Let us get started the hard way: compiling and launching a Java program from the command line. Open a shell window. Go to the CoreJavaBook/v1ch2/Welcome directory. (The CoreJavaBook directory is the directory into which you installed the source code for the book examples, as explained on page 18.) Then enter the following commands: javac Welcome.java java Welcome You should see the output shown in Figure 2-1 in the shell window. Figure 2-1. Compiling and running Welcome.java 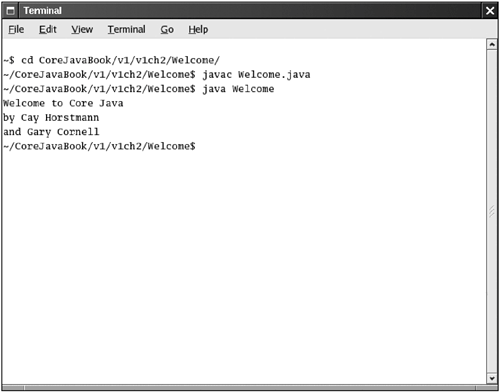
NOTE  | In Windows, follow these instructions to open a shell window. Select the "Run" option from the start menu. If you use Windows NT/2000/XP, type cmd; otherwise, type command. Press ENTER, and a shell window appears. If you've never seen one of these, we suggest that you work through a tutorial that teaches the basics about the command line. Many computer science departments have tutorials on the Web, such as http://www.cs.sjsu.edu/faculty/horstman/CS46A/windows/tutorial.html. |
Congratulations! You have just compiled and run your first Java program. What happened? The javac program is the Java compiler. It compiles the file Welcome.java into the file Welcome.class. The java program launches the Java virtual machine. It executes the bytecodes that the compiler placed in the class file. NOTE  | If you got an error message complaining about the line for (String g : greeting)
then you probably use an older version of the Java compiler. JDK 5.0 introduces a number of very desirable features to the Java programming language, and we take advantage of them in this book. If you are using an older version of Java, you need to rewrite the loop as follows: for (int i = 0; i < greeting.length; i++) System.out.println(greeting[i]);
In this book, we always use the language features of JDK 5.0. It is a straightforward matter to transform them into their old-fashioned counterparts see Appendix B for details. |
The Welcome program is extremely simple. It merely prints a message to the console. You may enjoy looking inside the program shown in Example 2-1 we explain how it works in the next chapter. Example 2-1. Welcome.java 1. public class Welcome 2. { 3. public static void main(String[] args) 4. { 5. String[] greeting = new String[3]; 6. greeting[0] = "Welcome to Core Java"; 7. greeting[1] = "by Cay Horstmann"; 8. greeting[2] = "and Gary Cornell"; 9. 10. for (String g : greeting) 11. System.out.println(g); 12. } 13. } Troubleshooting Hints In the age of visual development environments, many programmers are unfamiliar with running programs in a shell window. Any number of things can go wrong, leading to frustrating results. Pay attention to the following points: If you type in the program by hand, make sure you pay attention to uppercase and lowercase letters. In particular, the class name is Welcome and not welcome or WELCOME. The compiler requires a file name (Welcome.java). When you run the program, you specify a class name (Welcome) without a .java or .class extension. If you get a message such as "Bad command or file name" or "javac: command not found", then go back and double-check your installation, in particular the execution path setting. If javac reports an error "cannot read: Welcome.java", then you should check whether that file is present in the directory. Under UNIX, check that you used the correct capitalization for Welcome.java. Under Windows, use the dir shell command, not the graphical Explorer tool. Some text editors (in particular Notepad) insist on adding an extension .txt after every file. If you use Notepad to edit Welcome.java, then it actually saves it as Welcome.java.txt. Under the default Windows settings, Explorer conspires with Notepad and hides the .txt extension because it belongs to a "known file type." In that case, you need to rename the file, using the ren shell command, or save it again, placing quotes around the file name: "Welcome.java". If you launch your program and get an error message complaining about a java.lang.NoClassDefFoundError, then carefully check the name of the offending class. If you get a complaint about welcome (with a lowercase w), then you should reissue the java Welcome command with an uppercase W. As always, case matters in Java. If you get a complaint about Welcome/java, then you accidentally typed java Welcome.java. Reissue the command as java Welcome. If you typed java Welcome and the virtual machine can't find the Welcome class, then check if someone has set the class path on your system. For simple programs, it is best to unset it. You can unset the class path in the current shell window by typing set CLASSPATH=
This command works on Windows and UNIX/Linux with the C shell. On UNIX/Linux with the Bourne/bash shell, use export CLASSPATH=
Chapter 4 tells you how you can permanently set or unset your class path. If you get an error message about a new language construct, make sure that your compiler supports JDK 5.0. If you cannot use JDK 5.0 or later, you need to modify the source code, as shown in Appendix B. If you have too many errors in your program, then all the error messages fly by very quickly. The compiler sends the error messages to the standard error stream, so it's a bit tricky to capture them if they fill more than the window can display. On a UNIX system or a Windows NT/2000/XP system, capturing error messages is not a big problem. You can use the 2> shell operator to redirect the errors to a file: javac MyProg.java 2> errors.txt Under Windows 95/98/Me, you cannot redirect the standard error stream from the shell window. You can download the errout program from http://www.horstmann.com/corejava/faq.html and run errout javac MyProg.java > errors.txt TIP  | The excellent tutorial at http://java.sun.com/docs/books/tutorial/getStarted/cupojava/ goes into much greater detail about the "gotchas" that beginners can run into. |
|