.NET components can call COM components, and COM components can call .NET components. This means that you can migrate one component (a control, a class library, and so on) at a time and still keep all your code working together. Four basic reasons exist for maintaining part of a system in COM components while moving other parts to .NET components: It takes time to learn Visual C# .NET and the .NET Framework. While you're making your way up the learning curve, you might have to continue development or use of existing COM components. You might have components that can't be easily moved to .NET because they use language features that are no longer supported or because of other implementation quirks. It takes time to move code from one system to another. Unless you can afford extended downtime, a gradual move lets you write the converted code at a slower pace. Your application might depend on third-party controls or libraries for which you do not have the source code. In the following sections, you learn how to encapsulate COM components for use with .NET applications. There are both command-line and GUI tools for working with COM components. Understanding Runtime Callable Wrappers (RCW) Visual C# .NET creates code that operates within the .NET common language runtime (CLR). Code that operates within the CLR is called managed code. Managed code benefits from the services that the CLR offers, including garbage collection, memory management, and support for versioning and security. COM components are unmanaged code because COM was designed before the CLR existed, and COM components don't make use of any of the services of the CLR. With the help of a proxy, you can make a COM component look like a .NET component. A proxy accepts commands and messages from one component, modifies them, and passes them to another component. The particular type of proxy that enables you to use COM components within a .NET application is called a runtime callable wrapper (RCW). That is, it's a proxy that can be called by the CLR. Figure 9.1 shows schematically how the pieces fit together. Figure 9.1. RCW enables you to use COM components within the .NET framework. 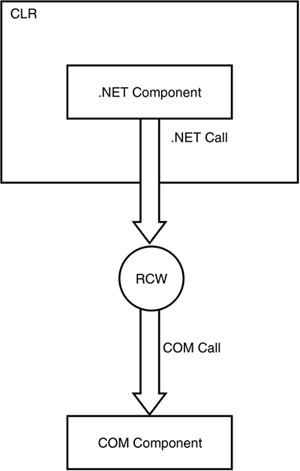 The task of using COM components from the .NET Framework is made substantially easier by the fact that COM components, like .NET components, have metadata that describes their interfaces. For .NET components, the metadata is embedded in the assembly manifest. For COM components, the metadata is stored in a type library. A type library can be a separate file, or (as with Visual Basic 6.0 class libraries) it can be embedded within another file. To see how COM interoperability works, you need a COM library. Follow these steps to build a simple one: Launch Visual Basic 6.0 and create a new ActiveX DLL project. Select the Project1 node in the Project Explorer window and rename it MyCustomer. Select the Class1 node in the Project Explorer window and rename it Balances. Add this code to the Balances class: Option Explicit Private mintCustomerCount As Integer Private macurBalances(1 To 10) As Currency ' Create a read-only CustomerCount property Public Property Get CustomerCount() As Integer CustomerCount = mintCustomerCount End Property ' Create a GetBalance method Public Function GetBalance(CustomerNumber As Integer)_ As Currency GetBalance = macurBalances(CustomerNumber) End Function ' Initialize the data Private Sub Class_Initialize() Dim intI As Integer mintCustomerCount = 10 For intI = 1 To 10 macurBalances(intI) = Int(Rnd(1) * 100000) / 100 Next intI End Sub Save the Visual Basic project. Select File, Make MyCustomer.dll to create the COM component. This step also registers the COM component on your computer. When you use Visual Basic 6.0 to build the MyCustomer.dll, the COM DLL is automatically registered in the Windows Registry. If you are using the code files from the CD, or if you have an unregistered COM DLL, you need to register the COM DLL in the Windows Registry before you can use it in .NET code. You can register by using regsvr32.exe at the command prompt, as shown here: regsvr32 MyCustomer.dll Using the Type Library Importer Tool (tlbimp.exe) The .NET Framework includes a tool, the Type Library Importer tool (tlbimp.exe), that can create an RCW from COM metadata contained in a type library. The following steps demonstrate how to use the Type Library Importer: Launch the Visual Studio .NET Command Prompt, navigate to the folder that contains the MyCustomer.dll COM library, and issue the following command to run the Type Library Importer tool: tlbimp MyCustomer.dll /out:NETMyCustomer.dll Open Visual Studio .NET and create a new blank solution named C09 at C:\EC70320. (If you have not done it before, set this directory as an IIS virtual root.) Add an ASP.NET Web Service project named Example9_1 to the solution. Right-click the References node in Solution Explorer and select Add Reference. Click the Browse button