Projects are where all of the real work is performed in Visual Studio. A project maps directly to a compiled component. Visual Studio supports a variety of different project types. Let's reexamine the project creation process. Creating a Project As we saw earlier when discussing solution creation, you create projects by selecting the New, Project option from the File menu. This launches the New Project dialog box (see Figure 4.10). Figure 4.10. Adding a project to the current solution. Table 4.2 shows the various project types supported in Visual Studio out of the box. Table 4.2. Supported Project TypesType | Language | Template |
---|
Database | Visual Basic, Visual C# | SQL Server project | Office | Visual Basic, Visual C# | Excel workbook | | | Excel template | | | Word document | | | Word template | Smart Device | Visual Basic, Visual C# | Pocket PC 2003 Device application | | | Pocket PC 2003 Control library | | | Pocket PC 2003 Empty project | | | Pocket PC 2003 Class library | | | Pocket PC 2003 Console application | | | Smartphone 2003 Device application | | | Smartphone 2003 Console application | | | Smartphone 2003 Class library | | | Smartphone 2003 Empty project | | | Windows CE 5.0 Device application | | | Windows CE 5.0 Control project | | | Windows CE 5.0 Empty project | | | Windows CE 5.0 Class library | | | Windows CE 5.0 Console application | | Visual C++ | ATL Smart Device project | | | MFC Smart Device project | | | Win32 Smart Device project | | | MFC Smart Device ActiveX control | | | MFC Smart Device DLL | Starter Kits | Visual Basic, Visual C# | Screen Saver Starter Kit | | | Movie Collection Starter Kit | | Visual J# | Calculator Starter Kit | Test | Visual Basic, Visual C# | Test project | Windows | Visual Basic, Visual C#, Visual J# | Windows application | | | Windows control library | | | Console application | | | Empty project | | | Class library | | | Web control library | | | Windows service | | | Crystal Reports Application |
Note Visual Studio supports the ability to create new project types and templates. In fact, this is one easy way to enforce standards and propagate usage guidance within a development team. For more details, see Chapter 17, "Web Services and Visual Studio." As outlined previously, creating a new project will also create a new containing solution. However, if you are creating a project and you already have a solution loaded in the IDE, the New Project dialog box will offer you the opportunity to add the new project to the existing solution. Compare Figure 4.10 with Figure 4.2; notice that there is a new option in the form of a drop-down box that allows you to indicate whether Visual Studio should create a new solution or add the project to the current solution. Website Projects Website projects are created in a slightly different way. Instead of selecting File, New, Project, you select File, New, Web Site. This launches the New Web Site dialog box (see Figure 4.11). Figure 4.11. Creating a new website project. As with other project types, you initiate website projects by selecting one of the predefined templates. In addition to the template, you also select a target source language and the location for the website. The location can be the file system, an HTTP site, or an FTP site. Unlike other project types, websites are not typically created within the physical folder tree that houses your solution. Even selecting the file system object will, by default, place the resulting source files in a Web Sites folder under the Visual Studio 2005 projects folder. Note The target source language for a website project simply represents the default language used for any code files. It does not constrain the languages you can use within the project. For instance, a website project created with C# as the target language can still contain Visual Basic code files. After you have created the website, you manage and maintain it just like the other project types within the IDE. Working with Project Definition Files As with solutions, projects also maintain their structure information inside a file. These files have different extensions depending on their underlying language. Table 4.3 shows the various extensions that Visual Studio uses to identify project files. Table 4.3. Project File ExtensionsProject File Extension | Language |
---|
.vbproj | Visual Basic | .csproj | Visual C# | .vjsproj | Visual J# | .vcproj | Visual C++ |
