The key to understanding Web services is knowing something about the protocols that make them possible. These protocols are as follows: Simple Object Access Protocol (SOAP) Disco and Universal Description, Discovery, and Integration (UDDI) Web Services Description Language (WSDL) These protocols use XML messages transmitted over the HTTP protocol. This has two benefits. First, because Web services messages are formatted as XML, they're reasonably easy for humans to read and understand. Second, because those messages are transmitted over the pervasive HTTP protocol, they can normally reach any machine on the Internet without worrying about firewalls. SOAP For Web services to manipulate objects through XML messages, there has to be a way to translate objects (and their methods and properties) into XML. SOAP is a way to encapsulate method calls as XML sent via HTTP. Here's a typical SOAP message sent from a Web services client to a Web services server: <?xml version="1.0" encoding="utf-8"?> <soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/" xmlns:soapenc="http://schemas.xmlsoap.org/soap/encoding/" xmlns:tns="http://www.capeclear.com/AirportWeather.wsdl" xmlns:types="http://www.capeclear.com/AirportWeather.wsdl/encodedTypes" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:xsd="http://www.w3.org/2001/XMLSchema"> <soap:Body soap:encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"> <q1:getLocation xmlns:q1="capeconnect:AirportWeather:Station"> <arg0 xsi:type="xsd:string">KDTW</arg0> </q1:getLocation> </soap:Body> </soap:Envelope> The SOAP message brings out some obvious points: The SOAP message consists of an envelope that contains a body, both of which are identified with a specific XML tag. This particular message invokes a method named getLocation() from a specified uniform resource locator (URL). The method takes a single parameter, arg0, which is transmitted as an XML element. Following is a portion of the SOAP message returned by the server: <?xml version="1.0" encoding="utf-8"?> <SOAP-ENV:Envelope xmlns:SOAP-ENV="http://schemas.xmlsoap.org/soap/envelope/" xmlns:xsd="http://www.w3.org/2001/XMLSchema" xmlns:cc1="http://www.capeclear.com/AirportWeather.xsd" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:SOAP-ENC="http://schemas.xmlsoap.org/soap/encoding/"> <SOAP-ENV:Body SOAP-ENV:encodingStyle="http://schemas.xmlsoap.org/soap/encoding/"> <cc2:getLocationResponse xmlns:cc2="capeconnect:AirportWeather:Station" SOAP-ENC:root="1"> <return xsi:type="xsd:string"> Detroit, Detroit Metropolitan Wayne County Airport, MI, United States </return> </cc2:getLocationResponse> </SOAP-ENV:Body> </SOAP-ENV:Envelope> In the response message, the getLocationResponse element is the result of the call to the object on the server. It includes a string wrapped as an XML element. Disco and UDDI Before you can use a Web service, you need to know where to find it. Protocols such as Disco and UDDI enable you to communicate with a Web server to discover the details of its available Web services. WSDL Another prerequisite for using a Web service is knowing the SOAP messages it can receive and send. You can obtain this knowledge by parsing Web Services Description Language (WSDL) files. WSDL is a standard by which a Web service can inform clients what messages it accepts and which results it will return. Here's a portion of a WSDL file: <?xml version="1.0" encoding="utf-16"?> <definitions xmlns:http="http://schemas.xmlsoap.org/wsdl/http/" xmlns:soap="http://schemas.xmlsoap.org/wsdl/soap/" xmlns:s="http://www.w3.org/2001/XMLSchema" xmlns:s0="http://www.capeclear.com/AirportWeather.xsd" xmlns:soapenc="http://schemas.xmlsoap.org/soap/encoding/" xmlns:tns="http://www.capeclear.com/AirportWeather.wsdl" xmlns:tm="http://microsoft.com/wsdl/mime/textMatching/" xmlns:mime="http://schemas.xmlsoap.org/wsdl/mime/" targetNamespace="http://www.capeclear.com/AirportWeather.wsdl" name="AirportWeather" xmlns="http://schemas.xmlsoap.org/wsdl/"> <types> <s:schema targetNamespace="http://www.capeclear.com/AirportWeather.xsd"> <s:complexType name="WeatherSummary"> <s:sequence> <s:element minOccurs="1" maxOccurs="1" name="location" nillable="true" type="s:string" /> <s:element minOccurs="1" maxOccurs="1" name="wind" nillable="true" type="s:string" /> <s:element minOccurs="1" maxOccurs="1" name="sky" nillable="true" type="s:string" /> <s:element