in the Add Reference dialog box. Browse to the NETMyCustomer.dll file that you created in step 1. Click OK to add the reference to the project. Add the following Web method definition to the Web service: [WebMethod] public decimal RetrieveBalance(short custNumber) { NETMyCustomer.Balances b = new NETMyCustomer.Balances(); return b.GetBalance(ref custNumber); } Run the project. You should see that a browser is launched showing the test page. Click on the RetrieveBalance method link. Enter a number between 1 and 10 for the custNumber parameter, and click the Invoke button. A second browser window opens with that customer's balance. The Type Library Importer creates an RCW for the COM type library. This RCW is a library that you can add to your .NET project as a reference. After you do that, you can use the classes in the COM component just like native .NET classes. When you use a class from the COM component, .NET makes the call to the RCW, which, in turn, forwards the call to the original COM component and returns the results to your .NET managed code. Table 9.1 displays the important command-line options of the Type Library Importer tool. Table 9.1. Important Command-Line Options for the Type Library Importer ToolOption | Meaning |
---|
/asmversion:versionNumber | Specifies the version number for the created assembly | /delaysign | Prepares the assembly for delay signing | /help | Displays help for command-line options | /keycontainer:containerName | Signs the assembly with the strong name from the specified key container | /keyfile:filename | Specifies a file containing public/private key pairs that are used to sign the resulting file | /namespace:namespace | Specifies the namespace for the created assembly | /out:filename | Specifies the name of the created assembly | /primary | Produces a primary interop assembly | /publickey:filename | Specifies the file containing a public key that is used to sign the resulting file | /reference:filename | Specifies a file to be used to resolve references from the file being imported | /silent | Suppresses information that would otherwise be displayed on the command line during conversion | /strictref | Refuses to create the assembly if one or more references cannot be resolved | /sysarray | Imports COM SAFEARRAY as instances of the System.Array type | /unsafe | Creates interfaces without the .NET Framework security checks | /verbose | Displays additional information on the command line during conversion | /? | Displays help about command-line options | 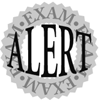 | If you want to use a COM DLL from a serviced component, or if you want to place the COM DLL in the Global Assembly Cache, you must assign a strong name to the COM DLL. To assign a strong name using a public/private key pair, you use the /keyfile option of the Type Library Importer tool (tlbimp.exe). |
Using COM Components Directly The Visual Studio .NET interface provides a streamlined way to use a COM component from your .NET code. To add a reference to a COM library in a project, you can select the COM tab in the Add Reference dialog box and select the COM library. When you directly reference a COM library from the Visual Studio .NET Integrated Development Environment (IDE), the effect is almost the same as if you used the Type Library Importer tool to import the same library. Visual Studio .NET creates a new namespace with the name of the original library and then exposes the classes from the library within that namespace. Although you can use either of the two methods described in this chapter to call a COM component from a .NET component, there are reasons to prefer one method over the other: For a COM component that will be used in only a single Visual C# .NET project and that you wrote yourself, use the easiest method: direct reference from the .NET project. This method is suitable only for a truly private component that does not need to be shared by the other projects. If a COM component is shared among multiple projects, use the Type Library Importer tool so that you can sign the resulting assembly and place it in the Global Assembly Cache (GAC). Shared code must be signed with a strong name. If you need to control details of the created assembly such as its name, namespace, or version number you must use the Type Library Importer tool. The direct reference method gives you no control over the details of the created assembly. 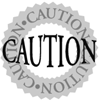 | You should not use either of the import methods on code that is written by another developer. You are not allowed to sign code that is written by someone else. If you need to use a COM component from another developer, you should obtain a primary interop assembly (PIA) from the component's original developer. Microsoft supplies PIAs for all its own common libraries. For example, there are PIAs for the components of Microsoft Office, available at www.msdn.com/downloads/list/office.asp. |
|