You can also create a Web service from an existing business object so that you don't need to rewrite all the functionality. However, you must modify the existing object slightly to enable service support. Let's modify the database business object you created in yesterday's lesson, "Using Business Objects." You need to do three things to turn this into a Web service: inherit from the System.Web.Services.WebService class, add the WebMethod attribute for the methods you want to expose, and change the OleDbDataReader into a DataSet because the former isn't transferable via XML (more on that later). Listing 16.4 shows this modified class, which is renamed DatabaseService. Tip You can leave the database class as-is and simply create a copy of the class in the same file with a slightly different name. (For instance, append the word "service" to the end.) Only the class that inherits from WebService will be exposed as a service. This allows you to create multiple versions of an object in one file! Listing 16.4 Modifying Your Database Business Object for Use as a Service 1: Imports System 2: Imports System.Data 3: Imports System.Data.OleDb 4: Imports System.Web.Services 5: 6: Namespace TYASPNET 7: 8: Public Class DatabaseService : Inherits WebService 9: private objConn as OleDbConnection 10: private objCmd as OleDbCommand 11 12: <WebMethod()> public function SelectSQL(strSelect as _ 13: string) as DataSet 14: try 15: objConn = new OleDbConnection("Provider=" & _ 16: "Microsoft.Jet.OLEDB.4.0;" & _ 17: "Data Source=c:\ASPNET\data\banking.mdb") 18: dim objDataCmd as OleDbDataAdapter = new _ 19: OleDbDataAdapter(strSelect, objConn) 20: 21: Dim objDS as new DataSet 22: objDataCmd.Fill(objDS, "tblUsers") 23: return objDS 24: catch ex as OleDbException 25: return nothing 26: end try 27: end function 28: 29: <WebMethod()> public function ExecuteNonQuery _ 30: (strQuery as string) as Boolean 31: try 32: objConn = new OleDbConnection("Provider=" & _ 33: "Microsoft.Jet.OLEDB.4.0;" & _ 34: "Data Source=c:\ASPNET\data\banking.mdb") 35: objCmd = new OleDbCommand(strQuery, objConn) 36: objCmd.Connection.Open() 37: objCmd.ExecuteNonQuery 38: objCmd.Connection.Close() 39: return true 40: catch ex as OleDbException 41: return false 42: end try 43: end function 44: 45: End Class 46: 47: End Namespace  | Save this file as DatabaseService.vb. On line 4, you import the additional namespace, System.Web.Services. In your class declaration on line 8, you inherit from the WebService class in that namespace. Next, you add <WebMethod()> to the functions you want to expose as services. Finally, in SelectSQL, you change the OleDbDataReader to a DataSet and change the OleDbCommand object into an OleDbDataAdapter. That's all there is to it. Recompile this file with the following command: | vbc /t:library/out:..\..\bin\TYASPNET.dll /r:System.dll /r:System.Data.Dll /r:System.Web.Services.dll /r:System.Xml.dll DatabaseService.vb ..\day15\User.vb This command compiles the new DatabaseService.vb file and User.vb from yesterday's lesson into the library file TYASPNET.dll. You also reference two new DLLs: System.Web.Services.dll and System.Xml.dll. The reason for referencing the first file is obvious; the second reference is included because it contains necessary methods to send the DataSet via XML. For more information on the VB.NET compiler, see yesterday's lesson, "Using Business Objects." The new objects should now be saved in the assembly cache and loaded from it. Let's create the .asmx file that will allow clients to access this object. See Listing 16.5. Listing 16.5 The .asmx File That Exposes Your Database Service 1 <%@ WebService %> That's all there is to it. You only have to change the class name to reference the database service you just compiled. Save this file as Database.asmx and request it from the browser. You should see the two exposed methods, SelectSQL and ExecuteNonQuery. Caution If you use a precompiled object for your Web service, it must be located in the assembly cache in order for ASP.NET to locate and use it. Click on the SelectSQL method and enter a SQL query in the test portion of the page: Select * FROM tblUsers. Then click the Invoke button. You should see something similar to Figure 16.8. Figure 16.8. The data returned from your database service.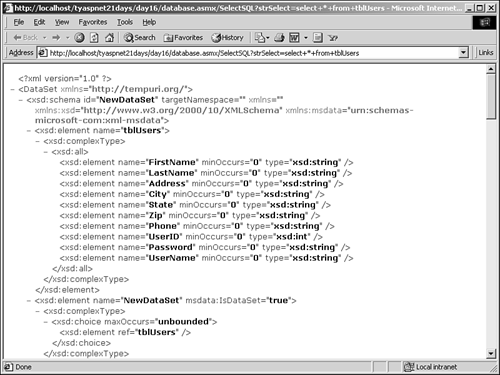 This XML document defines the structure of the DataSet your service returned. As you scroll down, you'll see the actual data values from the table. The client that receives this XML document can easily transform this object back into a DataSet if necessary. |