minOccurs="1" maxOccurs="1" name="temp" nillable="true" type="s:string" /> <s:element minOccurs="1" maxOccurs="1" name="humidity" nillable="true" type="s:string" /> <s:element minOccurs="1" maxOccurs="1" name="pressure" nillable="true" type="s:string" /> <s:element minOccurs="1" maxOccurs="1" name="visibility" nillable="true" type="s:string" /> </s:sequence> </s:complexType> </s:schema> </types> <message name="getHumidity"> <part name="arg0" type="s:string" /> </message> <message name="getHumidityResponse"> <part name="return" type="s:string" /> </message> <message name="getLocation"> <part name="arg0" type="s:string" /> </message> <message name="getLocationResponse"> <part name="return" type="s:string" /> </message> <message name="getOb"> <part name="arg0" type="s:string" /> </message> <message name="getObResponse"> <part name="return" type="s:string" /> </message> ... </definitions> WSDL files define everything about the public interface of a Web service, including the following: 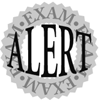 | Although the WSDL files enable interaction with Web services without any prior knowledge, these files are not required for a Web service to function. You can make a Web service available on the Internet without any WSDL files. In that case, only clients that already know the expected message formats and the location of the Web service are capable of using it. |
Invoking a Web Service Now that you know the basics, it's time to see a Web service in action. The following steps show how you can use a Web service in this case, one that supplies the weather at any airport worldwide. Create a blank solution using Visual Studio .NET. Name the solution C05 and specify its location as C:\EC70320. Add a new Visual C# ASP.NET Web Application project to the solution. Specify http://localhost/EC70320/C05/Example5_1 as the project's location. Right-click the References folder in Solution Explorer and select Add Web Reference. This opens the Add Web Reference dialog box. Type http://live.capescience.com/wsdl/AirportWeather.wsdl in the URL and press Enter. This connects to the Airport Weather Web service and downloads the information shown in Figure 5.1. Figure 5.1. The Add Web Reference dialog box enables you to connect to a Web service via the Internet. 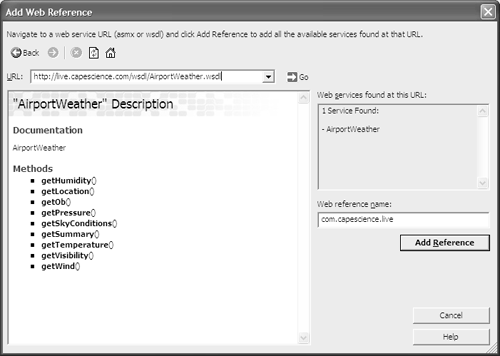
Click the Add Reference button to add a reference to the Airport Weather Service in your project. Open the Web form and place a Label control, TextBox control (txtCode), Button control (btnGetWeather), and Listbox control (lbResults) on the form. Change the Text property of the Label control to Airport Code:. Double-click the Button control and enter the following code to invoke the Web service when the user clicks the Get Weather button: private void btnGetWeather_Click(object sender, System.EventArgs e) { // Declare the Web service main object com.capescience.live.AirportWeather aw = new com.capescience.live.AirportWeather(); // Invoke the service to get a summary object com.capescience.live.WeatherSummary ws = aw.getSummary(txtCode.Text); // And display the results lbResults.Items.Clear(); lbResults.Items.Add(ws.location); lbResults.Items.Add("Wind " + ws.wind); lbResults.Items.Add("Sky " + ws.sky); lbResults.Items.Add("Temperature " + ws.temp); lbResults.Items.Add("Humidity " + ws.humidity); lbResults.Items.Add("Barometer " + ws.pressure); lbResults.Items.Add("Visibility " + ws.visibility); } Select Debug, Start to execute the Web form, and enter a four-letter ICAO airport code (such as KDTW for Detroit). Then click the Get Weather button. After a brief pause while the Web service is invoked, you'll see the current weather at that airport you selected.  | You can find a list of four-letter ICAO airport codes to use with this Web service at www.ar-group.com/icaoiata.htm. Codes for airports in the United States all start with K; codes for Canadian airports all start with C. |
There's a lot going on here, even though you don't see most of it happening. When you create the Web reference, for example, Visual Studio .NET reads the appropriate WSDL file to determine which classes and methods are available from the remote server. When you call a method on an object from that server, the .NET infrastructure translates your call and the results into SOAP messages without any intervention on your part. |