16.2. termios Overview All tty manipulation is done through one structure, struct termios, and through several functions, all defined in the <termios.h> header file. Of those functions, only six are commonly used, and when you do not have to set line speeds, you are likely to use only two of them. The two most important functions are the tcgetattr() and tcsetattr() functions: #include <termios.h> struct termios { tcflag_t c_iflag; /* input mode flags */ tcflag_t c_oflag; /* output mode flags */ tcflag_t c_cflag; /* control mode flags */ tcflag_t c_lflag; /* local mode flags */ cc_t c_line; /* line discipline */ cc_t c_cc[NCCS]; /* control characters */ }; int tcgetattr (int fd, struct termios *tp); int tcsetattr (int fd, int oact, struct termios *tp); In almost every situation, programs should use tcgetattr() to get a device's current settings, modify those settings, and then use tcsetattr() to make the modified settings active. Many programs also save a copy of the original settings and restore them before terminating. In general, modify only the settings that you know you care about; changing other settings may make it difficult for users to work around unusual system configurations (or bugs in your code). tcsetattr() may not honor all the settings you choose; it is allowed to ignore arbitrary settings. In particular, if the hardware simply does not support a setting, tcsetattr() ignores it rather than return an error. If you care that a setting really takes effect, you must use tcgetattr() after tcsetattr() and test to make sure that your change took effect. To get a tty device's settings, you have to open the device and use the file descriptor in the tcgetattr() call. This poses a problem with some tty devices; some normally may be opened only once, to prevent device contention. Fortunately, giving the O_NONBLOCK flag to open() causes it to be opened immediately and not block on any operations. However, you may still prefer to block on read(); if so, use fcntl() to turn off O_NONBLOCK mode before you read or write to it: fcntl(fd, F_SETFL, fcntl(fd, F_GETFL, 0) & ~O_NONBLOCK); The four termios flags control four distinct parts of managing input and output. The input flag, c_iflag, determines how received characters are interpreted and processed. The output flag, c_oflag, determines how characters your process writes to the tty are interpreted and processed. The control flag, c_cflag, determines serial protocol characteristics of the device and is useful only for physical devices. The local flag, c_lflag, determines how characters are collected and processed before they are sent to output processing. Figure 16.1 shows a simplified view of how each of these flags fits into the grand scheme of character processing. Figure 16.1. Simplified View of tty Processing 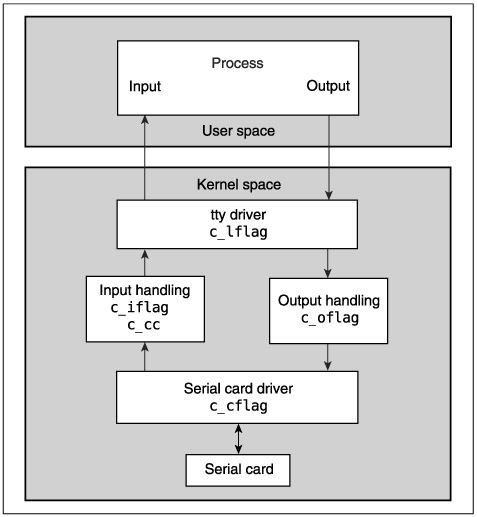 We first demonstrate ways to use termios, and then present a short reference to it. |