Putting all these exception-handling constructs together we have the following form: try { // One or more statements that can throw an exception } catch( ExceptionType1 e1 ) { // Handle an exception that can be thrown in the try block } catch( ExceptionType2 e2 ) { // Handle an exception that can be thrown in the try block } finally { // Do any cleanup } Any method call that can throw an exception must be contained within a try block, and there must be a catch block to catch that exception class or a superclass of that exception class. Exception classes are built on an inheritance hierarchy and if catch blocks specify a class higher in the inheritance hierarchy (one of the exception class's superclasses), it will handle all the exception's subclasses. The key to appropriately handling an exception is to define the more specific exceptions in earlier catch blocks, and the more general exceptions in the later catch blocks. A try block must be followed by either a catch block, a finally block, or both; it cannot be alone. If an exception occurs, the exception that is thrown is evaluated against each of the catch blocks in turn, starting from the top of the code until the exception class is found or a superclass of the exception class is found. At that point the body of the matching catch block is executed and, if there is a finally block, it is executed. For example consider the exception hierarchy defined in Figure 9.1. Figure 9.1. Example exception hierarchy class diagram. 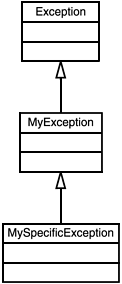 MySpecificException is a subclass of MyException, which is a subclass of Exception. Note that inheritance is specified in class diagrams by drawing an arrow pointing from the subclass to the superclass. If you want to handle each one of these exceptions, you would need to write your catch blocks starting with the most specialized class and moving to the most general class. For example: try { // Statement that can throw an exception } catch( MySpecificException mse ) { // do something } catch( MyException me ) { // do something } catch( Exception e ) { // do something } Thus, if a MySpecificException instance is thrown, the first catch block handles it. If a MyException instance is thrown, the first catch block is skipped, and the second one handles the exception. Now, if you reversed the order of the catch blocks you would be in trouble. try { // Statement that can throw an exception } catch( Exception e ) { // do something } catch( MyException me ) { // do something } catch( MySpecificException mse ) { // do something } In this case if a MySpecificException instance is thrown, the first catch block, or specifically the Exception catch block, would handle the exception; the MySpecificException catch block that you intended to handle it would never receive the exception. Furthermore, Java will give you a compilation error if you try to do this. |