Just as it is necessary to control your application's processing, it is necessary to configure your application's settings. This includes handling how pages are displayed, how they are compiled, controlling access to the parts of your application, and so on. As you'll see in a moment, ASP.NET contains a lot of configurable parts, and it takes an elegant system to manage all those settings gracefully. ASP.NET provides a hierarchical configuration system that is very extensible and, best of all, text-based, meaning that developers can access and modify the configuration remotely.  | With hierarchical configuration, the application configuration information is applied according to the virtual directory structure of your Web site. Subdirectories can inherit or override configuration options from their parent directories. In fact, all directories inherit from a default system configuration file, machine.config, typically located in the WinNT\Microsoft.NET\Framework\<version>\CONFIG directory. This provides you a lot of flexibility when designing your applications. | This robust system is implemented with the web.config file, an XML text-based file. Let's examine it further. web.config I've mentioned the web.config file quite a few times throughout this book. So far, you know that it holds information used by the application to control its behavior. There is nothing special about this file; it simply holds keys and values recognized by ASP.NET. These values are easily modifiable, and, in fact, you can add your own custom keys and values to control other settings that ASP.NET doesn't handle for you (more on that later). As you learned earlier, the ASP.NET configuration system is hierarchical. In terms of the web.config file, this means that the settings you supply in each web.config file apply only to the directory that contains the file, and those below it. So, if you want to provide settings for your entire application, simply place this file in your root application folder. If a subdirectory has special application needs (such as disallowing unauthorized users), simply create another web.config with the new settings. The subdirectory will inherit its parent's settings, overriding those that are duplicated. However, the hierarchical system is based on the virtual directory path, and not the actual physical path. To grasp this concept, imagine a Web site with the directory structure shown in Figure 18.4. Figure 18.4. A sample directory structure.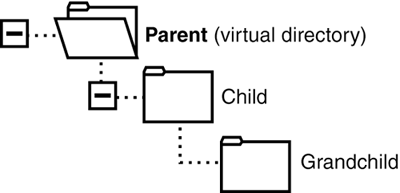 Let's assume that Parent is the only virtual directory. Parent, Child, and Grandchild all inherit the system-level web.config settings. The Parent directory can override or add settings with its own local web.config file. If a browser accesses Child with the URL http://localhost/Parent/Child, that directory will also inherit Parent's settings, as will Grandchild. The two subdirectories are also able to override any of Parent's settings with their own web.config files. Now let's assume that Child is also a virtual directory, and can be accessed as http://localhost/Child. Because web.config settings are processed according to virtual directories (and not physical paths), it will not inherit any of Parent's settings. Grandchild will now inherit different settings depending on how it is accessed. http://localhost/Parent/Child/Grandchild might produce different settings than http://localhost/Child/Grandchild, so be careful how you structure your virtual directories! Like the global.asax file, ASP.NET also prevents the web.config file from being accessed via a Web client. If you try to get at this file, the browser will return the same error shown in Figure 18.2. This is quite helpful for stopping prying eyes from breaking into your application. Changes to this file are automatically detected and, like the global.asax file, the application is automatically restarted for the new settings to take effect. Enough theory, let's take a look at this file! The basic format of the web.config file is simple: <configuration> <configSections> <!-- Handlers declared here --> </configSections> <system.web> <!-- ASP.NET Configuration Settings Would Go Here --> </system.web> <system.net> <!