Each project definition file contains all of the information necessary to describe the source files and the various project properties and options. This includes Visual Basic, Visual C#, and Visual J# project definition files are based on the same schema. Listing 4.2 contains a snippet from a Visual C# project definition file: Listing 4.2. Contents of a Visual C# Project Definition File <Project DefaultTargets="Build" xmlns="http://schemas.microsoft.com/developer/msbuild/2003"> <PropertyGroup> <Configuration Condition=" '$(Configuration)' == '' ">Debug</Configuration> <Platform Condition=" '$(Platform)' == '' ">AnyCPU</Platform> <ProductVersion>8.0.50215</ProductVersion> <SchemaVersion>2.0</SchemaVersion> <ProjectGuid>{E22301F1-5AD6-4514-A05D-266158AB1CAB}</ProjectGuid> <OutputType>WinExe</OutputType> <AppDesignerFolder>Properties</AppDesignerFolder> <RootNamespace>WindowsApplication2</RootNamespace> <AssemblyName>WindowsApplication2</AssemblyName> </PropertyGroup> <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Debug|AnyCPU' "> <DebugSymbols>true</DebugSymbols> <DebugType>full</DebugType> <Optimize>false</Optimize> <OutputPath>bin\Debug\</OutputPath> <DefineConstants>DEBUG;TRACE</DefineConstants> <ErrorReport>prompt</ErrorReport> <WarningLevel>4</WarningLevel> </PropertyGroup> <PropertyGroup Condition=" '$(Configuration)|$(Platform)' == 'Release|AnyCPU' "> <DebugType>pdbonly</DebugType> <Optimize>true</Optimize> <OutputPath>bin\Release\</OutputPath> <DefineConstants>TRACE</DefineConstants> <ErrorReport>prompt</ErrorReport> <WarningLevel>4</WarningLevel> </PropertyGroup> <ItemGroup> <Reference Include="System" /> <Reference Include="System.Data" /> <Reference Include="System.Deployment" /> <Reference Include="System.Drawing" /> <Reference Include="System.Windows.Forms" /> <Reference Include="System.Xml" /> </ItemGroup> <ItemGroup> <Compile Include="Form1.cs"> <SubType>Form</SubType> </Compile> <Compile Include="Form1.Designer.cs"> <DependentUpon>Form1.cs</DependentUpon> </Compile> <Compile Include="Program.cs" /> <Compile Include="Properties\AssemblyInfo.cs" /> <EmbeddedResource Include="Properties\Resources.resx"> <Generator>ResXFileCodeGenerator</Generator> <LastGenOutput>Resources.Designer.cs</LastGenOutput> <SubType>Designer</SubType> </EmbeddedResource> <Compile Include="Properties\Resources.Designer.cs"> <AutoGen>True</AutoGen> <DependentUpon>Resources.resx</DependentUpon> </Compile> <None Include="Properties\Settings.settings"> <Generator>SettingsSingleFileGenerator</Generator> <LastGenOutput>Settings.Designer.cs</LastGenOutput> </None> <Compile Include="Properties\Settings.Designer.cs"> <AutoGen>True</AutoGen> <DependentUpon>Settings.settings</DependentUpon> <DesignTimeSharedInput>True</DesignTimeSharedInput> </Compile> </ItemGroup> <Import Project="$(MSBuildBinPath)\Microsoft.CSharp.targets" /> </Project> | This project definition file would look relatively the same as a Visual Basic or Visual J# project. Visual C++ project files, however, use an entirely different schema. For completeness, and to contrast with the Visual Basic/Visual C#/Visual J# content, Listing 4.3 shows a sample Visual C++ project definition file in its entirety. Listing 4.3. Visual C++ Project Definition File <?xml version="1.0" encoding="Windows-1252"?> <VisualStudioProject ProjectType="Visual C++" Version="8.00" Name="Contoso.Fx.UI.BrowserShim" ProjectGU RootNamespace="ContosoFxUIBrowserShim" Keyword="MFCActiveXProj" SignManifests="true"> <Platforms> <Platform Name="Win32" /> </Platforms> <ToolFiles> </ToolFiles> <Configurations> <Configuration Name="Debug|Win32" OutputDirectory="Debug" IntermediateDirectory="Debug" ConfigurationType="2" UseOfMFC="2" CharacterSet="1"> <Tool Name="VCPreBuildEventTool" /> <Tool Name="VCCustomBuildTool" /> <Tool Name="VCXMLDataGeneratorTool" /> <Tool Name="VCWebServiceProxyGeneratorTool" /> <Tool Name="VCMIDLTool" PreprocessorDefinitions="_DEBUG" MkTypLibCompatible="false" TypeLibraryName="$(IntDir)/$(ProjectName).tlb" HeaderFileName="$(ProjectName)idl.h" ValidateParameters="false" /> <Tool Name="VCCLCompilerTool" Optimization="0" PreprocessorDefinitions="WIN32;_WINDOWS;_DEBUG;_USRDLL" MinimalRebuild="true" BasicRuntimeChecks="3" RuntimeLibrary="3" TreatWChar_tAsBuiltInType="true" UsePrecompiledHeader="2" WarningLevel="3" Detect64BitPortabilityProblems="true" DebugInformationFormat="4" /> <Tool Name="VCManagedResourceCompilerTool" /> <Tool Name="VCResourceCompilerTool" PreprocessorDefinitions="_DEBUG" Culture="1033" AdditionalIncludeDirectories="$(IntDir)" /> <Tool Name="VCPreLinkEventTool" /> <Tool Name="VCLinkerTool" RegisterOutput="true" OutputFile="$(OutDir)\$(ProjectName).ocx" LinkIncremental="2" ModuleDefinitionFile=".