is then "passed through" the class definition where various class properties (name , age , legs , and so on) are attached to it. During this process, the temporary Activation object is referenced by the term this , as shown in the class definition. Thus, when the class definition contains the following line: this.age = 16; It's saying that when an Activation object passes through here, its age property should be set to a value of 16. This process of creating an instance can be likened to moving the Activation object through an assembly line, adding parts to it as it moves along. When the activation object has completed the construction process, it is given a name (in this case person1 ) and becomes available for use. 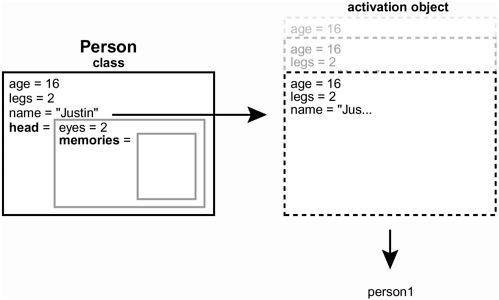 Although we've defined our Person class and know how to create instances of it, each of those instances will be pretty much identical. This is because the way our Person class is currently defined, every instance created has a name of "Justin", an age of 16, and so on. Since our Person class needs to do more than create clones, let's make some changes to its class definition so that we can create instances with different property values. -
Close the test movie to return to the authoring environment. With the Actions panel open, select Frame 1 and update the Person class definition as follows: Person = function(name, age){ this.name = name; this.age = age; this.legs = 2; this.head = new Object() this.head.eyes = 2; this.head.memories = new Array(); } Just like a regular function definition, our Person class definition now accepts two arguments: name and age . You'll notice that those same two values are used in the first two lines of the class definition. This enables us to create instances of the Person class that have unique property values. -
Update the line of script we added in Step 3 to read as shown below (in the first line), and then add the second line shown here below that: person1 = new Person ("Justin", 16); person2 = new Person ("Derek", 29); These two actions will create two instances of the Person class. These instances have different name and age values now that the Person class definition has been updated to allow it. We can pass strings, numbers, and even object names to create and set the properties of a new instance. Because we want to be able to create instances of the Person class easily, from any timeline (without having to enter a target path, just like Flash's built-in objects), we need to make our class definition global. -
Update the Person class definition as follows: _global.Person = function(name, age){ this.name = name; this.age = age; this.legs = 2; this.head = new Object() this.head.eyes = 2; this.head.memories = new Array(); } Notice the addition of _global to the first line of the script: This enables an instance of the Person class to be created from any timeline simply by using the following: new Person(); If we had not added _global and the class definition was defined on, say, the root timeline, you would need to use the following syntax: new _root.Person(); NOTE Even if you use _global to make a class definition accessible to all timelines without using a target path (as just described), individual instances will still exist in the timeline containing the script that created them. Thus, if you create an instance of the Person class named myBuddy and the timeline containing the script for creating that instance exists at _root.myMovieClip , the absolute target path to myBuddy will be _root.myMovieClip.myBuddy . Of course, you can make instances global, if you wish, by using the following syntax when creating them: _global.myBuddy = new Person("Dennis", 27); . -
Save your work as class1.fla. We'll continue to use this file in the following exercise Although we can now create instances of our custom Person class, we still need to do one thing to fine-tune it and that requires an understanding of the scary-sounding but powerful prototype object. |