-- .NET Runtime Configuration Settings Would Go Here --> </system.net> </configuration> No script blocks or HTML code, just pure XML. Inside the <configuration> tags are typically two different elements: configuration section handlers and configuration section settings. The first section declares the types of data in the web.config file, and the second contains the actual key/value pairs of settings. Configuration section handlers, denoted by the <configSections> element, process the XML data contained in web.config and return an appropriate object based on this data; you must specify these handlers so that ASP.NET knows what type of configuration data you have. For example, if a setting named "XmlFiles" contained a list of XML files, you would have a corresponding configuration section handler named "XmlFiles" that would return, for instance, an XmlTextReader object to your ASP.NET page when called. Luckily, ASP.NET already provides quite a few section handlers in the default machine.config file, so you don't have to declare these yourself. For example, Listing 18.3 shows a portion of the default configuration section for the system.web section and its subsections. Listing 18.3 Sample Configuration Section Handlers 1: <configuration> 2: <configSections> 3: <sectionGroup name="system.web"> 4: <section name="browserCaps" type="System.Web.Configuration. HttpCapabilitiesSectionHandler,System.Web" /> 5: <section name="clientTarget" type="System.Web.Configuration. ClientTargetSectionHandler,System.Web" /> 6: <section name="compilation" type="System.Web.UI. CompilationConfigurationHandler, System.Web" /> 7: <section name="pages" type="System.Web.UI.PagesConfigurationHandler, System.Web" /> 8: </sectionGroup> 9: </configSections> 10: </configuration> As you can see, this file becomes quite complex, so I won't go into depth here. Just know that each section defined by the name elements contains settings used by ASP.NET (you'll examine these settings in the next section). Refer to the .NET Framework SDK documentation for more information. Note Note the format of each element in this web.config file. In elements consisting of multiple words, for example, sectionGroup, the first letter of the first word is lowercase, whereas all subsequent first letters are capitalized. This is known as camel casing, and is standard for the web.config file. The web.config file is case sensitive, and without the proper casing, your application will generate errors. Configuration settings are the actual data that configure your application; the key/value pairs of information. There are, in turn, two types of these sections: system.net and system.web. The first section is for configuring the .NET runtime itself, so you won't bother much with that. The second is for controlling ASP.NET. All settings (with two exceptions, which I'll discuss in a moment) for your applications will be placed within the <system.web> tags. For example, Listing 18.4 shows a sample configuration file. Listing 18.4 Sample Configuration File 1: <configuration> 2: <system.web> 3: <trace 4: enabled="false" 5: requestLimit="10" 6: pageOutput="false" 7: traceMode="SortByTime" 8: localOnly="true" 9: /> 10: <globalization 11: requestEncoding="iso-8859-1" 12: responseEncoding="iso-8859-1" 13: /> 14: <customErrors mode="RemoteOnly" /> <!-- Possible values for mode: On, Off, RemoteOnly --> 15: </system.web> 16: </configuration>  | This listing contains three different settings for ASP.NET. The first, trace, on line 3, contains settings for the trace service of ASP.NET (you'll learn about that in Day 20. The second setting, on line 10, controls how your application sends output to the browser; you'll examine that tomorrow. The final section, customErrors, controls how errors are displayed in your application. | Note Again, note the camel casing of each element. Table 18.2 lists all the configuration settings available for use in web.config. I won't cover all of them in this book because some are used only rarely, but this table will provide a good reference. Table 18.2. web.config Configuration Settings Section Name | Description | <appSettings> | Used to store your own custom application settings. | <authentication> | Configures how ASP.NET authenticates users (see Day 21, "Securing Your ASP.NET Applications"). | <authorization> | Configures authorization of resources in ASP.NET (again, see Day 21). | <browserCaps> | Responsible for controlling the settings of the browser capabilities component. | <clientTarget> | Adds aliases for browser versions and names. | <compilation> | Responsible for all compilation settings used by ASP.NET. See Day 20 for more information. | <customErrors> | Tells ASP.NET how to display errors in the browser. | <globalization> | Responsible for configuring the globalization settings of an application (see Day 20). | <httpHandlers> | Responsible for mapping incoming URLs to IHttpHandler classes. Subdirectories do not inherit these settings. Also responsible for