As we suggested earlier in the chapter, there are a few ways to integrate CMS with your existing applications. To demonstrate what we mean, we've provided a couple of code samples to illustrate our point. The examples we've provided here are not an exhaustive list. In fact, our examples will probably be "best case" scenarios that probably don't exist in reality. However, the basics of each example should help you with your real integrations. Displaying Data from an External Data Source Displaying dynamic data from an external source is a very common operation to perform within a Web application. Performing the same operation isn't fundamentally different in CMS. If anything, displaying data from a database is exactly the same in a CMS template as it is in an ASP. NET page, since CMS templates are in fact ASP.NET pages. However, to prove this point to you, we've provided a small example in Listing 32-1. In this listing, we demonstrate how to retrieve a record set from a database and display the result in a data grid control. Listing 32-1 Displaying external data in a data grid [View full width] using System; using System.Collections; using System.ComponentModel; using System.Data; using System.Data.OleDb; using System.Drawing; using System.Web; using System.Web.SessionState; using System.Web.UI; using System.Web.UI.WebControls; using System.Web.UI.HtmlControls; using Microsoft.ContentManagement.Publishing; namespace botsconsulting.Templates { /// <summary> /// Summary description for generic. /// </summary> public class dataintegration : System.Web.UI.Page { protected Microsoft.ContentManagement.WebControls.RobotMetaTag RobotMetaTag1; protected System.Web.UI.WebControls.Literal PageTitle; protected Microsoft.ContentManagement.WebControls. SingleImagePlaceholderControl CallOut; protected Microsoft.ContentManagement.WebControls. SingleImagePlaceholderControl CallOutImage; protected Microsoft.ContentManagement.WebControls. HtmlPlaceholderControl CallOutImageCaption; protected System.Web.UI.WebControls.Literal BodyTitle; protected Microsoft.ContentManagement.WebControls. HtmlPlaceholderControl BodyCopy; protected System.Web.UI.WebControls.DataGrid myDataGrid; protected botsconsulting.UserControls.header Header; private OleDbConnection myConnection; private void Page_Load(object sender, System.EventArgs e) { this.BodyTitle.Text = "<b>" + CmsHttpContext.Current.Posting.DisplayName + "</b>"; CreateOleDbConnection(); BindDBtoDataGrid(); } public void CreateOleDbConnection() { string myConnString = "Provider=SQLOLEDB.1;Integrated Security=SSPI;Persist Security Info=False;Initial Catalog=Northwind;Data Source=localhost;"; myConnection = new OleDbConnection(myConnString); } public void BindDBtoDataGrid() { string myQuery = "Select LastName,FirstName,Title from employees"; OleDbCommand myCommand = new OleDbCommand(myQuery); myCommand.Connection = myConnection; myConnection.Open(); myDataGrid.DataSource = myCommand.ExecuteReader(); myDataGrid.DataBind(); myCommand.Connection.Close(); } } In this example, we connected to the Northwind database provided with SQL Server. We selected all the records in the Employee table, displaying three columns in a data grid. Figure 32-1 shows what this template looks like with a posting in the BOTS Consulting site. Figure 32-1. Dynamic data template in the BOTS site 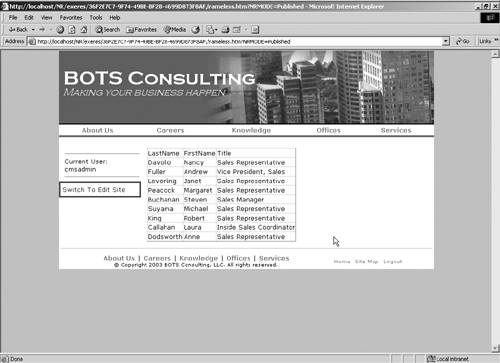 Allowing Content Contributors to Select What Data Is Displayed The earlier example is probably not a realistic example of what you would do in an application. However, it does demonstrate that displaying dynamic data in CMS isn't difficult. The real power, though, comes from taking the previous example and building on it. Again, one of the reasons you might integrate application code with a template is to provide content contributors with more flexibility in the deployment of that application. In this next example (Listing 32-2), we've created a custom placeholder that allows a content contributor to determine which record to display on the live page. Listing 32-2 Using a custom placeholder to determine which record to display [View full width] using System; using System.Data.OleDb; using System.Web.UI; using System.Web.UI.WebControls; using System.ComponentModel; using Microsoft.ContentManagement.Publishing.Extensions.Placeholders; using