\Contoso.Fx.UI.BrowserShim.def" GenerateDebugInformation="true" SubSystem="2" TargetMachine="1" /> <Tool Name="VCALinkTool" /> <Tool Name="VCManifestTool" /> <Tool Name="VCXDCMakeTool" /> <Tool Name="VCBscMakeTool" /> <Tool Name="VCFxCopTool" /> <Tool Name="VCAppVerifierTool" /> <Tool Name="VCWebDeploymentTool" /> <Tool Name="VCPostBuildEventTool" /> </Configuration> <Configuration Name="Release|Win32" OutputDirectory="Release" IntermediateDirectory="Release" ConfigurationType="2" UseOfMFC="2" CharacterSet="1" > <Tool Name="VCPreBuildEventTool" /> <Tool Name="VCCustomBuildTool" /> <Tool Name="VCXMLDataGeneratorTool" /> <Tool Name="VCWebServiceProxyGeneratorTool" /> <Tool Name="VCMIDLTool" PreprocessorDefinitions="NDEBUG" MkTypLibCompatible="false" TypeLibraryName="$(IntDir)/$(ProjectName).tlb" HeaderFileName="$(ProjectName)idl.h" ValidateParameters="false" /> <Tool Name="VCCLCompilerTool" Optimization="2" PreprocessorDefinitions="WIN32;_WINDOWS;NDEBUG;_USRDLL" MinimalRebuild="false" RuntimeLibrary="2" TreatWChar_tAsBuiltInType="true" UsePrecompiledHeader="2" WarningLevel="3" Detect64BitPortabilityProblems="true" DebugInformationFormat="3" /> <Tool Name="VCManagedResourceCompilerTool" /> <Tool Name="VCResourceCompilerTool" PreprocessorDefinitions="NDEBUG" Culture="1033" AdditionalIncludeDirectories="$(IntDir)" /> <Tool Name="VCPreLinkEventTool" /> <Tool Name="VCLinkerTool" RegisterOutput="true" OutputFile="$(OutDir)\$(ProjectName).ocx" LinkIncremental="1" ModuleDefinitionFile=".\Contoso.Fx.UI.BrowserShim.def" GenerateDebugInformation="true" SubSystem="2" OptimizeReferences="2" EnableCOMDATFolding="2" TargetMachine="1" /> <Tool Name="VCALinkTool" /> <Tool Name="VCManifestTool" /> <Tool Name="VCXDCMakeTool" /> <Tool Name="VCBscMakeTool" /> <Tool Name="VCFxCopTool" /> <Tool Name="VCAppVerifierTool" /> <Tool Name="VCWebDeploymentTool" /> <Tool Name="VCPostBuildEventTool" /> </Configuration> </Configurations> <References></References> <Files> <Filter Name="Source Files" Filter="cpp;c;cc;cxx;def;odl;idl;hpj;bat;asm;asmx" UniqueIdentifier="{4FC737F1-C7A5-4376-A066-2A32D752A2FF}"> <File RelativePath=".\Contoso.Fx.UI.BrowserShim.cpp"></File> <File RelativePath=".\Contoso.Fx.UI.BrowserShim.def"></File> <File RelativePath=".\Contoso.Fx.UI.BrowserShim.idl"></File> <File RelativePath=".\Contoso.Fx.UI.BrowserShimCtrl.cpp"> </File> <File RelativePath=".\Contoso.Fx.UI.BrowserShimPropPage.cpp"> </File> <File RelativePath=".\stdafx.cpp"> <FileConfiguration Name="Debug|Win32" > <Tool Name="VCCLCompilerTool" UsePrecompiledHeader="1" /> </FileConfiguration> <FileConfiguration Name="Release|Win32"> <Tool Name="VCCLCompilerTool" UsePrecompiledHeader="1" /> </FileConfiguration> </File> </Filter> <Filter Name="Header Files" Filter="h;hpp;hxx;hm;inl;inc;xsd" UniqueIdentifier="{93995380-89BD-4b04-88EB-625FBE52EBFB}"> <File RelativePath=".\Contoso.Fx.UI.BrowserShim.h"></File> <File RelativePath=".\Contoso.Fx.UI.BrowserShimCtrl.h"></File> <File RelativePath=".\Contoso.Fx.UI.BrowserShimPropPage.h"> </File> <File RelativePath=".\Resource.h"></File> <File RelativePath=".\stdafx.h"></File> </Filter> <Filter Name="Resource Files" Filter="rc;ico;cur;bmp;dlg;rc2;rct;bin;rgs;gif;jpg;jpeg;jpe;resx;tiff;tif;png;wav" UniqueIdentifier="{67DA6AB6-F800-4c08-8B7A-83BB121AAD01}"> <File RelativePath=".\Contoso.Fx.UI.BrowserShim.ico"></File> <File RelativePath=".\Contoso.Fx.UI.BrowserShim.rc"></File> <File RelativePath=".\Contoso.Fx.UI.BrowserShimCtrl.bmp"></File> </Filter> <File RelativePath=".\ReadMe.txt"></File> </Files> <Globals></Globals> </VisualStudioProject> | Working with Projects As source code containers, projects principally act as a settings applicator. They are used to control and organize your source code files and the various properties associated with the whole build and compile process (we cover the build process in depth in Chapter 9). As with solutions, projects can contain various different items that are germane to their development. Projects are language specific. You cannot mix different languages within a specific project. There is no similar limitation with solutions: A solution can contain many projects, each one in a different language. Project Items After a project is created, it will, by default, already contain one or more project items. These default items will vary depending on the project template you selected and on the language of the project. For instance, creating a project using the C# windows application template would result in the formation of a Form1.cs file, a Form1.Designer.cs file, and a Program.cs file. Projects are also preconfigured with references and properties that make sense for the given project type: The windows application template contains a reference to the System.Windows.Forms assembly, whereas the class library template does not. Projects, like solutions, can also have subfolders within them that you can employ to better manage and group project items. Unlike solutions, the folders that you create within a project are physical; they are created on disk within your project directory structure. These are examples of physical project items. Source code files are also physical in nature. Projects can also contain virtual itemsitems that are merely pointers or links to items that don't actually manifest themselves physically within your project structure. They are, for example, references to other assemblies, database connections, and virtual folders (virtual folders are described in Chapter 5). Figure 4.12 illustrates a fully described solution and project. Figure 4.12. Project structure. 