mapping incoming URLs to IHttpHandlerFactory classes. Data represented in <httpHandlerFactories> sections are hierarchically inherited by subdirectories. | <httpModules> | Responsible for configuring Http modules within an application. Http modules participate in the processing of every request into an application, and common uses include security and logging. | <httpRuntime> | Configures how your application runs for example, how many requests it can take and the maximum time a request is allowed to execute before it is shut down. | <identity> | Controls how ASP.NET accesses its resources (which identity it uses to access those resources). See Day 21. | <location> | A special tag that controls how settings apply to a directory in your application. | <machineKey> | Keys to use for encryption and decryption of cookie data. | <pages> | Controls page-specific configuration settings. | <processModel> | Configures the ASP.NET process model settings on IIS Web server systems. | <securityPolicy> | Maps security levels to policy files. | <sessionState> | Responsible for configuring session state. | <trace> | Responsible for configuring the ASP.NET trace service. | <trust> | The code access security level for an application. | <webServices> | Controls settings for Web services (see Days 16, "Creating Web Services," and 17, "Consuming and Securing XML Web Services"). | Configuration Sections Although you can define any configuration sections you choose, ASP.NET comes built in with several that might already serve your purposes. The following sections provide examples and syntax for the most commonly used configuration settings. Each section can support subelements, attributes, or both. Subelements are separate tags that are "below" the current tag, and can have their own subelements and attributes. Attributes define properties of the tag, such as the id attribute for server controls. Remember that each of these settings must be placed in the <system.web> configuration section, with two exceptions that we'll discuss when we come across them. <appSettings> This section allows you to define your own custom attributes for your ASP.NET application, such as database connection strings (see Day 8, "Beginning to Build Databases," for more information on those). It contains one subelement: add, which contains two attributes, key and value. This section is specifically designed to contain custom values; ASP.NET itself doesn't use the values here at all (unless, of course, you tell it to). The syntax is simple: <appSettings> <add key="ApplicationName" value="MyApp" /> <add key="DSN" value="Provider=Microsoft.Jet.OLEDB.4.0; "Data Source=c:\ASPNET\data\ banking.mdb"/> </appSettings> Accessing the values stored in <appSettings> is a bit different from accessing other configuration values; ASP.NET makes it easier to get these values. First, this tag does not have to be placed in the <system.web> section; it can reside directly under the <configuration> element. This is so that both ASP.NET applications and regular .NET applications can access the same values. Next, you don't have to use the GetConfig method, which we'll examine later today, to access these values. ASP.NET provides an easy property for this section, ConfigurationSettings.AppSettings. For example, using the previous <appSettings> section, the following Page_Load method would write "MyApp" to the browser: sub Page_Load(Sender as Object, e as EventArgs) Response.write(ConfigurationSettings.AppSettings _ ("ApplicationName")) end sub Simply supply the key name in parentheses (or brackets in C#) and you can easily retrieve the appropriate value. <browserCaps> This section contains many different elements and settings. It represents all the capabilities of the client browser, such as whether it is JavaScript capable, its browser version, and so on. We won't cover them all here. The syntax for this element is as follows: <configuration> <system.web> <browserCaps> browser="Unknown" version=0.0 majorver=0 minorver=0 frames=false tables=false ... </browserCaps> </system.web> </configuration> Refer to the .NET Framework SDK documentation to examine each property in more detail. <customErrors> This section allows you to define custom errors for your application or override built-in ASP.NET errors. For example, when a user tries to access a page that doesn't exist, he'll see a page similar to the one shown in Figure 18.5. Figure 18.5. A typical error page displayed when a user tries to request helloworld.aspx, which doesn't exist on the server.