Before you use a function, you must create or define it. You can do this by using one of two possible syntaxes. Syntax 1 The following code describes the first syntax: function myFunction (parameter1,parameter2,etc.) { //actions go here; } The above code represents the most common way of creating a function as well as the syntax we'll use most frequently in this lesson. You'll see that the function declaration begins with the word function, followed by the name of the function (which can be anything you choose, as long as it follows typical naming conventions). Following the function name is an area enclosed by parenthesis. You can leave this area blank, or you can fill it with information (parameter data) that the function can use. By leaving the area within the parenthesis blank, you create a "generic" function that is, one that performs the same way whenever it's called. If, however, your function contains parameters, it will perform in a unique way each time it's called based on the parameter information it receives. Giving the function information in this way is called passing in arguments or passing in parameters. You can use as many parameters as you wish; we'll tell you how to access them a bit later in this lesson. Following the optional parameter area is an open curly brace followed by a carriage return and then some actions before the curly brace that concludes the function. In this space between the curly braces, you write the actions that you wish your function to perform. These actions may also make use of any parameter information passed to the function (as you will see soon). TIP You can create a function skeleton (that is, a function that does not yet contain any actions) by clicking Actions > User-Defined Functions in the Actions panel. 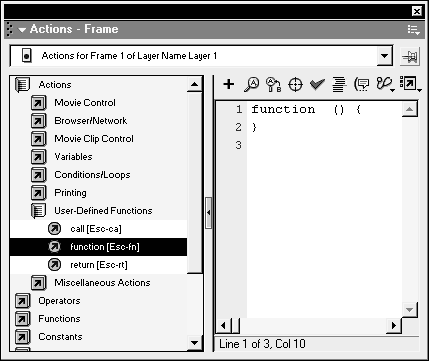 Syntax 2 The following code represents the second syntax for creating functions: myFunction = function (parameter1,parameter2,etc.) {/* actions go here */ }; You would use the above syntax to create a function dynamically or to define your own custom method of an object (which we'll discuss in Lesson 6, Customizing Objects). The only difference between this syntax and Syntax 1 is in the way the function name is assigned: The function name appears first, and the function value is assigned using the "=" assignment operator. You can only use this syntax by manually typing it into the Actions list window with the Actions panel in Expert mode. If a function contains no parameters, it can be called using the following syntax: myFunction(); When you call a function, you're telling Flash to execute all of the actions within that function. Thus, if myFunction() contained 20 actions, all of them could be executed by using this single line of script. If a function has been defined to accept parameter information, you can use the following syntax to call it: myFunction(parameter1, parameter2); The examples above assume that the function and function call reside on the same timeline. Just as each timeline contains its own variables and objects, each timeline also contains any functions you've defined there. To call a function on a specific timeline, you need to place the target path to that timeline in front of the function call, like this: _root.clip1.clip2.myFunction(); In this exercise, you'll create a Power button on a television remote control. With this button, the Flash-television can be toggled on and off using a function. TIP A function can be called dynamically based on a value (for instance, _root[aVariableName](); ) . Thus, if aVariableName had a value of "sayHello", the function call would actually look like _root.sayHello(); . -
Open television1.fla in the Lesson05/Assets folder. The movie's structure has already been created. The Actions layer is where you will include most of the ActionScript. Inside the TV layer is a movie clip instance named tv, which contains three layers on its timeline: The bottom layer (called Television) is just a graphic; the layer above that (called Screen) contains a movie clip instance called screen, which itself includes two layers and two frames, and contains graphical content that represents the various "programs" that will be seen when changing channels on the TV. On the main timeline there is another layer, named Remote, that contains a movie clip instance named remote. Inside remote, you'll find a layer that contains most of the remote-control graphics, including a movie clip with an instance name of light, as well as another layer that contains the buttons for the remote, all of which will eventually contain scripts. -
Select Frame 1 of the Actions layer on the main timeline. With the Actions panel open, add the following ActionScript: tvPower = false; function togglePower () { if (tvPower) { newChannel = 0; tvPower = false; } else { tvPower = true; newChannel = 1; } tv.screen.gotoAndStop(newChannel + 1); remote.light.play(); } 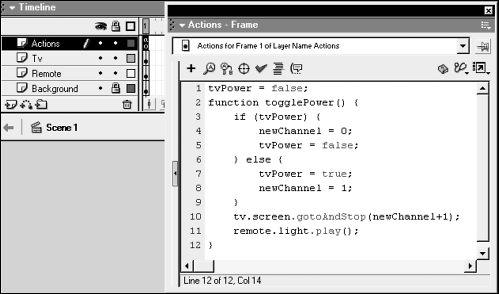 The first line of this script creates a variable named tvPower , which is used to track the current state of the TV: A value of true means the television is on; false means the television is off. The television will appear off initially, thus tvPower is set to false . The next seven lines represent a function definition for togglePower(): When called, this function will toggle the television power on and off. No parameters are passed into this function. Since this script exists on Frame 1, our togglePower() function is defined and a variable called tvPower is set to false as soon as the frame is loaded (that is, when the movie begins to play). TIP Because functions must be defined before they can be called, it's common practice to define all functions on Frame 1 so that they can be called at any time after that. The first part of the function uses an if statement to analyze the current value of tvPower . If tvPower is true (TV is on) when the function is called, the actions in the function change it to false (off) and set the value of the newChannel variable to 1; otherwise (else ), tvPower is set to true and newChannel to 0. Using the if statement in this manner causes the value of tvPower to be set to its opposite each time the function is called, thus toggling the value of newChannel . By the time this statement is finished, newChannel has a value of 0 or 1. The function then sends the screen movie clip instance (which is inside the tv movie clip instance) to a frame based on the current value of newChannel + 1 . You must add 1 to the value of newChannel to prevent the timeline from being sent to Frame 0 (since newChannel will sometimes contain a value of 0, and there's no such thing as Frame 0 in a Flash movie timeline). In the end, this part of the function will send the screen movie clip instance to Frame 1 (showing a blank TV screen) or Frame 2 (showing Channel 1). The function finishes by telling the light on the remote control to play (which causes it to blink, providing a visual indication that a button has been pressed). There is now a function on Frame 1 of the main, or root, timeline. Although this function contains several actions, none of them are executed until the function is called. -
Double-click the remote-control movie clip instance to edit it in place. With the Actions panel open, select the Power button and add this ActionScript: on (release) { _root.togglePower(); } 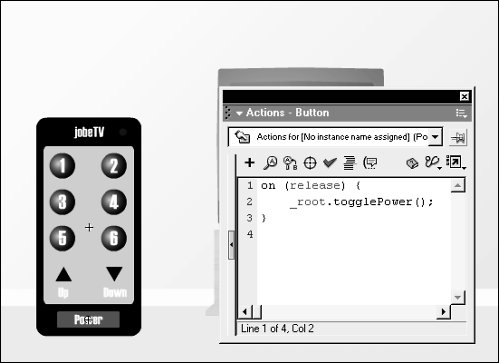 This ActionScript calls the togglePower() function we created in the previous step. It's important to note that since the togglePower() function resides on the main timeline, you must use _root as the target path when calling this function. -
Choose Control > Test Movie. Then click the Power button several times to view the TV on/off functionality you've created. Every time you press the Power button, the togglePower() function is called so that all of the actions within that function are performed. As mentioned, the actions within the function toggle the state of the TV. -
Close the test movie and save your work as television2.fla. You have now created and used a function! In the next section, we'll build on this idea to create a more powerful and versatile function. |