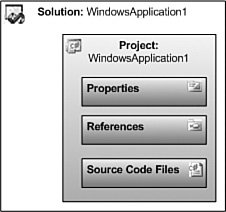 Project Properties Like solution properties, project properties are viewed and set using a series of property pages accessed through the Project, Properties menu. These property pages are hosted within a dialog box referred to as the Project Designer. Figure 4.13 shows the Project Designer that is displayed for a sample Visual Basic class library project. Different languages and different project types will actually surface different property pages within the Project Designer. For instance, the Application property page for a Visual Basic project looks different and contains slightly different information than an identical Visual C# project (although the basic intent of the page remains unchanged). Figure 4.13. Setting properties using the Project Designer. In general, you use project properties to control the following: General project attributes such as the assembly name and project type The way that the project will be built/compiled Debugger configuration for the project Resources used by the project Signing and security settings Let's examine each of the available project property pages and discuss briefly the options that can be set on each. Application The Application property page allows you to set the assembly name, default/root namespace, application/output type, and the startup object. For Windows forms applications, authentication modes and visual styles are also controlled via this property page. Assembly name This is the filename of the assembly that the project is compiled into. Typically, it defaults to the project name. The extension used is determined by the output type of the project. Root/Default namespace This specifies a namespace to be used by any types declared within the project. This can also be declared manually in code. Output type Displayed as the application type for Visual Basic projects, this value determines the fundamental project type (for example, class library, windows application, console application). Startup object This object is used to set the entry point for the project. For windows applications, this will be the default form (or in the case of C#, the program entry point for the form) that should be launched when the application is executed. For console applications, this will be the main subroutine procedure that implements the console. Class library projects do not have an entry point and will be set to (Not set). Icon This is the icon to associate with the assembly. It is not pertinent to class library or web projects. Resource File This text box can be used to specify a path and filename for a resource file. Resource files contain nonexecutable content, such as strings, images, or even persisted objects, that need to be deployed along with an application. Visual styles The Enable XP Visual Styles check box allows you to indicate whether the application will support XP themes when the user interface is rendered. This option is not applicable for non-Windows application projects. Windows application properties Visual Basic provides a series of properties that apply specifically to Windows application projects. These properties allow you to set the splash screen associated with the project, the authentication mode supported by the project (Windows or application-defined), and the shutdown mode of the project. The shutdown mode specifies whether the application should shut down when the initial form is closed or when the last loaded form in the application is closed. Build The Build property page is used with Visual C# and Visual J# projects to tweak settings associated with build configurations. Using this dialog box, you can select whether the DEBUG and TRACE constants are turned on, and you can specify conditional compilation symbols. Settings that affect the warning and error levels and the build output are also housed here. For more exploration of the options available here, see Chapter 9. Build Events Visual Studio will trigger a pre- and post-build event for each project. On the Build Events page, you can specify commands that should be run during either of these events. This page also allows you to indicate when the post-build event runs: always, after a successful build, or when the build updates the project output. Build events are particularly useful for launching system tests and unit tests against a project that has just been recompiled. If you launch a suite of, say, unit tests from within the post-build event, the test cycle can be embedded within the build cycle. Note If you specify commands in the pre- or post-build events, Visual Studio will create a batch file for each event and place it into the bin/debug directory. These files, titled PreBuildEvent.bat and PostBuildEvent.bat, will house the commands you enter on the Build Events property page. In the event of an error running the build event commands, you can manually inspect and run these files to try to chase