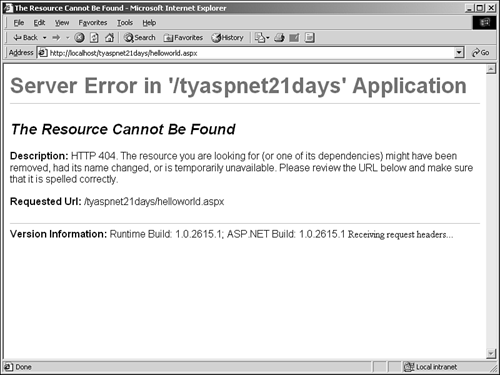 Although this page adequately informs the user of the error he has made, it might not fit into the design scheme of your site. Therefore, the <customErrors> element allows you to specify an alternative error page for this, and other errors. <customErrors> has two attributes: defaultRedirect, which specifies the URL the browser should go to if any error occurs; and mode, which specifies whether custom errors are enabled (On), disabled (Off), or shown only to remote clients (RemoteOnly). In other words, you won't see the custom errors when developing the application, but your end users will. This section also supports one subelement, error, which allows you to tailor handling for individual errors. For example, take a look at the following code: <customErrors defaultRedirect="error.htm" mode="On"> <error statusCode="404" redirect="error404.htm" /> </customerrors> This specifies that if a 404 error occurs (Resource Cannot Be Found, as shown in Figure 18.5), ASP.NET should redirect users to error404.htm. This file could contain a customized error message that fits in with your design scheme. If any other error occurs, this code tells ASP.NET to go to error.htm, which, again, could contain another more general error message. When custom error handling is on, and an error occurs, ASP.NET will pass a querystring parameter to the redirection page, aspxerrorpath, that contains the virtual path of the file that generated the error. For example, in the situation described earlier, when the user requests the file helloworld.aspx, the user would be redirected to the URL: http://localhost/tyaspnet21days/error404.htm?aspxerrorpath=/tyaspnet21days/helloworld.aspx This value can be used for a number of different reasons: You can inform the user of the invalid URL and instruct him to check the address or update his bookmarks, or even log this error to a database, which is useful for analyzing problems in your site later. <location> This is the second configuration section that does not require placement in the <system.web> section. Its purpose is to allow you to apply other settings to only a specific location on your Web server. It is most commonly used for security settings, so I'll discuss it more in depth in Day 21. Let's imagine a simple scenario. You have two pages in your site: default.aspx and members.aspx. The first is, obviously, the default page of your site, and anyone should be able to access it. The second should be accessible only by users who have registered and logged in to your site. Therefore, you want to apply security settings to only this one file. The code to do so would read as follows: <configuration> <location path="members.aspx"> <system.web> <authorization> <deny users="?"/> </authorization> </system.web> </location> </configuration> On the second line, you see the <location> tag with the path of the file to which the subsequent settings should apply; in this case, members.aspx (you can also specify directory names with this attribute). Don't worry about the <authorization> and <deny> tags you'll get to those in Day 21. Notice that inside the <location> tags, you must specify <system.web>; <location> itself isn't specific to ASP.NET or .NET, but any tags within usually are. Don't forget that the web.config file is hierarchical, so the <location> tag must also obey these rules. For example, take a look at the following code: <configuration> <location path="subdirectory1"> <system.web> <authorization> <deny users="?"/> </authorization> </system.web> </location> </configuration> The security settings would apply not only to the directory subdirectory1, but also to any subdirectories of that directory, and so on. <pages> This section allows you to control the page options that you would normally specify in page directives such as @ Page. The difference is that the settings in <pages> apply to all pages on the site, and not just one particular page. Let's look at the syntax: <configuration> <system.web> <pages buffer="true" enableSessionState="true" enableViewState="true" enableViewStateMac="true" autoEventWireup="true" smartNavigation="true" pageBaseType="TYASPNET, TYASPNET.dll" userControlBaseType="TYASPNET" </system.web> </configuration> This particular code snippet contains all the <pages> element's properties. It turns on buffering for all pages, turns on session state and viewstate for Macs as well as other machines (see Day 4, "Using ASP.NET Objects with C# and VB.NET," for information on buffering, session state, and viewstate), and turns on page events. Smart navigation enhances the user experience by persisting element focus and scroll bar