Microsoft.ContentManagement.WebControls; namespace botsconsultingwebcontrols { /// <summary> /// Summary description for DropDownPlaceholderControl. /// </summary> [ToolboxData("<{0}:DataDrivenDropDownListPlaceholder runat=server></{0} :DataDrivenDropDownListPlaceholder>")] public class DataDrivenDropDownListPlaceholder : Microsoft.ContentManagement .WebControls.BasePlaceholderControl { protected System.Web.UI.WebControls.DropDownList IndustryList; protected System.Web.UI.WebControls.DataGrid SelectedEmployees; protected override void CreateAuthoringChildControls (BaseModeContainer authoringContainer) { this.IndustryList = new DropDownList(); this.IndustryList.Items.Add(new ListItem("Sales Representative","Sales Representative")); this.IndustryList.Items.Add(new ListItem("Sales Manager","Sales Manager")); this.IndustryList.Items.Add(new ListItem("Inside Sales Coordinator" ,"Inside Sales Coordinator")); this.IndustryList.Items.Add(new ListItem("Vice President, Sales","Vice President, Sales")); this.IndustryList.EnableViewState = false; authoringContainer.Controls.Add(this.IndustryList); } protected override void SavePlaceholderContent (PlaceholderControlSaveEventArgs e) { EnsureChildControls(); try { ((HtmlPlaceholder)this.BoundPlaceholder).Html = this.IndustryList .SelectedItem.Value.ToString(); } catch (Exception ex) { string saveExceptionMessage = "Error saving placeholder contents: " + this.GetType().Name + " :: " + this.ID + " :: " + ex.Message; Exception saveException = new Exception (saveExceptionMessage, ex); throw saveException; } } protected override void LoadPlaceholderContentForAuthoring (PlaceholderControlEventArgs e) { EnsureChildControls(); try { ListItem savedSelectedIndustry = this.IndustryList.Items .FindByValue(((HtmlPlaceholder)this.BoundPlaceholder).Text); this.IndustryList.SelectedIndex = this.IndustryList.Items.IndexOf (savedSelectedIndustry); } catch (Exception ex) { string saveExceptionMessage = "Error loading placeholder contents: " + this.GetType().Name + " :: " + this.ID + " :: " + ex.Message; Exception saveException = new Exception (saveExceptionMessage, ex); throw saveException; } } protected override void CreatePresentationChildControls (BaseModeContainer presentationContainer) { SelectedEmployees = new DataGrid(); presentationContainer.Controls.Add(SelectedEmployees); } protected override void LoadPlaceholderContentForPresentation (PlaceholderControlEventArgs e) { EnsureChildControls(); try { // Grab the value from the placeholder string mySelectedEmployees = ((HtmlPlaceholder) this .BoundPlaceholder).Text; // Open a new connect and perform a search for values that match // the save placeholder value OleDbConnection myConnection; string myConnString = "Provider=SQLOLEDB.1;Integrated Security=SSPI;Persist Security Info=False;Initial Catalog=Northwind;Data Source=localhost;"; myConnection = new OleDbConnection(myConnString); string myQuery = "Select LastName,FirstName from Employees where Title = '" + mySelectedEmployees + "';"; OleDbCommand myCommand = new OleDbCommand(myQuery); myCommand.Connection = myConnection; myConnection.Open(); // Fill the datagrid with the returned records this.SelectedEmployees.DataSource = myCommand.ExecuteReader(); } catch (Exception ex) { string saveExceptionMessage = "Error loading placeholder contents: " + this.GetType().Name + " :: " + this.ID + " :: " + ex.Message; Exception saveException = new Exception(saveExceptionMessage, ex); throw saveException; } } } } In Listing 32-2 you can see how we fill the drop-down list box with the four titles that exist in the Employee table. Then, during presentation, we perform a SQL query against the table to find all records that have the selected value in the title field. In this way, the content contributor can select what employees they want to display based on the employee title. We could have taken this a step further by filling the drop-down list control with the actual values from the database as well. The implementation you choose is up to you. In Figures 32-2 and 32-3 you can see what each mode of the new custom placeholder looks like. Figure 32-2. The new custom placeholder in authoring mode 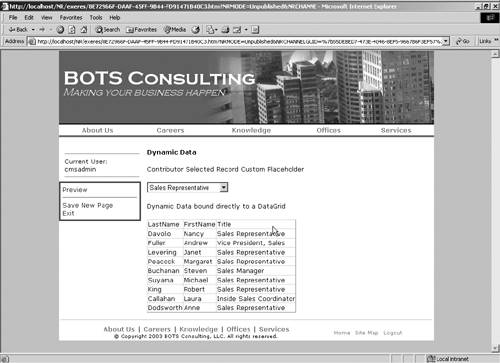 Figure 32-3. The new custom placeholder in presentation mode 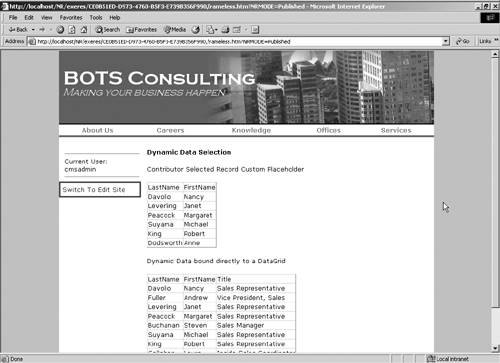 |