One of the powerful new features of Windows Forms is the consolidated Data Sources panel. This panel shows you a list of available data sources that can then be dragged onto any form in the application. For example, if you have created a DataSet, that DataSet will appear in the Data Sources panel and you can then drag it onto the surface of a form, creating either a grid view or a details view. One of the types of data sources is a web service. This section of the chapter walks you though building a web service that returns data through a web method. The schema of this data is then interpreted as a data source by the Windows Forms client, allowing that client to bind directly to data returned by a web service. The following steps will walk you through the process of using a web service as a data source. While reading these steps, it is important to keep in mind a very important distinction: The web service itself is not the data sourceonly the data types returned from that service are considered data sources. In other words, a web service data source will not automatically invoke methods; it only obtains the schema from the web service and uses that schema to prepare the data source. The following steps will take you through the process of binding to a web service: 1. | Create a new ASP.NET web service application by selecting File, then New, then Website from within Visual Studio 2005. You can call the service anything you like.
| 2. | Add a class called Customer to the App_Code directory within the service. The Customer class should have a few basic public string fields, such as FirstName, LastName, and CustomerID.
| | | 3. | Add a method to the service called GetAllCustomers() with the following code:
[WebMethod(Description="Retrieve all Customers")] public List<Customer> GetAllCustomers() { List<Customer> custList = new List<Customer>(); custList.Add(new Customer("Kevin", "Hoffman", "customer001")); custList.Add(new Customer("John", "Doe", "customer002")); return custList; } | 4. | Add a new Windows Forms project to the solution called WSBindingClient. Do not add a web reference to the new project at this point.
| 5. | Open the Data Sources pane and click the Add New Data Source button.
| 6. | Select Web Service as the data source type and browse the current solution for web services.
| 7. | Select the "Service" service from the provided list.
| 8. | Click the Add Reference button. You will see a confirmation dialog that indicates that all of the objects returned by the web service will be added to the Data Sources window. Confirm this by clicking Finish.
| 9. | Figure 33.5 shows what your Data Sources window should look like after completing step 8.
Figure 33.5. The Data Sources window after adding a web service data source. 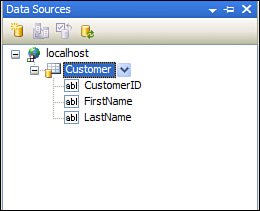 | 10. | Drag the Customer item from the Data Sources window onto the main form.
| 11. | Switch to the code view beneath the form and add the following two lines of code below the call to InitializeComponent(); in the form's constructor:
localhost.Service svc = new localhost.Service(); customerBindingSource.DataSource = svc.GetAllCustomers(); | 12. | Run the application. You should see a DataGridView that contains the two rows of data provided by the web service, as shown in Figure 33.6.
| Figure 33.6. A DataGridView populated by a web service. 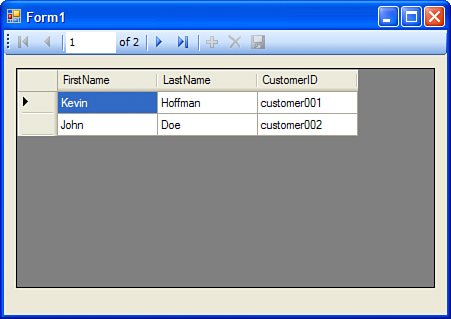 Unlike other kinds of data sources that can be automatically updated by the controls to which they are bound, you will have to write your own code to invoke the appropriate update methods on the web service because there is no way for the data source to know which methods on which web service to invoke in response to data change events. However, even with that minor bit of required coding, the ability to bind controls to data retrieved directly from web services is a powerful tool, especially for smart client applications and SOA infrastructures. |