position between pages, among other things. pageBaseType indicates which code-behind every ASP.NET page should inherit from by default (I'll explain code-behinds tomorrow). userControlBaseType specifies which code behind every user control should inherit from by default. This section is very useful if you need to make all your pages behave similarly. Be careful, however, because the default settings are usually sufficient; modifying these values for every single page could result in some repercussions in performance or possibly errors. You'll usually modify these settings on a per-page basis. <sessionState> ASP.NET provides many ways to customize session state. For instance, you can store session variables on a completely separate machine, in case the server crashes or needs to be replaced. This ensures that your session data is never lost. You can also store the session state in another process on the same system, which does something similar to what we just described. This section contains the following attributes: mode This attribute specifies where session information should be stored. Valid options are Off, which disables session state; InProc, which specifies that session information is stored locally (default); StateServer, session information is stored on another machine; and SQLServer, which stores information in a SQL Server database. cookieless Indicates whether cookieless session should be used (see Day 4 for more information on this topic and cookie munging). timeout The number of minutes of idle time before a session is abandoned by ASP.NET (default is 20 minutes). stateConnectionString The server name and port to store session information, when mode is set to StateServer; for example, "tcpip=127.0.0.1:42424". sqlConnectionString The connection string used to connect to the SQL server to store session information, when mode is set to SQLServer. See Day 10 for information on connection strings. The syntax is <configuration> <system.web> <sessionstate mode="InProc" cookieless="false" timeout="10" /> </system.web> </configuration> It might seem like a good idea to always store session information on another server, for safety reasons. However, when you use another server, performance suffers; ASP.NET must make connections over the network and communicate with a remote machine, which introduces serious lag times. Storing information InProc or on the same machine might not be as safe, but it is tremendously faster. Custom Configuration web.config is not limited to only the sections listed in Table 18.2 you can add any type of information you want to this file. What about the <appSettings> section? Doesn't that hold all the custom configuration information? You could, technically, put all custom information here, but you're limited to only key/value pairs here. When you use the ConfigurationSettings.AppSettings property (see the section on <appSettings> earlier today), ASP.NET creates a NameValueCollection object and populates it with the values from <appSettings>. This collection can easily be used to reference key/value pairs in this section. However, sometimes a NameValueCollection isn't suitable for your needs. For example, suppose that your application relies on an XML file that contains information on a library of books. It's easy enough to add this filename to the <appSettings> section like so: <appSettings> <add key="Library" value="c:\inetpub\wwwroot\tyaspnet21days\ day18\books.xml"/> </appSettings> When you retrieve this section from web.config, however, you'd rather receive an XmlTextReader, rather than a key/value pair describing the filename. ASP.NET allows you to build your own configuration sections, as well as their corresponding section handlers, which can return whatever type of object you want. Let's explore this situation a bit further. First, let's create a section handler that processes the input accordingly, and then build the section in web.config as well as an ASP.NET page to utilize it. Custom Section Handlers As described in the previous section, sometimes the built-in web.config section handlers aren't enough; they might not provide the functionality you need for your application. When this happens, you can easily create your own handlers to perform whatever actions are necessary. Let's take a look at an example, shown in Listings 18.5 and 18.6. Listing 18.5 A Custom web.config Section Handler Class in VB.NET 1: Imports System 2: Imports System.Configuration 3: Imports System.Xml 4: 5: Namespace TYASPNET.Handlers 6: Public Class LibrarySectionHandler : Implements IConfigurationSectionHandler 7: Public