Value Change Events Components in a web application often depend on each other. For example, in the application shown in Figure 7-2, the value of the "State" prompt depends on the "Country" menu's value. You can keep dependent components in synch with value change events, which are fired by input components after their new value has been validated. The application in Figure 7-2 attaches a value change listener to the "Country" menu and uses the onchange attribute to force a form submit after the menu's value is changed: <h:selectOneMenu value="#{form.country}" onchange="submit()" valueChangeListener="#{form.countryChanged}"> <f:selectItems value="#{form.countryNames}"/> </h:selectOneMenu> When a user selects a country from the menu, the JavaScript submit function is invoked to submit the menu's form, which subsequently invokes the JSF life cycle. After the Process Validations phase, the JSF implementation invokes the form bean's countryChanged method. That method changes the view root's locale, according to the new country value: private static String US = "United States"; ... public void countryChanged(ValueChangeEvent event) { FacesContext context = FacesContext.getCurrentInstance(); if (US.equals((String) event.getNewValue())) context.getViewRoot().setLocale(Locale.US); else context.getViewRoot().setLocale(Locale.CANADA); } } Like all value change listeners, the preceding listener is passed a value change event. The listener uses that event to access the component's new value. The ValueChangeEvent class extends FacesEvent, both of which reside in the javax.faces.event package. The most commonly used methods from those classes are listed below. javax.faces.event.ValueChangeEvent | -
UIComponent getComponent() Returns the input component that triggered the event. -
Object getNewValue() Returns the component's new value, after the value has been converted and validated. -
Object getOldValue() Returns the component's previous value. | javax.faces.event.FacesEvent | -
void queue() Queues the event for delivery at the end of the current life cycle phase. -
PhaseId getPhaseId() Returns the phase identifier corresponding to the phase during which the event is delivered. -
void setPhaseId(PhaseId) Sets the phase identifier corresponding to the phase during which the event is delivered. | The directory structure for the application in Figure 7-2 is shown in Figure 7-3 and the application is shown in Listing 7-1 through Listing 7-5. 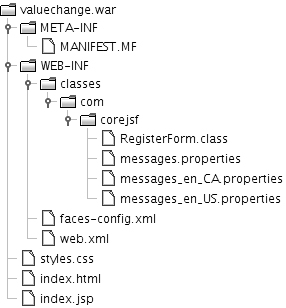 Listing 7-1. valuechange/web/index.jsp 1. <html> 2. <%@ taglib uri="http://java.sun.com/jsf/core" prefix="f" %> 3. <%@ taglib uri="http://java.sun.com/jsf/html" prefix="h" %> 4. <f:view> 5. <head> 6. <link href="styles.css" rel="stylesheet" type="text/css"/> 7. <title> 8. <h:outputText value="#{msgs.windowTitle}"/> 9. </title> 10. </head> 11. 12. <body> 13. <h:outputText value="#{msgs.pageTitle}" style/> 14. <p/> 15. <h:form> 16. <h:panelGrid columns="2"> 17. <h:outputText value="#{msgs.streetAddressPrompt}"/> 18. <h:inputText value="#{form.streetAddress}" 19. required="true"/> 20. 21. <h:outputText value="#{msgs.cityPrompt}"/> 22. <h:inputText value="#{form.city}"/> 23. 24. <h:outputText value="#{msgs.statePrompt}"/> 25. <h:inputText value="#{form.state}"/> 26. 27. <h:outputText value="#{msgs.countryPrompt}"/> 28. 29. <h:selectOneMenu value="#{form.country}" 30. onchange="submit()" 31. valueChangeListener="#{form.countryChanged}"> 32. <f:selectItems value="#{form.countryNames}"/> 33. </h:selectOneMenu> 34. </h:panelGrid> 35. <p/> 36. <h:commandButton value="#{msgs.submit}"/> 37. </h:form> 38. </body> 39. </f:view> 40. </html>
| Listing 7-2. valuechange/src/java/com/corejsf/RegisterForm.java 1. package com.corejsf; 2. 3. import java.util.ArrayList; 4. import java.util.Collection; 5. import java.util.Locale; 6. import javax.faces.context.FacesContext; 7. import javax.faces.event.ValueChangeEvent; 8. import javax.faces.model.SelectItem; 9. 10. public class RegisterForm { 11. private String streetAddress; 12. private String city; 13. private String state; 14. private String country; 15. 16. private static final String US = "United States"; 17. private static final String CANADA = "Canada"; 18. private static final String[] COUNTRY_NAMES = { US, CANADA }; 19. private static ArrayList<SelectItem> countryItems = null; 20. 21. // PROPERTY: countryNames 22. public Collection getCountryNames() { 23. if (countryItems == null) { 24. countryItems = new ArrayList<SelectItem>(); 25. for (int i = 0; i < COUNTRY_NAMES.length; i++) { 26. countryItems.add(new SelectItem(COUNTRY_NAMES[i])); 27. } 28. } 29. return countryItems; 30. } 31. 32. // PROPERTY: streetAddress 33. public void setStreetAddress(String newValue) { streetAddress = newValue; } 34. public String getStreetAddress() { return streetAddress; } 35. 36. // PROPERTY: city 37. public void setCity(String newValue) { city = newValue; } 38. public String getCity() { return city; } 39. 40. // PROPERTY: state 41. public void setState(String newValue) { state = newValue; } 42. public String getState() { return state; } 43. 44. // PROPERTY: country 45. public void setCountry(String newValue) { country = newValue; } 46. public String getCountry() { return country; } 47. 48. public void countryChanged(ValueChangeEvent event) { 49. FacesContext context = FacesContext.getCurrentInstance(); 50. 51. if (US.equals((String) event.getNewValue())) 52. context.getViewRoot().setLocale(Locale.US); 53. else 54. context.getViewRoot().setLocale(Locale.CANADA); 55. } 56. }
| Listing 7-3. valuechange/web/WEB-INF/faces-config.xml 1. <?xml version="1.0"?> 2. <faces-config xmlns="http://java.sun.com/xml/ns/javaee" 3. xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" 4. xsi:schemaLocation="http://java.sun.com/xml/ns/javaee 5. http://java.sun.com/xml/ns/javaee/web-facesconfig_1_2.xsd" 6. version="1.2"> 7. <application> 8. <resource-bundle> 9. <base-name>com.corejsf.messages</base-name> 10. <var>msgs</var> 11. </resource-bundle> 12. </application> 13. 14. <managed-bean> 15. <managed-bean-name>form</managed-bean-name> 16. <managed-bean-class>com.corejsf.RegisterForm</managed-bean-class> 17. <managed-bean-scope>session</managed-bean-scope> 18. </managed-bean> 19. </faces-config>
| Listing 7-4. valuechange/src/java/com/corejsf/messages_en_US.properties 1. windowTitle=Using Value Change Events 2. pageTitle=Please fill in your address 3. 4. streetAddressPrompt=Address 5. cityPrompt=City 6. statePrompt=State 7. countryPrompt=Country 8. submit=Submit address
| Listing 7-5. valuechange/src/java/com/corejsf/messages_en_CA.properties 1. windowTitle=Using Value Change Events 2. pageTitle=Please fill in your address 3. 4. streetAddressPrompt=Address 5. cityPrompt=City 6. statePrompt=Province 7. countryPrompt=Country 8. submit=Submit address
| |