down the bug. Code Analysis Visual Studio offers the capability to run a series of rules and checks against your code base to enforce best practices, catch common errors, and apply intelligent coding guidance across a variety of topics such as performance, security, and globalization (to name just a few). The Code Analysis property page enables you to turn on code analysis for the current project, and it allows you to select which rules you want to run during the analysis process. Compile (VB Only) The Compile property page is used by Visual Basic projects to control which optimizations will be performed during compile and also to control general compilation options for the output path and warnings versus errors raised during the compilation process. Compile options You use the Option strict and Option explicit drop-down to turn on or off these settings. You can also control whether the project will perform binary or text compares with the Option compare drop-down. Compiler conditions Visual Basic allows you to customize the level of notification provided upon detecting any of a handful of conditions during the compilation process. For instance, one condition defined is Unused Local Variable. If this condition is detected in the source code during the compile, you can elect to have it treated as a warning or an error, or to have it ignored altogether. Build events Visual Basic allows you to access the Build Events property page (see the previous section for an explanation) via a Build Events button located on this screen. Misc compile options You can choose to disable all compiler warnings, treat all warnings as errors, and generate an XML documentation file during the compile process. This will result in an XML file with the same name as the project; it will contain all of the code comments parsed out of your source code in a predefined format. Debug The Debug property page allows you to affect the behavior of the Visual Studio debugger. Start action You use this option to specify whether a custom program, a URL, or the current project itself should be started when the debugger is launched. Start options You use this option to specify command-line arguments to pass to the running project, set the working directory for the project, and debug a process on a remote machine. Enable debuggers You use the check boxes in this section to enable or disable such things as support for debugging unmanaged code, support for SQL stored procedure debugging, and use of Visual Studio as a host for the debugger process. Publish The Publish property page enables you to configure many ClickOnce-specific properties. You can specify the publish location for the application, the install location (if different from the publish location), and the various installation settings, including prerequisites and update options. You can also control the versioning scheme for the published assemblies. References (Visual Basic) The References property page is used within Visual Basic projects to select the assemblies referenced by the project and to import namespaces into the project. This screen also allows you to query the project in an attempt to determine whether some existing references are unused. You do this by using the Unused References button. Reference Paths (Visual C#, Visual J#) The Reference Paths property page allows you to provide path information meant to help Visual Studio find assemblies referenced by the project. Visual Studio will first attempt to resolve assembly references by looking in the current project directory. If the assembly is not found there, the paths provided on this property page will be used to search for the assemblies. Visual Studio will also probe the project's obj directory, but only after attempting to resolve first using the reference paths you have specified on this screen. Resources Resources are items such as strings, images, icons, audio, and files that are embedded in a project and used during design and runtime. The Resources property page allows you to add, edit, and delete resources associated with the project. Security For ClickOnce applications, the Security property page allows you to enforce code access security permissions for running the ClickOnce application. Various full trust and partial trust scenarios are supported. Settings Application settings are dynamically specified name/value pairs that can be used to store information specific to your project/application. The Settings property page allows you to add, edit, and delete these name/value pairs. Each setting can be automatically scoped to the application or to the user, and can have a default value specified. Applications can then consume these settings at runtime. Signing The Signing property page allows you to have Visual Studio code sign the project assemblyand its ClickOnce manifestsby specifying a key file. You can also enable Delay signing from this screen. |