Function Create(parent as Object, configContext as Object, section as XmlNode) as Object Implements IConfigurationSectionHandler.Create 8: dim strFileName as String 9: strFileName = section.Attributes.Item(0).Value 10: 11: dim objReader as new XmlTextReader(strFileName) 12: return objReader 13: End Function 14: End Class 15: 16: End Namespace Listing 18.6 A Custom web.config Section Handler Class in C# 1: using System; 2: using System.Configuration; 3: using System.Xml; 4: 5: namespace TYASPNET.Handlers { 6: public class LibrarySectionHandler: IConfigurationSectionHandler { 7: object IConfigurationSectionHandler.Create (Object parent, Object configContext, XmlNode section) { 8: string strFileName; 9: strFileName = section.Attributes.Item(0).Value; 10: 11: XmlTextReader objReader = new XmlTextReader (strFileName); 12: return objReader; 13: } 14: } 15: }  | Save this file as LibrarySectionHandler.vb or LibrarySectionHandler.cs. Before you examine the code, let's take a brief overview of this class. A web.config file can declare this class as the handler for a configuration section, similar to Listing 18.3. Whenever ASP.NET accesses that section, typically through some code you've written, this class is created to handle the data contained in the section. In this case, the class reads a file path from web.config, creates an XmlTextReader from the path, and passes it to whoever requested it. The requester can then use this XmlTextReader directly. | As you can see, a custom section handler starts off as a VB.NET or C# class, just as a business object does. The difference is that this class must implement the IConfigurationSectionHandler interface, shown on line 6. An interface is a class that describes what methods and properties other classes should have; the IConfigurationSectionHandler tells you that your LibrarySectionHandler class must contain a Create method. Don't worry too much about interfaces; think of them simply as templates for other classes (see the .NET Framework SDK Documentation for more information). Also note the namespace to which this class belongs: TYASPNET.Handlers. On line 6, you declare your section handler class, LibrarySectionHandler (recall that the Implements keyword is omitted for C#). This class will be responsible for retrieving the aforementioned XML library filename from the web.config file, and sending the appropriate output to the ASP.NET page. Next, on line 7, you declare your Create method. Remember that IConfigurationSectionHandler already provided the template for this method, so you only have to follow it. You do this in VB.NET by again using the Implements keyword, and in C# by preceding the method name with the name of the interface and a period. The IConfigurationSectionHandler interface tells you that you need three parameters. The first is an Object that contains information about the parent of this web.config section. In other words, if this section inherited information from another web.config file, this first parameter would provide a link to the appropriate section in the other web.config file. You won't use this parameter here. The second parameter, configContext, is another Object that contains some contextual information; this parameter typically will never be used, so you'll ignore it also. The last parameter, section, is the most important. It contains the actual XML data from the web.config file. You'll use this parameter to parse the necessary information and spit out the appropriate data. The next part of the listing should be simple because you've already covered XML in ASP.NET in Day 11. Lines 8 and 9 retrieve the sole attribute from the custom section in web.config (I'll show that in a moment). This attribute will contain the path to the XML file. Lines 11 and 12 create an XmlTextReader from this file path, and return it to the calling application. It seems like an awful lot of work, but in reality, it's very simple. This listing was boilerplate, so you can cut-and-paste it over and over again, changing only the implementation of the Create method to suit your needs. With this listing, you can create an army of custom section handlers! Before you are through here, you need to do one more thing: Compile this class and place it in your assembly cache. Again, you should be very familiar with this process. Enter the following command from the command prompt: vbc /t:library /out:..\..\bin\TYASPNET.Handlers.dll /r:System.dll /r:System.xml.dll /r: System.web.dll LibrarySectionHandler.vb or: csc /t:library /out:..\..\bin\TYASPNET.Handlers.dll /r:System.dll /r:System.xml.dll /r: System.web.dll LibrarySectionHandler.cs This command assumes that your assembly cache (the /bin directory) is two below the current directory (where the LibrarySectionHandler.vb file resides). It creates a new assembly called TYASPNET.Handlers.dll that contains your new handler class. Now let's look at how to use this new handler. Creating and Retrieving Custom web.config Sections This first step to use our custom handler is to declare the appropriate section in the web.config file. This is very simple, as shown in Listing 18.7. Listing 18.7 The Custom web.config 1: <configuration> 2: <configSections> 3: <sectionGroup name="system.web"> 4: <section name="Library" type="TYASPNET.Handlers. LibrarySectionHandler, TYASPNET.Handlers" /> 5: </sectionGroup> 6: </configSections> 7: 8: <system.web> 9: <Library file="c:\inetpub\wwwroot\tyaspnet21days\ day11\books.xml" /> 10: </system.web> 11: </configuration>  | Save this file as web.config, and place it in the same directory as the LibrarySectionHandler.vb file. Recall that there are two parts of the web.config file: the configuration handler section and the configuration settings. The first is denoted by the <configSections> element, shown on line 2. Line 3 tells you that the handler applies to the <system.web> section; you could just have easily specified system.net or even left this element out. It simply serves as a way to group settings. | On line 4, you declare a new section named Library. The type attribute specifies what handler will be associated with this section. In this case, you're using the LibrarySectionHandler you just created. You must specify its full namespace name, followed by the assembly it belongs to (TYASPNET.Handlers.dll). Now you can create the actual settings for your application. On line 9, you have the Library section (declared on line 4) and an attribute file that specifies the path of the XML file (note that you're using an XML file from Day 11). You're done with the web.config file, so let's build the ASP.NET page, shown in Listing 18.8. Listing 18.8 Retrieving the Custom Configuration Settings 1: <%@ Page Language="VB" %> 2: <%@ Import Namespace="System.Xml" %> 3: 4: <script runat="server"> 5: sub Page_Load(Sender as Object, e as EventArgs) 6: 'retrieve information 7: dim i as integer 8: 9: dim objLibrary as XmlTextReader = _ 10: ConfigurationSettings.GetConfig ("system.web/Library") 11: 12: 'display information 13: While objLibrary.Read() 14: Select Case objLibrary.NodeType 15: Case XmlNodeType.Element 16: if objLibrary.HasAttributes then 17: for i = 0 to objLibrary.AttributeCount - 1 18: lblLibrary.Text += objLibrary. _ 19: GetAttribute(i) & " " 20: next 21: lblLibrary.Text += ", " 22: end if 23: Case XmlNodeType.Text 24: lblLibrary.Text += objLibrary.Value & "<br>" 25: End Select 26: End While 27: 28: objLibrary.Close 29: end sub 30: </script> 31: 32: <html><body> 33: <asp:Label runat="server"/> 34: </body></html>  | The only new part of this listing is on lines 9 10, so let's analyze those first. You retrieve the Library section from your web.config file with the GetConfig method. This method returns whatever object is specified by your custom handler. Recall from Listing 18.5 that your handler returned an XmlTextReader. Note that the GetConfig method must specify the path of the section to retrieve. Because your Library section was embedded in the <system.web> element, you must call the method appropriately: | GetConfig("system.web/Library") Remember that web.config is case sensitive, so make sure that your capitalization is correct! Lines 12 28 simply parse the returned XmlTextReader and display the data in the label on line 33. Refer to Day 11 for more information on this process. This listing will produce the output shown in Figure 18.6. Figure 18.6. The web.config Information is parsed and returned to you as an XmlTextReader.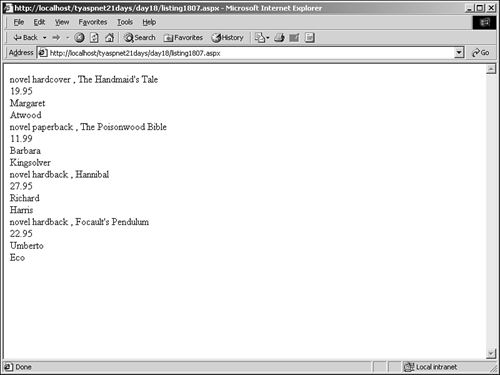 Let's summarize. You could have placed the XML file path in the <appSettings> section of web.config, and then retrieved it from your ASP.NET page using the ConfigurationSettings.AppSettings property. Then, the ASP.NET page would have to create an XmlTextReader itself, and finally display the data. However, by building a custom section handler, your ASP.NET pages do not need to worry about parsing the data in web.config and creating an XmlTextReader; the custom handler does that for you when you call the GetConfig method. All that is left for the ASP.NET pages to do is to display the data. Depending on how many ASP.NET pages use this XML file, you might have saved yourself quite a bit of extra work. |